Memory Management Algorithms and Implementation in C/C++
Total Page:16
File Type:pdf, Size:1020Kb
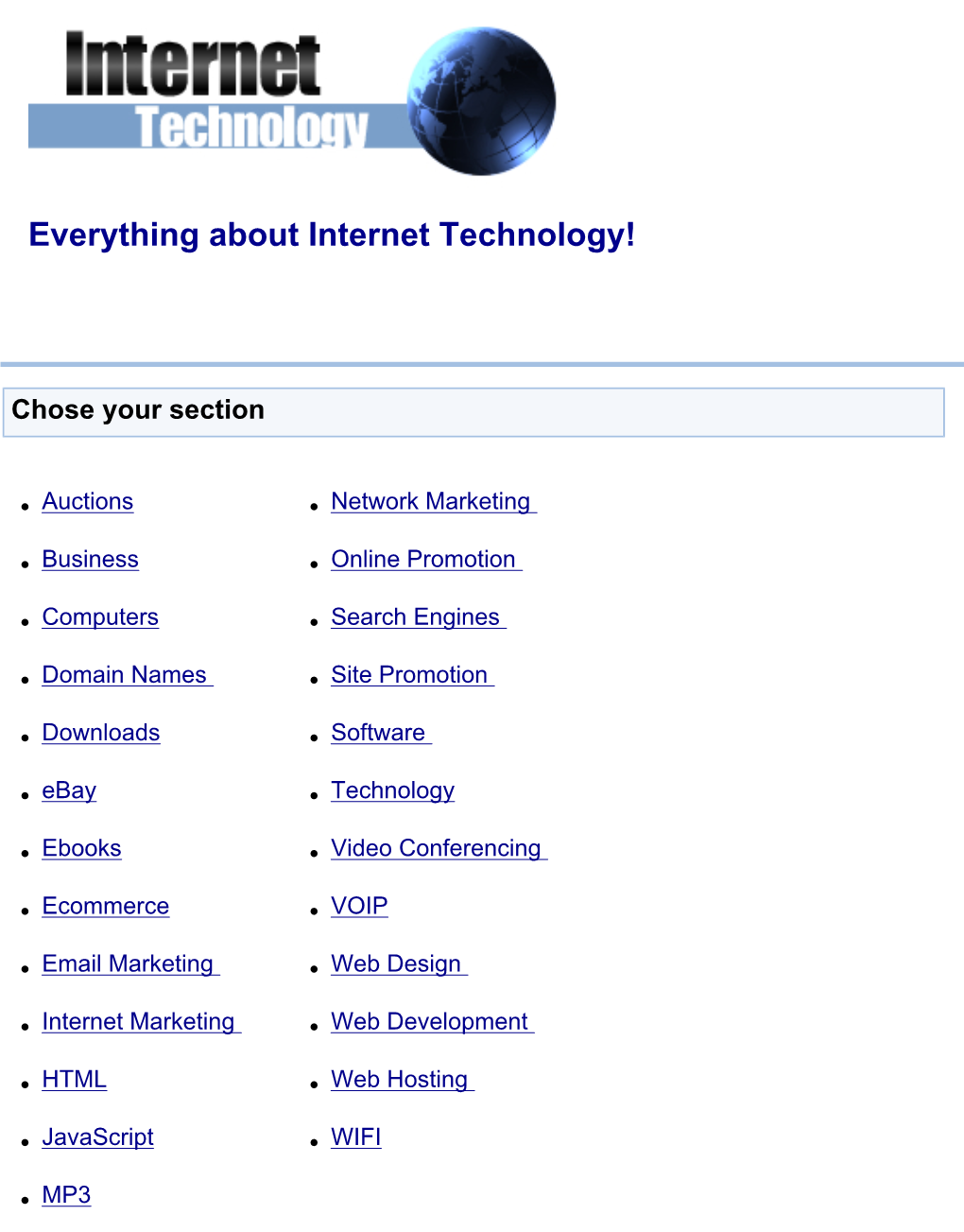
Load more
Recommended publications
-
Kontron / ICS Advent SB586P(V) Manual (Pdf)
Full-service, independent repair center -~ ARTISAN® with experienced engineers and technicians on staff. TECHNOLOGY GROUP ~I We buy your excess, underutilized, and idle equipment along with credit for buybacks and trade-ins. Custom engineering Your definitive source so your equipment works exactly as you specify. for quality pre-owned • Critical and expedited services • Leasing / Rentals/ Demos equipment. • In stock/ Ready-to-ship • !TAR-certified secure asset solutions Expert team I Trust guarantee I 100% satisfaction Artisan Technology Group (217) 352-9330 | [email protected] | artisantg.com All trademarks, brand names, and brands appearing herein are the property o f their respective owners. Find the Kontron / ICS Advent SB586PV at our website: Click HERE Model SB586P(V) Product Manual MANUAL NUMBER : 00431-027-3C Page - ii FOREWORD This product manual provides information to install, operate and or program the referenced product(s) manufactured or distributed by ICS Advent. The following pages contain information regarding the war- ranty and repair policies. Technical assistance is available at: 800-480-0044. Manual Errors, Omissions and Bugs: A "Bug Sheet" is included as the last page of this manual. Please use the "Bug Sheet" if you experience any problems with the manual that requires correction. The information in this document is provided for reference only. ICS Advent does not assume any liability arising from the application or use of the information or products described herein. This document may contain or reference information and products protected by copyrights or patents and does not convey any license under the patent rights of ICS Advent, nor the rights of others. -
SOS Internals
Understanding a Simple Operating System SOS is a Simple Operating System designed for the 32-bit x86 architecture. Its purpose is to understand basic concepts of operating system design. These notes are meant to help you recall the class discussions. Chapter 1 : Starting Up SOS 3 Registers in the IA-32 x86 Architecture BIOS (Basic Input/Ouput System) Routines Real Mode Addressing Organization of SOS on Disk and Memory Master Boot Record SOS Startup A20 Line 32-bit Protected Mode Addressing Privilege Level Global Descriptor Table (GDT) More on Privilege Levels The GDT Setup Enabling Protected Mode Calling main() Chapter 2 : SOS Initializations 10 In main() Disk Initialization Display Initialization Setting Up Interrupt Handlers Interrupt Descriptor Table (IDT) A Default Interrupt Handler Load IDT The Programmable Interrupt Controller (PIC) The Keyboard Interrupt Handler Starting the Console Putting It All Together Chapter 3: SOS1 – Single-tasking SOS 16 Running User Programs GDT Entries Default Exception Handler User Programs Executable Format System Calls Creating User Programs The run Command Understanding a Simple Operating System The DUMB Memory Manager Program Address Space Process Control Block Switching to a User Program Kernel-Mode Stack Chapter 4 : SOS2 – Multi-tasking SOS 24 Running Multiple User Programs NAÏVE Memory Manager Programmable Interval Timer (PIT) Process States Timer Interrupt Handler Sleep System Call The run Command Process Queue The Scheduler The Complete Picture ps Command Chapter 5 : SOS3 – Paging in SOS 31 -
The Bootstrap the Where Are Wenow?
Dedicated Operating System March2011 Prof. Dr.Antônio AugustoFröhlich http://www.lisha.ufsc.br/Guto Getting ready for the OS for the ready Getting [email protected] LISHA/UFSC The Boot: The March 2011 (http://www.lisha.ufsc.br) 1 Dedicated Operating System March2011 ● ● ● BIOSgot the system ready for BIOSbrought the system on ● Lots of “jmp” so far, no calls, why? ● First instruction fetched ● initializedBIOS a complex architecture Where the stack?Where is 0x7c00 BIST, POST, hooks The Bootstrap Where are we now? we are Where (http://www.lisha.ufsc.br) =>Today class 2 EPOS Bootstrap: m src/boot/pc_boot.S e t ; CONSTANTS s ;============================================================ y ; PHYSICAL MEMORY MAP S ; 0x0000 0000 -+-----------------------+ BOOT_IDT ; | IDT (4 K) | g ; 0x0000 1000 -+-----------------------+ BOOT_GDT ; | GDT (4 K) | n i ; 0x0000 2000 -+-----------------------+ t ; : : a ; | BOOT STACK (23 K) | r ; 0x0000 7c00 -+-----------------------+ BOOTSTRAP_STACK e ; | BOOT CODE (512 b) | BOOTSTRAP_CODE p ; 0x0000 7e00 -+-----------------------+ ; | RESERVED (512 b) | O ; 0x0000 8000 -+-----------------------+ DISK_IMAGE ; | DISK IMAGE (608 K) | d ; : : e ; | | t ; 0x000a 0000 -+-----------------------+ a ; | UNUSED (384K) | c ; : : i ; | | d ; 0x000f f000 -+-----------------------+ e D March 2011 (http://www.lisha.ufsc.br) 3 EPOS Bootstrap: Notes m e t s Code to be ran at real mode (16 bits) y S Interrupts (IDT) ● g At real mode, always at 0x0000 n ● i At protected mode, anywhere (IDTR) t a Segmentation (GDT) r ● e Always -
OS Structures and System Calls
COS 318: Operating Systems OS Structures and System Calls Kai Li Computer Science Department Princeton University (http://www.cs.princeton.edu/courses/cos318/) Outline Protection mechanisms OS structures System and library calls 2 Protection Issues CPU Kernel has the ability to take CPU away from users to prevent a user from using the CPU forever Users should not have such an ability Memory Prevent a user from accessing others’ data Prevent users from modifying kernel code and data structures I/O Prevent users from performing “illegal” I/Os Question What’s the difference between protection and security? 3 Architecture Support: Privileged Mode An interrupt or exception (INT) User mode Kernel (privileged) mode • Regular instructions • Regular instructions • Access user memory • Privileged instructions • Access user memory • Access kernel memory A special instruction (IRET) 4 Privileged Instruction Examples Memory address mapping Flush or invalidate data cache Invalidate TLB entries Load and read system registers Change processor modes from kernel to user Change the voltage and frequency of processor Halt a processor Reset a processor Perform I/O operations 5 x86 Protection Rings Privileged instructions Can be executed only When current privileged Level (CPR) is 0 Operating system kernel Level 0 Operating system services Level 1 Level 2 Applications Level 3 6 Layered Structure Hiding information at each layer Layered dependency Examples Level N THE (6 layers) . MS-DOS (4 layers) . Pros Level 2 Layered abstraction -
Asmc Macro Assembler Reference Asmc Macro Assembler Reference
Asmc Macro Assembler Reference Asmc Macro Assembler Reference This document lists some of the differences between Asmc, JWasm, and Masm. In This Section Asmc Command-Line Option Describes the Asmc command-line option. Asmc Error Messages Describes Asmc fatal and nonfatal error messages and warnings. Asmc Extensions Provides links to topics discussing Masm versus Asmc. Directives Reference Provides links to topics discussing the use of directives in Asmc. Symbols Reference Provides links to topics discussing the use of symbols in Asmc. Change Log | Forum Asmc Macro Assembler Reference Asmc Command-Line Reference Assembles and links one or more assembly-language source files. The command-line options are case sensitive. ASMC [[options]] filename [[ [[options]] filename]] options The options listed in the following table. Set CPU: 0=8086 (default), 1=80186, 2=80286, 3=80386, 4=80486, /[0|1|..|10][p] 5=Pentium,6=PPro,7=P2,8=P3,9=P4,10=x86-64. [p] allows privileged instructions. /assert Generate .assert(code). Same as .assert:on. /bin Generate plain binary file. Push user registers before stack-frame is created in a /Cs proc. /coff Generate COFF format object file. /Cp Preserves case of all user identifiers. /Cu Maps all identifiers to upper case (default). Link switch used with /pe -- subsystem:console /cui (default). /Cx Preserves case in public and extern symbols. Defines a text macro with the given name. If value is /Dsymbol[[=value]] missing, it is blank. Multiple tokens separated by spaces must be enclosed in quotation marks. /enumber Set error limit number. /elf Generate 32-bit ELF object file. /elf64 Generate 64-bit ELF object file. -
EZ-USB® FX3™ Technical Reference Manual
EZ-USB® FX3™ Technical Reference Manual Spec No.: 001-76074 Rev. *E May 31, 2017 Cypress Semiconductor 198 Champion Court San Jose, CA 95134-1709 www.cypress.com Copyrights Copyrights © Cypress Semiconductor Corporation, 2012-2017. This document is the property of Cypress Semiconductor Corporation and its subsidiaries, including Spansion LLC ("Cypress"). This document, including any software or firmware included or refer- enced in this document ("Software"), is owned by Cypress under the intellectual property laws and treaties of the United States and other countries worldwide. Cypress reserves all rights under such laws and treaties and does not, except as spe- cifically stated in this paragraph, grant any license under its patents, copyrights, trademarks, or other intellectual property rights. If the Software is not accompanied by a license agreement and you do not otherwise have a written agreement with Cypress governing the use of the Software, then Cypress hereby grants you a personal, non-exclusive, nontransferable license (without the right to sublicense) (1) under its copyright rights in the Software (a) for Software provided in source code form, to modify and reproduce the Software solely for use with Cypress hardware products, only internally within your organi- zation, and (b) to distribute the Software in binary code form externally to end users (either directly or indirectly through resell- ers and distributors), solely for use on Cypress hardware product units, and (2) under those claims of Cypress's patents that are infringed by the Software (as provided by Cypress, unmodified) to make, use, distribute, and import the Software solely for use with Cypress hardware products. -
Computing :: Operatingsystems :: DOS Beyond 640K 2Nd
DOS® Beyond 640K 2nd Edition DOS® Beyond 640K 2nd Edition James S. Forney Windcrest®/McGraw-Hill SECOND EDITION FIRST PRINTING © 1992 by James S. Forney. First Edition © 1989 by James S. Forney. Published by Windcrest Books, an imprint of TAB Books. TAB Books is a division of McGraw-Hill, Inc. The name "Windcrest" is a registered trademark of TAB Books. Printed in the United States of America. All rights reserved. The publisher takes no responsibility for the use of any of the materials or methods described in this book, nor for the products thereof. Library of Congress Cataloging-in-Publication Data Forney, James. DOS beyond 640K / by James S. Forney. - 2nd ed. p. cm. Rev. ed. of: MS-DOS beyond 640K. Includes index. ISBN 0-8306-9717-9 ISBN 0-8306-3744-3 (pbk.) 1. Operating systems (Computers) 2. MS-DOS (Computer file) 3. PC -DOS (Computer file) 4. Random access memory. I. Forney, James. MS-DOS beyond 640K. II. Title. QA76.76.063F644 1991 0058.4'3--dc20 91-24629 CIP TAB Books offers software for sale. For information and a catalog, please contact TAB Software Department, Blue Ridge Summit, PA 17294-0850. Acquisitions Editor: Stephen Moore Production: Katherine G. Brown Book Design: Jaclyn J. Boone Cover: Sandra Blair Design, Harrisburg, PA WTl To Sheila Contents Preface Xlll Acknowledgments xv Introduction xvii Chapter 1. The unexpanded system 1 Physical limits of the system 2 The physical machine 5 Life beyond 640K 7 The operating system 10 Evolution: a two-way street 12 What else is in there? 13 Out of hiding 13 Chapter 2. -
Protected Mode - Wikipedia
2/12/2019 Protected mode - Wikipedia Protected mode In computing, protected mode, also called protected virtual address mode,[1] is an operational mode of x86- compatible central processing units (CPUs). It allows system software to use features such as virtual memory, paging and safe multi-tasking designed to increase an operating system's control over application software.[2][3] When a processor that supports x86 protected mode is powered on, it begins executing instructions in real mode, in order to maintain backward compatibility with earlier x86 processors.[4] Protected mode may only be entered after the system software sets up one descriptor table and enables the Protection Enable (PE) bit in the control register 0 (CR0).[5] Protected mode was first added to the x86 architecture in 1982,[6] with the release of Intel's 80286 (286) processor, and later extended with the release of the 80386 (386) in 1985.[7] Due to the enhancements added by protected mode, it has become widely adopted and has become the foundation for all subsequent enhancements to the x86 architecture,[8] although many of those enhancements, such as added instructions and new registers, also brought benefits to the real mode. Contents History The 286 The 386 386 additions to protected mode Entering and exiting protected mode Features Privilege levels Real mode application compatibility Virtual 8086 mode Segment addressing Protected mode 286 386 Structure of segment descriptor entry Paging Multitasking Operating systems See also References External links History https://en.wikipedia.org/wiki/Protected_mode -
Open WATCOM Programmer's Guide
this document downloaded from... Use of this document the wings of subject to the terms and conditions as flight in an age stated on the website. of adventure for more downloads visit our other sites Positive Infinity and vulcanhammer.net chet-aero.com Watcom FORTRAN 77 Programmer's Guide Version 1.8 Notice of Copyright Copyright 2002-2008 the Open Watcom Contributors. Portions Copyright 1984-2002 Sybase, Inc. and its subsidiaries. All rights reserved. Any part of this publication may be reproduced, transmitted, or translated in any form or by any means, electronic, mechanical, manual, optical, or otherwise, without the prior written permission of anyone. For more information please visit http://www.openwatcom.org/ Portions of this manual are reprinted with permission from Tenberry Software, Inc. ii Preface The Watcom FORTRAN 77 Programmer's Guide includes the following major components: · DOS Programming Guide · The DOS/4GW DOS Extender · Windows 3.x Programming Guide · Windows NT Programming Guide · OS/2 Programming Guide · Novell NLM Programming Guide · Mixed Language Programming · Common Problems Acknowledgements This book was produced with the Watcom GML electronic publishing system, a software tool developed by WATCOM. In this system, writers use an ASCII text editor to create source files containing text annotated with tags. These tags label the structural elements of the document, such as chapters, sections, paragraphs, and lists. The Watcom GML software, which runs on a variety of operating systems, interprets the tags to format the text into a form such as you see here. Writers can produce output for a variety of printers, including laser printers, using separately specified layout directives for such things as font selection, column width and height, number of columns, etc. -
COMPILING FUNCTIONAL PROGRAMMING CONSTRUCTS to a LOGIC ENGINE by Harvey Abramson and Peter Ludemann Technical Report 86-24 November 1986
COMPILING FUNCTIONAL PROGRAMMING CONSTRUCTS TO A LOGIC ENGINE by Harvey Abramson and Peter Ludemann Technical Report 86-24 November 1986 Compiling Functional Programming Constructs to a Logic Engine Harvey Abramson t and Peter Ltidemann:I: Department of Computer Science University of British Columbia Vancouver, B.C., Canada V6T 1W5 Copyright © 1986 Abstract In this paper we consider how various constructs used in functional programming can be efficiently translated to code for a Prolog engine (designed by Ltidemann) similar in spirit but not identical to the Warren machine. It turns out that this Prolog engine which contains a delay mechanism designed to permit co routining and sound implementation of negation is sufficient to handle all common functional programming constructs; indeed, such a machine has about the same efficiency as a machine designed specifically for a functional programming language. This machine has been fully implemented. t Present address: Department of Computer Science, Bristol University, Bristol, England . f Present address: IBM Canada Ltd., 1 Park Centre, 895 Don Mills Road, North York, Ontario, Canada M3C 1W3 The proposals for combining the two paradigms Compiling Functional Programming have been roughly classified in [BoGi86] as being Constructs to a Logic Engine either functional plus logic or logic plus functional. Harvey Abramson t and Peter Ltidemanni In the former approach, invertibility, nondeterminism Department of Computer Science and unification are added to some higher order functional language; in the latter approach, first-order University of British Columbia functions are added to a first-order logic with an Vancouver, B.C., Canada V6T lWS equality theory. We do not take any position as to Copyright© 1986 which approach is best, but since a logic machine already deals with unification, nondeterminism and invertibility, we have taken, from an implementation Abstract viewpoint, the logic plus functional approach. -
Operating System
OPERATING SYSTEM INDEX LESSON 1: INTRODUCTION TO OPERATING SYSTEM LESSON 2: FILE SYSTEM – I LESSON 3: FILE SYSTEM – II LESSON 4: CPU SCHEDULING LESSON 5: MEMORY MANAGEMENT – I LESSON 6: MEMORY MANAGEMENT – II LESSON 7: DISK SCHEDULING LESSON 8: PROCESS MANAGEMENT LESSON 9: DEADLOCKS LESSON 10: CASE STUDY OF UNIX LESSON 11: CASE STUDY OF MS-DOS LESSON 12: CASE STUDY OF MS-WINDOWS NT Lesson No. 1 Intro. to Operating System 1 Lesson Number: 1 Writer: Dr. Rakesh Kumar Introduction to Operating System Vetter: Prof. Dharminder Kr. 1.0 OBJECTIVE The objective of this lesson is to make the students familiar with the basics of operating system. After studying this lesson they will be familiar with: 1. What is an operating system? 2. Important functions performed by an operating system. 3. Different types of operating systems. 1. 1 INTRODUCTION Operating system (OS) is a program or set of programs, which acts as an interface between a user of the computer & the computer hardware. The main purpose of an OS is to provide an environment in which we can execute programs. The main goals of the OS are (i) To make the computer system convenient to use, (ii) To make the use of computer hardware in efficient way. Operating System is system software, which may be viewed as collection of software consisting of procedures for operating the computer & providing an environment for execution of programs. It’s an interface between user & computer. So an OS makes everything in the computer to work together smoothly & efficiently. Figure 1: The relationship between application & system software Lesson No. -
Parameter Passing, Generics, Exceptions
Lecture 9: Parameter Passing, Generics and Polymorphism, Exceptions COMP 524 Programming Language Concepts Stephen Olivier February 12, 2008 Based on notes by A. Block, N. Fisher, F. Hernandez-Campos, and D. Stotts The University of North Carolina at Chapel Hill Parameter Passing •Pass-by-value: Input parameter •Pass-by-result: Output parameter •Pass-by-value-result: Input/output parameter •Pass-by-reference: Input/output parameter, no copy •Pass-by-name: Effectively textual substitution The University of North Carolina at Chapel Hill 2 Pass-by-value int m=8, i=5; foo(m); print m; # print 8 proc foo(int b){ b = b+5; } The University of North Carolina at Chapel Hill 3 Pass-by-reference int m=8; foo(m); print m; # print 13 proc foo(int b){ b = b+5; } The University of North Carolina at Chapel Hill 4 Pass-by-value-result int m=8; foo(m); print m; # print 13 proc foo(int b){ b = b+5; } The University of North Carolina at Chapel Hill 5 Pass-by-name •Arguments passed by name are re-evaluated in the caller’s referencing environment every time they are used. •They are implemented using a hidden-subroutine, known as a thunk. •This is a costly parameter passing mechanism. •Think of it as an in-line substitution (subroutine code put in-line at point of call, with parameters substituted). •Or, actual parameters substituted textually in the subroutine body for the formulas. The University of North Carolina at Chapel Hill 6 Pass-by-name array A[1..100] of int; array A[1..100] of int; int i=5; int i=5; foo(A[i], i); foo(A[i]); print A[i]; #print A[6]=7