Object Persistence with Ruby and Smalltalk BP2012H1
Total Page:16
File Type:pdf, Size:1020Kb
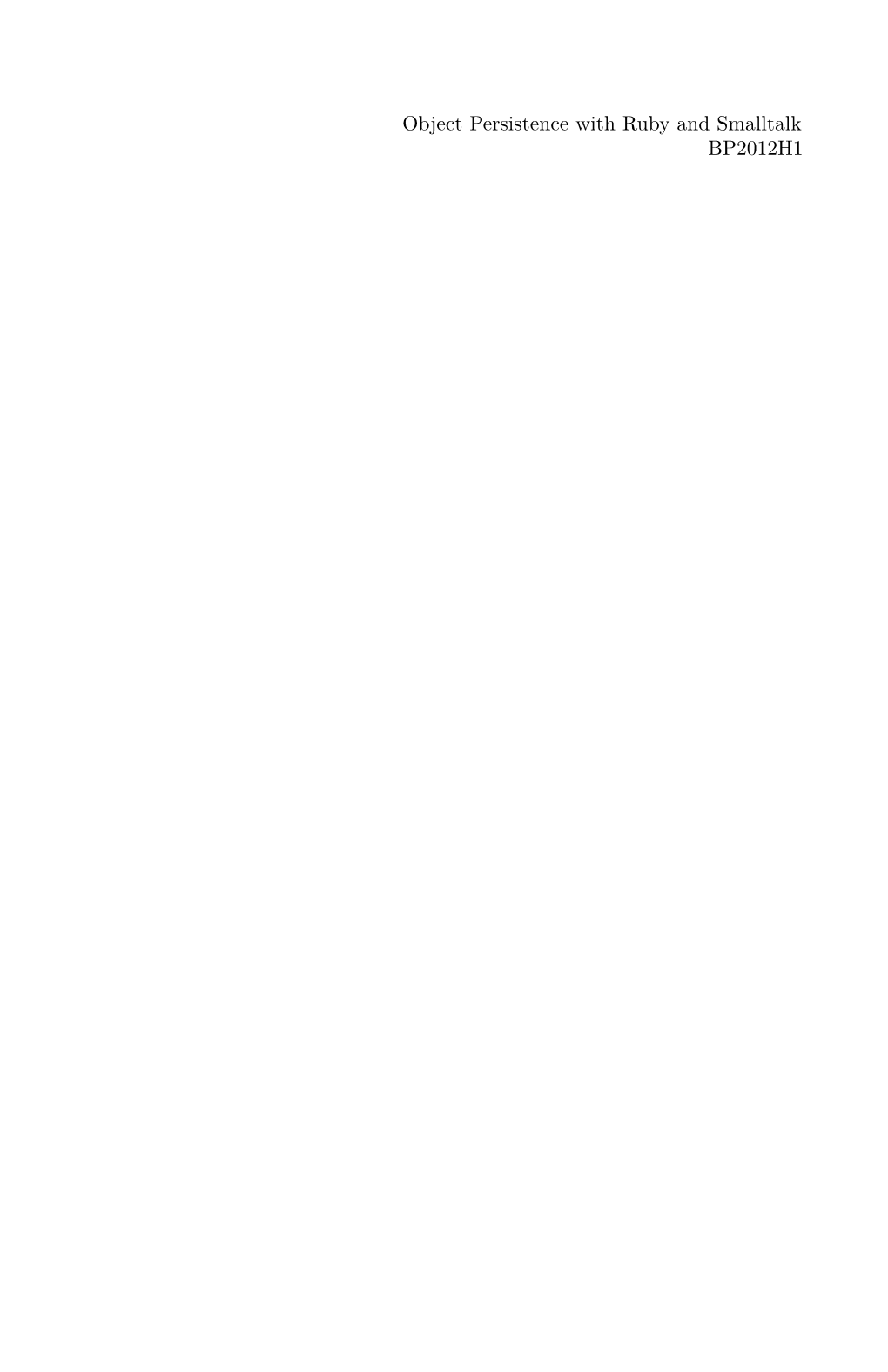
Load more
Recommended publications
-
The Future: the Story of Squeak, a Practical Smalltalk Written in Itself
Back to the future: the story of Squeak, a practical Smalltalk written in itself Dan Ingalls, Ted Kaehler, John Maloney, Scott Wallace, and Alan Kay [Also published in OOPSLA ’97: Proc. of the 12th ACM SIGPLAN Conference on Object-oriented Programming, 1997, pp. 318-326.] VPRI Technical Report TR-1997-001 Viewpoints Research Institute, 1209 Grand Central Avenue, Glendale, CA 91201 t: (818) 332-3001 f: (818) 244-9761 Back to the Future The Story of Squeak, A Practical Smalltalk Written in Itself by Dan Ingalls Ted Kaehler John Maloney Scott Wallace Alan Kay at Apple Computer while doing this work, now at Walt Disney Imagineering 1401 Flower Street P.O. Box 25020 Glendale, CA 91221 [email protected] Abstract Squeak is an open, highly-portable Smalltalk implementation whose virtual machine is written entirely in Smalltalk, making it easy to debug, analyze, and change. To achieve practical performance, a translator produces an equivalent C program whose performance is comparable to commercial Smalltalks. Other noteworthy aspects of Squeak include: a compact object format that typically requires only a single word of overhead per object; a simple yet efficient incremental garbage collector for 32-bit direct pointers; efficient bulk- mutation of objects; extensions of BitBlt to handle color of any depth and anti-aliased image rotation and scaling; and real-time sound and music synthesis written entirely in Smalltalk. Overview Squeak is a modern implementation of Smalltalk-80 that is available for free via the Internet, at http://www.research.apple.com/research/proj/learning_concepts/squeak/ and other sites. It includes platform-independent support for color, sound, and image processing. -
Smalltalk's Influence on Modern Programming
1 Smalltalk’s Influence on Modern Programming Smalltalk’s Influence on Modern Programming Matt Savona. February 1 st , 2008. In September 1972 at Xerox PARC, Alan Kay, Ted Kaeher and Dan Ingalls were discussing programming languages in the hallway of their office building. Ted and Dan had begun to consider how large a language had to be to have “great power.” Alan took a different approach, and “asserted that you could define the "most powerful language in the world" in "a page of code."” Ted and Dan replied, “Put up or shut up” (Kay). And with that, the bet to develop the most powerful language was on. Alan arrived at PARC every morning at 4am for two weeks, and devoted his time from 4am to 8am to the development of the language. That language was Smalltalk. Kay had “originally made the boast because McCarthy's self-describing LISP interpreter was written in itself. It was about "a page", and as far as power goes, LISP was the whole nine- yards for functional languages.” He was sure that he could “do the same for object-oriented languages” and still have a reasonable syntax. By the eighth morning, Kay had a version of Smalltalk developed where “symbols were byte-coded and the receiving of return-values from a send was symmetric” (Kay). Several days later, Dan Ingalls had coded Kay’s scheme in BASIC, added a “token scanner”, “list maker” and many other features. “Over the next ten years he made at least 80 major releases of various flavors of Smalltalk” (Kay). -
Cross-Language Interop Poster
Low Cognitive Overhead Bridging "Interoperability isn't just a technical problem, it's also a user interface problem. We have to make it easy to call between languages, so that users can The demo shown at FOSDEM always pick the most appropriate tool for the job at hand." example [ this year showed fully dynamic "This shows how to call a C function from Smalltalk" development spanning C objc_setAssociatedObject: { Objective-C and Smalltalk. The self. 42. developer can inspect the ’fishes’. existing class hierarchy and C enumValue: OBJC_ASSOCIATION_ASSIGN }. the code for any methods "Note how C enumerations can be accessed. When encountering this construct, the Pragmatic Smalltalk compiler will generate exactly the same code as an implemented in Smalltalk. It Objective-C compiler would for this line: is also possible to add, modify, objc_setAssociatedObject(self, 42, @"fishes", OBJC_ASSOCIATION_ASSIGN); or replace methods, add It will get the types for the function by parsing the header and automatically map Smalltalk types to C and Objective-C types. The instance variables and classes Easy Development programmer doesn't need to write any bridging or foreign function interface at run time. Invoking a code." nonexistent class or method (C objc_getAssociatedObject: {self . 42 }) log. ] pops up a dialog asking the user to implement it, all from within a running app. The final version is statically compiled, with no explicit user Cross-Language Interoperability for intervention required. Fast, Easy, and Maintainable Code David Chisnall The World Today: But I wanted to use the But libfoo is C, libbar Frobnicator framework, and it's I have a great idea is C++, and I want to only for Java. -
Avaliação De Performance De Interpretadores Ruby
Universidade Federal de Santa Catarina Centro Tecnológico Curso de Sistemas de Informação Wilson de Almeida Avaliação de Performance de Interpretadores Ruby Florianópolis 2010 Wilson de Almeida Avaliação de Performance de Interpretadores Ruby Monograa apresentada ao Curso de Sistemas de Informação da UFSC, como requisito para a obten- ção parcial do grau de BACHAREL em Sistemas de Informação. Orientador: Lúcia Helena Martins Pacheco Doutora em Engenharia Florianópolis 2010 Almeida, Wilson Avaliação de Performance de Interpretadores Ruby / Wilson Al- meida - 2010 xx.p 1.Performance 2. Interpretadores.. I.Título. CDU 536.21 Wilson de Almeida Avaliação de Performance de Interpretadores Ruby Monograa apresentada ao Curso de Sistemas de Informação da UFSC, como requisito para a obten- ção parcial do grau de BACHAREL em Sistemas de Informação. Aprovado em 21 de junho de 2010 BANCA EXAMINADORA Lúcia Helena Martins Pacheco Doutora em Engenharia José Eduardo De Lucca Mestre em Ciências da Computação Eduardo Bellani Bacharel em Sistemas de Informação Aos meus pais e meu irmão. Aos familiares e amigos, em especial pra mi- nha eterna amiga Liliana, que está torcendo por mim de onde ela estiver. Agradecimentos Agradeço ao meu amigo, colega de curso, parceiro de trabalhos e orientador Eduardo Bellani, pelo encorajamento, apoio e seus ricos conselhos sobre o melhor direci- onamento deste trabalho. A professora Lúcia Helena Martins Pacheco pela orientação, amizade, e pela paciência, sem a qual este trabalho não se realizaria. Ao professor José Eduardo Delucca, por seus conselhos objetivos e pontuais. Todos os meus amigos que incentivaram e compreenderam a minha ausência nesse período de corrida atrás do objetivo de concluir o curso. -
Smalltalk Language Mapping Specification
Smalltalk Language Mapping Specification New Edition: June 1999 Copyright 1995, 1996 BNR Europe Ltd. Copyright 1998, Borland International Copyright 1991, 1992, 1995, 1996 Digital Equipment Corporation Copyright 1995, 1996 Expersoft Corporation Copyright 1996, 1997 FUJITSU LIMITED Copyright 1996 Genesis Development Corporation Copyright 1989, 1990, 1991, 1992, 1995, 1996 Hewlett-Packard Company Copyright 1991, 1992, 1995, 1996 HyperDesk Corporation Copyright 1998 Inprise Corporation Copyright 1996, 1997 International Business Machines Corporation Copyright 1995, 1996 ICL, plc Copyright 1995, 1996 IONA Technologies, Ltd. Copyright 1996, 1997 Micro Focus Limited Copyright 1991, 1992, 1995, 1996 NCR Corporation Copyright 1995, 1996 Novell USG Copyright 1991,1992, 1995, 1996 by Object Design, Inc. Copyright 1991, 1992, 1995, 1996 Object Management Group, Inc. Copyright 1996 Siemens Nixdorf Informationssysteme AG Copyright 1991, 1992, 1995, 1996 Sun Microsystems, Inc. Copyright 1995, 1996 SunSoft, Inc. Copyright 1996 Sybase, Inc. Copyright 1998 Telefónica Investigación y Desarrollo S.A. Unipersonal Copyright 1996 Visual Edge Software, Ltd. The companies listed above have granted to the Object Management Group, Inc. (OMG) a nonexclusive, royalty-free, paid up, worldwide license to copy and distribute this document and to modify this document and distribute copies of the modified ver- sion. Each of the copyright holders listed above has agreed that no person shall be deemed to have infringed the copyright in the included material of any such copyright holder by reason of having used the specification set forth herein or having con- formed any computer software to the specification. PATENT The attention of adopters is directed to the possibility that compliance with or adoption of OMG specifications may require use of an invention covered by patent rights. -
Dynamic Object-Oriented Programming with Smalltalk
Dynamic Object-Oriented Programming with Smalltalk 1. Introduction Prof. O. Nierstrasz Autumn Semester 2009 LECTURE TITLE What is surprising about Smalltalk > Everything is an object > Everything happens by sending messages > All the source code is there all the time > You can't lose code > You can change everything > You can change things without restarting the system > The Debugger is your Friend © Oscar Nierstrasz 2 ST — Introduction Why Smalltalk? > Pure object-oriented language and environment — “Everything is an object” > Origin of many innovations in OO development — RDD, IDE, MVC, XUnit … > Improves on many of its successors — Fully interactive and dynamic © Oscar Nierstrasz 1.3 ST — Introduction What is Smalltalk? > Pure OO language — Single inheritance — Dynamically typed > Language and environment — Guiding principle: “Everything is an Object” — Class browser, debugger, inspector, … — Mature class library and tools > Virtual machine — Objects exist in a persistent image [+ changes] — Incremental compilation © Oscar Nierstrasz 1.4 ST — Introduction Smalltalk vs. C++ vs. Java Smalltalk C++ Java Object model Pure Hybrid Hybrid Garbage collection Automatic Manual Automatic Inheritance Single Multiple Single Types Dynamic Static Static Reflection Fully reflective Introspection Introspection Semaphores, Some libraries Monitors Concurrency Monitors Categories, Namespaces Packages Modules namespaces © Oscar Nierstrasz 1.5 ST — Introduction Smalltalk: a State of Mind > Small and uniform language — Syntax fits on one sheet of paper > -
Aplikacija Za Pregled Tehnologij Spletnih Projektov Na Podlagi Avtomatske Analize Repozitorijev
Univerza v Ljubljani Fakulteta za računalništvo in informatiko Aljana Polanc Aplikacija za pregled tehnologij spletnih projektov na podlagi avtomatske analize repozitorijev DIPLOMSKO DELO VISOKOŠOLSKI STROKOVNI ŠTUDIJSKI PROGRAM PRVE STOPNJE RAČUNALNIŠTVO IN INFORMATIKA Mentor: doc. dr. Aleš Smrdel Ljubljana, 2016 Rezultati diplomskega dela so intelektualna lastnina avtorja in Fakultete za računalništvo in informatiko Univerze v Ljubljani. Za objavljanje ali iz- koriščanje rezultatov diplomskega dela je potrebno pisno soglasje avtorja, Fakultete za računalništvo in informatiko ter mentorja. Besedilo je oblikovano z urejevalnikom besedil LATEX. Fakulteta za računalništvo in informatiko izdaja naslednjo nalogo: Tematika naloge: V okviru diplomskega dela razvijte aplikacijo, ki bo služila za vzdrževanje ažurne evidence o tehnologijah pri različnih spletnih projektih. Aplikacija mora omogočiti avtomatsko periodično pregledovanje vseh aktivnih projek- tov znotraj nekega podjetja in posodabljanje podatkov o uporabljenih teh- nologijah za vsakega izmed projektov. V ta namen najprej analizirajte teh- nologije, ki se uporabljajo pri izdelavi sodobnih spletnih projektov, nato pa realizirajte aplikacijo, ki se mora biti sposobna povezati z repozitorijem pro- jektov, prebrati vse projekte in na podlagi analize datotek projektov razbrati tehnologije, ki so uporabljene pri posameznem projektu. Za vsak projekt mora aplikacija razbrati uporabljene programske jezike, orodja, knjižnice ter razvijalce, ki sodelujejo pri posameznem projektu. Razvijte tudi spletni vme- snik, ki bo omogočal prikaz podatkov o projektih in tehnologijah znotraj ne- kega podjetja ter nadroben prikaz tehnologij uporabljenih pri posameznem projektu. Razvijte tudi administrativni spletni vmesnik, ki bo omogočal do- polnjevanje in popravljanje avtomatsko pridobljenih podatkov. Pri razvoju aplikacije izberite najprimernejše tehnologije na strani odjemalca in na strani strežnika. Uspešnost delovanja razvite aplikacije testirajte na nekem repozi- toriju projektov. -
Supporting C Extensions for Dynamic Languages an Abbreviated Version of [10]
High-Performance Language Composition: Supporting C Extensions for Dynamic Languages An abbreviated version of [10]. Grimmer Matthias1, Chris Seaton2, Thomas W¨urthinger2 and Hanspeter M¨ossenb¨ock1 1 Johannes Kepler University, Linz, Austria fgrimmer,[email protected] 2 Oracle Labs fchris.seaton,[email protected] Abstract. Many dynamic languages such as Ruby offer functionality for writing parts of applications in a lower-level language such as C. These C extension modules are usually written against the API of an interpreter, which provides access to the higher-level language's internal data struc- tures. Alternative implementations of the high-level languages often do not support such C extensions because implementing the same API as in the original implementations is complicated and limits performance. In this paper we describe a novel approach for modular composition of languages that allows dynamic languages to support C extensions through interpretation. We propose a flexible and reusable cross-language mechanism that allows composing multiple language interpreters. This mechanism allows us to efficiently exchange runtime data across different interpreters and also enables the dynamic compiler of the host VM to inline and optimize programs across multiple language boundaries. We evaluate our approach by composing a Ruby interpreter with a C interpreter. We run existing Ruby C extensions and show how our system executes combined Ruby and C modules on average over 3× faster than the conventional implementation of Ruby with native C extensions. 1 Introduction Most programming languages offer functionality for calling routines in modules that are written in another language. There are multiple reasons why program- mers want to do this, including to run modules already written in another lan- guage, to achieve higher performance than is normally possible in the primary language, or generally to allow different parts of the system to be written in the most appropriate language. -
Specialising Dynamic Techniques for Implementing the Ruby Programming Language
SPECIALISING DYNAMIC TECHNIQUES FOR IMPLEMENTING THE RUBY PROGRAMMING LANGUAGE A thesis submitted to the University of Manchester for the degree of Doctor of Philosophy in the Faculty of Engineering and Physical Sciences 2015 By Chris Seaton School of Computer Science This published copy of the thesis contains a couple of minor typographical corrections from the version deposited in the University of Manchester Library. [email protected] chrisseaton.com/phd 2 Contents List of Listings7 List of Tables9 List of Figures 11 Abstract 15 Declaration 17 Copyright 19 Acknowledgements 21 1 Introduction 23 1.1 Dynamic Programming Languages.................. 23 1.2 Idiomatic Ruby............................ 25 1.3 Research Questions.......................... 27 1.4 Implementation Work......................... 27 1.5 Contributions............................. 28 1.6 Publications.............................. 29 1.7 Thesis Structure............................ 31 2 Characteristics of Dynamic Languages 35 2.1 Ruby.................................. 35 2.2 Ruby on Rails............................. 36 2.3 Case Study: Idiomatic Ruby..................... 37 2.4 Summary............................... 49 3 3 Implementation of Dynamic Languages 51 3.1 Foundational Techniques....................... 51 3.2 Applied Techniques.......................... 59 3.3 Implementations of Ruby....................... 65 3.4 Parallelism and Concurrency..................... 72 3.5 Summary............................... 73 4 Evaluation Methodology 75 4.1 Evaluation Philosophy -
Lightweight Method Dispatch On
Lightweight Method Dispatch on MRI Koichi Sasada <[email protected]> EURUKO2015 Koichi Sasada A programmer from Japan Koichi is a Programmer •MRI committer since 2007/01 • Original YARV developer since 2004/01 • YARV: Yet Another RubyVM • Introduced into Ruby (MRI) 1.9.0 and later • Generational/incremental GC for 2.x “Why I wouldn’t use rails for a new company” by Jared Friedman “The ruby interpreter is just a volunteer effort. Between 2007- 2012, there were a number of efforts to fix the interpreter and make it fast (Rubinius, Jruby, YARV, etc) But lacking backers with staying power, the volunteers got bored and some of the efforts withered. JRuby is still active and recent versions are showing more promise with performance, but it’s been a long road.” Quoted from http://blog.jaredfriedman.com/2015/09/15/why-i- wouldnt-use-rails-for-a-new-company/ (September 15, 2015) Koichi is an Employee Koichi is a member of Heroku Matz team Mission Design Ruby language and improve quality of MRI Heroku employs three full time Ruby core developers in Japan named “Matz team” Heroku Matz team Matz Designer/director of Ruby Nobu Quite active committer Ko1 Internal Hacker Matz Title collector • He has so many (job) title • Japanese teacher • Chairman - Ruby Association • Fellow - NaCl • Chief architect, Ruby - Heroku • Research institute fellow – Rakuten • Chairman – NPO mruby Forum • Senior researcher – Kadokawa Ascii Research Lab • Visiting professor – Shimane University • Honorable citizen (living) – Matsue city • Honorable member – Nihon Ruby no Kai -
Load Testing of Containerised Web Services
UPTEC IT 16003 Examensarbete 30 hp Mars 2016 Load Testing of Containerised Web Services Christoffer Hamberg Abstract Load Testing of Containerised Web Services Christoffer Hamberg Teknisk- naturvetenskaplig fakultet UTH-enheten Load testing web services requires a great deal of environment configuration and setup. Besöksadress: This is especially apparent in an environment Ångströmlaboratoriet Lägerhyddsvägen 1 where virtualisation by containerisation is Hus 4, Plan 0 used with many moving and volatile parts. However, containerisation tools like Docker Postadress: offer several properties, such as; application Box 536 751 21 Uppsala image creation and distribution, network interconnectivity and application isolation that Telefon: could be used to support the load testing 018 – 471 30 03 process. Telefax: 018 – 471 30 00 In this thesis, a tool named Bencher, which goal is to aid the process of load testing Hemsida: containerised (with Docker) HTTP services, is http://www.teknat.uu.se/student designed and implemented. To reach its goal Bencher automates some of the tedious steps of load testing, including connecting and scaling containers, collecting system metrics and load testing results to name a few. Bencher’s usability is verified by testing a number of hypotheses formed around different architecture characteristics of web servers in the programming language Ruby. With a minimal environment setup cost and a rapid test iteration process, Bencher proved its usability by being successfully used to verify the hypotheses in this thesis. However, there is still need for future work and improvements, including for example functionality for measuring network bandwidth and latency, that could be added to enhance process even further. To conclude, Bencher fulfilled its goal and scope that were set for it in this thesis. -
Inter-Language Collaboration in an Object-Oriented Virtual Machine Bachelor’S Thesis
Inter-language Collaboration in an Object-oriented Virtual Machine Bachelor’s Thesis MATTHIAS SPRINGER, Hasso Plattner Institute, University of Potsdam TIM FELGENTREFF, Hasso Plattner Institute, University of Potsdam (Supervisor) TOBIAS PAPE, Hasso Plattner Institute, University of Potsdam (Supervisor) ROBERT HIRSCHFELD, Hasso Plattner Institute, University of Potsdam (Supervisor) Multi-language virtual machines have a number of advantages. They allow software developers to use soft- ware libraries that were written for different programming languages. Furthermore, language implementors do not have to bother with low-level VM functionality and their implementation can benefit from optimiza- tions in existing virtual machines. MagLev is an implementation of the Ruby programming language on top of the GemStone/S virtual machine for the Smalltalk programming language. In this work, we present how software components written in both languages can interact. We show how MagLev unifies the Smalltalk and the Ruby object model, taking into account Smalltalk meta classes, Ruby singleton classes, and Ruby modules. Besides, we show how we can call Ruby methods from Smalltalk and vice versa. We also present MagLev’s concept of bridge methods for implementing Ruby method calling conventions. Finally, we compare our solution to other language implementations and virtual machines. Additional Key Words and Phrases: Virtual Machines, Ruby, Smalltalk, MagLev, Language Implementation 1. MULTI-LANGUAGE VIRTUAL MACHINES Multi-language virtual machines are virtual machines that support the execution of source code written in multiple languages. According to Vraný [Vraný 2010], we have to distinguish multi-lanuguage virtual machines from interpreters and native code execution. Languages inside multi-language virtual machines are usually compiled to byte code and share the same object space.