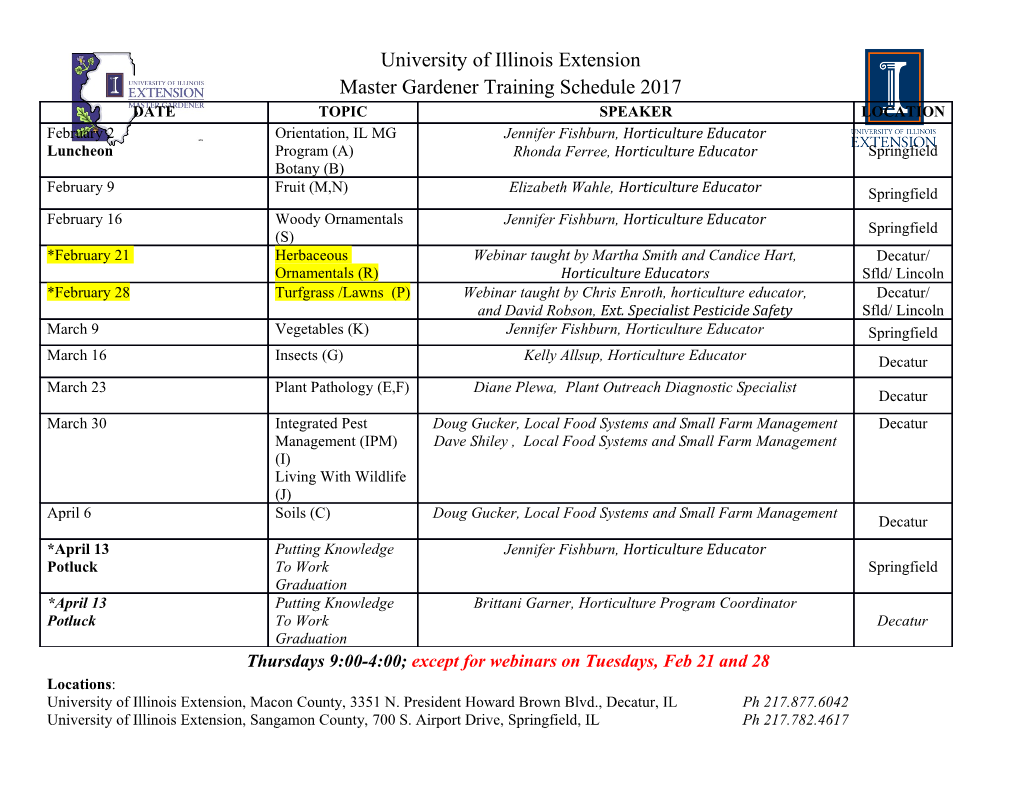
Turtle Graphics Python has a built-in module named turtle. See the Python turtle module API for details. Recursion with Turtles Use from turtle import * to use these commands: fd(dist) turtle moves forward by dist bk(dist) turtle moves backward by dist lt(angle) turtle turns left angle degrees rt(angle) turtle turns right angle degrees pu() (pen up) turtle raises pen in belly pd() (pen down) turtle lower pen in belly pensize(width) sets the thickness of turtle's pen to width CS111 Computer Programming pencolor(color) sets the color of turtle's pen to color shape(shp) sets the turtle's shape to shp Department of Computer Science home() turtle returns to (0,0) (center of screen) Wellesley College clear() delete turtle drawings; no change to turtle's state reset() delete turtle drawings; reset turtle's state setup(width,height) create a turtle window of given width and height 10-2 A Simple Example with Turtles Looping Turtles from turtle import * Loops can be used in conjunction with turtles to make interesting designs. setup(400,400) fd(100) # Draws a polygon with the specified number lt(60) # of sides, each with the specified length shape('turtle') def polygon(numSides, sideLength): pencolor('red') fd(150) rt(15) pencolor('blue') bk(100) pu() polygon(4,100) polygon(6,60) polygon(100,3) bk(50) pd() pensize(5) bk(250) pensize(1) home() 10-3 10-4 polygon(3,100) polygon(5,75) polygon(7,50) Looping Turtles Spiraling Turtles: A Recursion Example # Draws "flowers" with numPetals arranged around # a center point. Each petal is a polygon with # petalSides sides of length petalLen. def polyFlow(numPetals, petalSides, petalLen): spiral(200,90,0.9,10) spiral(200,72,0.97,10) spiral(200,80,0.95,10) polyFlow(7,4,80) polyFlow(10,5,75) polyFlow(11,6,60) spiral(200,121,0.95,15) spiral(200,95,0.93,10) 10-5 10-6 spiral(sideLen, angle, scaleFactor, minLength) Spiraling Turtles: A Recursion Example • sideLen is the length of the current side • angle is the amount the turtle turns left to draw the def spiral(sideLen, angle, scaleFactor, minLength): next side • scaleFactor is the multiplicative factor by which to scale the next side (it is between 0.0 and 1.0) • minLength is the smallest side length that the turtle will draw 400 spiral(625, 90, 0.8, 250) 320 500 256 625 10-7 10-8 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if sideLen >= minLength: fd(sideLen) lt(angle) spiral(sideLen*scaleFactor, angle, scaleFactor, minLength) 10-9 10-10 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(sideLen) fd(625) lt(angle) lt(angle) spiral(sideLen*scaleFactor, angle, spiral(sideLen*scaleFactor, angle, scaleFactor, minLength) scaleFactor, minLength) 10-11 10-12 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(sideLen*scaleFactor, angle, spiral(500, 90, scaleFactor, minLength) 0.8, 250) spiral(500, 90, 0.8, 250) if sideLen >= minLength: fd(sideLen) lt(angle) spiral(sideLen*scaleFactor, angle, scaleFactor, minLength) 10-13 10-14 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) 500 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(500, 90, 0.8, 250) if True: if True: fd(sideLen) fd(500) lt(angle) lt(angle) spiral(sideLen*scaleFactor, angle, spiral(sideLen*scaleFactor, angle, scaleFactor, minLength) scaleFactor, minLength) 10-15 10-16 spiral(625, 90, 0.8, 250) 500 spiral(625, 90, 0.8, 250) 500 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(500, 90, 0.8, 250) if True: if True: fd(500) fd(500) lt(90) lt(90) spiral(sideLen*scaleFactor, angle, spiral(400, 90, scaleFactor, minLength) 0.8, 250) spiral(400, 90, 0.8, 250) if sideLen >= minLength: fd(sideLen) lt(angle) spiral(sideLen*scaleFactor, angle, 10-17 scaleFactor, minLength) 10-18 400 spiral(625, 90, 0.8, 250) 500 spiral(625, 90, 0.8, 250) 500 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(500, 90, 0.8, 250) if True: if True: fd(500) fd(500) lt(90) lt(90) spiral(400, 90, spiral(400, 90, 0.8, 250) 0.8, 250) spiral(400, 90, 0.8, 250) spiral(400, 90, 0.8, 250) if True: if True: fd(sideLen) fd(400) lt(angle) lt(angle) spiral(sideLen*scaleFactor, angle, spiral(sideLen*scaleFactor, angle, scaleFactor, minLength) 10-19 scaleFactor, minLength) 10-20 400 400 spiral(625, 90, 0.8, 250) 500 spiral(625, 90, 0.8, 250) 500 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(500, 90, 0.8, 250) if True: if True: fd(500) fd(500) lt(90) lt(90) spiral(400, 90, spiral(400, 90, 0.8, 250) 0.8, 250) spiral(400, 90, 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) if True: if True: if sideLen >= minLength: fd(400) fd(400) fd(sideLen) lt(90) lt(90) lt(angle) spiral(sideLen*scaleFactor, angle, spiral(320, 90, spiral(sideLen*scaleFactor, angle, scaleFactor, minLength) 10-21 0.8, 250) scaleFactor, minLength)10-22 400 400 320 spiral(625, 90, 0.8, 250) 500 spiral(625, 90, 0.8, 250) 500 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(500, 90, 0.8, 250) if True: if True: fd(500) fd(500) lt(90) lt(90) spiral(400, 90, spiral(400, 90, 0.8, 250) 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) if True: if True: if True: if True: fd(400) fd(sideLen) fd(400) fd(320) lt(90) lt(angle) lt(90) lt(angle) spiral(320, 90, spiral(sideLen*scaleFactor, angle, spiral(320, 90, spiral(sideLen*scaleFactor, angle, 0.8, 250) scaleFactor, minLength)10-23 0.8, 250) scaleFactor, minLength)10-24 400 400 320 320 spiral(625, 90, 0.8, 250) 500 spiral(625, 90, 0.8, 250) 500 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) if True: if True: if sideLen >= minLength: fd(500) fd(500) fd(sideLen) lt(90) lt(90) lt(angle) spiral(400, 90, spiral(400, 90, spiral(sideLen*scaleFactor, angle, 0.8, 250) 0.8, 250) scaleFactor, minLength) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) if True: if True: if True: if True: fd(400) fd(320) fd(400) fd(320) lt(90) lt(90) lt(90) lt(90) spiral(320, 90, spiral(sideLen*scaleFactor, angle, spiral(320, 90, spiral(256, 90, 0.8, 250) scaleFactor, minLength)10-25 0.8, 250) 0.8, 250) 10-26 400 400 320 320 500 500 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) 256 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if True: fd(625) fd(625) lt(90) lt(90) spiral(500, 90, spiral(500, 90, 0.8, 250) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) if True: if True: if True: if True: fd(500) fd(sideLen) fd(500) fd(256) lt(90) lt(angle) lt(90) lt(angle) spiral(400, 90, spiral(sideLen*scaleFactor, angle, spiral(400, 90, spiral(sideLen*scaleFactor, angle, 0.8, 250) scaleFactor, minLength) 0.8, 250) scaleFactor, minLength) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) if True: if True: if True: if True: fd(400) fd(320) fd(400) fd(320) lt(90) lt(90) lt(90) lt(90) spiral(320, 90, spiral(256, 90, spiral(320, 90, spiral(256, 90, 0.8, 250) 0.8, 250) 10-27 0.8, 250) 0.8, 250) 10-28 400 400 320 320 500 500 spiral(625, 90, 0.8, 250) 256 spiral(625, 90, 0.8, 250) 256 625 625 spiral(625, 90, 0.8, 250) spiral(625, 90, 0.8, 250) spiral(204.8, 90, 0.8, 250) if True: if True: if sideLen >= minLength: fd(625) fd(625) fd(sideLen) lt(90) lt(90) lt(angle) spiral(500, 90, spiral(500, 90, spiral(sideLen*scaleFactor, angle, 0.8, 250) 0.8, 250) scaleFactor, minLength) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) if True: if True: if True: if True: fd(500) fd(256) fd(500) fd(256) lt(90) lt(90) lt(90) lt(90) spiral(400, 90, spiral(sideLen*scaleFactor, angle, spiral(400, 90, spiral(204.8, 90, 0.8, 250) scaleFactor, minLength) 0.8, 250) 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) spiral(400, 90, 0.8, 250) spiral(320, 90, 0.8, 250) if True: if True: if True: if True: fd(400) fd(320) fd(400) fd(320) lt(90) lt(90) lt(90) lt(90) spiral(320, 90, spiral(256, 90, spiral(320, 90, spiral(256, 90, 0.8, 250) 0.8, 250) 10-29 0.8, 250) 0.8, 250) 10-30 400 400 320 320 500 500 spiral(625, 90, 0.8, 250) 256 spiral(625, 90, 0.8, 250) 256 625 625 spiral(625, 90, 0.8, 250) spiral(204.8, 90, 0.8, 250) spiral(625, 90, 0.8, 250) if True: if False: if True: fd(625) fd(sideLen) fd(625) lt(90) lt(angle) lt(90) spiral(500, 90, spiral(sideLen*scaleFactor, angle, spiral(500, 90, 0.8, 250) scaleFactor, minLength) 0.8, 250) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) spiral(500, 90, 0.8, 250) spiral(256, 90, 0.8, 250) if True: if True:
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages20 Page
-
File Size-