Practical Uses of Wwdotnetbridge to Extend Your VFP Applications
Total Page:16
File Type:pdf, Size:1020Kb
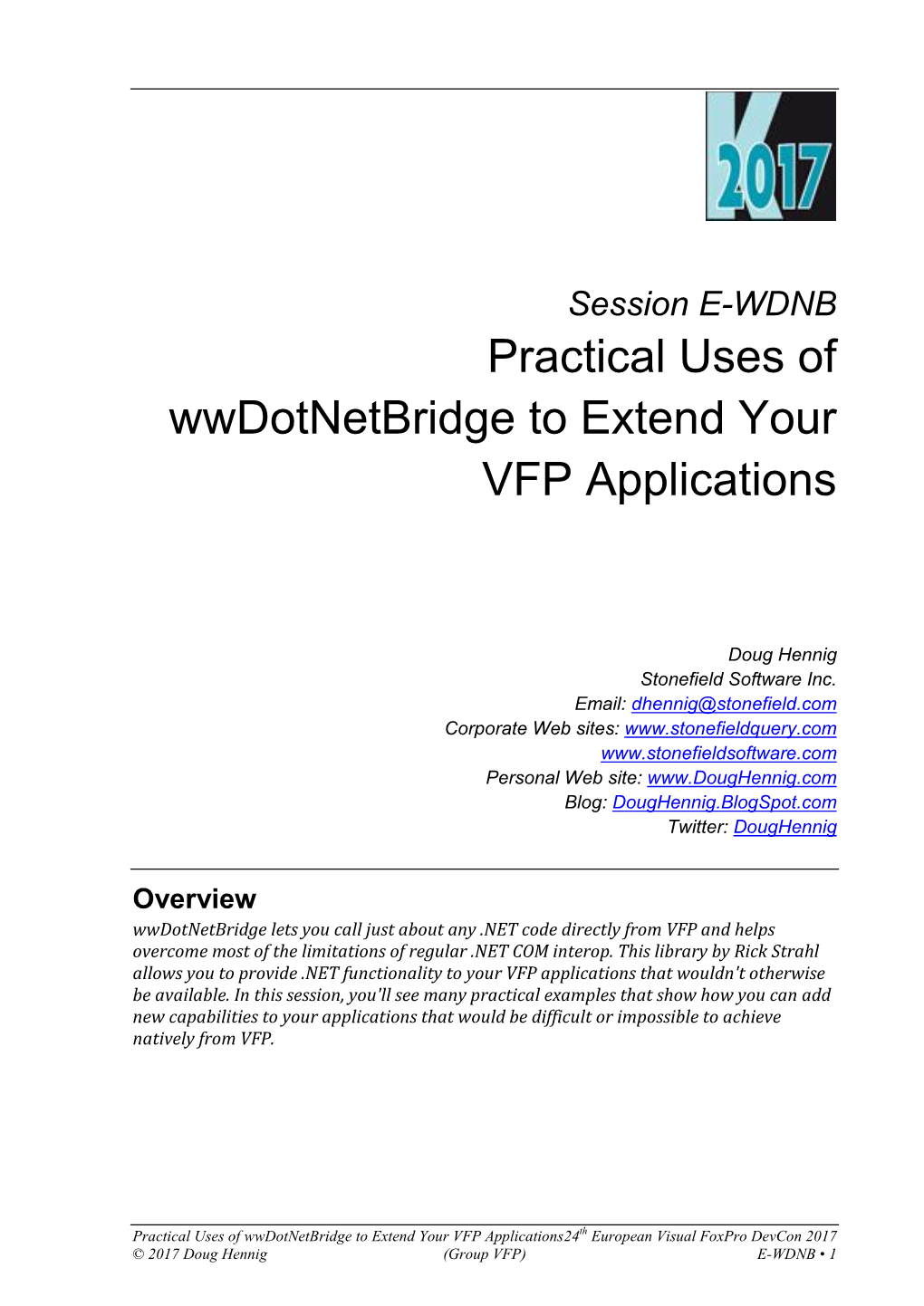
Load more
Recommended publications
-
Visual Foxpro
PRE201 Introduction to Visual FoxPro Ted Roche Ted Roche & Associates, LLC Visual FoxPro DevCon 2001 Ground Rules Pagers and cell phones silent, please. Rest breaks as appropriate How to ask questions Administrivia Conference Binder Schedule – Some sessions only given once Trade Show – T-shirt Tuesday, Drawing Wed. Evaluations - help the community Closing session questions – Wednesday noon Drink Tickets! Visual FoxPro DevCon 2001 Goals for this morning Learn the terminology used in VFP Understand the tools of VFP has Understand how VFP stores and retrieves data Know what applications VFP can create Know resources to learn more Visual FoxPro DevCon 2001 Who is Ted Roche? President of TR&A, LLC Consulting Microsoft Certified Solution Developer, Microsoft Certified Systems Engineer Co-Author of Hacker’s Guide to VFP 6 Author of Essential SourceSafe Microsoft Support MVP Visual FoxPro DevCon 2001 Outline Ground Rules & Pop Quiz Episode I: It’s the Data Part B: It’s the Coding Act III: Advanced Topics Part 4: Putting it all together Epilogue: So, Now What? Visual FoxPro DevCon 2001 What is Visual FoxPro? Visual FoxPro is… … a standalone tool for data manipulation. … a development tool for standalone, LAN, client-server, COM and Web applications. … a database engine. … a programming language. … part of Visual Studio. … an integral part of Microsoft Windows. … a religionVisual. FoxPro DevCon 2001 What is Visual FoxPro? Visual FoxPro is… … a standalone tool for data manipulation. … a development tool for standalone, LAN, client-server, COM and Web applications. … a database engine. … a programming language. … part of Visual Studio. … an integral part of Microsoft Windows. -
Visual Foxpro
GenGold - Local Business Partner Catalog Accounting/Tax Services Accountrrail, LLC Iselin, NJ 08830 347-512-0126 www.accountrail.com 10% discount and free initial consultation www.accountrail.com Air Sea Land Travel 155 Kearny Avenue Kearny, NJ 07032 201-991-3366 10% discount Apple Multiservice, LLC 65 Gould Avenue Paterson, NJ 07503 973-460-6904 For new customer $30.00 discount B & B Tax Preparation 611 Broadway Bayonne, NJ 07002 201-436-7600 www.jaragency.com 50% discount on 1st year tax prepartation service www.jaragency.com C.F.T. Tax Inc 2053 Woodbridge Avenue Suite A Edison, NJ 08817 732-626-5900 www.cfttaxinc.com 5% discount www.cfttaxinc.com Cadi's Muti Service 497 Central Avenue Newark, NJ 07107 973-732-3258 10% discount Castellano Associates 335 Union Avenue Belleville, NJ 07109 973-751-8923 Free consultation Page 1 The Provident Bank 06/18/15 GenGold - Local Business Partner Catalog Accounting/Tax Services Direct Point Services 34 Cianci Street Paterson, NJ 07501 973-345-4800 10% discount on Income Tax 2014; 1 free recharge international call Elliot L. Kaplan Cpa 56 Bryant Avenue Edison, NJ 08820 732-593-8200 10% discount Fabio Financial Services 105 Lock Street Suite 403 Newark, NJ 07103 973-824-7474 Free fries with purchase of lobster roll Monday thru Thursday M & I Services, Inc. 2 Fish House Road Kearny, NJ 07032 201-955-0013 10% discount on tax returns preparation before March 1 2015 MBC Firm 55 Washington Street East Orange, NJ 07017 973-672-1300 10% discount on first service Middlesex County Clerical Tax and Recording Co., L 434 Westgate Drive Edison, NJ 08820 973-568-4490 10% discount Xpertax Corp. -
Calling .NET Code from VFP the Easy Way
Session E-CALL Calling .NET Code from VFP the Easy Way Doug Hennig Stonefield Software Inc. Email: [email protected] Corporate Web site: www.stonefieldquery.com Personal Web site : www.DougHennig.com Blog: DougHennig.BlogSpot.com Twitter: DougHennig Overview At the German DevCon 2011, Doug’s “Creating ActiveX Controls for VFP Using .NET” session showed how to create .NET components that can be used in Visual FoxPro applications. However, these types of controls suffer from a couple of issues: they have to be registered for COM on the customer’s system and there are limitations in working with .NET Interop in VFP that prevent many things from working correctly. This session shows how Rick Strahl’s wwDotNetBridge eliminates these issues and provides some practical examples of how this tool can be used in your applications. Calling .NET Code from VFP the Easy Way 20th European Visual FoxPro DevCon 2013 © 2013 Doug Hennig (Group .NET) E-CALL • 1 Introduction The Microsoft .NET framework has a lot of powerful features that aren’t available in VFP. For example, dealing with Web Services is really ugly from VFP but is simple in .NET. .NET also provides access to most operating system functions, including functions added in newer version of the OS. While these functions are also available using the Win32 API, many of them can’t be called from VFP because they require callbacks and other features VFP doesn’t support, and accessing this functions via .NET is easier anyway. Fortunately, there are various mechanisms that allow you to access .NET code from VFP applications. -
Mhtml:File://C:\Users\Jbustamante
Visual FoxPro Toolkit for .NET The Visual FoxPro Toolkit for .NET is a class library with over 225 Visual FoxPro functions for use with any .NET language. The included VFPToolkitNET.DLL is a .NET managed code DLL (not a COM wrapper) and does not require Visual FoxPro. The functions supported execute extremely fast since they are all written in .NET and compiled into a managed code .NET DLL. Included is a reference and tutorial stand-alone CHM help file as well as Visual Studio .NET integrated dynamic help using the same CHM help file. Full IntelliSense and Dynamic Help is enabled for the VFP functions when programming in any .NET language. Using the VFP Toolkit or .NET, most Visual FoxPro functions become available in Visual Basic .NET or any other .NET language. Functions like STRTOFILE() convert a string to a file in only one line of code. The Visual FoxPro Toolkit for .NET does not teach developers Visual Studio .NET programming, but it does enable developers to do .NET programming much quicker and write less code based on what they are familiar with combined with how these functions reduce coding overall. It is not pseudo-VFP syntax, it is "real" VFP syntax and works most naturally in Visual Basic .NET since it no namespacing is required. The Windows .NET Framework and Visual Basic .NET (or any .NET language) must still be learned. What this toolkit does is assist Visual FoxPro developers using .NET since there is a much quicker learning curve for .NET programming having these functions available. The Visual FoxPro Toolkit for .NET is not a replacement to any .NET language. -
1. with Examples of Different Programming Languages Show How Programming Languages Are Organized Along the Given Rubrics: I
AGBOOLA ABIOLA CSC302 17/SCI01/007 COMPUTER SCIENCE ASSIGNMENT 1. With examples of different programming languages show how programming languages are organized along the given rubrics: i. Unstructured, structured, modular, object oriented, aspect oriented, activity oriented and event oriented programming requirement. ii. Based on domain requirements. iii. Based on requirements i and ii above. 2. Give brief preview of the evolution of programming languages in a chronological order. 3. Vividly distinguish between modular programming paradigm and object oriented programming paradigm. Answer 1i). UNSTRUCTURED LANGUAGE DEVELOPER DATE Assembly Language 1949 FORTRAN John Backus 1957 COBOL CODASYL, ANSI, ISO 1959 JOSS Cliff Shaw, RAND 1963 BASIC John G. Kemeny, Thomas E. Kurtz 1964 TELCOMP BBN 1965 MUMPS Neil Pappalardo 1966 FOCAL Richard Merrill, DEC 1968 STRUCTURED LANGUAGE DEVELOPER DATE ALGOL 58 Friedrich L. Bauer, and co. 1958 ALGOL 60 Backus, Bauer and co. 1960 ABC CWI 1980 Ada United States Department of Defence 1980 Accent R NIS 1980 Action! Optimized Systems Software 1983 Alef Phil Winterbottom 1992 DASL Sun Micro-systems Laboratories 1999-2003 MODULAR LANGUAGE DEVELOPER DATE ALGOL W Niklaus Wirth, Tony Hoare 1966 APL Larry Breed, Dick Lathwell and co. 1966 ALGOL 68 A. Van Wijngaarden and co. 1968 AMOS BASIC FranÇois Lionet anConstantin Stiropoulos 1990 Alice ML Saarland University 2000 Agda Ulf Norell;Catarina coquand(1.0) 2007 Arc Paul Graham, Robert Morris and co. 2008 Bosque Mark Marron 2019 OBJECT-ORIENTED LANGUAGE DEVELOPER DATE C* Thinking Machine 1987 Actor Charles Duff 1988 Aldor Thomas J. Watson Research Center 1990 Amiga E Wouter van Oortmerssen 1993 Action Script Macromedia 1998 BeanShell JCP 1999 AngelScript Andreas Jönsson 2003 Boo Rodrigo B. -
Chapter 2 Visual Foxpro for Client/Server Development
Chapter 2: Visual FoxPro for Client/Server Development 19 Chapter 2 Visual FoxPro for Client/Server Development After reading Chapter 1, you should have a good understanding of what makes client/server databases different and why you might want to use them. But the $64,000 question is: Why would you use Visual FoxPro to develop your client/server applications? This chapter will answer that question for you, the developer, and provide answers that you can give if your clients ask this question. More than half of all software developers today use a certain variation of Beginner’s All- purpose Symbolic Instruction Code (BASIC), according to Microsoft. Why? Partly because of familiarity, partly because of its versatility, and also partly because popularity begets popularity. But you might recall your parents telling you, “Just because everyone else does it doesn’t mean you should do it, too.” This chapter discusses six features that make Visual FoxPro the best client/server rapid application development tool available today: object- oriented programming, COM support, built-in client/server support, built-in local data engine, support for other data-access technologies such as ADO, and Rapid Application Development (RAD). Many of these features could be, or are, the topics of one or more books of their own. While each feature is discussed separately, keep in mind that it is the combination of these features that makes VFP such a great client/server development tool. Not many development tools can offer the combination of features found in FoxPro. Visual Basic, for example, offers great COM support and support for ADO and is an excellent RAD environment, but it isn’t object-oriented, while C++ is a great object-oriented programming language but lacks built-in client/server or local database support and has never been accused of being good for RAD. -
An Introduction to Ruby and Rails
An Introduction to Ruby and Rails This paper was originally presented at the Southwest Fox conference in Gilbert, Arizona in October, 2010. http://www.swfox.net An Introduction to Ruby and Rails Rick Borup Information Technology Associates 701 Devonshire Dr, Suite 127 Champaign, IL 61820 Voice: (217) 359-0918 Fax: (217) 398-0413 Email: [email protected] Ruby is a powerful, object-oriented, open-source language with a clean and easily understandable syntax. Originally released to the public in 1995, wide-spread interest in the Ruby language really began to take off in 2003 with the release of the Ruby on Rails® framework. Also known as "RoR" or simply "Rails", Ruby on Rails is an open-source Web framework that makes it possible to quickly and easily create data-based Web applications. Together, the Ruby language and the Ruby on Rails framework can become an exciting and powerful addition to any developer's toolbox. This session introduces both the Ruby language and the Rails framework, focusing on their installation and use on a Windows® platform with a perspective geared toward experienced Visual FoxPro® developers. © 2010 Rick Borup Page 1 of 64 An Introduction to Ruby and Rails Table of Contents Introduction............................................................................................................................................................ 4 Installing Ruby and Rails on Windows......................................................................................................... 4 Installing Instant Rails -
Visual Foxpro
RANDOLPH TOWNSHIP SCHOOL DISTRICT va_chkr3.101405 11/10/2011 Check Register By Check Number Posted Checks : Selected Cycle : November Invoice Account Vendor Ba- Check Check Check Check Check # PO # Number Number No./ Name tch Amount Description Date Type POSTED CHECKS 71124 1103732 1282 11-000-263-420-18-7208 9234/D & L PAVING CONTRACTORS, 0 10,203.50REPL CHK# 70851 11/07/2011 C INC. 1201581 1281 12-000-400-450-18-9102 9234/D & L PAVING CONTRACTORS, 0 5,006.25REPL CHK# 70851 11/07/2011 C INC. 1200725 1284 12-000-400-450-18-9102 9234/D & L PAVING CONTRACTORS, 0 306,340.00REPL CHK# 70851 11/07/2011 C INC. 1201582 1291 12-000-400-450-18-9102 9234/D & L PAVING CONTRACTORS, 0 12,090.00REPL CHK# 70851 11/07/2011 C INC. Total For Check Number 71124 $333,639.75 71125 1201872A 11-000-291-280-40-8210 9805/ALTIS; MEGHAN 0 475.00Adolescent Brain Spring 10-11 11/08/2011 C 1201873A 11-000-291-280-40-8210 9805/ALTIS; MEGHAN 0 475.00Promote Stud Learnin Spr 10-11 11/08/2011 C Total For Check Number 71125 $950.00 71126 1202097 1078119 60-000-310-400-60-0001 9525/METZ & ASSOCIATES INC.59 3,044.15EQUIPMENT REPAIR-FSMC 11/10/2011 C 1202097 1078119 60-000-310-500-60-1000 9525/METZ & ASSOCIATES INC.59 8,131.27SALARIES-FSMC MGMT 11/10/2011 C 1202097 1078119 60-000-310-500-60-1001 9525/METZ & ASSOCIATES INC.59 2,683.32TAX & FRINGE-MGMT-FSMC 11/10/2011 C 1202097 1078119 60-000-310-500-60-2000 9525/METZ & ASSOCIATES INC.59 6,582.08SALARIES-STAFF-FSMC 11/10/2011 C 1202097 1078119 60-000-310-500-60-2001 9525/METZ & ASSOCIATES INC.59 2,172.09TAX & FRINGE-STAFF-FSMC -
Visual Foxpro
Anticipated Recipient : PASSAIC VALLEY SC Project No. : 340430-02 Eligible Project Cost : $ 100,557,651 FY09 Rank : 1 County : ESSEX Total State Amount : $ 100,557,651 I. LOCAL ENVIRONMENTAL ENHANCEMENT Possible Points Points Scored PLANNING ACTIVITIES 0-200 0 II. PROJECT DISCHARGE TYPE Possible Points Points Scored CSO/SSO 600 600 Treatment Plant Improvements 500 0 SS Rehabilitation 450 0 Sludge Disposal/Treatment 350 0 WW Reuse 300 0 Septic Repair 275 0 New Systems 250 0 Stormwater/NPS Management 225 0 Landfill Closure, Land Acq, Well Sealing 150 0 Landfill Constr, Site Remediation 75 0 Conduit Projects 50 0 Subtotal 600 III. WATER USE/WATER QUALITY POINTS A. Water Use Possible Points Points Scored Potable Water Supply 200 0 Shellfish 125 125 Recreation(Primary Contact) 125 0 Freshwater Fisheries(Trout/NonTrout) 75/25 0 Public Nuisance(On Site Systems Only) 50 0 Agriculture Water Use 25 0 Industrial Water Use 25 25 Subtotal 150 B. Existing Water Quality Meets Marginally Does Not Parameter Standards Meets Standards Meet Standards Points Scored Dissolved Oxygen 0 50 100 100 Fecal Coliform 0 50 100 100 Nutrients 0 25 50 0 Toxics 0 25 50 50 Subtotal 250 State Planning Commission 50.00000 Population 1.35000 BDA Points 0 Transit Villages 0 TOTAL POINTS 1051.35000 Project Name, Number Priority List Rank PASSAIC VALLEY SC 1 340430-02 CSO ABATEMENT County ESSEX Existing Population 1,350,000 Service Area East Newark, Harrison, Kearny, Newark, Paterson Need for Project Wastewater generated in the PVSC service area is treated at its secondary treatment facility located in the City of Newark. -
Energy Systems Laboratory
ESL-TR-09-02-02 ANALYSIS OF EMISSIONS CALCULATORS FOR THE NATIONAL CENTER OF EXCELLENCE ON DISPLACED EMISSION REDUCTIONS (CEDER) ANNUAL REPORT 2008 Annual Report to the United States Environmental Protection Agency Bahman Yazdani, P.E.; Charles Culp, Ph.D., P.E.; Jeff Haberl, Ph.D., P.E.; Juan-Carlos Baltazar, Ph.D.; Sung Lok Do February 2009 modified in March 2009 ENERGY SYSTEMS LABORATORY Texas Engineering Experiment Station Texas A&M University System Page 2 Disclaimer This report is provided by the Texas Engineering Experiment Station (TEES) as required under Section 388.003 (e) of the Texas Health and Safety Code and is distributed for purposes of public information. The information provided in this report is intended to be the best available information at the time of publication. TEES makes no claim or warranty, express or implied, that the report or data herein is necessarily error-free. Reference herein to any specific commercial product, process, or service by trade name, trademark, manufacturer, or otherwise, does not constitute or imply its endorsement, recommendation, or favoring by the Energy Systems Laboratory or any of its employees. The views and opinions of authors expressed herein do not necessarily state or reflect those of the Texas Engineering Experiment Station or the Energy Systems Laboratory. March 2009 Energy Systems Laboratory, Texas A&M University System Page 3 ANNUAL REPORT ANALYSIS OF EMISSIONS CALCULATORS FOR NATIONAL CENTER OF EXCELLENCE ON DISPALCED EMISSION REDUCTIONS (CEDER) 1. EXECUTIVE SUMMARY In August 2004, the USEPA issued guidance on quantifying the air emission benefits from electric sector energy efficiency and renewable energy. -
Warum Dynamische Sprachen ?? Wo Würde Ich Persönlich Sie Verwenden, Wo Nicht ;-)
{ Helden brauchen weniger } Frank Fischer Manager Evangelism and Marketing http://blogs.msdn.com/frankfi Microsoft Deutschland GmbH Jim Hugunin 100K 80K 60K 101K 90K 40K 80K 46K 50K 36K20K 0K Python Python Python IronPython IronPython IronPython 2.1 2.3 2.5 0.1 1.0 2.0alpha1 Jim Hugunin Principal Architect Microsoft Corp. Eine Geschichte zum Beginn… (hatten wir gerade) Was sind „dynamische Sprachen“ ?? Der Versuch einer Definition Warum dynamische Sprachen ?? Wo würde ich persönlich sie verwenden, wo nicht ;-) Was hat Microsoft damit zu tun?? Eine Geschichte zum Beginn… (hatten wir gerade) Was sind „dynamische Sprachen“ ?? Der Versuch einer Definition Warum dynamische Sprachen ?? Wo würde ich persönlich sie verwenden, wo nicht ;-) Was hat Microsoft damit zu tun?? Dynamic programming language is a term used broadly in computer science to describe a class of high level programming languages that execute at runtime many common behaviors that other languages might perform during compilation, if at all. These behaviors could include extension of the program, by adding new code, by extending objects and definitions, or by modifying the type system, all during program execution. These behaviors can be emulated in nearly any language of sufficient complexity, but dynamic languages provide direct tools to make use of them. Dynamic languages and dynamic typing are not identical concepts, and a dynamic language need not be dynamically typed, though many dynamic languages are dynamically typed. http://en.wikipedia.org/wiki/Dynamic_programming_language -
Cxp-Intro-Print-En.Pdf
Overview . Prologue . Use Cases . Introduction . Features/Samples . Roadmap . Call for Action (c) Alaska Software Inc. Preface:Introduction One Tool Visual FoxPro One Vendor Language Inheritance Different Devices RIA UI War is and Over Form Factors (c) Alaska Software Inc. Preface:Introduction One Tool Visual FoxPro One Vendor Language Inheritance Different Devices RIA UI War is and Over Form Factors (c) Alaska Software Inc. Context:<CXP/> Context Xbase++ 2.0 . Scheduled Release: Q1/Q2Q12011 2012 (Arctica) . Unified-SQL and Universal-SQL CTP . PostgreSQL Isam Access . <CXP/> for Web and Mobile Application Development . VFP Language Extensions Visual Xbase++ 3.0 . Planned Preview: Q4 2012 (PolarFox) . Visual Design Tools . VFP Modernization (Runtime and Transpiler) (c) Alaska Software Inc. Use Cases Mobile Application Dynamic Web Page Web Application (like uservoice.com): • 6 <CXP/> files • One <CXP/> page per view • 8 views (<CXP/>), 2 controllers (prg), 7 models • Each around 40 lines of code • Contains HTML mixed with code (prg) • <1000 lines of code (Add here your or your customer‘s Forms, Image Creation demands…) (c) Alaska Software Inc. Intro (What‘s the idea behind it!) <CXP/>: – can create dynamic and interactive web pages/content – is a powerful alternative to ASP.NET and PHP – „speaks“ the language Visual FoxPro, FoxPro and Xbase++ developers know – executes native code, whereas ASP/PHP is interpreted Hello.cxp: Intermediate Code: (c) Alaska Software Inc. Intro (c) Alaska Software Inc. Features (1/2) Easy to Use • Edit and run • Compiler error messages with great detail Very Fast • Executes compiled native code versus interpreted code • Uses random distribution on multi-core servers • Dynamic I/O chunking for large data upload/download • Developer can use threads to offload work or even defer results Secure • Code injection doesn‘t work as it executes compiled code • AES encryption comes with Xbase++ • Ready-to-use authentication and user account management (RBAC) included (c) Alaska Software Inc.