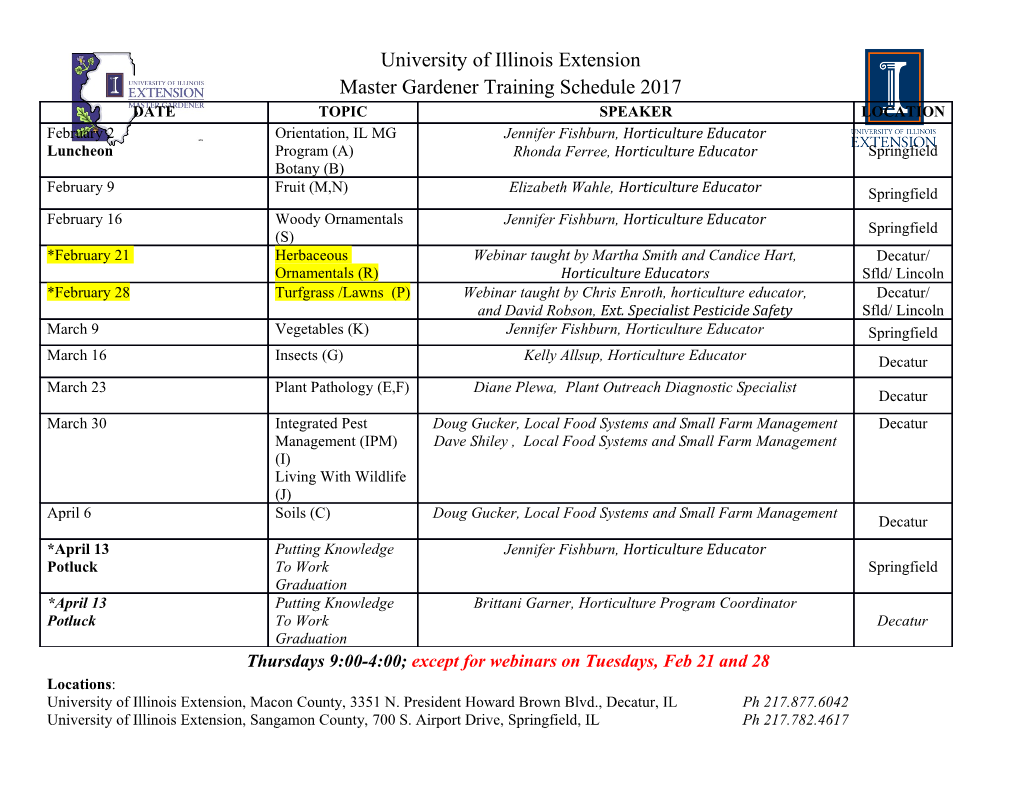
Linköping University | Department of Computer and Information Science Master’s thesis, 30 ECTS | Computer Science and Engineering 2021 | LIU-IDA/LITH-EX-A--21/054--SE Implementation and Evaluation of an Emulated Permission Sys‐ tem for VS Code Extensions using Abstract Syntax Trees Implementation och Utvärdering av ett Emulerat Be‐ hörighetssystem för Extensions i VS Code med hjälp av Abstrakta Syntaxträd David Åström Supervisor : Rouhollah Mahfouzi Examiner : Ahmed Rezine External supervisor : Magnus Kraft Linköpings universitet SE–581 83 Linköping +46 13 28 10 00 , www.liu.se Upphovsrätt Detta dokument hålls tillgängligt på Internet ‐ eller dess framtida ersättare ‐ under 25 år frånpublicer‐ ingsdatum under förutsättning att inga extraordinära omständigheter uppstår. Tillgång till dokumentet innebär tillstånd för var och en att läsa, ladda ner, skriva ut enstaka kopiorför enskilt bruk och att använda det oförändrat för ickekommersiell forskning och för undervisning. Över‐ föring av upphovsrätten vid en senare tidpunkt kan inte upphäva detta tillstånd. All annan användning av dokumentet kräver upphovsmannens medgivande. För att garantera äktheten, säkerheten och till‐ gängligheten finns lösningar av teknisk och administrativ art. Upphovsmannens ideella rätt innefattar rätt att bli nämnd som upphovsman i den omfattning somgod sed kräver vid användning av dokumentet på ovan beskrivna sätt samt skydd mot att dokumentet än‐ dras eller presenteras i sådan form eller i sådant sammanhang som är kränkande för upphovsmannens litterära eller konstnärliga anseende eller egenart. För ytterligare information om Linköping University Electronic Press se förlagets hemsida http://www.ep.liu.se/. Copyright The publishers will keep this document online on the Internet ‐ or its possible replacement ‐ for a period of 25 years starting from the date of publication barring exceptional circumstances. The online availability of the document implies permanent permission for anyone to read, to down‐ load, or to print out single copies for his/hers own use and to use it unchanged for non‐commercial research and educational purpose. Subsequent transfers of copyright cannot revoke this permission. All other uses of the document are conditional upon the consent of the copyright owner. The publisher has taken technical and administrative measures to assure authenticity, security and accessibility. According to intellectual property law the author has the right to be mentioned when his/her work is accessed as described above and to be protected against infringement. For additional information about the Linköping University Electronic Press and its procedures for publication and for assurance of document integrity, please refer to its www homepage: http://www.ep.liu.se/. © David Åström Abstract Permission systems are a common security feature in browser extensions and mobile ap- plications to limit their access to resources outside their own process. IDEs such as Visual Studio Code, however, have no such features implemented, and therefore leave extensions with full user permissions. This thesis explores how VS Code extensions access exter- nal resources and presents a proof-of-concept tool that emulates a permission system for extensions. This is done through static analysis of extension source code using abstract syntax trees, scanning for usage of Extension API methods and Node.js dependencies. The tool is evaluated and used on 56 popular VS Code extensions to evaluate what re- sources are most prevalently accessed and how. The study concludes that most extensions use minimal APIs, but often rely on Node.js libraries rather than the API for external functionality. This leads to the conclusion the inclusion of Node.js dependencies and npm packages is the largest hurdle to implementing a permission system for VS Code. Acknowledgments First of all, I would like to thank Cybercom Stockholm for the opportunity to conduct my thesis at their Innovation Zone and their constant support throughout the project. I would especially like to thank my supervisor Magnus Kraft as well as the other thesis students at the company for all the help and moral support during the distance work situation. I also want to thank my university supervisor Rouhollah Mahfouzi and examiner Ahmed Rezine for their feedback and aid with the project. iv Contents Abstract iii Acknowledgments iv Contents v List of Figures vii List of Tables viii Listings 1 1 Introduction 2 1.1 Motivation .......................................... 2 1.2 Aim .............................................. 2 1.3 Research questions ..................................... 3 1.4 Delimitations ........................................ 3 2 Theory 4 2.1 Software Extensions .................................... 4 2.2 Permission systems ..................................... 5 2.3 Visual Studio Code .................................... 6 2.4 TypeScript .......................................... 7 2.5 Static Analysis ....................................... 8 2.6 Related work ........................................ 8 3 Method 12 3.1 Pre-study .......................................... 12 3.2 Implementation ....................................... 13 3.3 Evaluation .......................................... 21 4 Results 23 4.1 Pre-study .......................................... 23 4.2 Implementation ....................................... 23 4.3 Evaluation .......................................... 24 5 Discussion 27 5.1 Results ............................................ 27 5.2 Method ............................................ 29 5.3 Source criticism ....................................... 31 5.4 The work in a wider context ............................... 31 6 Conclusion 33 6.1 Future work ......................................... 34 v Bibliography 35 A Tested Extensions 39 B Non-namespace permission messages 41 C Testing results - Permissions 43 D Testing results - Dependencies 45 vi List of Figures 3.1 Overview of the analyser architecture ............................ 13 3.2 AST generated from the line let variable = object.function(prop1, prop2) 14 3.3 Visualisation of the traversal algorithm ........................... 15 3.4 Visualisation of the visitor pattern ............................. 15 vii List of Tables 2.1 VS Code API namespaces, with description if applicable, as cited from the official documentation. ......................................... 7 3.1 Permission messages related to each namespace. ..................... 20 4.1 Permission messages detected in Bracket Pair Colorizer 2. 24 4.2 Permission messages detected in Path Intellisense. .................... 25 4.3 Permission messages detected in Live Server. ....................... 25 4.4 Precision and Recall Metrics. ................................. 25 4.5 Commonly occurring and interesting permissions found during testing . 26 4.6 Commonly occurring and interesting dependencies found during testing . 26 viii Listings 3.1 Tree traversal algorithm .................................. 14 3.2 Default import ....................................... 16 3.3 Namespace import ..................................... 16 3.4 Named import ....................................... 16 3.5 Combined type import .................................. 16 3.6 Require calls ........................................ 17 3.7 Variable declaration and property assignment ..................... 17 3.8 Variable assignment binary expression ......................... 17 3.9 Function declaration .................................... 17 3.10 Method call expression .................................. 17 3.11 Example of the ImportReference JSON structure. ................. 18 3.12 Example of the usedProps JSON structure ...................... 18 3.13 Example of the output data JSON structure ..................... 20 3.14 Bash command to extract installed extensions .................... 22 4.1 Bash command to run the analysis tool ........................ 23 4.2 Code line triggering Interact with the editor environment .............. 24 4.3 Code line triggering Access all visible extensions ................... 24 4.4 Code line triggering Access the active editor ...................... 25 1 1 Introduction 1.1 Motivation Many popular Integrated Development Environments (IDEs) and text editors use extensions or plugins as a way for the user to extend functionality and tune the system to their liking. This offers customisation to the user but could also pose risks. Extensions are external software, often open-source, which are installed onto a system and given rights to control certain parts of the application and/or system. It is therefore very possible that they could introduce new security vulnerabilities. Most web browsers implement similar systems for extensions and research has been done on the security of browser extensions. Results have shown that extensions have the possibility to introduce vulnerabilities, or even function as malware. However, little to no formal research seems to have been made on the security of IDE extensions and it is rarely discussed in internet communities. Despite this, many developers tend to install such plugins without much consideration. In today’s work environment, which is becoming more and more remote, the dependency on these digital tools and extensions become even more important. At the same time, the possibility for companies to control and monitor them becomes even lower, which would make this issue very interesting to explore. In this thesis, the security of
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages60 Page
-
File Size-