Server-Side Scripting
Total Page:16
File Type:pdf, Size:1020Kb
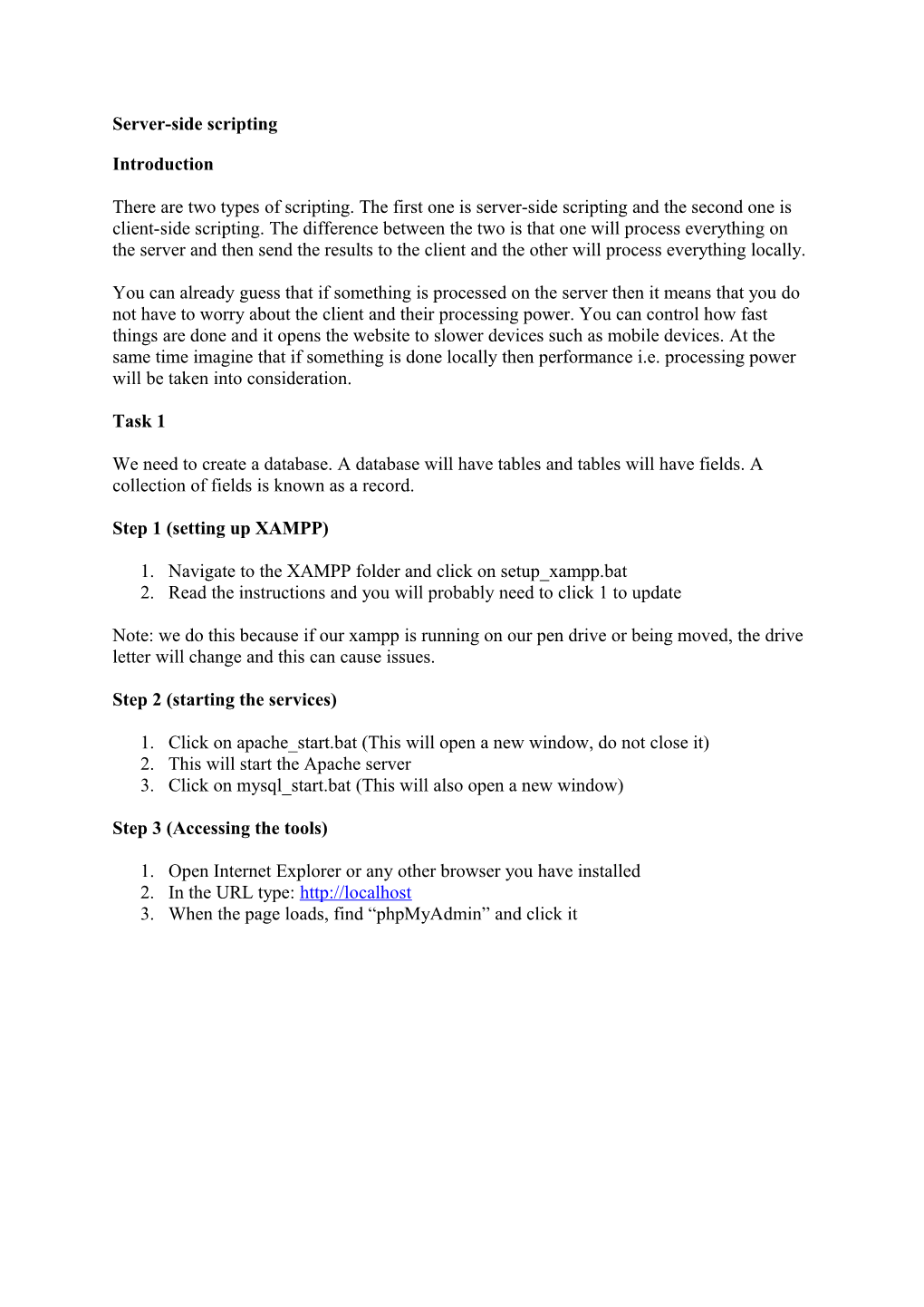
Server-side scripting
Introduction
There are two types of scripting. The first one is server-side scripting and the second one is client-side scripting. The difference between the two is that one will process everything on the server and then send the results to the client and the other will process everything locally.
You can already guess that if something is processed on the server then it means that you do not have to worry about the client and their processing power. You can control how fast things are done and it opens the website to slower devices such as mobile devices. At the same time imagine that if something is done locally then performance i.e. processing power will be taken into consideration.
Task 1
We need to create a database. A database will have tables and tables will have fields. A collection of fields is known as a record.
Step 1 (setting up XAMPP)
1. Navigate to the XAMPP folder and click on setup_xampp.bat 2. Read the instructions and you will probably need to click 1 to update
Note: we do this because if our xampp is running on our pen drive or being moved, the drive letter will change and this can cause issues.
Step 2 (starting the services)
1. Click on apache_start.bat (This will open a new window, do not close it) 2. This will start the Apache server 3. Click on mysql_start.bat (This will also open a new window)
Step 3 (Accessing the tools)
1. Open Internet Explorer or any other browser you have installed 2. In the URL type: http://localhost 3. When the page loads, find “phpMyAdmin” and click it Step 4 (Creating the database)
1. Give your database a name like the example above “tbooks” 2. Leave the default collation 3. Click create
Step 5 (creating the table)
1. Give the table a name i.e. “tcollection” 2. Specify the number of fields i.e. columns 3. Click on Go
Step 6 (Creating the fields)
We will have the following fields:
ID (unique to reference the book and this will be our primary key) Title (This will be the title for the book) Author (This will be who has written the book) Cost (This will be price in £)
Others we may add are whether it is available or not.
ID: Integer Index:Primary Title: VARCHAR Length: 200 Author: VARCHAR Length:200 Currency: Decimal
Click on Save.
This is what should have been inserted: CREATE TABLE `tbooks`.`tcollection` ( `ID` INT NOT NULL , `Title` VARCHAR( 200 ) NOT NULL , `Author` VARCHAR( 200 ) NOT NULL , `Cost` DECIMAL NOT NULL , PRIMARY KEY ( `ID` ) ) ENGINE = INNODB;
Example of what it will look like.
Step 7 (Creating your php page)
Last lesson we created a html page. Let’s quickly finish that design as a group and create a php page.
1. Open your XAMPP folder 2. Go to htdocs (This is where all of your php files will need to be stored) 3. Click file and save as in dreamweaver (you should have your web page open) 4. Navigate to that folder above and call the filename index.php
Step 8 (Connect to the database)
1. Declare php tags 2.
$link = mysql_connect ('localhost', 'root', ''); if(!$link){ die("Cannot connect to any database"); } $database = mysql_select_db ("tbooks", $link); if (!$database) { die("Could not connect to the database");
}
1. We make a connection 2. We connect to the database 3. If you get an error, you know it has not worked Step 9 (creating a form)
Step 10 (adding data)
@$title = $_POST['title']; @$author = $_POST['author']; @$cost = $_POST['cost'];
$sql = "INSERT INTO tcollection ( `ID` , `Title` , `Author` , `Cost`) VALUES ( '' , '$title', '$author', '$cost' )"; $result = mysql_query($sql, $link); if (!$result) { echo "DB Error, could not query the database\n"; echo 'MySQL Error: ' . mysql_error(); exit; }
Step 11 (reading data)
$sql2 = "SELECT * FROM tcollection"; $result2 = mysql_query($sql2, $link); if (!$result2) { echo "DB Error, could not query the database\n"; echo 'MySQL Error: ' . mysql_error(); exit; } while ($row = mysql_fetch_assoc($result2)) { echo "
Book Title | Book author | Cost |
"; echo $row['Title']; echo " | ";"; echo $row['Author']; echo " | ";"; echo $row['Cost']; echo " | ";