Chapter 9 Procedures and Arrays
Total Page:16
File Type:pdf, Size:1020Kb
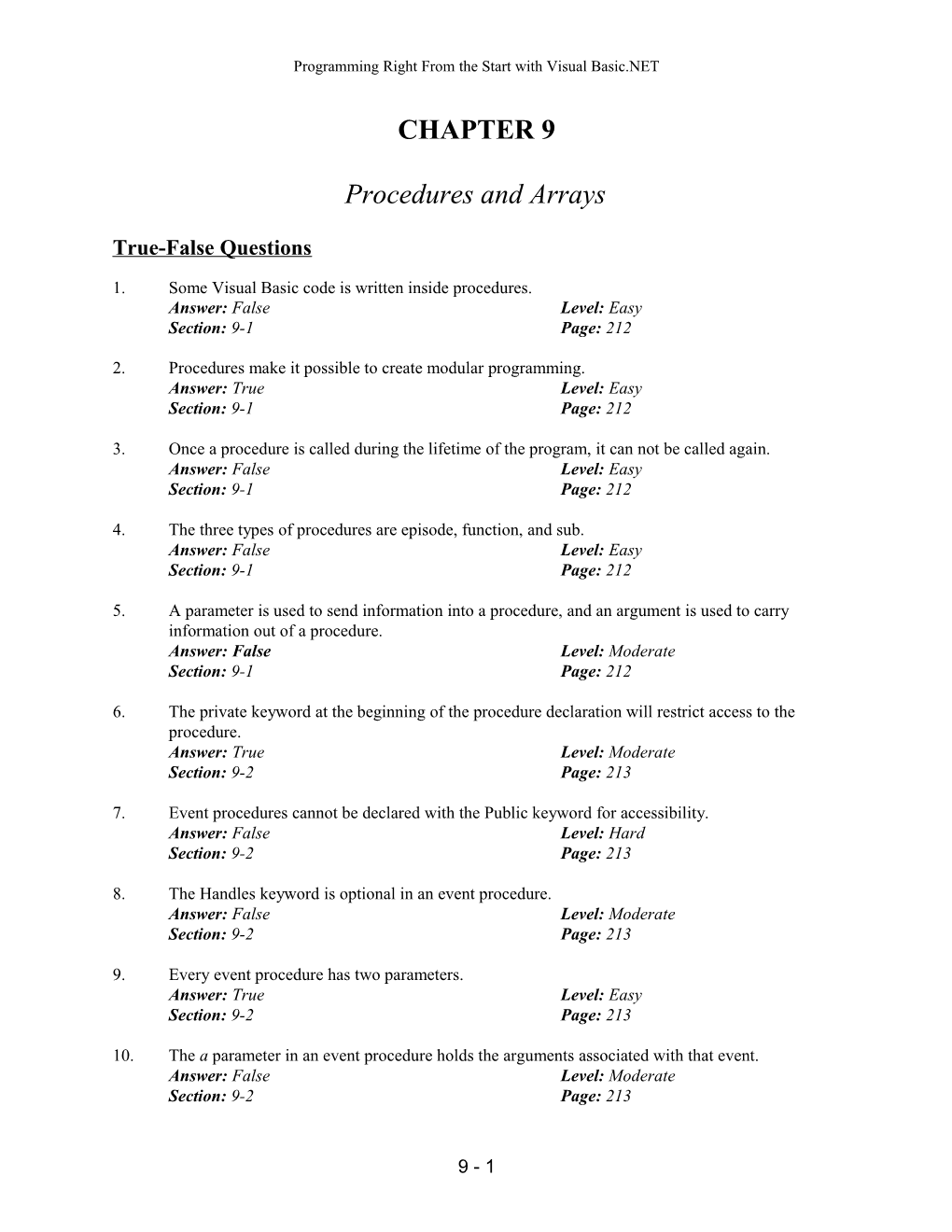
Programming Right From the Start with Visual Basic.NET
CHAPTER 9
Procedures and Arrays
True-False Questions
1. Some Visual Basic code is written inside procedures. Answer: False Level: Easy Section: 9-1 Page: 212
2. Procedures make it possible to create modular programming. Answer: True Level: Easy Section: 9-1 Page: 212
3. Once a procedure is called during the lifetime of the program, it can not be called again. Answer: False Level: Easy Section: 9-1 Page: 212
4. The three types of procedures are episode, function, and sub. Answer: False Level: Easy Section: 9-1 Page: 212
5. A parameter is used to send information into a procedure, and an argument is used to carry information out of a procedure. Answer: False Level: Moderate Section: 9-1 Page: 212
6. The private keyword at the beginning of the procedure declaration will restrict access to the procedure. Answer: True Level: Moderate Section: 9-2 Page: 213
7. Event procedures cannot be declared with the Public keyword for accessibility. Answer: False Level: Hard Section: 9-2 Page: 213
8. The Handles keyword is optional in an event procedure. Answer: False Level: Moderate Section: 9-2 Page: 213
9. Every event procedure has two parameters. Answer: True Level: Easy Section: 9-2 Page: 213
10. The a parameter in an event procedure holds the arguments associated with that event. Answer: False Level: Moderate Section: 9-2 Page: 213
9 - 1 Chapter 9 – Procedures and Arrays
11. The sender parameter in an event procedure holds a reference to the object that raised the event. Answer: True Level: Moderate Section: 9-2 Page: 213
12. The default is for parameters to be sent as ByRef. Answer: False Level: Moderate Section: 9-2 Page: 213
13. To create an event procedure you must type the procedure declaration in the code editor. Answer: False Level: Easy Section: 9-2 Page: 213
14. Double clicking on a Button control in the Designer window will automatically create a click event procedure stub in the Code Editor window. Answer: True Level: Moderate Section: 9-2 Page: 213
15. Some sub procedures cannot be called multiple times. Answer: False Level: Easy Section: 9-3 Page: 213
16. One important purpose of a sub procedure is to make the code easier to understand. Answer: True Level: Easy Section: 9-3 Page: 213
17. When calling a sub procedure, parentheses are optional if there are no arguments. Answer: False Level: Easy Section: 9-3 Page: 215
18. The Handles keyword is optional in a sub procedure. Answer: False Level: Hard Section: 9-3 Page: 215
19. Parameters are optional in a sub procedure. Answer: True Level: Easy Section: 9-3 Page: 215
20. A function must have an assigned data type. Answer: True Level: Easy Section: 9-4 Page: 216
21. A function procedure must have one or more parameters. Answer: False Level: Easy Section: 9-4 Page: 216
9 - 2 Chapter 9 – Procedures and Arrays
22. A function procedure must have a Return statement. Answer: False Level: Hard Section: 9-4 Page: 216
23. Only one Return statement is allowed in a function procedure. Answer: False Level: Moderate Section: 9-4 Page: 216
24. A function can act like an expression in an assignment statement. Answer: True Level: Moderate Section: 9-4 Page: 216
25. Modular programming aims to decrease coupling and increase cohesion. Answer: True Level: Hard Section: 9-4 Page: 217
26. A function procedure is identical to a sub procedure. Answer: False Level: Easy Section: 9-4 Page: 217
27. Local variables are declared in the General Declaration Section of the program. Answer: False Level: Easy Section: 9-5 Page: 218
28. Module variables can be accessed from any procedure in a module. Answer: True Level: Easy Section: 9-5 Page: 218
29. The scope of a variable means how long the variable lasts in memory. Answer: False Level: Moderate Section: 9-5 Page: 218
30. It is best to declare all variables as module because then any procedure can access any variable. Answer: False Level: Moderate Section: 9-5 Page: 218
31. A local variable is recreated anew every time its procedure is activated. Answer: True Level: Moderate Section: 9-5 Page: 218
32. A module variable will not work unless its name starts with the letter m as a prefix. Answer: False Level: Moderate Section: 9-5 Page: 218
33. The index for the first element of an array is either 0 or 1. Answer: False Level: Easy Section: 9-6 Page: 219
9 - 3 Chapter 9 – Procedures and Arrays
34. An array consists of multiple elements. Answer: True Level: Easy Section: 9-6 Page: 219
35. The upper bound of an array equals the length of the array. Answer: False Level: Easy Section: 9-6 Page: 219
36. When declaring an array, the upper bound of an array must be included. Answer: False Level: Moderate Section: 9-6 Page: 220
37. When an array is made larger, the Preserve statement will prevent the values in the data elements from being lost. Answer: True Level: Easy Section: 9-6 Page: 222
38. A structure can hold multiple values of a single datatype. Answer: False Level: Easy Section: 9-7 Page: 226
39. A member of a structure is the same as a variable declared in a structure. Answer: True Level: Moderate Section: 9-7 Page: 226
40. A period is used to reference the name of a variable in a structure. Answer: True Level: Easy Section: 9-7 Page: 226
41. An array cannot hold a structure datatype. Answer: False Level: Easy Section: 9-7 Page: 226
42. An array can hold controls as their datatype. Answer: True Level: Easy Section: 9-8 Page: 228
43. A special DimControl statement is needed to create an array of controls. Answer: False Level: Easy Section: 9-8 Page: 228
44. The only way to create an array of controls is to assign existing controls to the array during the Form Load event. Answer: False Level: Moderate Section: 9-8 Page: 228
45. The Tag property is only associated with label and textbox controls. Answer: False Level: Moderate Section: 9-8 Page: 228
9 - 4 Chapter 9 – Procedures and Arrays
46. The Tag property is used to hold any kind of information needed in the program. Answer: True Level: Moderate Section: 9-8 Page: 228
47. Pressing the Shift, Control, or Alt key by itself will not generate a KeyPress event. Answer: True Level: Moderate Section: 9-9 Page: 233
48. Arrow keys do not generate a KeyPress event, but they do generate a KeyDown event. Answer: False Level: Moderate Section: 9-9 Page: 233
49. The KeyChar property in a KeyPress event can distinguish between an upper and lower case letter. Answer: True Level: Easy Section: 9-9 Page: 233
50. If the Handled Property in a KeyPress event is set to True, the letter typed will not appear in the textbox. Answer: True Level: Easy Section: 9-9 Page: 233
Multiple Choice Questions
51. Which is a type of procedure found in VB.Net? a.) Event b.) Function c.) Sub d.) Both a and b. e.) All of the above.
Answer: e Level: Easy Section: 9-1 Page: 212
52. The methodology where code is broken into small, logical procedures is called: a.) event-driven programming. b.) functional programming. c.) granular programming. d.) modular programming. e.) procedural programming.
Answer: d Level: Hard Section: 9-1 Page: 212
9 - 5 Chapter 9 – Procedures and Arrays
53. When using a procedure the calling code sends data via the: a.) actual argument to the formal parameter of the procedure. b.) formal argument to the actual parameter of the procedure. c.) actual parameter to the formal argument of the procedure. d.) formal parameter to the actual argument of the procedure. e.) All of the above.
Answer: a Level: Hard Section: 9-1 Page: 212
54. From how many places in the code can a procedure be called? a.) 0 b.) 1 c.) 2 d.) 3 e.) As many times as needed.
Answer: e Level: Easy Section: 9-1 Page: 212
55. Which parameter is found in an event procedure? a.) e b.) Sender c.) Receiver d.) Both a and b. e.) All of the above.
Answer: e Level: Moderate Section: 9-2 Page: 213
56. Which is an optional element of an event procedure? a.) End Sub b.) Handles c.) Object_Event d.) Statements e.) Sub
Answer: d Level: Moderate Section: 9-2 Page: 213
57. What happens when a parameter in a procedure is declared ByVal? a.) Only arguments of numeric data types are allowed. b.) A reference to the argument is sent to the procedure. c.) A copy of the argument is sent to the procedure. d.) Both a and b. e.) All of the above.
Answer: c Level: Easy Section: 9-2 Page: 213
9 - 6 Chapter 9 – Procedures and Arrays
58. Which is a valid way to write the procedure stub for an object’s default event? a.) Use the Class and Method combo boxes in the Code Editor window. b.) Double click on the object in the Form Designer window. c.) Type the procedure declaration in the Code Editor window. d.) Both a and b. e.) All of the above.
Answer: e Level: Moderate Section: 9-2 Page: 214
59. A sub procedure is valuable because it: a.) makes code easier to maintain. b.) splits the logic to solve a problem into small, manageable units. c.) limits the number of times the code can be accessed. d.) Both a and b. e.) All of the above.
Answer: d Level: Moderate Section: 9-3 Page: 214
60. Which is not an optional element of a sub procedure declaration? a.) Parameters b.) Public c.) Private d.) Statements e.) Sub
Answer: e Level: Moderate Section: 9-3 Page: 215
61. Which is a valid way to write a sub procedure declaration? a.) Use the Class and Method combo boxes in the Code Editor window. b.) Double click on the object in the Form Designer window. c.) Type the procedure declaration in the Code Editor window. d.) Both a and b. e.) All of the above.
Answer: c Level: Moderate Section: 9-3 Page: 215
9 - 7 Chapter 9 – Procedures and Arrays
62. Which statement will send the value generated by a function procedure, called CalculateTax, back to the calling code? a.) Return Sales*0.08 b.) CalculateTax = Sales*0.08 c.) Return CalculateTax (Sales*0.08) d.) Both a and b. e.) All of the above.
Answer: d Level: Moderate Section: 9-4 Page: 217
63. Which part of a function procedure declaration statement is optional? a.) Datatype b.) Function c.) Parameters d.) Private e.) ProcedureName
Answer: c Level: Hard Section: 9-4 Page: 217
64. How many return statements are allowed in a Function Procedure? a.) 0 b.) 1 c.) 2 d.) 3 e.) There is no limit.
Answer: e Level: Easy Section: 9-4 Page: 217
65. Why should a variable not be declared as a module variable? a.) It prevents a procedure from being self contained. b.) It makes it easier to document the code. c.) Local variable names can be reused in other procedures. d.) Both a and b. e.) All of the above.
Answer: e Level: Moderate Section: 9-5 Page: 219
66. Which variable name uses a standard naming convention for module variables? a.) mWeight b.) mdWeight c.) modWeight d.) moduleWeight e.) module_Weight
Answer: a Level: Easy Section: 9-5 Page: 218
9 - 8 Chapter 9 – Procedures and Arrays
67. The scope of a variable refers to: a.) the length of the variable. b.) the name of the variable. c.) the accessibility of the variable. d.) the datatype of the variable. e.) the lifetime of the variable.
Answer: c Level: Moderate Section: 9-5 Page: 218
68. What is the value of the index for the first element in a VB.NET array? a.) 0 b.) 1 c.) 2 d.) 3 e.) Depends on what the assigned value is.
Answer: a Level: Moderate Section: 9-6 Page: 219
69. Which method will return the number of elements in an array? a.) Dimension b.) Length c.) Number d.) Size e.) UpperBound
Answer: b Level: Moderate Section: 9-6 Page: 219
70. In the statement, Dim Days(7) as String, what part of the array does the number 7 refer to? a.) Array name b.) Datatype c.) Lowerbound d.) Upperbound e.) Size
Answer: d Level: Moderate Section: 9-6 Page: 220
71. What is required to reference an element in an array? a.) Array name b.) Index value of the element c.) Element value d.) Both a and b. e.) All of the above.
Answer: d Level: Moderate Section: 9-6 Page: 220
9 - 9 Chapter 9 – Procedures and Arrays
72. Which method will arrange the elements of an array in alphabetical order? a.) Arrange b.) Assemble c.) Order d.) Rank e.) Sort
Answer: e Level: Easy Section: 9-6 Page: 222
73. The number of variables allowed in a structured is: a.) 0 b.) 1 c.) 2 d.) 3 e.) Any number of variables can be declared in an array.
Answer: e Level: Easy Section: 9-7 Page: 226
74. The variable inside a structure is called a(n): a.) associate. b.) constituent. c.) element. d.) member. e.) part.
Answer: d Level: Moderate Section: 9-7 Page: 226
75. Which datatype can an array not hold? a.) TextBoxes b.) Labels c.) Structures d.) Controls e.) An array can hold all of the above.
Answer: e Level: Easy Section: 9-8 Page: 228
76. An array of controls can be populated by: a.) assigning existing controls to the array. b.) creating controls and assigning them to the array. c.) borrowing controls that will automatically assign them to the array. d.) Both a and b. e.) All of the above.
Answer: d Level: Moderate Section: 9-8 Page: 228
9 - 10 Chapter 9 – Procedures and Arrays
77. The Tag property can: a.) only hold string values. b.) only hold integer values. c.) only hold Boolean values. d.) only hold controls. e.) hold any data defined by the programmer.
Answer: e Level: Hard Section: 9-8 Page: 228
78. The KeyPress event will capture pressing the key: a.) A. b.) shift. c.) control. d.) Both a and b. e.) All of the above.
Answer: a Level: Easy Section: 9-9 Page: 233
79. Which argument in the KeyPress parameter list contains the Handled property? a.) Sender b.) e c.) Object d.) KeyPressEventArgs e.) None of the above.
Answer: b Level: Hard Section: 9-9 Page: 233
Fill in the Blank Questions
80. The advantage of ___modular___ programming is that it is easier to maintain. Level: Moderate Section: 9-1 Page: 212
81. The calling code uses a(n) ___argument___ to send information to a procedure. Level: Moderate Section: 9-1 Page: 212
82. A procedure has ___parameters___ that act like variables to hold data from the calling code. Level: Moderate Section: 9-1 Page: 212
83. The three types of procedures in VB.Net are ___events___, ___subs___, and ___functions___. Level: Easy Section: 9-1 Page: 212
9 - 11 Chapter 9 – Procedures and Arrays
84. Another term for an event procedure is ___event handler___. Level: Easy Section: 9-2 Page: 213
85. A procedure stub must be declared as having either ___public___ or ___private___ accessibility. Level: Moderate Section: 9-2 Page: 213
86. The Handles keyword is required in the declaration of a(n) ___event___ procedure. Level: Easy Section: 9-2 Page: 213
87. The ___sender___ parameter of an event procedure represents the object that generated the event. Level: Moderate Section: 9-2 Page: 213
88. The ___e___ parameter of an event procedure represents the parameters unique to that type of event. Level: Moderate Section: 9-2 Page: 213
89. The ___ByVal___ keyword will cause a copy of the argument to be sent to a procedure. Level: Easy Section: 9-2 Page: 213
90. The ___ByRef___ keyword will cause a pointer to the argument to be sent to a procedure. Level: Easy Section: 9-2 Page: 213
91. When a parameter is declared as ___ByRef ___ the argument sent to the procedure can be changed by the code inside the procedure. Level: Moderate Section: 9-2 Page: 213
92. The ___click___ event procedure stub is created when a button on a form in the Designer window is double clicked. Level: Moderate Section: 9-2 Page: 214
93. The ___End Sub___ statement will cause the control of the program to exit the sub procedure and return to the calling procedure. Level: Moderate Section: 9-3 Page: 215
94. One advantage of a sub procedure is that it can be called ___multiple___ times. Level: Moderate Section: 9-3 Page: 215
9 - 12 Chapter 9 – Procedures and Arrays
95. Another name for a sub procedure is ___subroutine___. Level: Easy Section: 9-3 Page: 214
96. A function procedure is also called a ___function___. Level: Easy Section: 9-4 Page: 216
97. The ___return___ statement sends the function’s value back to the code that called the function. Level: Moderate Section: 9-4 Page: 216
98. The amount of interdependency between procedures is called ___coupling___. Level: Hard Section: 9-4 Page: 217
99. The amount of uniformity within a procedure is called ___cohesion___. Level: Hard Section: 9-4 Page: 217
100. The difference between a sub and function procedure is that a function will ___return a value___. Level: Easy Section: 9-4 Page: 216
101. A ___local___ variable is declared in a procedure. Level: Easy Section: 9-5 Page: 218
102. A ___module___ variable is declared in the General Declaration Section. Level: Easy Section: 9-5 Page: 218
103. The ___scope___ of a variable indicates which parts of the code can reference the variable. Level: Moderate Section: 9-5 Page: 218
104. A ___local___ variable is erased from memory each time an End Sub statement is run. Level: Moderate Section: 9-5 Page: 218
105. The values of an array are stored in its ___elements___. Level: Easy Section: 9-6 Page: 219
106. Another name for an index is ___subscript___. Level: Moderate Section: 9-6 Page: 219
9 - 13 Chapter 9 – Procedures and Arrays
107. The index of the last element in an array is the ___upper bound___. Level: Moderate Section: 9-6 Page: 219
108. The statement that changes the size of an array is ___ReDim___. Level: Easy Section: 9-6 Page: 222
109. An array of ___structures___ can hold different data types. Level: Moderate Section: 9-7 Page: 226
110. Another name for the variables in a structure is a(n) ___member___. Level: Moderate Section: 9-7 Page: 226
111. An array that contains checkboxes, groupboxes, and labels is called a(n) ___control___ array. Level: Easy Section: 9-8 Page: 228
112. The ___Tag___ property of a control can contain integers, strings, or Boolean values. Level: Moderate Section: 9-8 Page: 228
113. The ___KeyDown___ Event can capture when the user presses the alt key in a textbox. Level: Moderate Section: 9-9 Page: 233
114. The ___KeyPress___ Event cannot capture when the user presses the alt key in a textbox. Level: Moderate Section: 9-9 Page: 233
115. The ____e____ argument in the KeyPress event contains the KeyChar property. Level: Moderate Section: 9-9 Page: 233
116. The ___Handled___ property, when set to True, will stop further processing of a key press. Level: Moderate Section: 9-9 Page: 233
9 - 14 Chapter 9 – Procedures and Arrays
Essay Questions
117. Describe three ways to create the declaration for an Event Procedure? Which is the best method and why?
In the Form Design window double-clicking on the object will cause the IDE to automatically write the event procedure stub for the default event of an object.
The programmer can type the event procedure stub into the Code Editor window.
In the Code Editor window, the programmer can first select the object from the Class combo box (at the top of the Code Editor window) and then select the Event from the Method combo box. This will cause the IDE to automatically write the event procedure stub for that object.
The easiest method is the double-click method, but this only works for one event in each object.
The combo box method can be used for any event and any object. Its advantage is that the procedure stub is created automatically.
The typing method should not be used because it is prone to operator error. The benefit of using the VB.Net IDE is that it can automatically and accurately create code. This is why VB.Net is a popular language for Rapid Application Development (RAD). Typing in code by hand defeats the benefit of having automatic code generation capabilities.
118. a) Create a structure named Employee that contains three variables: EmployeeID (Integer), LastName (String), FirstName (String), and Salary (Decimal). Then create a module level array that holds ten employees.
Structure Employee Dim EmployeeID As Integer Dim LastName As String Dim FirstName As String Dim Salary As Decimal End Structure
Dim mEmployees(9) As Employee
b) Create a Sub Procedure named InitializeID that uses a loop to put the values 1 to 10 in the EmployeeID for the employee's array.
Private Sub InitializeID() Dim I As Integer
For I = 0 To UBound(mEmployees) mEmployees(I).EmployeeID = I + 1 Next I
End Sub
9 - 15