2.1 Dr. Brookshear's Machine Language Summary
Total Page:16
File Type:pdf, Size:1020Kb
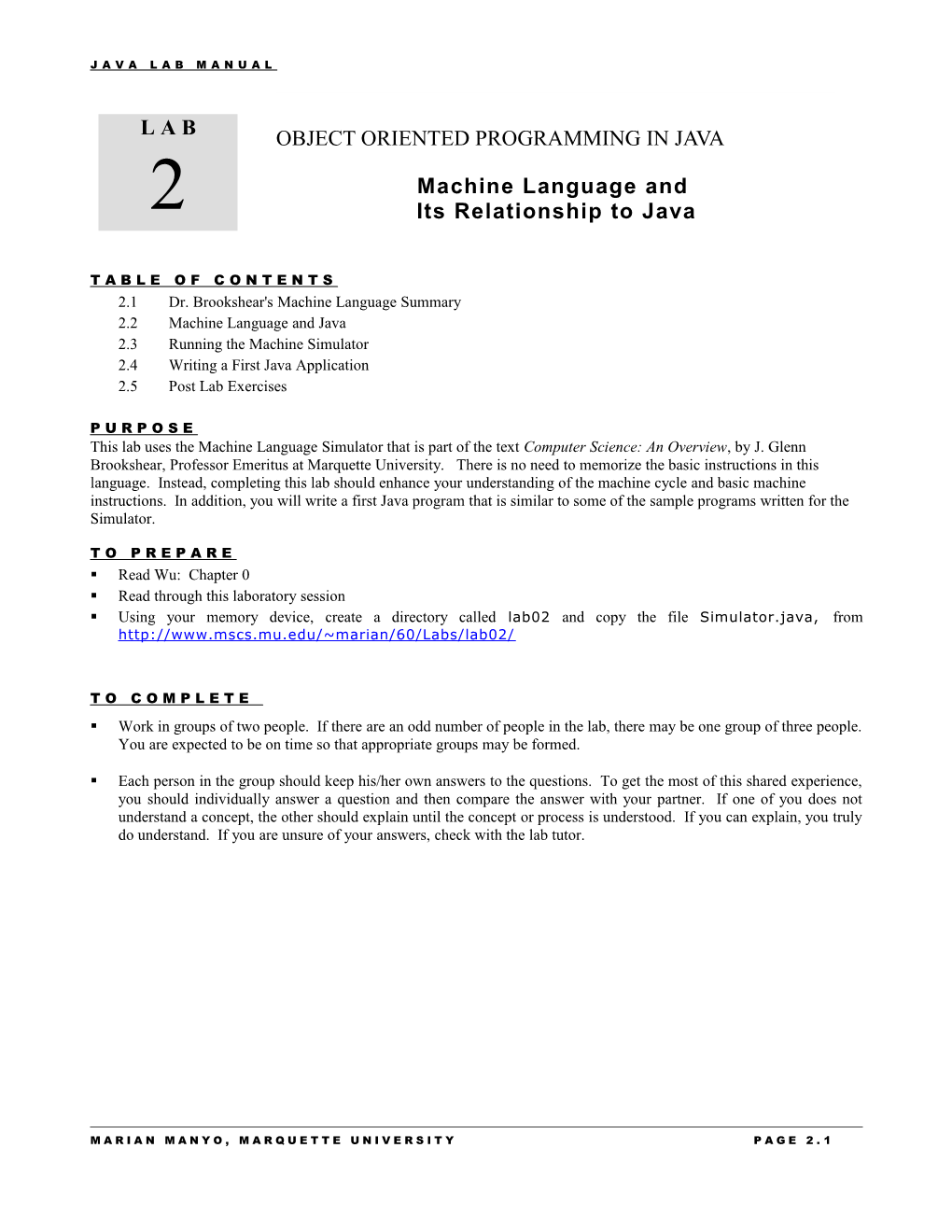
J A V A L A B M A N U A L
L A B OBJECT ORIENTED PROGRAMMING IN JAVA
Machine Language and 2 Its Relationship to Java
T A B L E O F C O N T E N T S 2.1 Dr. Brookshear's Machine Language Summary 2.2 Machine Language and Java 2.3 Running the Machine Simulator 2.4 Writing a First Java Application 2.5 Post Lab Exercises
P U R P O S E This lab uses the Machine Language Simulator that is part of the text Computer Science: An Overview, by J. Glenn Brookshear, Professor Emeritus at Marquette University. There is no need to memorize the basic instructions in this language. Instead, completing this lab should enhance your understanding of the machine cycle and basic machine instructions. In addition, you will write a first Java program that is similar to some of the sample programs written for the Simulator.
T O P R E P A R E . Read Wu: Chapter 0 . Read through this laboratory session . Using your memory device, create a directory called lab02 and copy the file Simulator.java, from http://www.mscs.mu.edu/~marian/60/Labs/lab02/
T O C O M P L E T E . Work in groups of two people. If there are an odd number of people in the lab, there may be one group of three people. You are expected to be on time so that appropriate groups may be formed.
. Each person in the group should keep his/her own answers to the questions. To get the most of this shared experience, you should individually answer a question and then compare the answer with your partner. If one of you does not understand a concept, the other should explain until the concept or process is understood. If you can explain, you truly do understand. If you are unsure of your answers, check with the lab tutor.
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 1 J A V A L A B M A N U A L
2.1 BROOKSHEAR'S MACHINE LANGUAGE SUMMARY The following table summarizes the machine language presented in Computer Science: An Overview. Each instruction is 16 bits, or two bytes, long and thus is represented by four hexadecimal digits.
Op – code Operand Description
1 RXY LOAD register R with the contents of the memory cell at address XY.
2 RXY LOAD register R with the value XY.
3 RXY STORE the contents of register R at memory location XY.
4 0RS MOVE the contents of register R to register S.
5 RST ADD the integer contents of registers S and T and leave the result in register R. Integers are stored using two's complement notation.
6 RST ADD the floating-point contents of registers S and T and leave the result in register R.
7 RST OR the contents of registers S and T and place the result in register R.
8 RST AND the contents of registers S and T and place the result in register R.
9 RST EXCLUSIVE OR the contents of registers S and T and leave the result in register R.
A R0X ROTATE the contents of register R one bit to the right X times.
B RXY JUMP to the instruction located at memory address XY if the contents of register R equals that of register 0.
C 000 HALT
Examples The machine instruction 1234 has op – code 1 and means: LOAD register R2 with the contents of the memory cell at address 34 The machine instruction 20FF has op – code 2 and means: LOAD register R0 with the value FF The machine instruction 34B0 has op – code 3 and means: STORE the contents of register R4 at memory location B0 The machine instruction 5602 has op – code 4 and means: ADD the contents of registers R0 and R2 and place the sum in register R6 The machine instruction B6A4 has op – code B and means: JUMP to the instruction in memory location A4 if the contents of register R6 equals that of register R0.
P A G E 2 . 2 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y J A V A L A B M A N U A L
2.2 MACHINE LANGUAGE AND JAVA The Central Processing Unit, or CPU, of a computer contains the circuitry that defines the instructions that the machine understands. These machine instructions are referred to as machine language. Developing complex programs in machine language is a tedious process. A high – level language is a programming language that is more compatible with human language and, therefore, easier for the programmer to use. In general, once a program has been written in a high-level language, it is translated into a machine language by a program called a translator, or compiler. The translated version of the program can then be executed by the machine. However, the translated version can be executed only by a machine that understands that particular machine language. This constraint is not compatible with the need to execute programs on different machines throughout the Internet. For example, a program that controls the animation of a Web page must be able to execute on any machine viewing the page. To overcome this obstacle, compilers for the Java language translate programs into a "generic machine language" called bytecode, which was developed by Sun Microsystems. Bytecode was designed so that different machines could "understand" programs in bytecode efficiently via a program called an interpreter, which interprets a single bytecode instruction into machine language instructions one instruction at a time. Today, most browsers used to surf the Web contain a bytecode interpreter. These browsers are said to be "Java enabled." This means that once a Java program has been translated into bytecode, the bytecode version can be transferred over the Internet to a variety of machines, each of which can execute the program efficiently by means of the Java interpreter. In short, a Java program is normally translated into a bytecode program and then this bytecode version is converted into machine-level instructions by means of an interpreter. The Java code is called source code and is stored in a file with a .java extension, called a source file. The compiled file contains bytecode and is stored in a file with a .class extension, called a bytecode file.
2.3 RUNNING THE MACHINE SIMULATOR The machine simulator program that we will run is a Java program written by Dr. Glenn Brookshear and Dr. Michael Slattery of Marquette University. This program should be on your memory device (see page 2.1) in the directory lab02 and is called Simulator.java. All Java programs are stored in a file with a .java extension.
To run the program, open the file Simulator.java inside the development environment software TextPad. Do the following.
1. Double click on the desktop icon for TextPad 2. Click on the open folder icon or select File – Open Change directories to the lab02 directory of your memory device. Select the source file Simulator.java and open it. You are not expected to read or understand this source code. 3. Save the file in the MyDocuments folder of the PC's hard drive. 4. To compile the source file into a bytecode file select Tools – Compile Java. A sound will ring and the source file will reappear when the compilation process is complete, which will take 5 – 10 seconds. The result should be several files with .class extentions that contain Java bytecode. If you would like to see the listing of these files, again go to Files – Open and choose Files of Type: All Files (*.*) and you should see the names of the bytecode files. Do not open these files since they are not readable. 5. To run the simulator, select Tools – Run Java Application . When the program runs you should see:
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 3 J A V A L A B M A N U A L
The Data Input Window allows you to type in data, including program instructions. The CPU contains sixteen registers, numbered 0 – F and two special registers PC, program counter, and IR, instruction register. The Main Memory consists of 256 bytes of memory. Each byte has an address, which can be determined by joining the row number and the column number. Thus the first byte in the first column of memory has address 00 and the last byte in the first column has address F0.
Across the bottom of the display window are buttons whose actions are: Button Action Clear Memory Change the contents of all memory cells to 00. Load Data Transfer data from the input window into the machine. Run Begin executing the machine cycle with the instruction stored at PC Single Step Execute a single machine cycle. Halt Stop executing the machine cycle Help Display a help package describing how to use the simulator.
P A G E 2 . 4 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y J A V A L A B M A N U A L
How to correctly use the program The machine is programmed by first typing data in the data input window and then transferring it to the machine by clicking the Load Data button. The syntax for typing data is as follows:
[P C] followed by a two-digit, hexadecimal address assigns an address to the program counter.
For e xa m ple [PC] 80 would change the program counter to 8016.
[nn] followed by a sequence of two-digit, hexadecimal values assigns the values to consecutive memory cells starting at address nn.
For e xa m ple [30] 40 56 C0 00 assigns the value 4016 to the memory cell at address 30 and the values 56, C0, 00 to the following cells at addresses 31, 32, and 33.
Once changes have been entered into the data input window they can be transferred into the machine by clicking the Load Data button. Thus, entering [PC] 00 [00] 20 FF 40 02 C0 00 in the data input window and then clicking the Load Data button sets the program counter to 00 and the memory cells starting at address 00 to 20, FF, 40, 02, C0, and 00.
Experiment 2.1 Familiarize yourself with the simulation program by following the steps below to enter and execute the following machine language program. Address Content
10 23 231F is the first instruction 11 1F 12 12 13 20 14 50 15 23 16 30 17 40 18 C0 19 00
S te p 1: Translate the program in this experiment into English. 231F ______1220 ______5023 ______3040 ______C000 ______If the contents at memory location 20 is 03, predict the final result. That is, explain what happens and what changes you expect to occur in memory. Predict: ______S te p 2: Run the simulation program and enter these three lines by typing this data in the input window.
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 5 J A V A L A B M A N U A L
[10] 23 1F 12 20 50 23 30 40 C0 00 program begins at memory cell 10 [20] 03 value at memory cell 20 is 03 [PC] 10 set Program Counter to 10
S te p 3: Transfer the data from the input window to the simulated machine by clicking the Load Data button. Inspect the main display to confirm that the program has been properly placed in main memory starting at location 10, 03 has been stored in memory location 20 and that the program counter is set to 10. Then, execute a single machine cycle, fetch – decode – execute, by clicking the Single Step button. At this point, what is the value stored in :
program counter ? ______instruction register ? ______register 3 ? ______
S te p 4 : Execute a single machine cycle again, Record the changes in the registers.
program counter ? ______instruction register ? ______register 2 ? ______
S te p 5 : Execute a single machine cycle again, Record the changes in the registers.
program counter ? ______instruction register ? ______register 0 ? ______
S te p 6 : Execute a single machine cycle again, Record the changes in the registers.
program counter ? ______instruction register ? ______memory location 40 ? ______
S te p 7 : Execute a single machine cycle again, Record the changes in the registers.
program counter ? ______instruction register ? ______
S te p 8: Was your prediction in correct?
______riting it in Java: A single Java instruction that performs the same operation as the above program is y = 31 + x; which loads a register with the decimal value 31 ( 1F16), loads the value stored in a memory location that we call x, adds the two values, and stores the result in a memory location that we call y. In algebra, the character '=' is read as "equals" and indicates that what is to the left of the '=' is equal to what is to its right. In Java, the character '=' is called the assignment operator. The statement y = 31 + x is read as " assign the sum of 31 and x to y ". S te p 9: Change the contents of the memory cell at address 14 to the hexadecimal value 90, instead of 50. Then, execute this modified program by changing the program counter back to 10 and clicking the Run button. Explain the difference in the actions of this program from those of the original program and in the results.
______
Experiment 2.2 While the following looks like an illegal algebraic statement, it is a valid Java statement. x = x + 5;
P A G E 2 . 6 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y J A V A L A B M A N U A L
This instruction assigns the sum of 5 and x to x. Write a machine language program for the Simulator, overwrites a value stored in memory by adding 5 onto it. Store the program beginning at memory location C0. Assume that x refers to memory location B0. The original value you store in x is up to you.
S te p 1: For each program instruction, record a statement that explains its effect.
______
S te p 2: Using the machine simulator, enter your program into the input box of the machine simulator, set the value of memory location B0, and set the program counter. Click the Load Data button. Run the program. Record the results, specifically record the value stored in B0 before and after the program is run.
______
S te p 3: Modify the program to accomplish the following: x = x + x; Run the program. Record your modification and the results, specifically record the value stored in B0 before and after the program is run.
Modification:______Results: ______
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 7 J A V A L A B M A N U A L
Experiment 2.3 (Optional) This experiment assumes that you have learned about the storage of integers using Two's Complement notation. You will be asked to decode the numbers that are computed and stored in the machine simulator using Two's Complement.
S te p 1: Write a program to find the sum of 7F16 and 0516, storing the sum at memory location 00. Record your program instructions.
______
S te p 2: Using the machine simulator, click on the Clear Memory button. Enter your program into the input box of the machine simulator and set the program counter. Click the Load Data button. Run the program. Record the results, specifically record the value stored in 00 before and after the program is run.
______
S te p 3: Record the addition problem in base 16, and base 2. Given that integers are stored using Two's Complement, interpret and explain these results in terms of the decimal equivalents.
______
P A G E 2 . 8 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y J A V A L A B M A N U A L
Experiment 2.4 In this experiment you will investigate the branch instruction, with op – code B, found in the machine language for the machine simulator. This program has two branch instructions, which together produce a loop. Recall the loops that were used in Lab 01 to describe the algorithms for converting a decimal whole number and a decimal fraction to binary.
S te p 1: Clear the simulated machine's main memory. Then, place the program below in the memory cells from address F0 to FD. [F0] 20 00 21 01 23 04 B3 FC 50 01 B0 F6 C0 00 [PC] F0
S te p 2: Record each instruction and a statement that explains its effect.
______
S te p 3: Execute the program one step at a time by clicking the Single Step button. Record the values of the program counter across. When a program counter value is a repeat, begin recording on a new line. For example: 00 02 04 06 08 04 06 08 04 0A 0D
Stop when the HALT instruction is loaded.
______
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 9 J A V A L A B M A N U A L
S te p 4: Here is the current program, showing the memory addresses of each instruction. The indicates which instructions are in the loop. Addresses Instruction F0 – F1 2000 F2 – F3 2101 F4 – F5 2304 F6 – F7 B3FC F8 – F9 5001 FA – FB B0F6 FC – FD C000 Again, execute using the single step button. Record on paper the values that are stored in the registers R0, R1 and R3 as the program is executed. When the value in a register changes, do not erase it. Instead, lightly cross out the overwritten value and then record the new value.
R0 R1 R3 00 00 00
Explain what condition determines when the loop ends? ______
What instruction is executed after the loop ends? ______
S te p 5: What changes should be made to the program in Step 1 if it is to be placed in memory starting at location A0? Be explicit.
______
S te p 6: W ri ti ng i t i n Ja va Java has three looping structures, one of which is called the while loop. This is how the loop in this experiment could be written in a high – level language such as Java. The condtion count != 4 is read as " count is not equal to 4 ". The statement count = count + 1 is repeatedly executed as long as (while) the condition is true. count = 0; while (count != 4) count = count + 1;
Intially, count is 0. The condition "count is not equal to 4" is true, so count + 1 is assigned to count, giving count a new value of 1. Again, the condition "count is not equal to 4" is true, so count is assigned 2. Again, the condition is true, so count is assigned 3. Again the condition is true, so count is assigned 4. Now, the condition " count is not equal to 4" is false, so the loop ends.
Com pl e te: A. The variable count above is equivalent to register ______in the machine language program.
B. The 4 above is stored in register ______in the machine language program.
C. What in the above code is equivalent to the first jump instruction in the machine code? ______D. Explain the effect of the second jump instruction in the machine code. ______
P A G E 2 . 1 0 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y J A V A L A B M A N U A L
2.4 WRITING A FIRST JAVA APPLICATION U S I N G T EX T PA D TO E D I T, C O M P I L E A N D RU N A J AVA P R O G R A M
This portion of the lab will take you through the step – by – step process for editing, compiling and running your first Java application, First. The program mimics the programs written in Experiments 2.1 and 2.2 for the Machine Simulator. The discussion of the design and implementation phases of a more complex program will be dealt with in class and future Labs.
S te p 1: Understand what problem the program is supposed to solve. P r oble m : Write a program that adds five onto a value stored in memory.
S te p 2: Hand write the program. Once you understand what a program is supposed to do, you should write the program using pencil and paper. This should always be done before you begin to enter the code in a text editor. Since I am supplying the code, you can bypass this step for now. //First Java Program by Tom Jones class First { public static void main(String[] args) { int number; number = 31; number = number + 5; } }
A brief explanation of the Java code above: //First Java Program by Tom Jones The two forward slashes define an in-line comment. Comments are not part of the program, but are for the benefit of the reader. You should replace the name Tom Jones with your name. class First - All Java code must be inside of a class. We begin with the Java reserved word class and then choose an appropriate name for the class. I have chosen First. The class is defined between a set of matching braces, {}, which define the beginning and end of the class. We say the braces delimit, define the beginning and end of, the class. The braces are called delimiters. public static void main(String[ ] args) is the header of the main method and must appear in all Java applications. A method contains a set of statements that are executed by the computer when the program is run. Again, the body of a method is delimited by a set of matching braces. int number; is a variable declaration statement . Such a statement reserves memory that can be accessed with the name number instead of using the actual memory address, as is necessary with machine language. In addition, this memory location will store an int, a four – byte integer value. It is the responsibility of the computer to keep a table of all variables used in a program along with information about each variable that includes the memory address associated with the variable and the type of data that is stored in this memory location. number = 31; assigns 31 (a decimal value) to the memory location that has the name number. number = number + 5; assigns number + 5 to number. Once the memory location number is overwritten with 36, the original value, 31, is lost.
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 1 1 J A V A L A B M A N U A L
A program statement ends with a semicolon. You should notice that the body of the main method contains three statements that declare the variable number, assign an initial value to number and then calculate and assign a new value to number.
S te p 3: Edit (Enter and save) the program Use a text editor to implement the program. Open TextPad. You should have an empty document that you will use to type the program. If you do not have a blank document, then either click on the New Document icon or go to File – New. Type in the program. When done save the file in the MyDocuments folder of the PC with a .java extension, as follows: o click on File – Save As. o When the Save As window opens o if necessary, navigate to the MyDoucments folder o look at the bottom portion of the window for the Save as type: textfield. If Java(*.java) does not appear in the textfield click on the menu arrow and choose Java(*.java). o type First into the File name: textfield since the name of the file should match the name of the class. o click the Save button.
S te p 4: Compile the program To compile the source file into a bytecode file, click on Tools – Compile Java. In order for a program to compile, it must follow all of the rules of Java syntax. If your file contains any kind of syntax error, such as a missing semi-colon or quote, the program will not compile. How can you tell if the program did or did not compile? . If it does not compile, you will see one or more error messages in Textpad. Error messages can be very informative. But, since this is a first program, any error message may be difficult for you to read. You must go back to Step 2 and edit the existing file to remove any syntax errors and then recompile the code. Except for spacing, you must follow the sample code exactly.
. If the file does compile, you will hear a sound and your file First.java will be displayed. The result of the compilation process is the creation of a new file called First.class that contains the Java bytecode version of the program. If you would like to see the listing of this file, again go to Files – Open and choose Files of Type: All Files (*.*) and you should see the name of the bytecode file. Do not open this file since it is not a readable file.
S te p 5: Run the program
Click on Tools – Run Java Application Record the results.
______
S te p 6: Maintain the program At this point, you can make changes to your program by going back to Step 2 followed by Steps 3 & 4. Modify your program by adding the highlighted lines.
P A G E 2 . 1 2 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y J A V A L A B M A N U A L
//First Java Program by Tom Jones class First { public static void main(String[] args) { int number; number = 31; System.out.println(number); number = number + 5; System.out.println(number); } }
System.out.println(number); prints the value stored in the memory location number to the monitor. System.out is the monitor. println is a message to print a new line. What is printed in this new line is determined by what is placed between the pair of parentheses. In this case, the variable number is passed, so the value stored in number is printed twice, after number is initialized to 31 and after 5 is added onto the value stored in number. Compile and run the modified program. Record the results.
______
L A B 0 2 I S A L M O S T C O M P L E T E 1. For 5 points, ask your Lab tutor to verify that you have successfully compiled and executed your first program for this class. 2. Al w a ys do a cl ea nup of M y Doc um e nts o Save any .java files in the lab02 directory on your memory device. o Remove the .java and .class files from MyDocuments. . close the open files inTextPad . double click the Shortcut to MyDocuments icon on the Desktop . highlight the .java and .class files that you created and delete them by choosing File – Delete or click on the big red X on the menu bar. 3. Take a 15 point open – note quiz
A S P A R T O F A S S I G N M E N T 0 2 Y O U W I L L L E A R N H O W T O Transfer your files to studsys for back-up Hand in a paper version of your program
M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y P A G E 2 . 1 3 J A V A L A B M A N U A L
2.5 POST LAB EXERCISES 2.6 Write a program in machine language that finds the sum of 45, 36 and 29. Store the sum in memory location 00. Record your program listing and write an explanation of what occurs when the program is run, 2.7 This exercise assumes that you know how integers are stored using two's complement notation. Write a program in machine language that finds the sum of 45, -36 and -29. Store the sum in memory location 00. Record your program listing and write an explanation of what occurs when the program is run, 2.8 In Lab 01, Exercise 1.10 you were asked to AND, OR and XOR the binary numerals 10101010 and 11110000. Write one program that finds these values, storing the results in address A0, B0 and C0. Record your program listing and write an explanation of what occurs when the program is run, 2.9 This assumes that you know how integers are stored using two's complement notation. Write a program that stores, in memory location A0, the opposite of the number stored in memory location B0. For example, if B0 stores the integer 5, ( or 05) then, after the program is run, A0 stores the integer -5 ( or FB). Hint, you must write instructions to perform the Two's Complement conversion. Record your program listing and write an explanation of what occurs when the program is run, 2.10 What does the following machine language program do? Write an explanation of what occurs when the program, which contains a loop, is run, To determine the purpose of the program, consider what happens if the content of memory location B4 is changed to some other value, such as 03, 06 or 0A. Address Instruction A0 – A1 10B4 A2 – A3 2100 A4 – A5 2201 A6 – A7 2300 A8 – A9 B3B0 AA – AB 5323 AC – AD 5113 AE – AF B0A8 B0 – B1 31B5 B2 – B3 C000 B4 – B5 0500
[A0] 10 B4 21 00 22 01 23 00 B3 B0 53 23 51 13 B0 A8 31 B5 C0 00 05 00 [PC] A0
P A G E 2 . 1 4 M A R I A N M A N Y O , M A R Q U E T T E U N I V E R S I T Y