Methods (Part 1 Class Methods)
Total Page:16
File Type:pdf, Size:1020Kb
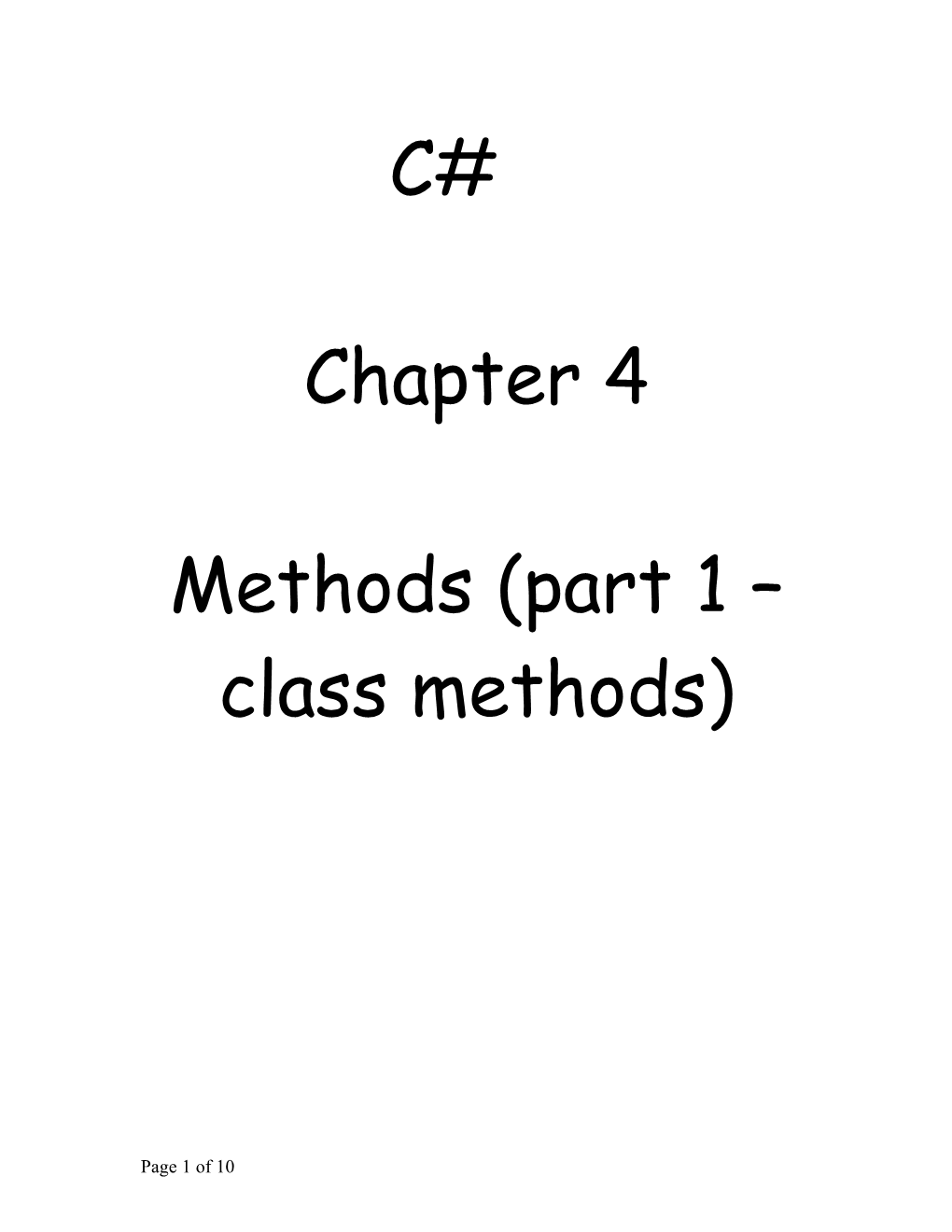
C#
Chapter 4
Methods (part 1 – class methods)
Page 1 of 10 Summary In Chapter 4 you will learn about methods. You will learn to write your class methods and how to call them (how to get them to run). You will also get experience in working with several Math class methods that are already part of the C# .NET library.
These notes contain some of the highlights of this chapter. You should add any additional notes on the page(s) at the end of this packet. If you run out of space, use loose leaf paper and put them after these pages. Include anything you feel is helpful or important from your work in this chapter.
Page 2 of 10 Methods
As programmers we need the ability to break large programs into smaller chunks that are easier to handle. In C# this is done by dividing your code into chunks known as methods. Methods are equivalent to what other languages call functions, procedures, or subroutines.
The purpose of methods is three-fold: First, they can be used over and over again preventing you from having to have to write that chunk of code, multiple times throughout your program Second, it is easier to code a single method correctly then to code it multiple times throughout the program (less chance for errors). Third, a well crafted method reduces the complexity of the program (better readability & easier to debug).
A well-defined method should stand for some concept; what does it do? What is it’s purpose? You should be able to describe the purpose of the method without using the words and or or. The method should do one thing. Name your methods appropriately.
Part 1: Class Methods There are two types of methods. Class Methods and Instance Methods. For now we will only be discussing Class Methods. When we start working on true object oriented programs, we will work with Instance Methods also. Class Methods provide the operations or behaviors for you data. Each class method performs a specific function. C# has many predefined class methods, one of which you are already very familiar with (Console.WriteLine). o Some Predefined Methods: ReadLine() method – returns a string o Use ReadLine() method to get any input from a user. ReadKey( ) method – this method is perfect to use when asking the user to ‘Hit Any Key to Continue’ (without hitting Enter). o Advanced: This method returns a data type that is out of scope for this class. We will only use it when asking the user to hit any key to continue. Read() method – returns the ASCII integer that represents the character entered.(not very useful for us right now). o DON’T use this to get input from the user. o Read ( ) is typically used in a loop to identify all characters that have been entered. o See teacher’s program called “ReadMethodTest2” for example. This will show that when you use the Read(
Page 3 of 10 ) method that two characters are tagged to the end of the entry. This information is saved until the next time you ask for input. So if you use the Read( ) method to ask for a letter, and then ask it again for another letter, the first entry would be filled with the first letter while the second entry would be filled with the return character (NOT GOOD! This is why we don’t use Read( ) to get input). You can also see from this program that Read ( ) actually returns an integer.
In addition to the predefined class methods, we will be writing our own class methods, so we can perform specific tasks.
Part 2: Anatomy of a Class Method When you define your method, you need to label the parts of its heading properly. Let’s look at the Main method to help us understand the parts of the heading of a Method.
private static void Main ( )
it’s parts are…
Modifiers: “private” (typically ‘public’ or ‘private’) & “static” (or nothing) Modifiers are added to a method’s heading to indicate how it can be accessed. For Class methods that we make we will use “public” always. o ‘Public’ means that other classes within your same solution (remember, a solution can have many classes) can access this method. o Advanced: When you create Instance Methods, you will often use ‘private’ instead of public. o If this is not specified then ‘private’ is assumed The Main method is usually private (or blank) to ensure data security. For Class Methods that we make we will use “static” always. o Advanced: When you learn about Instance Methods, you will learn that those types of methods do not use the “static” keyword.
Return Type: “void” (or any type) Return types tell what type (string, int, double, …) of value is returned when the method is completed. If the method is not sending back any information, then “void” is used. The Return Type is always listed before the name of the method.
Page 4 of 10 If the method does send data back, a return statement with the variable being sent back must be placed at the end of your method. **Your methods are only allowed to return one variable.**
Method Name: “Main” (or whatever you call yours) Make your name descriptive. Use PascalCasing if you are using multiple words. Use a verb or action phrase to name your method.
Argument List: ( ) If your method has variables that require input, then you will have variables declared in your parentheses after your method name. These variables are local to your method, but need to be initialized (“filled-in”) with some value. An example of a method heading that has an argument is given below; it says that the method has a string variable names “side” that needs a value sent to it when the method is called.
o Public static double GetDimension (string side)
If your method does not need any information from the outside world, then ( ), is used (empty). Note: your method can have variables declared in the Argument List and variables declared elsewhere in the Method Body (see below). The only difference is that the variables declared in the Argument List need values sent to them when the method is called.
Part 3: Writing a Class Method
Method Body: It’s like writing a mini-program Your method is written after the Main { }; that is after the Main opening and closing curly-braces. Also note that your method heading does NOT have a semicolon (;) placed at the end of it (just like the Main method heading didn’t). Each method has its own { } (opening and closing curly-braces); this is where you write all the coding for the method. Your file can have multiple methods. Writing your method o Declare / Initialize variables local to the method o You can only use variables that are defined in your method (either those defined in the argument list, or those declared in your method. o The Return statement is needed if your Return type is anything other than ‘void’. Else it’s optional.
Page 5 of 10 Part 4: Calling Class Methods (predefined or your own) When you call a Class Method you Invoke it; you make it run/execute; you make it do what it’s supposed to do After a method completes its execution, it returns control to the point where it was called from (it goes to the next statement after the call). Below is the structure to call a class method and an example we are used to seeing already.
[qualifier].MethodName> (
Example: Console.WriteLine(“Test Tomorrow”)
Exercise: Creating and Calling a Void Method: Write a method that prompts the user to hit any key to continue. Use the ReadKey method in your custom method. Call this method from the main program 3 times. Call your project “Ch04_FirstMethod”. When done, put this custom method in your toolbox so you can use it in future programs. o Also do in above exercise: Overload methods (show what this means when working on the previous exercise). Discuss how to see what options are available for a method call and what some of them do.
About using Ref and Out in Your
Page 6 of 10 More on Methods that are NOT Void If you call a method that returns some value it is not a VOID method. For these methods, you must make provisions for a location to store the value upon return from the method. For this, an assignment statement can be used.
Exercise: Calling a predefined Method that’s NOT Void Use the ReadLine method and create a custom method that prompts the user to input a string. You will call this method twice. Once to get the user’s first name, and a second time to get the user’s last name. Show the user’s whole name on a single line when done. Also when done, put this custom method on your toolbox so you can use it for future programs. Call your project “Ch04_SecondMethod”
Part 5: Working with Predefined Methods from the Math Class Next we are going to work with some predefined methods. Particularly, methods that perform mathematical functions. As we saw when we wrote our ‘SecondMethod’ program our method required we send it data of the type string. If we tried to send it an integer or any other type of data, it would have given errors. Predefined methods also require you to send it data of a specific type. A table of some of the Math class methods and the type of data they need to be sent (shown in the method calls) is given below. (page. 155 of the text has a more complete table). Method Description Method Heading Abs Returns the absolute value of myInt = Math.Abs(
Page 7 of 10 Note: second argument is exponent Sqrt Returns the square root of the myDouble = Math.Sqrt(
Often your programs will ask the user for input and then you will do something to the input probably using a method or some other operation.
Remember, when you ask a user for input using the ‘ReadLine’ method, you are ALWAYS returned a string.
Often the method or operation you perform on the input needs the data in a format other than the string type we always get from the ReadLine method. In order to convert the user’s string data into another type we can use the following methods from the convert class. These Convert class methods can be used to convert just about any type of data into another type.
Convert.To(
Where is typically one of the following: Int32, Bool, Char, Decimal, Double, String
The text talks about using the Parse method versus the Convert method. The Parse method has the DISADVANTAGE of only being able to handle string types as input, while the Convert methods (if you look at their overloads) can handle just about any type as input. For this reason, we will use the Convert class methods to change data types.
Exercise: Create a program that asks the user to enter 2 numbers (choose doubles as your numbers). Use your method from your “Ch04_SecondMethod” program when asking for user input. Find the smaller of the two numbers, and then take its square root and round it to the hundredths place. Display your results in a method as well. *Create at least 2 separate methods here* Call this program “Ch04_MathMethods”.
Page 8 of 10 MISCELLENAOUS TIPS WriteLine & ReadLine advance to the next line (Write & Read do not advance to the next line.) o If prompting the user for input that’s not too long, use ‘Write’ instead of ‘WriteLine’ to have the input be entered on the same line as the question you are asking. It looks better! GREAT ONLINE RESOURCE: http://dotnetperls.com/console Semicolons (;) are placed at the end of variable declarations, calls to methods, and assignment statements (not at the end of method headings, class headings, or namespace headings. Use ‘Breakpoints’ in your program when trying to debug. Look at the Local window when stopped at Breakpoints. Working with
Page 9 of 10 YOUR CHAPTER 4 NOTES In this space write any important notes that you have learned from answering the chapter exercises, writing the programming exercises, from the Quick Review, or from any class discussions.
Page 10 of 10