Implementing Functional Languages with Abstract Machines
Total Page:16
File Type:pdf, Size:1020Kb
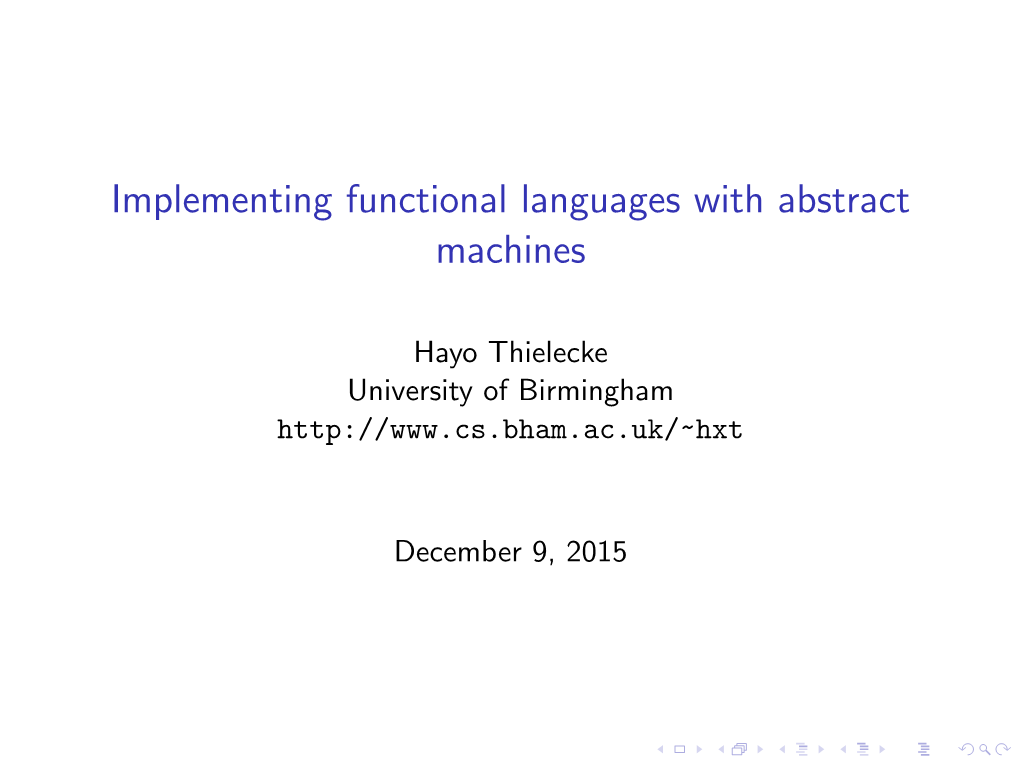
Load more
Recommended publications
-
Biorthogonality, Step-Indexing and Compiler Correctness
Biorthogonality, Step-Indexing and Compiler Correctness Nick Benton Chung-Kil Hur Microsoft Research University of Cambridge [email protected] [email protected] Abstract to a deeper semantic one (‘does the observable behaviour of the We define logical relations between the denotational semantics of code satisfy this desirable property?’). In previous work (Benton a simply typed functional language with recursion and the opera- 2006; Benton and Zarfaty 2007; Benton and Tabareau 2009), we tional behaviour of low-level programs in a variant SECD machine. have looked at establishing type-safety in the latter, more semantic, The relations, which are defined using biorthogonality and step- sense. Our key notion is that a high-level type translates to a low- indexing, capture what it means for a piece of low-level code to level specification that should be satisfied by any code compiled implement a mathematical, domain-theoretic function and are used from a source language phrase of that type. These specifications are to prove correctness of a simple compiler. The results have been inherently relational, in the usual style of PER semantics, capturing formalized in the Coq proof assistant. the meaning of a type A as a predicate on low-level heaps, values or code fragments together with a notion of A-equality thereon. These Categories and Subject Descriptors F.3.1 [Logics and Mean- relations express what it means for a source-level abstractions (e.g. ings of Programs]: Specifying and Verifying and Reasoning about functions of type A ! B) to be respected by low-level code (e.g. Programs—Mechanical verification, Specification techniques; F.3.2 ‘taking A-equal arguments to B-equal results’). -
Forms of Semantic Speci Cation
THE LOGIC IN COMPUTER SCIENCE COLUMN by Yuri GUREVICH Electrical Engineering and Computer Science Department University of Michigan Ann Arb or MI USA Forms of Semantic Sp ecication Carl A Gunter UniversityofPennsylvania The way to sp ecify a programming language has b een a topic of heated debate for some decades and at present there is no consensus on how this is b est done Real languages are almost always sp ecied informally nevertheless precision is often enough lacking that more formal approaches could b enet b oth programmers and language implementors My purp ose in this column is to lo ok at a few of these formal approaches in hop e of establishing some distinctions or at least stirring some discussion Perhaps the crudest form of semantics for a programming language could b e given by providing a compiler for the language on a sp ecic physical machine If the machine archi tecture is easy to understand then this could b e viewed as a way of explaining the meaning of a program in terms of a translation into a simple mo del This view has the advantage that every higherlevel programming language of any use has a compiler for at least one machine and this sp ecication is entirely formal Moreover the sp ecication makes it known to the programmer how eciency is b est achieved when writing programs in the language and compiling on that machine On the other hand there are several problems with this technique and I do not knowofany programming language that is really sp ecied in this way although Im told that such sp ecications do exist -
A Rational Deconstruction of Landin's SECD Machine
A Rational Deconstruction of Landin’s SECD Machine Olivier Danvy BRICS, Department of Computer Science, University of Aarhus, IT-parken, Aabogade 34, DK-8200 Aarhus N, Denmark [email protected] Abstract. Landin’s SECD machine was the first abstract machine for the λ-calculus viewed as a programming language. Both theoretically as a model of computation and practically as an idealized implementation, it has set the tone for the subsequent development of abstract machines for functional programming languages. However, and even though variants of the SECD machine have been presented, derived, and invented, the precise rationale for its architecture and modus operandi has remained elusive. In this article, we deconstruct the SECD machine into a λ- interpreter, i.e., an evaluation function, and we reconstruct λ-interpreters into a variety of SECD-like machines. The deconstruction and reconstruc- tions are transformational: they are based on equational reasoning and on a combination of simple program transformations—mainly closure conversion, transformation into continuation-passing style, and defunc- tionalization. The evaluation function underlying the SECD machine provides a precise rationale for its architecture: it is an environment-based eval- apply evaluator with a callee-save strategy for the environment, a data stack of intermediate results, and a control delimiter. Each of the com- ponents of the SECD machine (stack, environment, control, and dump) is therefore rationalized and so are its transitions. The deconstruction and reconstruction method also applies to other abstract machines and other evaluation functions. 1 Introduction Forty years ago, Peter Landin wrote a profoundly influencial article, “The Me- chanical Evaluation of Expressions” [27], where, in retrospect, he outlined a substantial part of the functional-programming research programme for the fol- lowing decades. -
From Interpreter to Compiler and Virtual Machine: a Functional Derivation Basic Research in Computer Science
BRICS Basic Research in Computer Science BRICS RS-03-14 Ager et al.: From Interpreter to Compiler and Virtual Machine: A Functional Derivation From Interpreter to Compiler and Virtual Machine: A Functional Derivation Mads Sig Ager Dariusz Biernacki Olivier Danvy Jan Midtgaard BRICS Report Series RS-03-14 ISSN 0909-0878 March 2003 Copyright c 2003, Mads Sig Ager & Dariusz Biernacki & Olivier Danvy & Jan Midtgaard. BRICS, Department of Computer Science University of Aarhus. All rights reserved. Reproduction of all or part of this work is permitted for educational or research use on condition that this copyright notice is included in any copy. See back inner page for a list of recent BRICS Report Series publications. Copies may be obtained by contacting: BRICS Department of Computer Science University of Aarhus Ny Munkegade, building 540 DK–8000 Aarhus C Denmark Telephone: +45 8942 3360 Telefax: +45 8942 3255 Internet: [email protected] BRICS publications are in general accessible through the World Wide Web and anonymous FTP through these URLs: http://www.brics.dk ftp://ftp.brics.dk This document in subdirectory RS/03/14/ From Interpreter to Compiler and Virtual Machine: a Functional Derivation Mads Sig Ager, Dariusz Biernacki, Olivier Danvy, and Jan Midtgaard BRICS∗ Department of Computer Science University of Aarhusy March 2003 Abstract We show how to derive a compiler and a virtual machine from a com- positional interpreter. We first illustrate the derivation with two eval- uation functions and two normalization functions. We obtain Krivine's machine, Felleisen et al.'s CEK machine, and a generalization of these machines performing strong normalization, which is new. -
An Introduction to Landin's “A Generalization of Jumps and Labels”
Higher-Order and Symbolic Computation, 11, 117–123 (1998) c 1998 Kluwer Academic Publishers, Boston. Manufactured in The Netherlands. An Introduction to Landin’s “A Generalization of Jumps and Labels” HAYO THIELECKE [email protected] qmw, University of London Abstract. This note introduces Peter Landin’s 1965 technical report “A Generalization of Jumps and Labels”, which is reprinted in this volume. Its aim is to make that historic paper more accessible to the reader and to help reading it in context. To this end, we explain Landin’s control operator J in more contemporary terms, and we recall Burge’s solution to a technical problem in Landin’s original account. Keywords: J-operator, secd-machine, call/cc, goto, history of programming languages 1. Introduction In the mid-60’s, Peter Landin was investigating functional programming with “Applicative Expressions”, which he used to provide an account of Algol 60 [10]. To explicate goto, he introduced the operator J (for jump), in effect extending functional programming with control. This general control operator was powerful enough to have independent interest quite beyond the application to Algol: Landin documented the new paradigm in a series of technical reports [11, 12, 13]. The most significant of these reports is “A Generalization of Jumps and Labels” (reprinted in this volume on pages 9–27), as it presents and investigates the J-operator in its own right. The generalization is a radical one: it introduces first-class control into programming languages for the first time. Control is first-class in that the generalized labels given by the J-operator can be passed as arguments to and returned from procedures. -
Foundations of Functional Programming
Foundations of Functional Programming Computer Science Tripos Part IB Easter Term Lawrence C Paulson Computer Laboratory University of Cambridge [email protected] Copyright © 2021 by Lawrence C. Paulson Contents 1 Introduction 1 2 Equality and Normalization 6 3 Encoding Data in the λ-Calculus 11 4 Writing Recursive Functions in the λ-calculus 16 5 The λ-Calculus and Computation Theory 23 6 ISWIM: The λ-calculus as a Programming Language 29 7 Lazy Evaluation via Combinators 39 8 Compiling Methods Using Combinators 45 i ii 1 1 Introduction This course is concerned with the λ-calculus and its close relative, combinatory logic. The λ-calculus is important to functional programming and to computer science generally: 1. Variable binding and scoping in block-structured languages can be mod- elled. 2. Several function calling mechanisms — call-by-name, call-by-value, and call-by-need — can be modelled. The latter two are also known as strict evaluation and lazy evaluation. 3. The λ-calculus is Turing universal, and is probably the most natural model of computation. Church’s Thesis asserts that the ‘computable’ functions are precisely those that can be represented in the λ-calculus. 4. All the usual data structures of functional programming, including infinite lists, can be represented. Computation on infinite objects can be defined formally in the λ-calculus. 5. Its notions of confluence (Church-Rosser property), termination, and nor- mal form apply generally in rewriting theory. 6. Lisp, one of the first major programming languages, was inspired by the λ-calculus. Many functional languages, such as ML, consist of little more than the λ-calculus with additional syntax. -
Simplification of Abstract Machine for Functional Language and Its Theoretical Investigation
Journal of Software Simplification of Abstract Machine for Functional Language and Its Theoretical Investigation Shin-Ya Nishizaki*, Kensuke Narita, Tomoyuki Ueda Department of Computer Science, Tokyo Institute of Technology, Ookayama, Meguro, Tokyo, Japan. * Corresponding author. Tel.: +81-3-5734-2772; email: [email protected] Manuscript submitted February 18, 2015; accepted March 4, 2015. doi: 10.17706/jsw.10.10.1148-1159 Abstract: Many researchers have studied abstract machines in order to give operational semantics to various kinds of programming languages. For example, Landin's SECD machine and Curien's Categorical Abstract Machine are proposed for functional programming languages and useful not only for theoretical studies but also implementation of practical language processors. We study simplification of SECD machine and design a new abstract machine, called Simple Abstract Machine. We achieve the simplification of SECD machine by abstracting substitutions for variables. In Simple Abstract Machine, we can formalize first-class continuations more simply and intelligibly than SECD machine. Key words: Programming language theory, lambda calculus, first-class continuation, abstract machine. 1. Introduction Many researchers have proposed abstract machines in order to give operational semantics to various kinds of programming languages, such as Landin's SECD machine [1], [2] and Curien's Categorical Abstract machine [3], [4]. From the theoretical viewpoint, there are many kinds of researches of abstract machines. Graham formalized and verified implementation of SECD machine using the HOL prover [5]. Hardin et al. formulated abstract machines for functional languages in the framework of explicit substitutes [6]. Ohori studied abstract machines from the proof-theoretic approach [7]. Curien et al. -
Abstract Λ-Calculus Machines
Abstract λ-Calculus Machines Werner E. Kluge Department of Computer Science University of Kiel D–24105 Kiel, Germany E–mail: [email protected] Abstract. This paper is about fully normalizing λ-calculus machines that permit sym- bolic computations involving free variables. They employ full-fledged β-reductions to pre- serve static binding scopes when substituting and reducing under abstractions. Abstrac- tions and variables thus become truly first class objects: both may be freely substituted for λ-bound variables and returned as abstraction values. This contrasts with implementa- tions of conventional functional languages which realize a weakly normalizing λ-calculus that is capable of computing closed terms (or basic values) only. The two machines described in this paper are descendants of a weakly normalizing secd- machine that supports a nameless λ-calculus which has bound variable occurrences re- placed by binding indices. Full normalization is achieved by a few more state transition rules that η-extend unapplied abstractions to full applications, inserting in ascending order binding indices for missing arguments. Updating these indices in the course of performing β-reductions is accomplished by means of a simple counting mechanism that inflicts very little overhead. Both machines realize a head-order strategy that emphasizes normaliza- tion along the leftmost spine of a λ-expression. The simpler fn secd-machine abides by the concept of saving (and unsaving) on a dump structure machine contexts upon each individual β-reduction. The more sophisticated fn se(m)cd-machine performs what are called β-reductions-in-the-large that head-normalize entire spines in the same contexts. -
Lambda and Pi Calculi, CAM and SECD Machines
Lambda and pi calculi, CAM and SECD machines Vasco Thudichum Vasconcelos Department of Informatics Faculty of Sciences University of Lisbon February 2001 (revised November 2002, August 2003) Abstract We analyse machines that implement the call-by-value reduction strategy of the λ-calculus: two environment machines—CAM and SECD—and two encodings into the π-calculus—due to Milner and Vasconcelos. To establish the relation between the various machines, we setup a notion of reduction machine and two notions of corre- spondences: operational—in which a reduction step in the source ma- chine is mimicked by a sequence of steps in the target machine—and convergent—where only reduction to normal form is simulated. We show that there are operational correspondences from the λ-calculus into CAM, and from CAM and from SECD into the π-calculus. Plotkin completes the picture by showing that there is a convergent correspon- dence from the λ-calculus into SECD. 1 Introduction The call-by-value reduction strategy of the λ-calculus equips a large number of today’s programming languages. To study the operational aspects of this reduction strategy Plotkin used Landin’s SECD, a stack-based, environment machine [6, 11]. Based on the Categorical Abstract Machine and inspired by the Krivine’s lazy abstract machine, Curien proposed a different environment machine, which we call CAM throughout this paper [4]. From a different community, two interpretations of the call-by-value λ-calculus into the π- calculus are known: one proposed by Milner, and further studied by Pierce 1 and Sangiorgi [7, 8, 9], the other proposed by the author, but lacking, to date a systematic study [13]. -
Programming Languages and Lambda Calculi (Utah CS7520 Version)
Programming Languages and Lambda Calculi (Utah CS7520 Version) Matthias Felleisen Matthew Flatt Draft: March 8, 2006 Copyright c 1989, 2003 Felleisen, Flatt 2 Contents I Models of Languages 7 Chapter 1: Computing with Text 9 1.1 Defining Sets ...................................... 9 1.2 Relations ........................................ 10 1.3 Relations as Evaluation ................................ 11 1.4 Directed Evaluation .................................. 11 1.5 Evaluation in Context ................................. 12 1.6 Evaluation Function .................................. 13 1.7 Notation Summary ................................... 13 Chapter 2: Structural Induction 15 2.1 Detecting the Need for Structural Induction ..................... 15 2.2 Definitions with Ellipses ................................ 17 2.3 Induction on Proof Trees ................................ 17 2.4 Multiple Structures ................................... 18 2.5 More Definitions and More Proofs .......................... 19 Chapter 3: Consistency of Evaluation 21 Chapter 4: The λ-Calculus 25 4.1 Functions in the λ-Calculus .............................. 25 4.2 λ-Calculus Grammar and Reductions ......................... 26 4.3 Encoding Booleans ................................... 28 4.4 Encoding Pairs ..................................... 29 4.5 Encoding Numbers ................................... 30 4.6 Recursion ........................................ 31 4.6.1 Recursion via Self-Application ........................ 32 4.6.2 Lifting Out Self-Application ......................... -
Closure (Computer Programming) - Wikipedia, the Free Encyclopedia
Closure (computer programming) - Wikipedia, the free encyclopedia http://en.wikipedia.org/w/index.php?title=Closure_(computer_programm... From Wikipedia, the free encyclopedia In programming languages, a closure (also lexical closure or function closure) is a function or reference to a function together with a referencing environment—a table storing a reference to each of the non-local variables (also called free variables or upvalues) of that function.[1] A closure—unlike a plain function pointer—allows a function to access those non-local variables even when invoked outside its immediate lexical scope. The concept of closures was developed in the 1960s and was first fully implemented in 1975 as a language feature in the Scheme programming language to support lexically scoped first-class functions. The use of closures is associated with functional programming languages such as Lisp and ML. Traditional imperative languages such as Algol, C and Pascal do not support returning nested functions as results from higher-order functions. Many modern garbage-collected imperative languages support closures, such as Smalltalk (the first object-oriented language to do so),[2] OCaml, Python, Perl, Ruby, JavaScript, Go, Dart, Scala, Swift, C#, Rust, Julia and C++ since C++11. 1 Example 2 History and etymology 3 Implementation and theory 4 Applications 4.1 First-class functions 4.2 State representation 4.3 Other uses 5 Differences in semantics 5.1 Lexical environment 5.2 Closure leaving 6 Closure-like constructs 6.1 Callbacks (C) 6.2 Local classes and Lambda functions (Java) 6.3 Blocks (C, C++, Objective-C 2.0) 6.4 Delegates (C#, D) 6.5 Function objects (C++) 6.6 Inline agents (Eiffel) 7 See also 8 References 9 External links 1 of 15 6/22/2014 1:33 PM Closure (computer programming) - Wikipedia, the free encyclopedia http://en.wikipedia.org/w/index.php?title=Closure_(computer_programm.. -
Deriving the Full-Reducing Krivine Machine from the Small-Step Operational Semantics of Normal Order *
Deriving the Full-Reducing Krivine Machine from the Small-Step Operational Semantics of Normal Order * Alvaro Garcia-Perez Pablo Nogueira Juan Jose Moreno-Navarro EvIDEA Software Institute and Universidad Politecnica de Madrid EvIDEA Software Institute and Universidad Politecnica de Madrid [email protected] Universidad Politecnica de Madrid [email protected] [email protected] within the hole, and recomposing the resulting term.) A reduction- free normaliser is a program implementing a big-step natural se mantics. Finally, abstract machines are first-order, tail-recursive implementations of state transition functions that, unlike virtual machines, operate directly on terms, have no instruction set, and no need for a compiler. There has been considerable research on inter-derivation by program transformation of such 'semantic artefacts', of which the Abstract works [3, 5,12,14] are noticeable exemplars. The transformations consist of equivalence-preserving steps (CPS transformation, in We derive by program transformation Pierre Cregut's full-reducing verse CPS, defunctionaUsation, refunctionalisation, inlining, light Krivine machine KN from the structural operational semantics of weight fusion by fixed-point promotion, etc.) which establish a the normal order reduction strategy in a closure-converted pure formal correspondence between the artefacts: that all implement lambda calculus. We thus establish the correspondence between the the same reduction strategy. strategy and the machine, and showcase our technique for deriving Research in inter-derivation is important not only for the corres full-reducing abstract machines. Actually, the machine we obtain pondences and general framework it establishes in semantics. More is a slightly optimised version that can work with open terms and pragmatically, it provides a semi-automatic way of proving the cor may be used in implementations of proof assistants.