Fast Splittable Pseudorandom Number Generators
Total Page:16
File Type:pdf, Size:1020Kb
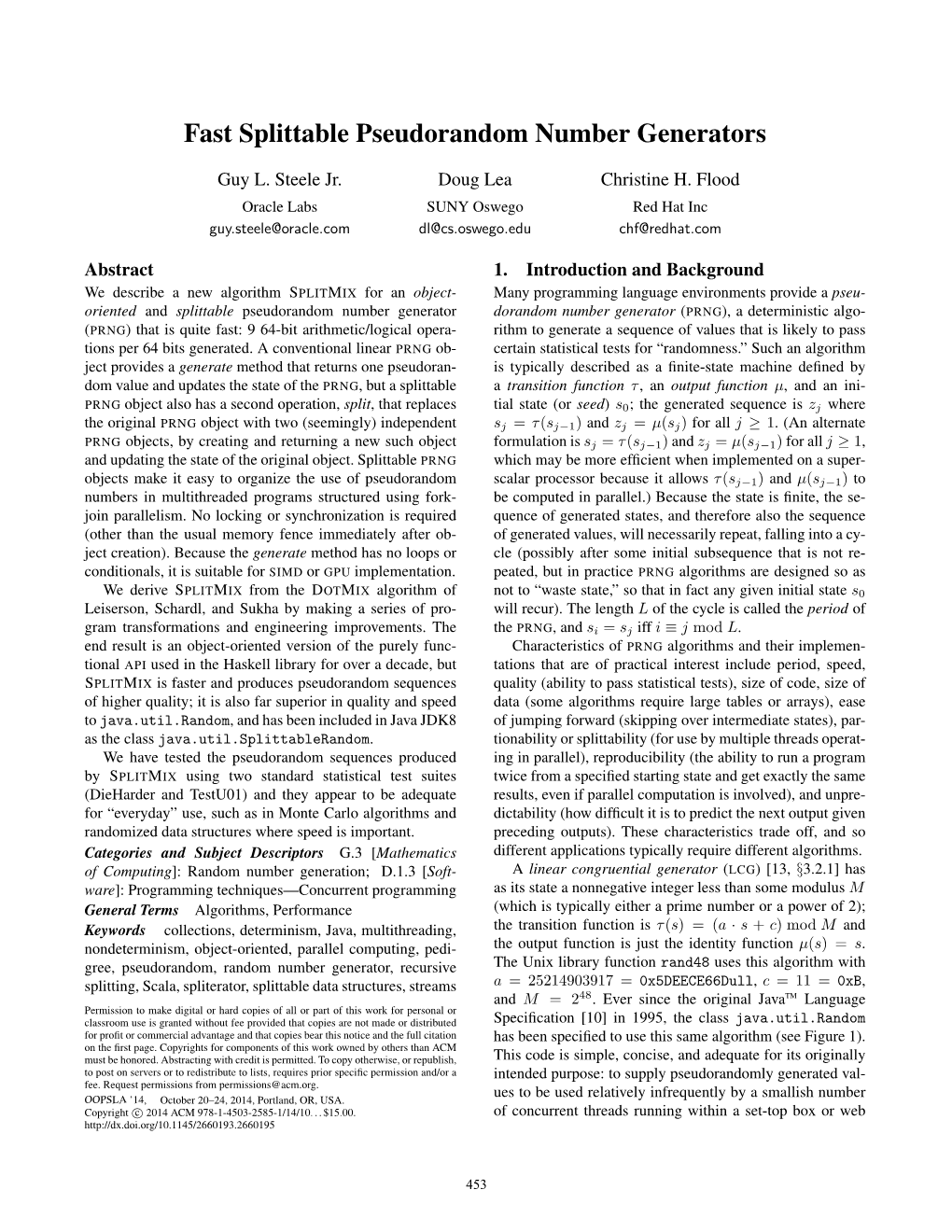
Load more
Recommended publications
-
Analisis Dan Perbandingan Berbagai Algoritma Pembangkit Bilangan Acak
Analisis dan Perbandingan berbagai Algoritma Pembangkit Bilangan Acak Micky Yudi Utama - 13514011 Program Studi Teknik Informatika Sekolah Teknik Elektro dan Informatika Institut Teknologi Bandung, Jl. Ganesha 10 Bandung 40132, Indonesia [email protected] Abstrak—Makalah ini bertujuan untuk membandingkan dan Terdapat banyak algoritma yang telah dibuat untuk menganalisis berbagai pembangkit bilangan acak yang sudah membangkitkan bilangan acak, seperti Mersenne Twister dan dibangun. Pembangkit bilangan acak memiliki peranan yang luas variannya, HC-256, ChaCha20, ISAAC64, PCG dan dalam berbagai bidang. Oleh karena itu, perlu diketahui variannya, xoroshiro128+, xorshift+, Random123, dll. Setiap kelebihan dan kekurangan dari setiap pembangkit bilangan acak, agar dapat ditentukan algoritma yang akan digunakan pada algoritma tentu memiliki kelebihan dan kelemahan domain tertentu. Algoritma yang akan dianalisis adalah masing-masing. Oleh karena itu, pada makalah ini, akan Mersenne Twister, xoroshiro128+, PCG, SplitMix, dan Lehmer. dilakukan eksperimen dan analisis terhadap beberapa algoritma Untuk masing-masing algoritma, akan dilakukan pengukuran PRNG. Algoritma yang dipilih adalah dua varian dari kualitas statistik dengan TestU01 dan kecepatannya. Selain itu, Mersenne Twister yakni MT19937 dan SFMT dikarenakan akan dianalisis kompleksitas, penggunaan memori, dan periode banyaknya penggunaan Mersenne Twister dalam berbagai dari masing-masing algoritma. aplikasi. Selain itu, dipilih juga xoroshiro128+ dan PCG yang dianggap sebagai dua algoritma terbaik saat ini. Kemudian, Kata kunci—Pembangkit bilangan acak, kelebihan, dipilih SplitMix sebagai salah satu algoritma yang cukup baru kekurangan, TestU01 dan terkenal. Yang terakhir, dipilih Lehmer64 yang merupakan I. PENDAHULUAN pengembangan dari LCG. Tujuan dari makalah ini adalah untuk mengetahui kelebihan Pembangkit bilangan acak merupakan salah satu aplikasi dan kekurangan dari masing-masing algoritma. -
CUDA Toolkit 4.2 CURAND Guide
CUDA Toolkit 4.2 CURAND Guide PG-05328-041_v01 | March 2012 Published by NVIDIA Corporation 2701 San Tomas Expressway Santa Clara, CA 95050 Notice ALL NVIDIA DESIGN SPECIFICATIONS, REFERENCE BOARDS, FILES, DRAWINGS, DIAGNOSTICS, LISTS, AND OTHER DOCUMENTS (TOGETHER AND SEPARATELY, "MATERIALS") ARE BEING PROVIDED "AS IS". NVIDIA MAKES NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. Information furnished is believed to be accurate and reliable. However, NVIDIA Corporation assumes no responsibility for the consequences of use of such information or for any infringement of patents or other rights of third parties that may result from its use. No license is granted by implication or otherwise under any patent or patent rights of NVIDIA Corporation. Specifications mentioned in this publication are subject to change without notice. This publication supersedes and replaces all information previously supplied. NVIDIA Corporation products are not authorized for use as critical components in life support devices or systems without express written approval of NVIDIA Corporation. Trademarks NVIDIA, CUDA, and the NVIDIA logo are trademarks or registered trademarks of NVIDIA Corporation in the United States and other countries. Other company and product names may be trademarks of the respective companies with which they are associated. Copyright Copyright ©2005-2012 by NVIDIA Corporation. All rights reserved. CUDA Toolkit 4.2 CURAND Guide PG-05328-041_v01 | 1 Portions of the MTGP32 (Mersenne Twister for GPU) library routines are subject to the following copyright: Copyright ©2009, 2010 Mutsuo Saito, Makoto Matsumoto and Hiroshima University. -
A Random Number Generator for Lightweight Authentication Protocols: Xorshiftr+
Turkish Journal of Electrical Engineering & Computer Sciences Turk J Elec Eng & Comp Sci (2017) 25: 4818 { 4828 http://journals.tubitak.gov.tr/elektrik/ ⃝c TUB¨ ITAK_ Research Article doi:10.3906/elk-1703-361 A random number generator for lightweight authentication protocols: xorshiftR+ Umut Can C¸ABUK, Omer¨ AYDIN∗, G¨okhanDALKILIC¸ Department of Computer Engineering, Faculty of Engineering, Dokuz Eyl¨ulUniversity, Izmir,_ Turkey Received: 30.03.2017 • Accepted/Published Online: 05.09.2017 • Final Version: 03.12.2017 Abstract: This paper presents the results of research that aims to find a suitable, reliable, and lightweight pseudorandom number generator for constrained devices used in the Internet of things. Within the study, three reduced versions of the xorshift+ generator are built. They are tested using the TestU01 suite as well as the NIST suite to measure their ability to produce randomness and performance values along with some other existing generators. The best of our reduced variations according to our tests, called the xorshiftR+, demonstrated great suitability for lightweight devices considering its randomness, performance, and resource usage. Key words: TestU01, xorshift, lightweight cryptography, Internet of things 1. Introduction The rapidly emerging concept of the Internet of things (IoT) brings new approaches to everyday problems as well as industrial applications. These approaches rely on bundles of cheap, efficient, and dedicated networked devices that work and communicate continuously. These so-called lightweight devices of the IoT have limited power, space, and computation resources; hence, there is a huge need for developing suitable security protocols and methodologies tailored for those. Furthermore, most of the contemporary security protocols are not optimized for lightweight environments and require more sources than IoT devices may efficiently provide. -
High-Performance Pseudo-Random Number Generation on Graphics Processing Units
High-Performance Pseudo-Random Number Generation on Graphics Processing Units Nimalan Nandapalan1, Richard P. Brent1;2, Lawrence M. Murray3, and Alistair Rendell1 1 Research School of Computer Science, The Australian National University 2 Mathematical Sciences Institute, The Australian National University 3 CSIRO Mathematics, Informatics and Statistics Abstract. This work considers the deployment of pseudo-random num- ber generators (PRNGs) on graphics processing units (GPUs), devel- oping an approach based on the xorgens generator to rapidly produce pseudo-random numbers of high statistical quality. The chosen algorithm has configurable state size and period, making it ideal for tuning to the GPU architecture. We present a comparison of both speed and statistical quality with other common parallel, GPU-based PRNGs, demonstrating favourable performance of the xorgens-based approach. Keywords: Pseudo-random number generation, graphics processing units, Monte Carlo 1 Introduction Motivated by compute-intense Monte Carlo methods, this work considers the tailoring of pseudo-random number generation (PRNG) algorithms to graph- ics processing units (GPUs). Monte Carlo methods of interest include Markov chain Monte Carlo (MCMC) [5], sequential Monte Carlo [4] and most recently, particle MCMC [1], with numerous applications across the physical, biological and environmental sciences. These methods demand large numbers of random variates of high statistical quality. We have observed in our own work that, after acceleration of other components of a Monte Carlo program on GPU [13, 14], the PRNG component, still executing on the CPU, can bottleneck the whole pro- cedure, failing to produce numbers as fast as the GPU can consume them. The aim, then, is to also accelerate the PRNG component on the GPU, without com- promising the statistical quality of the random number sequence, as demanded by the target Monte Carlo applications. -
Accelerating Probabilistic Computing with a Stochastic Processing Unit
Accelerating Probabilistic Computing with a Stochastic Processing Unit by Xiangyu Zhang Department of Electrical and Computer Engineering Duke University Date: Approved: Alvin R. Lebeck, Advisor Hai Li Sayan Mukherjee Daniel J. Sorin Lisa Wu Wills Dissertation submitted in partial fulfillment of the requirements for the degree of Doctor of Philosophy in the Department of Electrical and Computer Engineering in the Graduate School of Duke University 2020 Abstract Accelerating Probabilistic Computing with a Stochastic Processing Unit by Xiangyu Zhang Department of Electrical and Computer Engineering Duke University Date: Approved: Alvin R. Lebeck, Advisor Hai Li Sayan Mukherjee Daniel J. Sorin Lisa Wu Wills An abstract of a dissertation submitted in partial fulfillment of the requirements for the degree of Doctor of Philosophy in the Department of Electrical and Computer Engineering in the Graduate School of Duke University 2020 Copyright c 2020 by Xiangyu Zhang All rights reserved except the rights granted by the Creative Commons Attribution-Noncommercial Licence Abstract Statistical machine learning becomes a more important workload for computing sys- tems than ever before. Probabilistic computing is a popular approach in statistical machine learning, which solves problems by iteratively generating samples from pa- rameterized distributions. As an alternative to Deep Neural Networks, probabilistic computing provides conceptually simple, compositional, and interpretable models. However, probabilistic algorithms are often considered too slow on the conventional processors due to sampling overhead to 1) computing the parameters of a distribu- tion and 2) generating samples from the parameterized distribution. A specialized architecture is needed to address both the above aspects. In this dissertation, we claim a specialized architecture is necessary and feasible to efficiently support various probabilistic computing problems in statistical machine learning, while providing high-quality and robust results. -
Arxiv:1811.04035V1 [Cs.CR] 3 Nov 2018 Generated Random Numbers for Various Purposes
A Search for Good Pseudo-random Number Generators : Survey and Empirical Studies a a a, Kamalika Bhattacharjee , Krishnendu Maity , Sukanta Das ∗ aDepartment of Information Technology, Indian Institute of Engineering Science and Technology, Shibpur, West Bengal, India 711103 Abstract In today’s world, several applications demand numbers which appear random but are generated by a background algorithm; that is, pseudo-random num- bers. Since late 19th century, researchers have been working on pseudo-random number generators (PRNGs). Several PRNGs continue to develop, each one de- manding to be better than the previous ones. In this scenario, this paper targets to verify the claim of so-called good generators and rank the existing genera- tors based on strong empirical tests in same platforms. To do this, the genre of PRNGs developed so far has been explored and classified into three groups – linear congruential generator based, linear feedback shift register based and cellular automata based. From each group, well-known generators have been chosen for empirical testing. Two types of empirical testing has been done on each PRNG – blind statistical tests with Diehard battery of tests, TestU01 library and NIST statistical test-suite and graphical tests (lattice test and space- time diagram test). Finally, the selected 29 PRNGs are divided into 24 groups and are ranked according to their overall performance in all empirical tests. Keywords: Pseudo-random number generator (PRNG), Diehard, TestU01, NIST, Lattice Test, Space-time Diagram I. Introduction History of human race gives evidence that, since the ancient times, people has arXiv:1811.04035v1 [cs.CR] 3 Nov 2018 generated random numbers for various purposes. -
A Guideline on Pseudorandom Number Generation (PRNG) in the Iot
If you cite this paper, please use the ACM CSUR reference: P. Kietzmann, T. C. Schmidt, M. Wählisch. 2021. A Guideline on Pseudorandom Number Generation (PRNG) in the IoT. ACM Comput. Surv. 54, 6, Article 112 (July 2021), 38 pages. https://dl.acm.org/doi/10.1145/3453159 112 A Guideline on Pseudorandom Number Generation (PRNG) in the IoT PETER KIETZMANN and THOMAS C. SCHMIDT, HAW Hamburg, Germany MATTHIAS WÄHLISCH, Freie Universität Berlin, Germany Random numbers are an essential input to many functions on the Internet of Things (IoT). Common use cases of randomness range from low-level packet transmission to advanced algorithms of artificial intelligence as well as security and trust, which heavily rely on unpredictable random sources. In the constrained IoT, though, unpredictable random sources are a challenging desire due to limited resources, deterministic real-time operations, and frequent lack of a user interface. In this paper, we revisit the generation of randomness from the perspective of an IoT operating system (OS) that needs to support general purpose or crypto-secure random numbers. We analyse the potential attack surface, derive common requirements, and discuss the potentials and shortcomings of current IoT OSs. A systematic evaluation of current IoT hardware components and popular software generators based on well-established test suits and on experiments for measuring performance give rise to a set of clear recommendations on how to build such a random subsystem and which generators to use. CCS Concepts: • Computer systems organization → Embedded systems; • Mathematics of computing → Random number generation; • Software and its engineering → Operating systems; Additional Key Words and Phrases: Internet of Things, hardware random generator, cryptographically secure PRNG, physically unclonable function, statistical testing, performance evaluation, survey 1 INTRODUCTION Random numbers are essential in computer systems to enfold versatility and enable security. -
A PCG: a Family of Simple Fast Space-Efficient Statistically Good Algorithms for Random Number Generation
A PCG: A Family of Simple Fast Space-Efficient Statistically Good Algorithms for Random Number Generation MELISSA E. O’NEILL, Harvey Mudd College This paper presents a new uniform pseudorandom number generation scheme that is both extremely practical and statistically good (easily passing L’Ecuyer and Simard’s TestU01 suite). It has a number of important properties, including solid mathematical foundations, good time and space performance, small code size, multiple random streams, and better cryptographic properties than are typical for a general-purpose generator. The key idea is to pass the output of a fast well-understood “medium quality” random number generator to an efficient permutation function (a.k.a. hash function), built from composable primitives, that enhances the quality of the output. The algorithm can be applied at variety of bit sizes, including 64 and 128 bits (which provide 32- and 64-bit outputs, with periods of 264 and 2128). Optionally, we can provide each b-bit generator b 1 with a b 1 bit stream-selection constant, thereby providing 2 ¡ random streams, which are full period and ¡ b entirely distinct. An extension adds up to 2b-dimensional equidistribution for a total period of 2b2 . The construction of the permutation function and the period-extension technique are both founded on the idea of permutation functions on tuples. In its standard variants, b-bit generators use a 2b/2-to-1 function to produce b/2 bits of output. These functions can be designed to make it difficult for an adversary to discover the generator’s internal state by examining its output, and thus make it challenging to predict. -
Monte Carlo Automatic Integration with Dynamic Parallelism in CUDA
Monte Carlo Automatic Integration with Dynamic Parallelism in CUDA Elise de Doncker, John Kapenga and Rida Assaf Abstract The rapidly evolving CUDA environment is well suited for numerical in- tegration of high dimensional integrals, in particular by Monte Carlo or quasi-Monte Carlo methods. With some care, near peak performance can be obtained on impor- tant applications. A basis for efficient numerical integration using kernels in CUDA GPUs is presented, showing several ways to use CUDA features, provide automatic error control and prevention or detection of roundoff errors. This framework allows easy extension to multiple GPUs, clusters and clouds for addressing problems that were impractical to attack in the past, and is the basis for an update to the PARINT numerical integration package. 1 Introduction Problems in computational geometry, computational physics, computational fi- nance, and other fields give rise to computationally expensive integrals. This chap- ter will address applications of CUDA programming for Monte Carlo integration. A Monte Carlo method approximates the expected value of a stochastic process by sampling, i.e., by performing function evaluations over a large set of random points, and returns the sample average of the evaluations as the end result. The functions that are found in the application areas mentioned above are usually not smooth and may have singularities within the integration domain, which enforces the generation of large sets of random numbers. The algorithms described here will be incorporated in the PARINT multivariate integration package, where we are concerned with the automatic computation of an integral approximation Elise de Doncker, John Kapenga and Rida Assaf Western Michigan University, 1903 W. -
It Is High Time We Let Go of the Mersenne Twister∗
It Is High Time We Let Go Of The Mersenne Twister∗ SEBASTIANO VIGNA, Università degli Studi di Milano, Italy When the Mersenne Twister made his first appearance in 1997 it was a powerful example of how linear maps on F2 could be used to generate pseudorandom numbers. In particular, the easiness with which generators with long periods could be defined gave the Mersenne Twister a large following, in spite of the fact thatsuch long periods are not a measure of quality, and they require a large amount of memory. Even at the time of its publication, several defects of the Mersenne Twister were predictable, but they were somewhat obscured by other interesting properties. Today the Mersenne Twister is the default generator in C compilers, the Python language, the Maple mathematical computation system, and in many other environments. Nonetheless, knowledge accumulated in the last 20 years suggests that the Mersenne Twister has, in fact, severe defects, and should never be used as a general-purpose pseudorandom number generator. Many of these results are folklore, or are scattered through very specialized literature. This paper surveys these results for the non-specialist, providing new, simple, understandable examples, and it is intended as a guide for the final user, or for language implementors, so that they can take an informed decision about whether to use the Mersenne Twister or not. 1 INTRODUCTION “Mersenne Twister” [22] is the collective name of a family of PRNGs (pseudorandom number 1 generators) based on F2-linear maps. This means that the state of the generator is a vector of bits of size n interpreted as an n-dimensional vector on F2, the field with two elements, and the next-state function of the generator is an F2-linear map. -
Curand Library
cuRAND Library Programming Guide PG-05328-050_v11.4 | September 2021 Table of Contents Introduction.........................................................................................................................viii Chapter 1. Compatibility and Versioning..............................................................................1 Chapter 2. Host API Overview...............................................................................................2 2.1. Generator Types........................................................................................................................ 3 2.2. Generator Options..................................................................................................................... 3 2.2.1. Seed..................................................................................................................................... 3 2.2.2. Offset....................................................................................................................................3 2.2.3. Order....................................................................................................................................4 2.3. Return Values............................................................................................................................ 6 2.4. Generation Functions................................................................................................................ 7 2.5. Host API Example......................................................................................................................8 -
Survey on Hardware Implementation of Random
Survey on hardware implementation of random number generators on FPGA: Theory and experimental analyses Mohammed Bakiri, Christophe Guyeux, Jean Couchot, Abdelkrim Oudjida To cite this version: Mohammed Bakiri, Christophe Guyeux, Jean Couchot, Abdelkrim Oudjida. Survey on hardware im- plementation of random number generators on FPGA: Theory and experimental analyses. Computer Science Review, Elsevier, 2018, 27, pp.135 - 153. hal-02182827 HAL Id: hal-02182827 https://hal.archives-ouvertes.fr/hal-02182827 Submitted on 13 Jul 2019 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. Survey on Hardware Implementation of Random Number Generators on FPGA: Theory and Experimental Analyses Mohammed Bakiria,b,∗, Christophe Guyeuxa, Jean-Fran¸coisCouchota, Abdelkrim Kamel Oudjidab aFemto-ST Institute, DISC Department, UMR 6174 CNRS, University of Bourgogne Franche-Comt´e,Belfort, 90010, France bCentre de D´eveloppement des Technologies Avanc´ees,ASM/DMN Department, Cit 20 aot 1956 Baba Hassen, B. P 17,16303, Alger, Algeria Abstract Random number generation refers to many applications such as simulation, nu- merical analysis, cryptography etc. Field Programmable Gate Array (FPGA) are reconfigurable hardware systems, which allow rapid prototyping. This re- search work is the first comprehensive survey on how random number generators are implemented on Field Programmable Gate Arrays (FPGAs).