Starting out with App Inventor for Android Tony Gaddis • Rebecca Halsey This Page Intentionally Left Blank
Total Page:16
File Type:pdf, Size:1020Kb
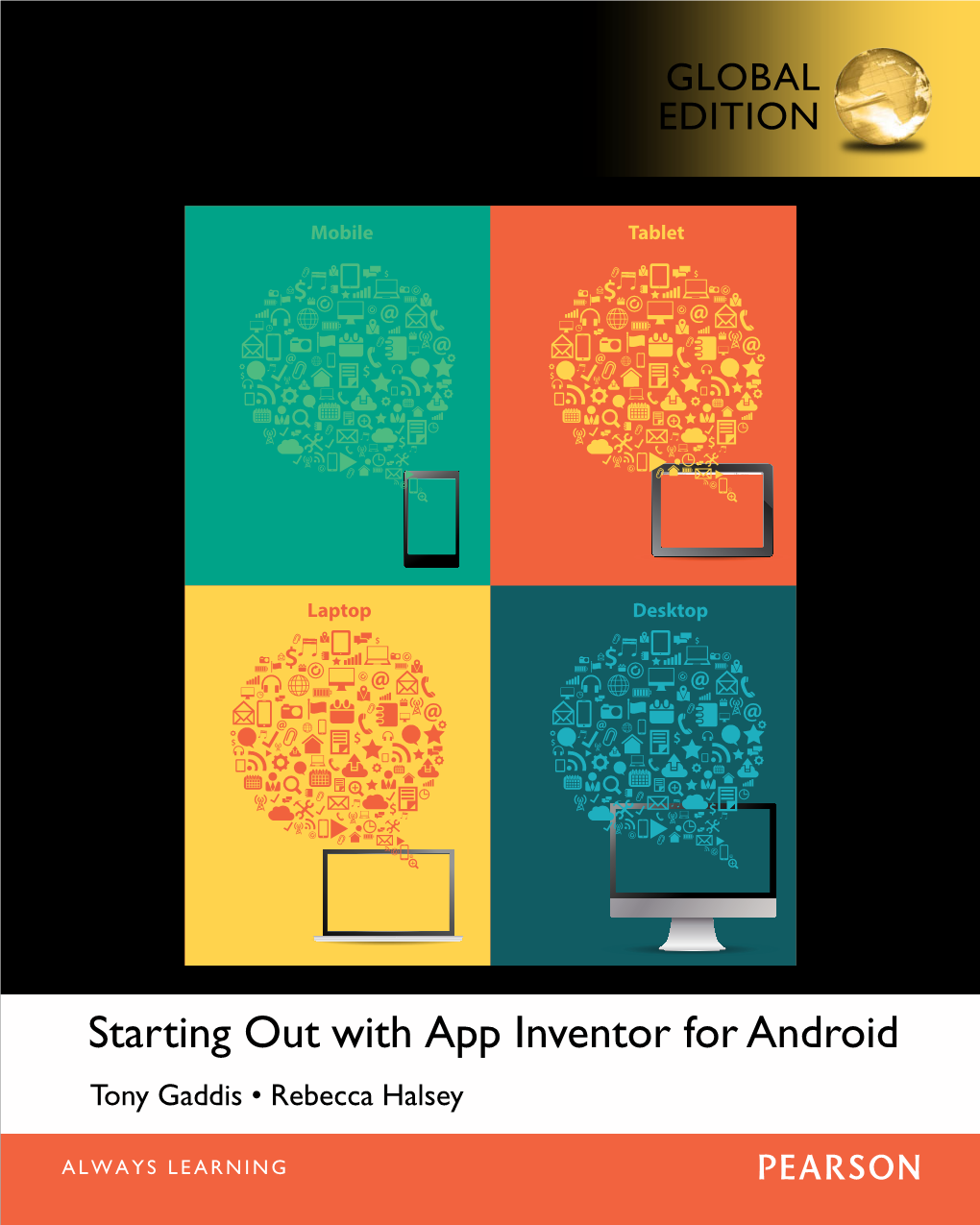
Load more
Recommended publications
-
Android (Operating System) 1 Android (Operating System)
Android (operating system) 1 Android (operating system) Android Home screen displayed by Samsung Nexus S with Google running Android 2.3 "Gingerbread" Company / developer Google Inc., Open Handset Alliance [1] Programmed in C (core), C++ (some third-party libraries), Java (UI) Working state Current [2] Source model Free and open source software (3.0 is currently in closed development) Initial release 21 October 2008 Latest stable release Tablets: [3] 3.0.1 (Honeycomb) Phones: [3] 2.3.3 (Gingerbread) / 24 February 2011 [4] Supported platforms ARM, MIPS, Power, x86 Kernel type Monolithic, modified Linux kernel Default user interface Graphical [5] License Apache 2.0, Linux kernel patches are under GPL v2 Official website [www.android.com www.android.com] Android is a software stack for mobile devices that includes an operating system, middleware and key applications.[6] [7] Google Inc. purchased the initial developer of the software, Android Inc., in 2005.[8] Android's mobile operating system is based on a modified version of the Linux kernel. Google and other members of the Open Handset Alliance collaborated on Android's development and release.[9] [10] The Android Open Source Project (AOSP) is tasked with the maintenance and further development of Android.[11] The Android operating system is the world's best-selling Smartphone platform.[12] [13] Android has a large community of developers writing applications ("apps") that extend the functionality of the devices. There are currently over 150,000 apps available for Android.[14] [15] Android Market is the online app store run by Google, though apps can also be downloaded from third-party sites. -
UNIT – I - SIT1402 - Mobile Application Development
SCHOOL OF COMPUTING DEPARTMENT OF COMPUTER SCIENCE & ENGINEERING UNIT – I - SIT1402 - Mobile Application Development Introduction and UI interface • 1. Introduction to mobile technologies • 2. Mobile operating systems • 3. Mobile devices – pros and cons • 4. Introduction to Android, Versions,Features • 5. Android architecture • 6. UI Layouts • 7. UI Controls / Widgets • 8. Event handling • 9. Required Tools- Eclipse, ADT, AVD • 10. Application structure • 11. Android manifest file • 12. Android design philosophy 2 • 13. Creating andriod applications Mobile Networks / Technologies GSM GPRS EDGE 1G, 2G, 3G, 4G, 5G IEEE 802.11 Infrared Bluetooth Cellular Network • Base stations transmit to and receive from mobiles at the assigned spectrum – Multiple base stations use the same spectrum (spectral reuse) • The service area of each base station is called a cell • Each mobile terminal is typically served by the ‘closest’ base stations – Handoff when terminals move Cellular Network Generations • It is useful to think of cellular Network/telephony in terms of generations: – 0G: Briefcase-size mobile radio telephones – 1G: Analog cellular telephony – 2G: Digital cellular telephony – 3G: High-speed digital cellular telephony (including video telephony) – 4G: IP-based “anytime, anywhere” voice, data, and multimedia telephony at faster data rates than 3G (to be deployed in 2012–2015) Frequency Division Multiple Access • Each mobile is assigned a separate frequency channel for the duration of the call • Sufficient guard band is required to prevent adjacent channel interference • Usually, mobile terminals will have one downlink frequency band and one uplink frequency band • Different cellular network protocols use different frequencies • Frequency is a precious and scare resource. We are running out of it. -
Term Paper Google Labs, Fascinating Examples
Term paper Google Labs, fascinating examples Winter Semester 2011/2012 Submitted to Prof. Dr. Eduard Heindl Prepared by Yongwoo Kim Fakultät Wirtschaftsinformatik Hochschule Furtwangen University <References> ¥ ¡ ¢ £ ¤ ¦ § ¨ © © © $ $ ! " % # l / 1 1 222 $ $ 1 © © © ¡ - © 0 )% )) % ) " )) ! 3 ' '( # ' . *+ , l & © © / © 6 2 © 6 © 6 - ! " !% % % 7% 7 " % % )7 ' # 5' 5 ' 3 ' . 8 , , 4 4 l 4 : 62 : / 11 / 6 $ 1 / - © !% % %7% ! 0 ) %) ! ) " ! ' ' # # # ' ' . 9 # * * * * * , 6 6 7 " % !%)7 '' 3 ' . * * *; ++< $ $ / 1 1 ! ! ! 0 . # . # l ## $ 222 $ ! ' l # # 222 $2 6 $ %7 % % # l ' <Table of contents> 1. Introduction 2. Various Projects of Google Labs 2.1. Fast Flip 2.2. Earth Engine 2.3. Scribe 2.4. Follow Finder 2.5. Apps for Android 3. Art Project 3.1. Zooming in High-Level Detail View 3.2. Inside the Museums View 3.3. Creating Individual Collection 4. Strategy and the Future of Google 1. Introduction Since a few years, Google has become perhaps a single vision of the Internet – from Google search and YouTube to Gmail and Android phones. When most people, including me and you, use the Internet, they normally use Google to search for information on a regular basis. But you probably don't exactly know about all of the interesting things going on at Google that is available to you online. To keep people in the Google way of life, the company constantly launches new services. In fact, Google has an official "20 percent" rule that asks every employee to spend "one day a week working on projects that aren't necessarily in our job descriptions." These extracurricular experiments lived at GoogleLabs.com where anyone could try out the almost-finished projects. -
App Inventor Create Your Own Android Apps
App Inventor Create Your Own Android Apps App Inventor Create Your Own Android Apps David Wolber, Hal Abelson, Ellen Spertus & Liz Looney Beijing · Cambridge · Farnham · Köln · Sebastopol · Tokyo App Inventor by David Wolber, Hal Abelson, Ellen Spertus & Liz Looney Copyright © 2011 David Wolber, Hal Abelson, Ellen Spertus & Liz Looney. All rights reserved. Printed in Canada. Published by O’Reilly Media, Inc., 1005 Gravenstein Highway North, Sebastopol, CA 95472. O’Reilly books may be purchased for educational, business, or sales promotional use. Online editions are also available for most titles (safari.oreilly.com). For more information, contact our corporate/insti- tutional sales department: 800-998-9938 or [email protected]. Editors: Courtney Nash and Brian Jepson Indexer: Denise Getz Production Editor: Holly Bauer Cover Designer: Mark Paglietti Copyeditor: Rachel Monaghan Interior Designer: Ron Bilodeau Proofreader: Holly Bauer Illustrator: Robert Romano Printing History: April 2011: First Edition. Nutshell Handbook, the Nutshell Handbook logo, and the O’Reilly logo are registered trademarks of O’Reilly Media, Inc. App Inventor and related trade dress are trademarks of O’Reilly Media, Inc. Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in this book, and O’Reilly Media, Inc., was aware of a trademark claim, the designations have been printed in caps or initial caps. While every precaution has been taken in the preparation of this book, the publisher and authors assume no responsibility for errors or omissions, or for damages resulting from the use of the informa- tion contained herein. 978-1-4493-9748-7 [TI] For Tomás, who reinvents me every day. -
App Inventor for Android
01_9781119991335-ffirs.indd v 3/28/11 12:44 PM App Inventor for Android: Build Your Own Apps — No Experience Required! 01_9781119991335-ffirs.indd i 3/28/11 12:44 PM 01_9781119991335-ffirs.indd ii 3/28/11 12:44 PM App Inventor for Android: Build Your Own Apps — No Experience Required! Jason Tyler A John Wiley and Sons, Ltd, Publication 01_9781119991335-ffirs.indd iii 3/28/11 12:44 PM App Inventor for Android: Build Your Own Apps — No Experience Required! Th is edition fi rst published 2011 © 2011 John Wiley & Sons, Ltd Registered offi ce John Wiley & Sons Ltd, Th e Atrium, Southern Gate, Chichester, West Sussex, PO19 8SQ, United Kingdom For details of our global editorial offi ces, for customer services and for information about how to apply for permission to reuse the copyright material in this book, please see our Web site at www.wiley.com. Th e right of the author to be identifi ed as the author of this work has been asserted in accordance with the Copyright, Designs and Patents Act 1988. All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by any means, electronic, mechanical, photocopying, recording or otherwise, except as permitted by the UK Copyright, Designs and Patents Act 1988, without the prior permission of the publisher. GOOGLE is a trademark of Google Inc. Th e Android Robot is created and shared by Google and used according to terms described in the Creative Commons 3.0 Attribution License located at http://creativecommons.org/licenses/by/3.0/. -
2011 ATTW Bibliography
2011ATTW Bibliography Page 1 of 109 2011 ATTW Bibliography Paul R. Sawyer, Editor, Southeastern Louisiana University Dianna Laurent, Associate Editor, Southeastern Louisiana University Deborah Balzhiser, Texas State University Michael J. K. Bokor, Long Island University, Brooklyn Campus Darren Elzie, University of Memphis Lyn F. Gattis, Missouri State University Morgan Gresham, University of South Florida, St. Petersburg Jeffrey Rice, Western Kentucky University BOOKS General Bai, Yong and Qiang Bai. Subsea Structural Engineering Handbook. Burlington, MA: Gulf Professional, 2011. Boje, David M. Storytelling and the Future of Organizations: An Antenarrative Handbook. New York: Routledge, 2011. Burkett, John Walt. Aristotle, Rhetoric III: A Commentary. Fort Worth, TX: Texas Christian University, 2011. Butler, Shane. The Matter of the Page: Essays in Search of Ancient and Medieval Authors. Madison: U of Wisconsin P, 2011. Carroll, Evan and John Romano. Your Digital Afterlife: When Facebook, Flickr and Twitter are Your Estate, What’s Your Legacy? Berkeley, CA: New Riders, 2011. 2011ATTW Bibliography Page 2 of 109 Clark, Robert M. The Technical Collection of Intelligence. Washington, DC: CQ P, 2011. Creswell, John W. Research Design: Qualitative, Quantitative, and Mixed Methods Approaches. New Delhi: Sage, 2011. Cummins, Tim, Mark David, Katherine Kawamoto, and International Association for Contract and Commercial Management. Contract and Commercial Management: The Operational Guide. Netherlands: Van Haren, 2011. Ermolinskiy, Andrey and Scott Shenker. Design and Implementation of a Hypervisor- Based Platform for Dynamic Information Flow Tracking in a Distributed Environment. Berkeley, CA: EECS Department, University of California, 2011. French, Derek, Stephen W. Mayson, and Christopher L. Ryan. Mayson, French & Ryan on Company Law: 2011–2012. -
Universidad Veracruzana
UNIVERSIDAD VERACRUZANA FACULTAD DE INSTRUMENTACION´ ELECTRONICA´ Sistema de Portero Electr´onico Utilizando la Tarjeta de Desarrollo Pandaboard y Comunicaci´on con Smartphones TESIS Que para obtener el grado de Maestro en Ingenier´ıa Electr´onica y Computaci´on Presenta Abel Porfirio Ochoa Aguilar Director de la tesis: Dr. Agust´ın Gallardo del Angel´ Co-Director de la tesis: Ph.D(C) Jorge Eduardo P´erez-J´acome Friscione Xalapa-Enr´ıquez, Ver. Enero, 2014 ii iv Agradecimientos Cuando decides algo de forma consciente por mucho tiempo, esa idea se graba en el subconsciente, s´ıa esa idea le das fuerza con fe, esfuerzo y dedicaci´on, el universo guiar´atu camino hasta conseguirlo; a tu alrededor coincidir´as con personas que apoyaran tu esfuerzo, que allanar´an tu camino y te ayudar´an a superar los obst´aculos. A pesar de los tropiezos, vivencias y experiencias adquiridas durante el tiempo de mis estudios en la maestr´ıa, me d´ıcuenta que existen personas que sin conocerte, pueden extenderte la mano sin pedir nada a cambio; en algunos de esos momentos cre´ı no merecerlo, le pido a Dios que todo lo bueno que me dieron se les multiplique; en esta etapa de mi vida s´ıalgo he aprendido es por lo menos a dar las gracias de todo coraz´on. Mis padres me ense˜naron a tener fe, a no bajar los brazos ni la cabeza a pesar de las situaciones, a aceptar que a veces se requiere pedir ayuda y no or eso perdemos dignidad, sobre todo a dar gracias por la misma. -
Design of a Smart Greenhouse Adaptation and Control Irrigation System Based on Arduino and Android Application
ALNEELAIN UNIVERSITY FACULTY OF ENGINEERING M.Sc. OF EMBEDDED SYSTEMS Design of a Smart Greenhouse Adaptation and Control Irrigation System based on Arduino and Android Application Thesis Submitted of Partial Fulfillment for M.Sc. Degree in Embedded Systems Prepared by: Motaz Alhassin Alhassan Abdalrahman Supervisor: Prof. Shareif Fadul Babikir February 2020 I اﻻستهﻻل بسى اهلل انشحًٍ انشحٍى قبل حعبنى : ﴿ نَرْفَعُ دَرَجَاتٍ مَّن نَّشَاءُ ۗ وَفَىْقَ كُلِّ ذِي عِلْمٍ عَلِيمٌ ﴾ سىرة يىسف - اﻵية )67( I DEDICATION I am very grateful to Almighty Allah for helping me through this long journey, May He continues to bless, help and guide us to the right path. I dedicate this project to all those that helped me toward this success, specially my parents, my families, my teachers and my colleagues. And do not forget also those who departed from our world of teachers. Thank you all… II ACKNOWLEDGEMENTS I would like to express my gratitude and appreciation to all those who gave me the possibility to complete this project. A special thanks to my supervisor Prof. Sharief Fadul Babikir, who helped me, stimulating suggestions and encouragement, help gratitude to coordinate my project and writing this Thesis. III ABSTRACT Greenhouse is a kind of place which can change growth environment of plants, create the best conditions for plant growth, and avoid influence on plant growth due to outside changing. In this thesis, Android and Arduino based system applied to monitor and control greenhouse irrigation, temperature and humidity designed. The main objective of thesis to afford a cheap technology to control agriculture process. The methodology followed for show the results and control the system by using low power consumption Arduino kit and Bluetooth module. -
Gsm Based Home Automation System Using App-Inventor for Android Mobile Phone
R.Harinath et al, International Journal of Computer Science and Mobile Computing, Vol.4 Issue.4, April- 2015, pg. 158-167 Available Online at www.ijcsmc.com International Journal of Computer Science and Mobile Computing A Monthly Journal of Computer Science and Information Technology ISSN 2320–088X IJCSMC, Vol. 4, Issue. 4, April 2015, pg.158 – 167 RESEARCH ARTICLE GSM BASED HOME AUTOMATION SYSTEM USING APP-INVENTOR FOR ANDROID MOBILE PHONE R.HARINATH, M.E., CSE (PT), SCSVMV, KANCHIPURAM, [email protected] Dr. S. Santhi, M.E., Ph.D, Associate Professor, SCSVMV, [email protected] ABSTRACT: Nowadays, the remote Home Automation turns out to be more and more significant and appealing. It improves the value of our lives by automating various electrical appliances or instruments. This paper describes GSM (Global System Messaging) based secured device control system using App Inventor for Android mobile phones. App Inventor is a latest visual programming platform for developing mobile applications for Android-based smart phones. The Android Mobile Phone Platform becomes more and more popular among software developers, because of its powerful capabilities and open architecture. It is a fantastic platform for the real world interface control, as it offers an ample of resources and already incorporates a lot of sensors. No need to write programming codes to develop apps in the App Inventor, instead it provides visual design interface as the way the apps looks and use blocks of interlocking components to control the app’s behaviour. The App Inventor aims to make programming enjoyable and accessible to novices. KEYWORDS: GSM, App Inventor, Home Automation, Android, Mobile Phone, Short Messaging Service (SMS) I. -
Mobile App Development Tutor Pack
I ♥ My Smartphone A Computing Science Course in Mobile App Development by Jeremy Scott TUTOR NOTES Version 2, August 2016 I ♥ My Smartphone Acknowledgements Acknowledgements This resource was partially funded by a grant from Education Scotland. We are also grateful for the help and support provided by the following contributors: Bridge of Don Academy Crieff High School George Heriot’s School Johnstone High School Kelso High School CompEdNet, Scottish Forum for Computing Science Teachers Computing At School Council of Professors and Heads of Computing (CPHC) Professor Hal Abelson, MIT Mitchel Resnick, MIT Scottish Informatics and Computer Science Alliance (SICSA) Edinburgh Napier University School of Computing Glasgow Caledonian University School of Engineering and Built Environment Heriot-Watt University School of Mathematical and Computer Sciences Robert Gordon University School of Computing University of Edinburgh School of Informatics University of Aberdeen Department of Computing University of Dundee School of Computing University of Glasgow School of Computing Science University of St Andrews School of Computer Science University of Stirling Department of Computing Science and Mathematics University of Strathclyde Department of Computer and Information Sciences University of the West of Scotland School of Computing ScotlandIS 4J Studios Brightsolid Google JP Morgan Microsoft Research Oracle O2 RunRev Sword Ciboodle Special thanks go to Ian King who assisted with updating these materials for App Inventor 2. The contribution of the following individuals who served on the RSE/BCS Project Advisory Group is also gratefully acknowledged: Professor Sally Brown (chair), Mr David Bethune, Professor Alan Bundy, Professor Quintin Cutts, Ms Kate Farrell, Mr William Hardie, Dr Fiona McNeill, Professor Greg Michaelson, Dr Bill Mitchell and Professor Judy Robertson. -
Control Language Programming for the AS/400
Control Language Programming for the AS/400; Essentials of Subfile Programming and Advanced Fortress Rochester: The Inside Story of the IBM Second Edition Topics in RPG IV iSeries ILE by Example Introduction to AS/400 System Operations; Implementing AS/400 Security; Fourth Edition Second Edition Java and the AS/400: Practical Examples for the Mastering the AS/400: A Practical; Hands-On iSeries and AS400; Second Edition Guide; Third Edition OPNQRYF By Example Programming in RPG IV; Third Edition RPG IV Jump Start: Your Guide to the New RPG; RPG TnT: 101 Dynamite Tips 'n Techniques with Fourth Edition RPG IV SQL/400 Developer's Guide Essentials of Interactive Computer Graphics: Starter Kit for the IBM iSeries & AS/400 Concepts and Implementation Fundamentals of Computer Graphics; Second Edition Machines Who Think: A Personal Inquiry into the History and Prospects of Artificial Intelligence Real Sound Synthesis for Interactive Applications Real-Time Rendering; Third Edition 3G Evolution: HSPA and LTE for Mobile 4G; LTE Evolution and the Road to 5G; Third Broadband Edition 4G: LTE/LTE-Advanced for Mobile Broadband; Advances in Computers: Dataflow Processing; Second Edition Advances in Computers; Volume Ninety-Seven Volume Ninety Six Advances in Computers: Energy Efficiency in Data Centers and Clouds; Volume One Hundred Bent Functions: Results and Applications to Computer and Machine Vision: Theory; Cryptography Algorithms; Practicalities; Fourth Edition Data Mining Applications with R Digital Evidence and Computer Crime: Forensic Science; -
Teaching Computational Thinking Through Mobile Apps
Teaching Computational Thinking Through Mobile Apps Workshop July 19-20, 2016 Richard Lucic - [email protected] Susan Rodger - [email protected] Student Assistants: Ademola Olayinka Carolyn Sun Anh Trinh Sponsored by IBM Workshop Overview • Day 1 - Welcome/Introductions - Workshop Overview/App Demo - App Inventor 2 / IBM Cloud Tools - App 1 & 2 Labs - Discussion: Using App Inventor to teach CS Principles Workshop Overview • Day 2 - App 3 Lab - Discussion-continued: Using App Inventor to teach CS Principles - App 3 - Wrap-up Discussion Introductions • Where are you from? • What do you teach? • Why did you enroll in this workshop? • Your favorite App, and what do you like about it? What is This Workshop About? • How do mobile apps work? Programming - Lab Projects (3) • What can be created using methodologies from computing and computer science? Creativity Workshop Goals • Explore the context for mobile computing • Learn the fundamentals of coding mobile apps • Understand the app development workflow (tools such as App Inventor & other resources) • Gain proficiency in the foundations of user interface design • Examine the app distribution process • Explore the use of App Inventor in CS Education Have Fun! Workshop Documents • http://bit.ly/29P1Pcd I ❤ Candy Demo I ❤ Candy Workflow • Text my mobile phone “I ❤ Candy” • My phone receives text and stores your phone number in a data list • When I press the button in my I ❤ Candy app, the app randomly picks a phone number from the list and places a call to the winner AI2 Program Text… I Candy! To… (919) 908-4105 App Inventor for Android Do-it-yourself App Creation Developing Mobile Apps • iPhone – Swift/Objective C – High level tools to turn pre-existing content into an app.