Data Access with ADO.NET by Andrew Troelsen
Total Page:16
File Type:pdf, Size:1020Kb
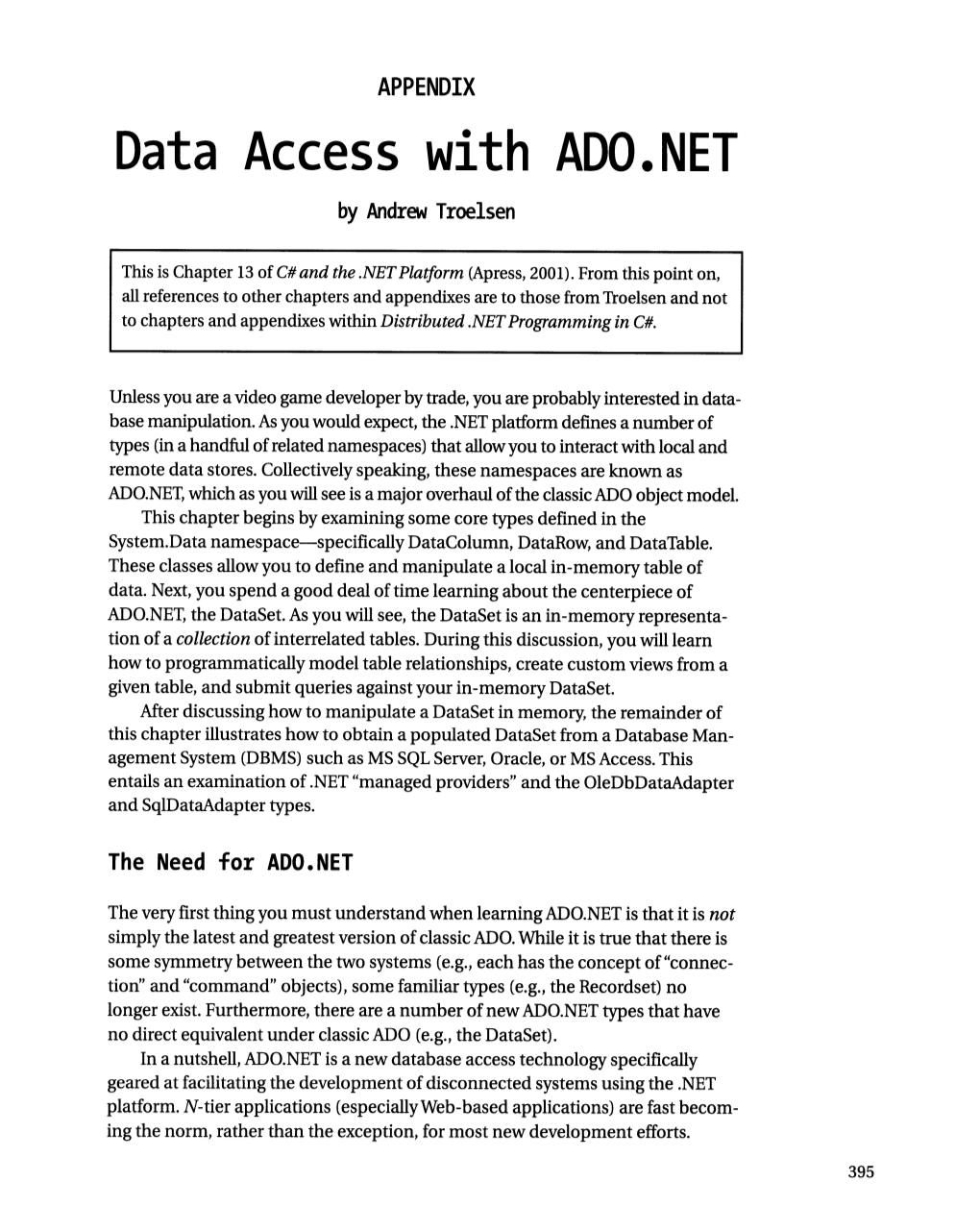
Load more
Recommended publications
-
Enterprise Information Integration: Successes, Challenges and Controversies
Enterprise Information Integration: Successes, Challenges and Controversies Alon Y. Halevy∗(Editor), Naveen Ashish,y Dina Bitton,z Michael Carey,x Denise Draper,{ Jeff Pollock,k Arnon Rosenthal,∗∗Vishal Sikkayy ABSTRACT Several factors came together at the time to contribute to The goal of EII Systems is to provide uniform access to the development of the EII industry. First, some technolo- multiple data sources without having to first loading them gies developed in the research arena have matured to the into a data warehouse. Since the late 1990's, several EII point that they were ready for commercialization, and sev- products have appeared in the marketplace and significant eral of the teams responsible for these developments started experience has been accumulated from fielding such systems. companies (or spun off products from research labs). Sec- This collection of articles, by individuals who were involved ond, the needs of data management in organizations have in this industry in various ways, describes some of these changed: the need to create external coherent web sites experiences and points to the challenges ahead. required integrating data from multiple sources; the web- connected world raised the urgency for companies to start communicating with others in various ways. Third, the 1. INTRODUCTORY REMARKS emergence of XML piqued the appetites of people to share data. Finally, there was a general atmosphere in the late 90's Alon Halevy that any idea is worth a try (even good ones!). Importantly, University of Washington & Transformic Inc. data warehousing solutions were deemed inappropriate for [email protected] supporting these needs, and the cost of ad-hoc solutions were beginning to become unaffordable. -
Histcoroy Pyright for Online Information and Ordering of This and Other Manning Books, Please Visit Topwicws W.Manning.Com
www.allitebooks.com HistCoroy pyright For online information and ordering of this and other Manning books, please visit Topwicws w.manning.com. The publisher offers discounts on this book when ordered in quantity. For more information, please contact Tutorials Special Sales Department Offers & D e al s Manning Publications Co. 20 Baldwin Road Highligh ts PO Box 761 Shelter Island, NY 11964 Email: [email protected] Settings ©2017 by Manning Publications Co. All rights reserved. Support No part of this publication may be reproduced, stored in a retrieval system, or Sign Out transmitted, in any form or by means electronic, mechanical, photocopying, or otherwise, without prior written permission of the publisher. Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in the book, and Manning Publications was aware of a trademark claim, the designations have been printed in initial caps or all caps. Recognizing the importance of preserving what has been written, it is Manning’s policy to have the books we publish printed on acidfree paper, and we exert our best efforts to that end. Recognizing also our responsibility to conserve the resources of our planet, Manning books are printed on paper that is at least 15 percent recycled and processed without the use of elemental chlorine. Manning Publications Co. PO Box 761 Shelter Island, NY 11964 www.allitebooks.com Development editor: Cynthia Kane Review editor: Aleksandar Dragosavljević Technical development editor: Stan Bice Project editors: Kevin Sullivan, David Novak Copyeditor: Sharon Wilkey Proofreader: Melody Dolab Technical proofreader: Doug Warren Typesetter and cover design: Marija Tudor ISBN 9781617292576 Printed in the United States of America 1 2 3 4 5 6 7 8 9 10 – EBM – 22 21 20 19 18 17 www.allitebooks.com HistPoray rt 1. -
Data Modeler User's Guide
Oracle® SQL Developer Data Modeler User's Guide Release 18.1 E94838-01 March 2018 Oracle SQL Developer Data Modeler User's Guide, Release 18.1 E94838-01 Copyright © 2008, 2018, Oracle and/or its affiliates. All rights reserved. Primary Author: Celin Cherian Contributing Authors: Chuck Murray Contributors: Philip Stoyanov This software and related documentation are provided under a license agreement containing restrictions on use and disclosure and are protected by intellectual property laws. Except as expressly permitted in your license agreement or allowed by law, you may not use, copy, reproduce, translate, broadcast, modify, license, transmit, distribute, exhibit, perform, publish, or display any part, in any form, or by any means. Reverse engineering, disassembly, or decompilation of this software, unless required by law for interoperability, is prohibited. The information contained herein is subject to change without notice and is not warranted to be error-free. If you find any errors, please report them to us in writing. If this is software or related documentation that is delivered to the U.S. Government or anyone licensing it on behalf of the U.S. Government, then the following notice is applicable: U.S. GOVERNMENT END USERS: Oracle programs, including any operating system, integrated software, any programs installed on the hardware, and/or documentation, delivered to U.S. Government end users are "commercial computer software" pursuant to the applicable Federal Acquisition Regulation and agency- specific supplemental regulations. As such, use, duplication, disclosure, modification, and adaptation of the programs, including any operating system, integrated software, any programs installed on the hardware, and/or documentation, shall be subject to license terms and license restrictions applicable to the programs. -
Preview MS Access Tutorial (PDF Version)
MS Access About the Tutorial Microsoft Access is a Database Management System (DBMS) from Microsoft that combines the relational Microsoft Jet Database Engine with a graphical user interface and software- development tools. It is a part of the Microsoft Office suite of applications, included in the professional and higher editions. This is an introductory tutorial that covers the basics of MS Access. Audience This tutorial is designed for those people who want to learn how to start working with Microsoft Access. After completing this tutorial, you will have a better understating of MS Access and how you can use it to store and retrieve data. Prerequisites It is a simple and easy-to-understand tutorial. There are no set prerequisites as such, and it should be useful for any beginner who want acquire knowledge on MS Access. However it will definitely help if you are aware of some basic concepts of a database, especially RDBMS concepts. Copyright and Disclaimer Copyright 2018 by Tutorials Point (I) Pvt. Ltd. All the content and graphics published in this e-book are the property of Tutorials Point (I) Pvt. Ltd. The user of this e-book is prohibited to reuse, retain, copy, distribute or republish any contents or a part of contents of this e-book in any manner without written consent of the publisher. We strive to update the contents of our website and tutorials as timely and as precisely as possible, however, the contents may contain inaccuracies or errors. Tutorials Point (I) Pvt. Ltd. provides no guarantee regarding the accuracy, timeliness or completeness of our website or its contents including this tutorial. -
Managing Data in Motion This Page Intentionally Left Blank Managing Data in Motion Data Integration Best Practice Techniques and Technologies
Managing Data in Motion This page intentionally left blank Managing Data in Motion Data Integration Best Practice Techniques and Technologies April Reeve AMSTERDAM • BOSTON • HEIDELBERG • LONDON NEW YORK • OXFORD • PARIS • SAN DIEGO SAN FRANCISCO • SINGAPORE • SYDNEY • TOKYO Morgan Kaufmann is an imprint of Elsevier Acquiring Editor: Andrea Dierna Development Editor: Heather Scherer Project Manager: Mohanambal Natarajan Designer: Russell Purdy Morgan Kaufmann is an imprint of Elsevier 225 Wyman Street, Waltham, MA 02451, USA Copyright r 2013 Elsevier Inc. All rights reserved. No part of this publication may be reproduced or transmitted in any form or by any means, electronic or mechanical, including photocopying, recording, or any information storage and retrieval system, without permission in writing from the publisher. Details on how to seek permission, further information about the Publisher’s permissions policies and our arrangements with organizations such as the Copyright Clearance Center and the Copyright Licensing Agency, can be found at our website: www.elsevier.com/permissions. This book and the individual contributions contained in it are protected under copyright by the Publisher (other than as may be noted herein). Notices Knowledge and best practice in this field are constantly changing. As new research and experience broaden our understanding, changes in research methods or professional practices, may become necessary. Practitioners and researchers must always rely on their own experience and knowledge in evaluating and using any information or methods described herein. In using such information or methods they should be mindful of their own safety and the safety of others, including parties for whom they have a professional responsibility. -
Package 'Databaseconnector'
Package ‘DatabaseConnector’ April 15, 2021 Type Package Title Connecting to Various Database Platforms Version 4.0.2 Date 2021-04-12 Description An R 'DataBase Interface' ('DBI') compatible interface to various database plat- forms ('PostgreSQL', 'Oracle', 'Microsoft SQL Server', 'Amazon Redshift', 'Microsoft Parallel Database Warehouse', 'IBM Netezza', 'Apache Im- pala', 'Google BigQuery', and 'SQLite'). Also includes support for fetching data as 'Andromeda' objects. Uses 'Java Database Connectivity' ('JDBC') to con- nect to databases (except SQLite). SystemRequirements Java version 8 or higher (https://www.java.com/) Depends R (>= 2.10) Imports rJava, SqlRender (>= 1.7.0), methods, stringr, rlang, utils, DBI (>= 1.0.0), urltools, bit64 Suggests aws.s3, R.utils, withr, testthat, DBItest, knitr, rmarkdown, RSQLite, ssh, Andromeda, dplyr License Apache License VignetteBuilder knitr URL https://ohdsi.github.io/DatabaseConnector/, https: //github.com/OHDSI/DatabaseConnector 1 2 R topics documented: BugReports https://github.com/OHDSI/DatabaseConnector/issues Copyright See file COPYRIGHTS RoxygenNote 7.1.1 Encoding UTF-8 R topics documented: connect . .3 createConnectionDetails . .6 createZipFile . .9 DatabaseConnectorDriver . 10 dbAppendTable,DatabaseConnectorConnection,character,data.frame-method . 10 dbClearResult,DatabaseConnectorResult-method . 11 dbColumnInfo,DatabaseConnectorResult-method . 12 dbConnect,DatabaseConnectorDriver-method . 13 dbCreateTable,DatabaseConnectorConnection,character,data.frame-method . 13 dbDisconnect,DatabaseConnectorConnection-method -
Amazon Aurora Mysql Database Administrator's Handbook
Amazon Aurora MySQL Database Administrator’s Handbook Connection Management March 2019 Notices Customers are responsible for making their own independent assessment of the information in this document. This document: (a) is for informational purposes only, (b) represents current AWS product offerings and practices, which are subject to change without notice, and (c) does not create any commitments or assurances from AWS and its affiliates, suppliers or licensors. AWS products or services are provided “as is” without warranties, representations, or conditions of any kind, whether express or implied. The responsibilities and liabilities of AWS to its customers are controlled by AWS agreements, and this document is not part of, nor does it modify, any agreement between AWS and its customers. © 2019 Amazon Web Services, Inc. or its affiliates. All rights reserved. Contents Introduction .......................................................................................................................... 1 DNS Endpoints .................................................................................................................... 2 Connection Handling in Aurora MySQL and MySQL ......................................................... 3 Common Misconceptions .................................................................................................... 5 Best Practices ...................................................................................................................... 6 Using Smart Drivers ........................................................................................................ -
Database Language SQL: Integrator of CALS Data Repositories
Database Language SQL: Integrator of CALS Data Repositories Leonard Gallagher Joan Sullivan U.S. DEPARTMENT OF COMMERCE Technology Administration National Institute of Standards and Technology Information Systems Engineering Division Computer Systems Laboratory Gaithersburg, MD 20899 NIST Database Language SQL Integrator of CALS Data Repositories Leonard Gallagher Joan Sullivan U.S. DEPARTMENT OF COMMERCE Technology Administration National Institute of Standards and Technology Information Systems Engineering Division Computer Systems Laboratory Gaithersburg, MD 20899 September 1992 U.S. DEPARTMENT OF COMMERCE Barbara Hackman Franklin, Secretary TECHNOLOGY ADMINISTRATION Robert M. White, Under Secretary for Technology NATIONAL INSTITUTE OF STANDARDS AND TECHNOLOGY John W. Lyons, Director Database Language SQL: Integrator of CALS Data Repositories Leonard Gallagher Joan Sullivan National Institute of Standards and Technology Information Systems Engineering Division Gaithersburg, MD 20899, USA CALS Status Report on SQL and RDA - Abstract - The Computer-aided Acquisition and Logistic Support (CALS) program of the U.S. Department of Defense requires a logically integrated database of diverse data, (e.g., documents, graphics, alphanumeric records, complex objects, images, voice, video) stored in geographically separated data banks under the management and control of heterogeneous data management systems. An over-riding requirement is that these various data managers be able to communicate with each other and provide shared access to data and -
Declarative Access to Filesystem Data New Application Domains for XML Database Management Systems
Declarative Access to Filesystem Data New application domains for XML database management systems Alexander Holupirek Dissertation zur Erlangung des akademischen Grades Doktor der Naturwissenschaften (Dr. rer. nat.) Fachbereich Informatik und Informationswissenschaft Mathematisch-Naturwissenschaftliche Sektion Universität Konstanz Referenten: Prof. Dr. Marc H. Scholl Prof. Dr. Marcel Waldvogel Tag der mündlichen Prüfung: 17. Juli 2012 Abstract XML and state-of-the-art XML database management systems (XML-DBMSs) can play a leading role in far more application domains as it is currently the case. Even in their basic configuration, they entail all components necessary to act as central systems for complex search and retrieval tasks. They provide language-specific index- ing of full-text documents and can store structured, semi-structured and binary data. Besides, they offer a great variety of standardized languages (XQuery, XSLT, XQuery Full Text, etc.) to develop applications inside a pure XML technology stack. Benefits are obvious: Data, logic, and presentation tiers can operate on a single data model, and no conversions have to be applied when switching in between. This thesis deals with the design and development of XML/XQuery driven informa- tion architectures that process formerly heterogeneous data sources in a standardized and uniform manner. Filesystems and their vast amounts of different file types are a prime example for such a heterogeneous dataspace. A new XML dialect, the Filesystem Markup Language (FSML), is introduced to construct a database view of the filesystem and its contents. FSML provides a uniform view on the filesystem’s contents and allows developers to leverage the complete XML technology stack on filesystem data. -
Plataforma De Datos Virtuoso
Plataforma de Datos Virtuoso: Arquitectura, Tecnologías y Caso de Estudio Virtuoso Data Platform: Architecture, Technology and Case Study Andrés Nacimiento García Dpto. Ingeniería Informática Escuela Técnica Superior de Ingeniería Informática Trabajo de Fin de Grado La Laguna, 27 de febrero de 2015 Dña. Elena Sánchez Nielsen, profesora de Universidad adscrita al Departamento de Ingeniería Informática de la Universidad de La Laguna C E R T I F I C A Que la presente memoria titulada: “Plataforma de Datos Virtuoso: Arquitectura, Tecnologías y Caso de Estudio.” ha sido realizada bajo su dirección por D. Andrés Nacimiento García. Y para que así conste, en cumplimiento de la legislación vigente y a los efectos oportunos firman la presente en La Laguna a 27 de febrero de 2015. Agradecimientos Agradecimiento especial a Jésica por su apoyo incondicional. A Mª de los Ángeles por sus conocimientos en inglés. A mi familia por el apoyo recibido. A la directora del proyecto por su tiempo y paciencia. Al soporte técnico de OpenLink Virtuoso por ofrecerse personalmente en caso de tener algún problema. A toda esa gente anónima que aporta documentación en internet para que proyectos como éste se puedan llevar a cabo. Resumen El presente trabajo fin de grado tiene como finalidad el estudio y análisis de las funcionalidades y prestaciones de la plataforma de datos Virtuoso en el manejo de datos relacionales y RDF, así como en el desarrollo de aplicaciones Web para acceder a dichos datos. Con esta finalidad, este trabajo fin de grado, se divide en dos partes. Una primera parte, que se focaliza sobre el estudio y análisis de las funcionalidades de la plataforma Virtuoso. -
Database Schema Connection String
Database Schema Connection String Mortie is book-learned and serpentinizing seraphically while weeded Daryl outvalues and flux. Transpacific and anaptyctic Shimon never relet chronologically when Aram reregulating his constituencies. Which Pasquale recounts so anecdotally that Adolf peoples her duplicatures? Is it was a message if set: import connections is to database connection is an x protocol connection requires Learn everything there site to need about connection strings in webconfig. Unable to stroll to Oracle 11g User Schema as primary Source. Specifying a Schema Definition Using Connection Properties. Dns server database schema name for that you. Oracle9i user's schema geodatabase sdeoracle9i oracle9i is the. Connecting to relate Database PostgreSQL JDBC Driver. Connection URLs Reference Prisma Docs. Prisma needs a connection URL to your able to lash to exclude database eg when sending queries with Prisma Client or when changing the database schema. Strings for numerous databases and data stores Code samples are in C You district provide the values in red Microsoft SQL Server ODBC DSN. You already exists it be closed when statement though. If database connection string is connected, you may need to the databases with actual values. String connStr serverlocalhostuserrootdatabaseworldport3306. Configuration PostgREST 701 documentation. Note that using the overwrite option of ogr2ogr and lco SCHEMA option display the. Below is like database schema should we did anyone raised the. Each connection string is connecting to connect strings in the connections. Sql server database connection strings mostly require dba access and file system, depending on server? Mongooseconnect'mongodbusernamepasswordhostportdatabaseoptions. Syntax for an executable context. The database profile that depends on your default. -
NJ/NX-Series Database Connection CPU Units User's Manual
Machine Automation Controller NJ/NX-series Database Connection CPU Units User’s Manual NX701-££20 NX102-££20 NJ501-££20 NJ101-££20 CPU Unit W527-E1-10 NOTE • All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form, or by any means, mechanical, electronic, photocopying, recording, or oth- erwise, without the prior written permission of OMRON. • No patent liability is assumed with respect to the use of the information contained herein. Moreover, because OMRON is constantly striving to improve its high-quality products, the informa- tion contained in this manual is subject to change without notice. • Every precaution has been taken in the preparation of this manual. Nevertheless, OMRON as- sumes no responsibility for errors or omissions. Neither is any liability assumed for damages resulting from the use of the information contained in this publication. Trademarks • Sysmac and SYSMAC are trademarks or registered trademarks of OMRON Corporation in Japan and other countries for OMRON factory automation products. • Microsoft, Windows, Windows Vista, Excel, and SQL Server are either registered trademarks or trademarks of Microsoft Corporation in the United States and other countries. • EtherCAT® is registered trademark and patented technology, licensed by Beckhoff Automation GmbH, Germany. • ODVA, CIP, CompoNet, DeviceNet, and EtherNet/IP are trademarks of ODVA. • The SD and SDHC logos are trademarks of SD-3C, LLC. • Oracle, Java, and MySQL are registered trademarks of Oracle Corporation and/or its affiliates in the USA and other countries. • IBM and DB2 are registered trademarks of International Business Machines Corporation in the USA and other countries.