CSCI 130 Principles of Programming I
Total Page:16
File Type:pdf, Size:1020Kb
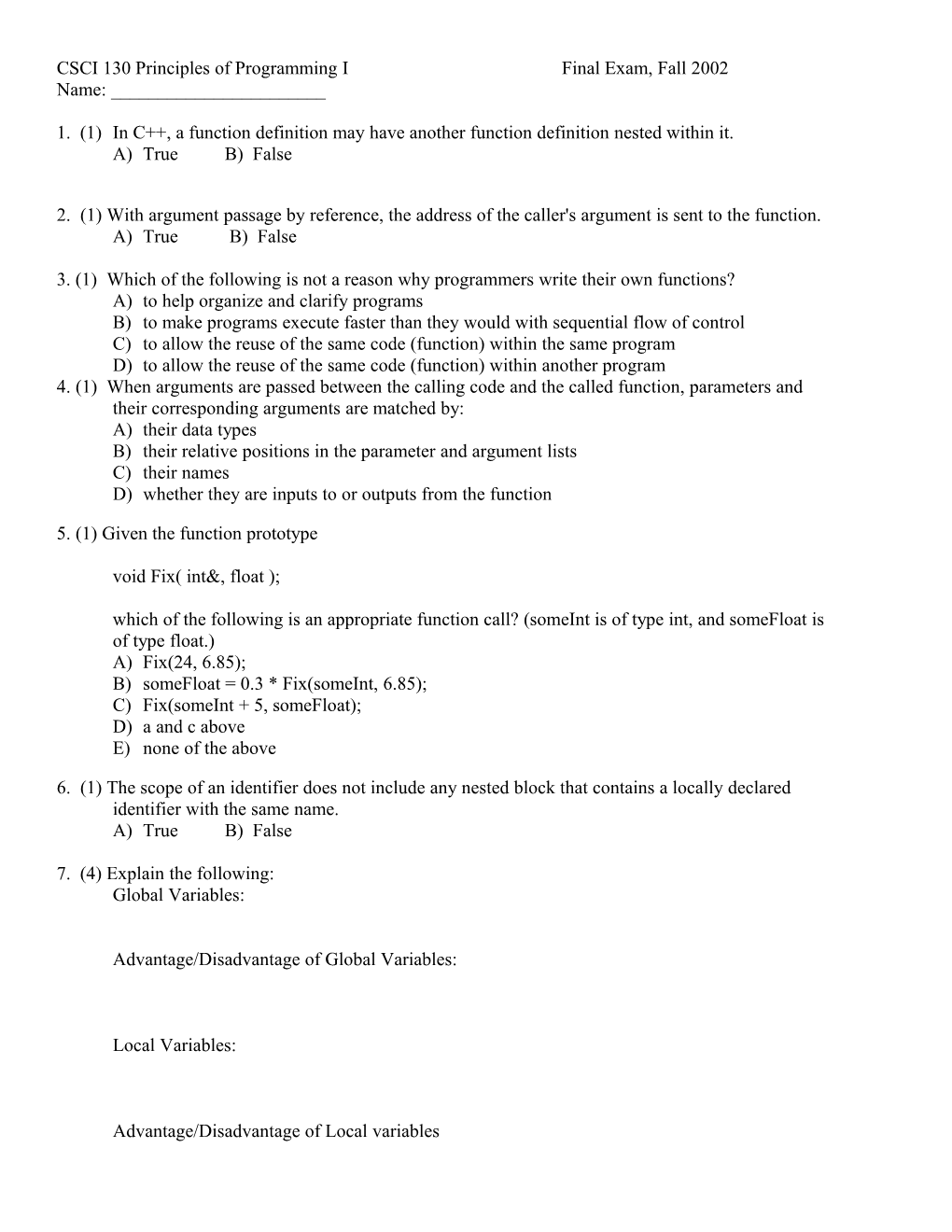
CSCI 130 Principles of Programming I Final Exam, Fall 2002 Name: ______
1. (1) In C++, a function definition may have another function definition nested within it. A) True B) False
2. (1) With argument passage by reference, the address of the caller's argument is sent to the function. A) True B) False
3. (1) Which of the following is not a reason why programmers write their own functions? A) to help organize and clarify programs B) to make programs execute faster than they would with sequential flow of control C) to allow the reuse of the same code (function) within the same program D) to allow the reuse of the same code (function) within another program 4. (1) When arguments are passed between the calling code and the called function, parameters and their corresponding arguments are matched by: A) their data types B) their relative positions in the parameter and argument lists C) their names D) whether they are inputs to or outputs from the function
5. (1) Given the function prototype
void Fix( int&, float );
which of the following is an appropriate function call? (someInt is of type int, and someFloat is of type float.) A) Fix(24, 6.85); B) someFloat = 0.3 * Fix(someInt, 6.85); C) Fix(someInt + 5, someFloat); D) a and c above E) none of the above
6. (1) The scope of an identifier does not include any nested block that contains a locally declared identifier with the same name. A) True B) False
7. (4) Explain the following: Global Variables:
Advantage/Disadvantage of Global Variables:
Local Variables:
Advantage/Disadvantage of Local variables 8. (1) The body of a Do-While loop will always execute at least once, whereas the body of a For loop may never execute. A) True B) False
9. (1) What is the output of the following code fragment? (beta is of type int.)
beta = 5; do { switch (beta) { case 1 : cout << 'R'; break; case 2 : case 4 : cout << 'O'; break; case 5 : cout << 'L'; } beta--; } while (beta > 1); cout << 'X'; A) X B) ROOLX C) LOOX D) LOORX E) ROOX
10. (1) What is the output of the following code fragment? (loopCount is of type int.)
for (loopCount = 1; loopCount > 3; loopCount++) cout << loopCount << ' '; cout << "Done" << endl; A) Done B) 1 Done C) 1 2 Done D) 1 2 3 Done E) 1 2 3 4 Done
11. (1) What is the output of the following code fragment? (All variables are of type int.)
n = 2; for (loopCount = 1; loopCount <= 3; loopCount++) while (n <= 4) n = 2 * n; cout << n << endl; A) 4 B) 8 C) 16 D) 32 E) 64 12. (1) Alphanumeric characters are stored in the computer as integers. A) True B) False
13. (1) Because of representational error, two floating-point numbers should not be compared for exact equality. A) True B) False
14. (1) Which of the following stores into min the smaller of alpha and beta? A) if (alpha < beta) min = alpha; else min = beta; B) min = (alpha < beta) ? beta : alpha; C) min = (alpha < beta) ? alpha : beta; D) a and b above E) a and c above
15. (1) Given the declarations
enum Days {YESTERDAY, TODAY, TOMORROW}; days day = TODAY;
what is the value of the expression int(day) ? A) TODAY D) 2 B) 1 E) none of the above C) TOMORROW
16. (1) Assume you have the following declarations:
enum StudentType {MARY, JANE, BILL}; StudentType oneStudent;
and that oneStudent has been assigned some value. Which of the following outputs Jane's name if oneStudent contains the value JANE? A) if (oneStudent == JANE) cout << oneStudent; B) if (oneStudent == "JANE") cout << StudentType(oneStudent); C) if (oneStudent == JANE) cout << JANE; D) if (oneStudent == "JANE") cout << "JANE"; E) if (oneStudent == JANE) cout << "JANE";
17. (1) You have created some useful type declarations and constant declarations and have stored them into a file named mystuff.h. Which of the following would you use to insert the contents of this file into a program? A) #include
19. (7) Write a program that requests a number and displays a hollow rectangle of asterisks with each outer row and column having that many stars.
For example, the user types in 5, your program prints out:
***** Remember this is a sample, if your program only prints out a box with sides of 5 stars * * you won’t receive any credit. * * * * ***** Answers:
1. B 2. A 3. B 4. B 5. E 6. False 8. A 9. C 10. A 11. B 12. A 13. A 14. E 15. B 16. E 17. B