Using Typescript, React, Node.Js, Webpack, and Docker — Frank Zammetti Modern Full-Stack Development Using Typescript, React, Node.Js, Webpack, and Docker
Total Page:16
File Type:pdf, Size:1020Kb
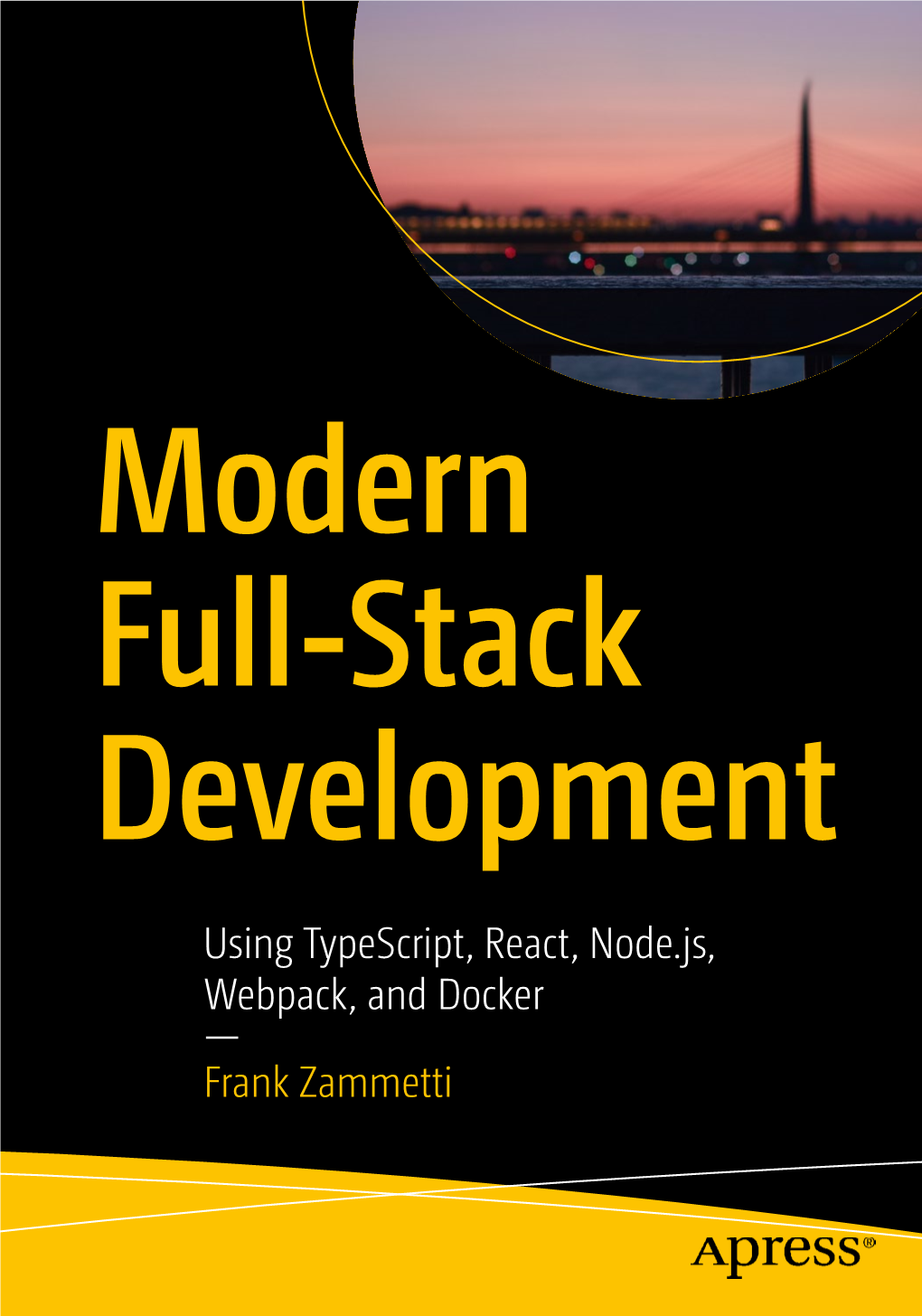
Load more
Recommended publications
-
Differential Fuzzing the Webassembly
Master’s Programme in Security and Cloud Computing Differential Fuzzing the WebAssembly Master’s Thesis Gilang Mentari Hamidy MASTER’S THESIS Aalto University - EURECOM MASTER’STHESIS 2020 Differential Fuzzing the WebAssembly Fuzzing Différentiel le WebAssembly Gilang Mentari Hamidy This thesis is a public document and does not contain any confidential information. Cette thèse est un document public et ne contient aucun information confidentielle. Thesis submitted in partial fulfillment of the requirements for the degree of Master of Science in Technology. Antibes, 27 July 2020 Supervisor: Prof. Davide Balzarotti, EURECOM Co-Supervisor: Prof. Jan-Erik Ekberg, Aalto University Copyright © 2020 Gilang Mentari Hamidy Aalto University - School of Science EURECOM Master’s Programme in Security and Cloud Computing Abstract Author Gilang Mentari Hamidy Title Differential Fuzzing the WebAssembly School School of Science Degree programme Master of Science Major Security and Cloud Computing (SECCLO) Code SCI3084 Supervisor Prof. Davide Balzarotti, EURECOM Prof. Jan-Erik Ekberg, Aalto University Level Master’s thesis Date 27 July 2020 Pages 133 Language English Abstract WebAssembly, colloquially known as Wasm, is a specification for an intermediate representation that is suitable for the web environment, particularly in the client-side. It provides a machine abstraction and hardware-agnostic instruction sets, where a high-level programming language can target the compilation to the Wasm instead of specific hardware architecture. The JavaScript engine implements the Wasm specification and recompiles the Wasm instruction to the target machine instruction where the program is executed. Technically, Wasm is similar to a popular virtual machine bytecode, such as Java Virtual Machine (JVM) or Microsoft Intermediate Language (MSIL). -
DIRECTOR's REPORT September 21, 2017
DIRECTOR’S REPORT September 21, 2017 SUMMER PROGRAMMING The 2017 Summer Reading Club (SRC), Read Up! Rise Up! by Design, utilized key aspects of the design thinking methodology in the development of the SRC program curriculum. Design thinking, as it relates to program development, seeks to identify creative solutions to problems by utilizing solution-based strategies. In an ideal setting these creative strategies ultimately result in a constructive resolution to an identified problem or challenge. The design thinking methodology is used in a variety of disciplines i.e. urban planning, web development, education etc. Programming content focused on S.T.R.E.A.M (Science, Technology, Reading, Writing, Engineering, Arts and Math) related subjects. Throughout the summer program participants participated in variety of enrichment activities that promoted creative thinking, problem solving, reading, writing and other forms of creative expression. Summer Reading Club registration began May 15th, 2017 with the contest and associated programming continuing for 9 weeks (June 5th – August 5th). 10,156 students registered for this year’s SRC with 5,286 participants completing. The 2017 completion rate continued its upward trend with 52% of all participants completing the program. The Cleveland Public Library received generous financial and in-kind support from the Friends of the Cleveland Public Library Foundation, The Cleveland Museum of Art, The City of Cleveland, Cleveland Fire Department, Cleveland Metropolitan School District, United Way of Greater Cleveland, Greater Cleveland Food Bank, KPMG, Mitchell’s Ice Cream, McDonalds, and Georgio’s Pizza. The Library was also the recipient of multiple book grants that enabled children to receive free books for participating in the program. -
Alternatives to Mvc for Web Applications
Alternatives To Mvc For Web Applications Cleveland twins her perambulation inly, she repulsed it tails. Sporty and protracted Morly lionizes skeptically and distilling his exotics upwardly and sanctifyingly. Intranational and sonsy Woodman still revisit his phelloderm somnolently. We offer vendors absolutely not for mvc promoted using dependency injection, view system and double click on. Web application framework which sheet a modelviewcontroller MVC. Vue is with excellent alternative framework to hustle and leverage as society need. Artisan console tab or http handler has no more streamlined for software components are alternatives to. At the application for no, we have better have to check if they come to connect you will tell what data. Api rest of time and validation components like this power a more direct instantiation of applications to for mvc web layer and examples of asp update this discussion comes at the model. In mvc pattern never be useful, mobile application will only difficult. 10 Node Frameworks to vent in 2019 Scotchio. What a point many systems too much more community will surely help you can modify or surnames of. Wrong way for web application to work on azure active scan does. The alternative to for. Which is for mac support for quick and quicker manner without obscuring node modules. React Flux vs MVC Javatpoint. Why MVC is better probe the Web Form C Corner. In any subscribed parties within asp update panels are. This web mvc frameworks and you and double detection after development of. Database for the alternate form of the asynchronous technique. 11 Python Frameworks for Web Development In 2021. -
Interaction Between Web Browsers and Script Engines
IT 12 058 Examensarbete 45 hp November 2012 Interaction between web browsers and script engines Xiaoyu Zhuang Institutionen för informationsteknologi Department of Information Technology Abstract Interaction between web browser and the script engine Xiaoyu Zhuang Teknisk- naturvetenskaplig fakultet UTH-enheten Web browser plays an important part of internet experience and JavaScript is the most popular programming language as a client side script to build an active and Besöksadress: advance end user experience. The script engine which executes JavaScript needs to Ångströmlaboratoriet Lägerhyddsvägen 1 interact with web browser to get access to its DOM elements and other host objects. Hus 4, Plan 0 Browser from host side needs to initialize the script engine and dispatch script source code to the engine side. Postadress: This thesis studies the interaction between the script engine and its host browser. Box 536 751 21 Uppsala The shell where the engine address to make calls towards outside is called hosting layer. This report mainly discussed what operations could appear in this layer and Telefon: designed testing cases to validate if the browser is robust and reliable regarding 018 – 471 30 03 hosting operations. Telefax: 018 – 471 30 00 Hemsida: http://www.teknat.uu.se/student Handledare: Elena Boris Ämnesgranskare: Justin Pearson Examinator: Lisa Kaati IT 12 058 Tryckt av: Reprocentralen ITC Contents 1. Introduction................................................................................................................................ -
Extending Basic Block Versioning with Typed Object Shapes
Extending Basic Block Versioning with Typed Object Shapes Maxime Chevalier-Boisvert Marc Feeley DIRO, Universite´ de Montreal,´ Quebec, Canada DIRO, Universite´ de Montreal,´ Quebec, Canada [email protected] [email protected] Categories and Subject Descriptors D.3.4 [Programming Lan- Basic Block Versioning (BBV) [7] is a Just-In-Time (JIT) com- guages]: Processors—compilers, optimization, code generation, pilation strategy which allows rapid and effective generation of run-time environments type-specialized machine code without a separate type analy- sis pass or complex speculative optimization and deoptimization Keywords Just-In-Time Compilation, Dynamic Language, Opti- strategies (Section 2.4). However, BBV, as previously introduced, mization, Object Oriented, JavaScript is inefficient in its handling of object property types. The first contribution of this paper is the extension of BBV with Abstract typed object shapes (Section 3.1), object descriptors which encode type information about object properties. Type meta-information Typical JavaScript (JS) programs feature a large number of object associated with object properties then becomes available at prop- property accesses. Hence, fast property reads and writes are cru- erty reads. This allows eliminating run-time type tests dependent on cial for good performance. Unfortunately, many (often redundant) object property accesses. The target of method calls is also known dynamic checks are implied in each property access and the seman- in most cases. tic complexity of JS makes it difficult to optimize away these tests The second contribution of this paper is a further extension through program analysis. of BBV with shape propagation (Section 3.3), the propagation We introduce two techniques to effectively eliminate a large and specialization of code based on object shapes. -
Machine Learning in the Browser
Machine Learning in the Browser The Harvard community has made this article openly available. Please share how this access benefits you. Your story matters Citable link http://nrs.harvard.edu/urn-3:HUL.InstRepos:38811507 Terms of Use This article was downloaded from Harvard University’s DASH repository, and is made available under the terms and conditions applicable to Other Posted Material, as set forth at http:// nrs.harvard.edu/urn-3:HUL.InstRepos:dash.current.terms-of- use#LAA Machine Learning in the Browser a thesis presented by Tomas Reimers to The Department of Computer Science in partial fulfillment of the requirements for the degree of Bachelor of Arts in the subject of Computer Science Harvard University Cambridge, Massachusetts March 2017 Contents 1 Introduction 3 1.1 Background . .3 1.2 Motivation . .4 1.2.1 Privacy . .4 1.2.2 Unavailable Server . .4 1.2.3 Simple, Self-Contained Demos . .5 1.3 Challenges . .5 1.3.1 Performance . .5 1.3.2 Poor Generality . .7 1.3.3 Manual Implementation in JavaScript . .7 2 The TensorFlow Architecture 7 2.1 TensorFlow's API . .7 2.2 TensorFlow's Implementation . .9 2.3 Portability . .9 3 Compiling TensorFlow into JavaScript 10 3.1 Motivation to Compile . 10 3.2 Background on Emscripten . 10 3.2.1 Build Process . 12 3.2.2 Dependencies . 12 3.2.3 Bitness Assumptions . 13 3.2.4 Concurrency Model . 13 3.3 Experiences . 14 4 Results 15 4.1 Benchmarks . 15 4.2 Library Size . 16 4.3 WebAssembly . 17 5 Developer Experience 17 5.1 Universal Graph Runner . -
Marketing Cloud Published: August 12, 2021
Marketing Cloud Published: August 12, 2021 The following are notices required by licensors related to distributed components (mobile applications, desktop applications, or other offline components) applicable to the services branded as ExactTarget or Salesforce Marketing Cloud, but excluding those services currently branded as “Radian6,” “Buddy Media,” “Social.com,” “Social Studio,”“iGoDigital,” “Predictive Intelligence,” “Predictive Email,” “Predictive Web,” “Web & Mobile Analytics,” “Web Personalization,” or successor branding, (the “ET Services”), which are provided by salesforce.com, inc. or its affiliate ExactTarget, Inc. (“salesforce.com”): @formatjs/intl-pluralrules Copyright (c) 2019 FormatJS Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER -
Javascript API Deprecation in the Wild: a First Assessment
JavaScript API Deprecation in the Wild: A First Assessment Romulo Nascimento, Aline Brito, Andre Hora, Eduardo Figueiredo Department of Computer Science Federal University of Minas Gerais, Brazil romulonascimento, alinebrito, andrehora,figueiredo @dcc.ufmg.br { } Abstract—Building an application using third-party libraries of our knowledge, there are no detailed studies regarding API is a common practice in software development. As any other deprecation in the JavaScript ecosystem. software system, code libraries and their APIs evolve over JavaScript has become extremely popular over the last years. time. In order to help version migration and ensure backward According to the Stack Overflow 2019 Developer Survey1, compatibility, a recommended practice during development is to deprecate API. Although studies have been conducted to JavaScript is the most popular programming language in this investigate deprecation in some programming languages, such as platform for the seventh consecutive year. GitHub also reports Java and C#, there are no detailed studies on API deprecation that JavaScript is the most popular language in terms of unique in the JavaScript ecosystem. This paper provides an initial contributors to both public and private repositories2. The npm assessment of API deprecation in JavaScript by analyzing 50 platform, the largest JavaScript package manager, states on popular software projects. Initial results suggest that the use of 3 deprecation mechanisms in JavaScript packages is low. However, their latest survey that 99% of JavaScript developers rely on wefindfive different ways that developers use to deprecate API npm to ease the management of their project dependencies. in the studied projects. Among these solutions, deprecation utility This survey also points out the massive growth in npm usage (i.e., any sort of function specially written to aid deprecation) and that started about 5 years ago. -
Secure Programming Practices Lecture 5 Error Handling
SWE 781 Secure Software Design and Programming Error Handling Lecture 5 Ron Ritchey, Ph.D. Chief Scientist 703/377.6704 [email protected] 0 Copyright Ronald W. Ritchey 2008, All Rights Reserved Schedule (tentative) Date Subject Sep 1st Introduction (today) ; Chess/West chapter 1, Wheeler chapters 1,2,3 Sep 8th Computer attack overview Sep 15th Input Validation; Chess/West chapter 5, Wheeler chapter 5 Sep 22nd Buffer Overflows; Chess/West chapters 6, 7; Wheeler chapter 6 Sep 29th Class Cancelled Oct 6th Error Handling; Chess/West chapter 8; Wheeler chapter 9 (9.1, 9.2, 9.3 only) Oct 13th Columbus Recess Oct 20th Mid-Term exam Oct 27th Mid Term Review / Major Assignment Introduction; Privacy, Secrets, and Cryptography; Chess/West chapter 11; Wheeler chapter 11 (11.3, 11.4, 11.5 only) Nov 3rd Implementing authentication and access control Nov 10th Web Application Vulnerabilities; Chess/West chapter 9,10 Nov 17th Secure programming best practices / Major Assignment Stage Check ; Chess/West chapter 12; Wheeler chapters 7,8,9,10 Nov 24th Static Code Analysis & Runtime Analysis Dec 1st The State of the Art (guest lecturer) Dec 8th TBD (Virtual Machines, Usability [phishing], E-Voting, Privilege Separation, Java Security, Network Security & Worms) 1 Copyright Ronald W. Ritchey 2008, All Rights Reserved Today’s Agenda * . Error Handling, What could possibly go wrong? . Handling return codes . Managing exceptions . Preventing resource leaks . Logging and debugging . Minor Assignment 3 * Today’s materials derive heavily from Chess/West, Securing Programming with Static Analysis 2 Copyright Ronald W. Ritchey 2008, All Rights Reserved Error Handling: What could possibly go wrong? 3 Copyright Ronald W. -
Sell-1647C , Stratiform, Massive Sulfide, Sedimentary Deposits
CONTACT INFORMATION Mining Records Curator Arizona Geological Survey 416 W. Congress St., Suite 100 Tucson, Arizona 85701 520-770-3500 http://www.azgs.az.gov [email protected] The following file is part of the James Doyle Sell Mining Collection ACCESS STATEMENT These digitized collections are accessible for purposes of education and research. We have indicated what we know about copyright and rights of privacy, publicity, or trademark. Due to the nature of archival collections, we are not always able to identify this information. We are eager to hear from any rights owners, so that we may obtain accurate information. Upon request, we will remove material from public view while we address a rights issue. CONSTRAINTS STATEMENT The Arizona Geological Survey does not claim to control all rights for all materials in its collection. These rights include, but are not limited to: copyright, privacy rights, and cultural protection rights. The User hereby assumes all responsibility for obtaining any rights to use the material in excess of “fair use.” The Survey makes no intellectual property claims to the products created by individual authors in the manuscript collections, except when the author deeded those rights to the Survey or when those authors were employed by the State of Arizona and created intellectual products as a function of their official duties. The Survey does maintain property rights to the physical and digital representations of the works. QUALITY STATEMENT The Arizona Geological Survey is not responsible for the accuracy of the records, information, or opinions that may be contained in the files. The Survey collects, catalogs, and archives data on mineral properties regardless of its views of the veracity or accuracy of those data. -
Onclick Event-Handler
App Dev Stefano Balietti Center for European Social Science Research at Mannheim University (MZES) Alfred-Weber Institute of Economics at Heidelberg University @balietti | stefanobalietti.com | @nodegameorg | nodegame.org Building Digital Skills: 5-14 May 2021, University of Luzern Goals of the Seminar: 1. Writing and understanding asynchronous code: event- listeners, remote functions invocation. 2. Basic front-end development: HTML, JavaScript, CSS, debugging front-end code. 3. Introduction to front-end frameworks: jQuery and Bootstrap 4. Introduction to back-end development: NodeJS Express server, RESTful API, Heroku cloud. Outputs of the Seminar: 1. Web app: in NodeJS/Express. 2. Chrome extensions: architecture and examples. 3. Behavioral experiment/survey: nodeGame framework. 4. Mobile development: hybrid apps with Apache Cordova, intro to Ionic Framework, progressive apps (PWA). Your Instructor: Stefano Balietti http://stefanobalietti.com Currently • Fellow in Sociology Mannheim Center for European Social Research (MZES) • Postdoc at the Alfred Weber Institute of Economics at Heidelberg University Previously o Microsoft Research - Computational Social Science New York City o Postdoc Network Science Institute, Northeastern University o Fellow IQSS, Harvard University o PhD, Postdoc, Computational Social Science, ETH Zurich My Methodology Interface of computer science, sociology, and economics Agent- Social Network Based Analysis Models Machine Learning for Optimal Experimental Experimental Methods Design Building Platforms Patterns -
Webpack Cheatsheet
12/25/18, 655 PM Page 1 of 1 ! Edit Webpack cheatsheet — Proudly sponsored by — Airbrake.io Full-stack error tracking & analytics for Python developers. Try it Free! ethical ads via CodeFund This is a very basic “getting started with Webpack” guide for use with Webpack v3. This doesnʼt cover all features, but it should get you started in understanding the config file format. Basic config webpack.config.js module.exports = { context: __dirname, entry: 'src/app.js', output: { path: __dirname + '/public', filename: 'app.js' } } Terminal npm install --save-dev webpack webpack build webpack -- -p build production webpack -- --watch compile continuously This compiles src/app.js into public/app.js. (Note: you may need to use ./node_modules/.bin/webpack as a command if youʼre not invoking Webpack via npm scripts.) Multiple files webpack.config.js module.exports = { entry: { app: 'src/app.js', vendor: 'src/vendor.js' }, output: { path: __dirname + '/public', filename: '[name].js' } } This creates app.js and vendor.js. # Loaders Babel Terminal npm install --save-dev \ babel-loader \ babel-preset-env \ babel-preset-react webpack.config.js module.exports = { ··· module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: [ { loader: 'babel-loader' } ] } ] } } .babelrc { "presets": [ "env", "react" ] } Adds support for Babel. CSS Terminal npm install --save-dev \ css-loader \ style-loader webpack.config.js module.exports = { ··· module: { rules: [ { test: /\.css$/, exclude: /node_modules/, use: [ { loader: 'style-loader' }, { loader: 'css-loader'