Copyright by Tommy Marcus Mcguire 2004
Total Page:16
File Type:pdf, Size:1020Kb
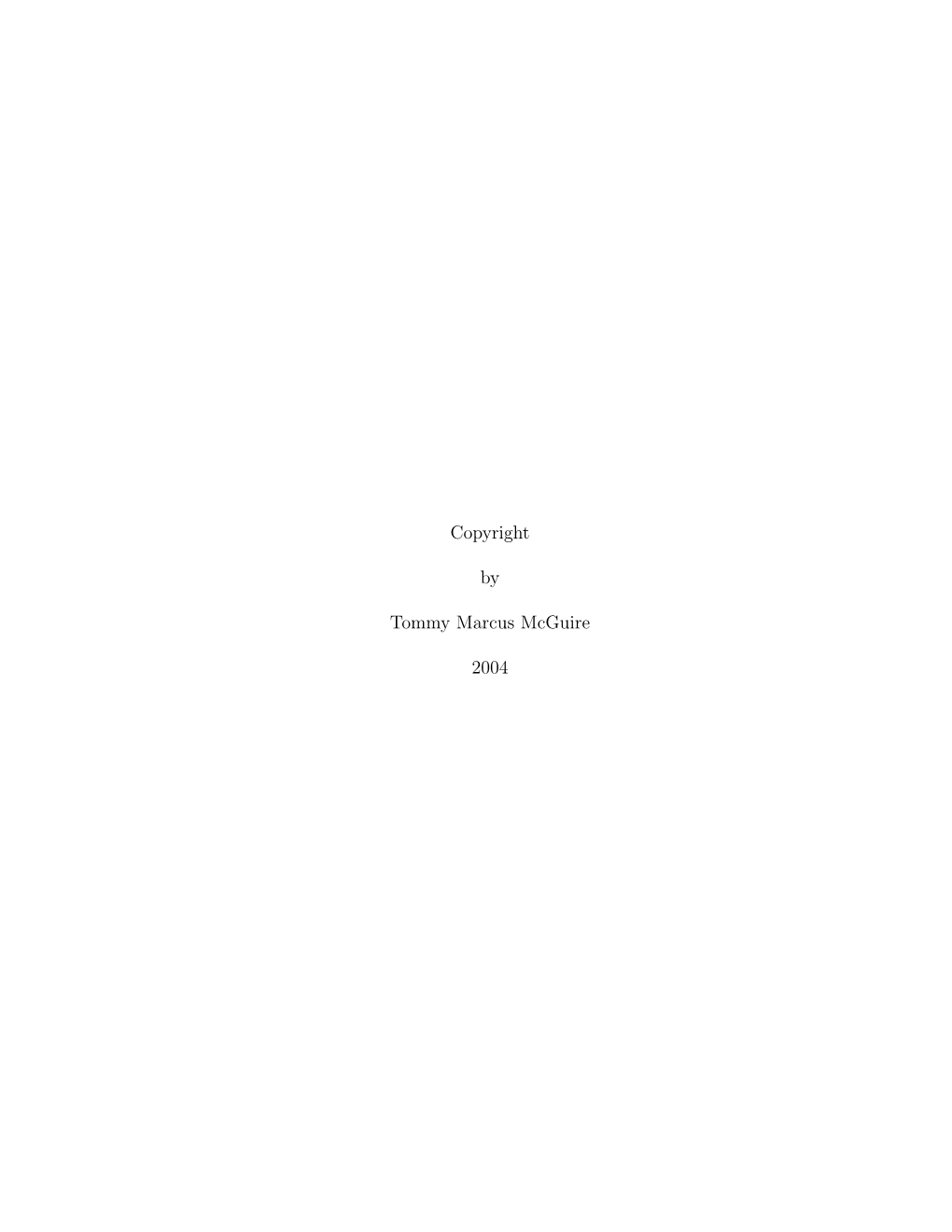
Load more
Recommended publications
-
Linux Ipv6 HOWTO (En) Peter Bieringer Linux Ipv6 HOWTO (En) Peter Bieringer
Linux IPv6 HOWTO (en) Peter Bieringer Linux IPv6 HOWTO (en) Peter Bieringer Abstract The goal of the Linux IPv6 HOWTO is to answer both basic and advanced questions about IPv6 on the Linux op- erating system. This HOWTO will provide the reader with enough information to install, configure, and use IPv6 applications on Linux machines. Intermediate releases of this HOWTO are available at mirrors.bieringer.de [http:// mirrors.bieringer.de/Linux+IPv6-HOWTO/] or mirrors.deepspace6.net [http://mirrors.deepspace6.net/Linux+IPv6- HOWTO/]. See also revision history for changes. Table of Contents 1. General .......................................................................................................................... 1 Copyright, license and others ........................................................................................ 1 Copyright ........................................................................................................... 1 License .............................................................................................................. 1 About the author ................................................................................................. 1 Category .................................................................................................................... 2 Version, History and To-Do .......................................................................................... 2 Version ............................................................................................................. -
Linux Ipv6 HOWTO (En)
Linux IPv6 HOWTO (en) Peter Bieringer pb at bieringer dot de Revision History Revision 0.65 2009-12-13 Revised by: PB Revision 0.64 2009-06-11 Revised by: PB Revision 0.60 2007-05-31 Revised by: PB Revision 0.51 2006-11-08 Revised by: PB The goal of the Linux IPv6 HOWTO is to answer both basic and advanced questions about IPv6 on the Linux operating system. This HOWTO will provide the reader with enough information to install, configure, and use IPv6 applications on Linux machines. Intermediate releases of this HOWTO are available at mirrors.bieringer.de or mirrors.deepspace6.net. See also revision history for changes. Linux IPv6 HOWTO (en) Table of Contents Chapter 1. General..............................................................................................................................................1 1.1. Copyright, license and others............................................................................................................1 1.1.1. Copyright.................................................................................................................................1 1.1.2. License.....................................................................................................................................1 1.1.3. About the author......................................................................................................................1 1.2. Category............................................................................................................................................2 -
Simple Network Administration Portal Box
Simple Network Administration Portal Box 1 194 W Evangilene Oaks Circle, The Woodlands, TX 77384 USA Off: (936)273-2266 Fax: (832)201-0847 1303 W Walnut Hill Ln, Suite 103/104, Irving TX 75038 www.s4consultants.com email: [email protected] EXECUTIVE SUMMARY S4 Consultants Inc. will be introducing "SNAP BOX" -Simple Network Administration Portal Box, in the US Market. This document includes a comprehensive analysis of the entire market, Product, Target Market, Marketing Research, Market Segmentation, Financial planning, Resource Estimates, Project Time Frame. In addition, product requirement specifications and preliminary architecture of this product are also included here. In summary, S4 should be introducing this product into the US Market. SNAP BOX would be profit inducing product since its an unexplored market. It has only one competitor and we believe the market potential has not been explored fully. We will be introducing our product firstly in US Market and then in the next phase we will be exploring other markets like Europe, India etc depending on the outcome of our experience in US Market. The Estimated Market size for this product is 1,600,000 (1.6 Million) Units. For SNAP BOX the End User has been classified into Small Offices, Home Offices. It includes the current businesses as well as the Start -Up companies. Start -Ups companies are classified into Heavy Users and Low Users in terms of IT Usage. Low ITusers wants IT as an enabler, a profitable component for their business. S4 conducted a Research study for new product introduction. The main objective of the study was to have a brief overview of the SNAP Box Market which included information about main competitors, end users, market size, financial details. -
Linux Ipv6 HOWTO
Linux IPv6 HOWTO Peter Bieringer <pb at bieringer.de> Revision History Revision Release 0.41 2003−03−22 Revised by: PB See revision history for more Revision Release 0.40 2003−02−10 Revised by: PB See revision history for more Revision Release 0.39.2 2003−02−10 Revised by: GK See revision history for more Revision Release 0.39 2003−01−13 Revised by: PB See revision history for more The goal of the Linux IPv6 HOWTO is to answer both basic and advanced questions about IPv6 on the Linux operating system. This HOWTO will provide the reader with enough information to install, configure, and use IPv6 applications on Linux machines. Linux IPv6 HOWTO Table of Contents Chapter 1. General..............................................................................................................................................1 1.1. Copyright, license and others............................................................................................................1 1.1.1. Copyright.................................................................................................................................1 1.1.2. License.....................................................................................................................................1 1.1.3. About the author......................................................................................................................1 1.2. Category............................................................................................................................................2 -
The Pcq Cloud Computing Resource
c o V E R S t o R Y SOFTWARE FOR PRIvaTE CLOUD The PCQ Cloud Computing Resource Kit Our hand-picked collection of all the free and open source software you’ll need to build your own private cloud - Raj Kumar Maurya Tools to Build & Manage a Private Cloud SparkleShare: A self-hosted cloud storage solution, Eucalyptus: Released as an open-source (under SparkleShare is a good storage option for files that change a FreeBSD-style license) infrastructure for cloud often and are accessed by a lot of people. computing on clusters that duplicates the functionality Syncany: Syncany is similar to Dropbox, but you can of Amazon EC2, Eucalyptus directly uses the Amazon use it with your own server or one of the popular public command-line tools. cloud services like Amazon, Google or Rackspace. It en- Xen hypervisor: The Xen hypervisor is a powerful crypts files locally, adding security for sensitive files. open source tool to build private and public clouds and it is a native (bare-metal) hypervisor providing services that Open Source NAS/SAN Building allow multiple computer operating systems to execute on Software the same computer hardware concurrently. FreeNAS: It can be used to turn standard hardware into a UShareSoft App Center: It provides open source BSD-based NAS device with lots of features. multi-tier software appliances (CloudStack, Eucalyptus, NAS4Free: Like FreeNAS, NAS4Free makes it easy to JasperSoft, WordPress&Talend) that can be used freely. create your own BSD-based storage solution from stan- Built with UForge (www.usharesoft.com) the appliances dard hardware. -
Linux Ipv6 HOWTO (En)
Linux IPv6 HOWTO (en) Peter Bieringer pb at bieringer dot de Revision History Revision Release 0.49 2005−10−03 Revised by: PB See revision history for more Revision Release 0.48.1 2005−01−15 Revised by: PB See revision history for more Revision Release 0.48 2005−01−11 Revised by: PB See revision history for more Revision Release 0.47.1 2005−01−01 Revised by: PB See revision history for more Revision Release 0.47 2004−08−30 Revised by: PB See revision history for more Revision Release 0.46 2004−03−16 Revised by: PB See revision history for more The goal of the Linux IPv6 HOWTO is to answer both basic and advanced questions about IPv6 on the Linux operating system. This HOWTO will provide the reader with enough information to install, configure, and use IPv6 applications on Linux machines. Linux IPv6 HOWTO (en) Table of Contents Chapter 1. General..............................................................................................................................................1 1.1. Copyright, license and others............................................................................................................1 1.1.1. Copyright.................................................................................................................................1 1.1.2. License.....................................................................................................................................1 1.1.3. About the author......................................................................................................................1 -
Network Attack Injection
UNIVERSIDADE DE LISBOA FACULDADE DE CIÊNCIAS DEPARTAMENTO DE INFORMÁTICA Network Attack Injection João Alexandre Simões Antunes DOUTORAMENTO EM INFORMÁTICA ESPECIALIDADE CIÊNCIA DA COMPUTAÇÃO 2012 UNIVERSIDADE DE LISBOA FACULDADE DE CIÊNCIAS DEPARTAMENTO DE INFORMÁTICA Network Attack Injection João Alexandre Simões Antunes Tese orientada pelo Prof. Doutor Nuno Fuentecilla Maia Ferreira Neves especialmente elaborada para a obtenção do grau de doutor em Informática, especialidade de Ciência da Computação. 2012 Abstract The increasing reliance on networked computer systems demands for high levels of dependability. Unfortunately, new threats and forms of attack are constantly emerging to exploit vulnerabilities in systems, compromising their correctness. An intrusion in a network server may affect its users and have serious repercussions in other services, possi- bly leading to new security breaches that can be exploited by further attacks. Software testing is the first line of defense in opposing attacks because it can support the discovery and removal of weaknesses in the systems. However, searching for flaws is a difficult and error-prone task, which has invariably overlooked vulnerabilities. The thesis proposes a novel methodology for vulnerability discovery that systematically generates and injects attacks, while monitoring and analyzing the target system. An attack that triggers an unexpected behavior provides a strong indication of the presence of a flaw. This attack can then be given to the developers as a test case to reproduce the anomaly and to assist in the correction of the problem. The main focus of the investigation is to provide a theoretical and experimental framework for the implementation and execution of at- tack injection on network servers. -
Linux Ipv6 HOWTO (It)
Linux IPv6 HOWTO (it) Peter Bieringer pb at bieringer.de Linux IPv6 HOWTO (it) Peter Bieringer L’obiettivo di questo HOWTO è quello di rispondere alle domande, sia di base che avanzate, sull’implementazione di IPv6 sul sistema operativo Linux. Questo HOWTO fornirà al lettore informazioni sufficienti per installare, configurare ed usare applicazioni che utilizzano IPv6 su macchine Linux. Traduzione a cura di Michele Ferritto (m.ferritto--at--virgilio.it) e revisione a cura di Daniele Masini (d.masini--at--tiscali.it). Diario delle revisioni Revisione Versione 0.47.it.1 04-09-2004 Corretto da: MF Si veda lo storico delle revisioni per maggiori dettagli Revisione Versione 0.46.it.1 24-03-2004 Corretto da: MF Si veda lo storico delle revisioni per maggiori dettagli Revisione Versione 0.45.it.2 23-01-2004 Corretto da: MF Si veda lo storico delle revisioni per maggiori dettagli Revisione Versione 0.45.it.1 12-01-2004 Corretto da: MF Si veda lo storico delle revisioni per maggiori dettagli Revisione Versione 0.44.2.it.1 16-10-2003 Corretto da: MF Si veda lo storico delle revisioni per maggiori dettagli Sommario 1. Generale.....................................................................................................................................................................1 1.1. Copyright, licenza d’uso e altro .....................................................................................................................1 1.2. Categoria ........................................................................................................................................................2 -
Setting up LAMP : Getting Linux, Apache, Mysql, and PHP Working Together
4337Book.fm Page i Saturday, June 19, 2004 6:21 PM Setting Up LAMP: Getting Linux, Apache, MySQL, and PHP Working Together 4337Book.fm Page ii Saturday, June 19, 2004 6:21 PM 4337Book.fm Page iii Saturday, June 19, 2004 6:21 PM Setting Up LAMP: Getting Linux, Apache, MySQL, and PHP Working Together Eric Rosebrock Eric Filson San Francisco • London 4337Book.fm Page iv Saturday, June 19, 2004 6:21 PM Associate Publisher: Joel Fugazzotto Acquisitions Editor: Tom Cirtin Developmental Editor: Tom Cirtin Production Editor: Lori Newman Technical Editor: Sean Schluntz Copyeditor: Sharon Wilkey Compositor: Laurie Stewart, Happenstance Type-O-Rama Proofreaders: Nancy Riddiough, Laurie O’Connell Indexer: Nancy Guenther Cover Designer: Caryl Gorska, Gorska Design Cover Photographer: Peter Samuels, Tony Stone Copyright © 2004 SYBEX Inc., 1151 Marina Village Parkway, Alameda, CA 94501. World rights reserved. No part of this publication may be stored in a retrieval system, transmitted, or reproduced in any way, including but not limited to photocopy, photograph, magnetic, or other record, without the prior agreement and written permission of the publisher. Library of Congress Card Number: 2004104101 ISBN: 0-7821-4337-7 SYBEX and the SYBEX logo are either registered trademarks or trademarks of SYBEX Inc. in the United States and/or other countries. Transcend Technique is a trademark of SYBEX Inc. TRADEMARKS: SYBEX has attempted throughout this book to distinguish proprietary trademarks from descriptive terms by following the capitalization style used by the manufacturer. The author and publisher have made their best efforts to prepare this book, and the content is based upon final release software whenever pos- sible. -
Linux Ipv6 HOWTO (En)
Linux IPv6 HOWTO (en) Peter Bieringer pb at bieringer dot de Revision History Revision 0.61 2007-10-06 Revised by: PB Revision 0.60 2007-05-31 Revised by: PB Revision 0.51 2006-11-08 Revised by: PB The goal of the Linux IPv6 HOWTO is to answer both basic and advanced questions about IPv6 on the Linux operating system. This HOWTO will provide the reader with enough information to install, configure, and use IPv6 applications on Linux machines. Intermediate releases of this HOWTO are available at mirrors.bieringer.de or mirrors.deepspace6.net. See also revision history for changes. Linux IPv6 HOWTO (en) Table of Contents Chapter 1. General..............................................................................................................................................1 1.1. Copyright, license and others............................................................................................................1 1.1.1. Copyright.................................................................................................................................1 1.1.2. License.....................................................................................................................................1 1.1.3. About the author......................................................................................................................1 1.2. Category............................................................................................................................................2 1.3. Version, History and To-Do.............................................................................................................2 -
Linux Ipv6 HOWTO (Pt BR)
Linux IPv6 HOWTO (pt_BR) Peter Bieringer pb at bieringer dot de Linux IPv6 HOWTO (pt_BR) by Peter Bieringer A meta deste HOWTO de IPv6 em Linux é responder as questões básicas e avançadas sobre a versão 6 do protocolo IP em um sistema com Linux. Este HOWTO dará ao leitor informação suficiente para instalar, configurar e usar aplicações IPv6 em máquinas com o Linux. Versões intermediárias deste HOWTO estão disponíveis nos endereços mirrors.bieringer.de (http://mirrors.bieringer.de/Linux+IPv6-HOWTO/) ou mirrors.deepspace6.net (http://mirrors.deepspace6.net/Linux+IPv6-HOWTO/). Veja também revision history para saber das mudanças. Revision History Revision 0.66wip 2020-04-04 Revised by: PB Revision 0.65 2009-12-13 Revised by: PB Revision 0.64 2009-06-11 Revised by: PB Revision 0.60 2007-05-31 Revised by: PB Revision 0.51 2006-11-08 Revised by: PB Table of Contents 1. Geral...........................................................................................................................................................................1 1.1. Copyright, licença e outros.............................................................................................................................1 1.1.1. Copyright...........................................................................................................................................1 1.1.2. Licença...............................................................................................................................................1 1.1.3. Sobre o autor......................................................................................................................................1 -
The Posadis DNS Server User Documentation for Posadis 0.60.5
The Posadis DNS server user documentation for Posadis 0.60.5 Tutorial and reference manual for Posadis Updated for Posadis 0.60.5 Meilof Veeningen <[email protected]> Table of Contents Table of Contents.......................................................................................2 Part 1............................................................................................4 Do I need DNS?.........................................................................................5 The Domain Name System........................................................................6 The Authoritative DNS tree..................................................................6 A distributed system..............................................................................7 Caching..................................................................................................8 Forwarding............................................................................................9 So, what do I do next?...........................................................................9 Authoritative DNS servers..................................................................10 Reverse-mapping.................................................................................11 So, what way do I go now?.................................................................11 Installing your DNS server......................................................................12 Configuring Posadis............................................................................12