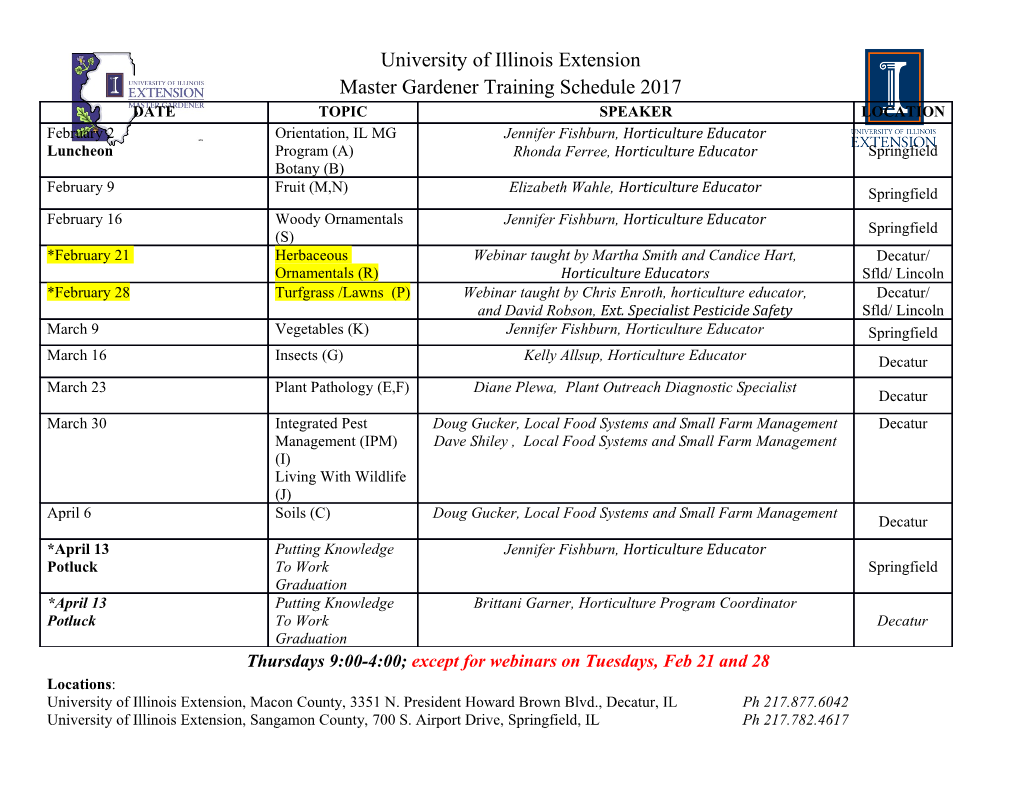
P0194R0- Static reflection (revision 4) Document number: P0194R0 Date: 2016-02-08 Project: Programming Language C++ Audience: Reflection (SG7) / EWG Reply-to: Mat´uˇsChochl´ık([email protected]), Axel Naumann ([email protected]) Static reflection (revision 4) Mat´uˇsChochl´ık,Axel Naumann Abstract This paper is the follow-up to N3996, N4111 and N4451 and it is the fourth revision of the proposal to add static reflection to the C++ standard. It also introduces and briefly describes a partial, experimental implementation of this proposal. Contents 1. Introduction 3 1.1. Revision history . .3 2. Concept specification 4 2.1. StringConstant . .4 2.2. Meta-Object . .5 2.2.1. is metaobject .......................................5 2.2.2. Definition . .5 2.2.3. reflects same .......................................5 2.2.4. get source location ...................................6 2.3. Meta-ObjectSequence . .6 2.3.1. is sequence ........................................6 2.3.2. Definition . .6 2.3.3. get size ..........................................6 2.3.4. get element ........................................7 2.4. Meta-Named . .7 2.4.1. has name ..........................................7 2.4.2. Definition . .7 2.4.3. get name ..........................................8 2.5. Meta-Typed . .8 2.5.1. has type ..........................................8 2.5.2. Definition . .8 2.5.3. get type ..........................................9 2.6. Meta-Scoped . .9 2.6.1. has scope ..........................................9 2.6.2. Definition . .9 2.6.3. get scope ..........................................9 2.7. Meta-Scope . 10 2.7.1. is scope .......................................... 10 2.7.2. Definition . 10 1 P0194R0- Static reflection (revision 4) 2.8. Meta-Alias . 10 2.8.1. is alias .......................................... 10 2.8.2. Definition . 11 2.8.3. get aliased ........................................ 11 2.9. Meta-Linkable . 11 2.9.1. is linkable ........................................ 11 2.9.2. Definition . 12 2.9.3. is static .......................................... 12 2.10. Meta-ClassMember . 12 2.10.1. is class member ...................................... 12 2.10.2. Definition . 12 2.10.3. is public .......................................... 13 2.11. Meta-GlobalScope . 13 2.11.1. is global scope ...................................... 13 2.11.2. Definition . 13 2.12. Meta-Namespace . 13 2.12.1. is namespace ........................................ 13 2.12.2. Definition . 14 2.13. Meta-NamespaceAlias . 14 2.13.1. Definition . 14 2.14. Meta-Type . 14 2.14.1. is type ........................................... 14 2.14.2. Definition . 15 2.14.3. get reflected type .................................... 15 2.15. Meta-TypeAlias . 15 2.15.1. Definition . 15 2.16. Meta-Class . 15 2.16.1. is class .......................................... 15 2.16.2. Definition . 16 2.16.3. get data members ..................................... 16 2.16.4. get all data members ................................... 16 2.17. Meta-Enum . 17 2.17.1. is enum ........................................... 17 2.17.2. Definition . 17 2.18. Meta-EnumClass . 17 2.18.1. Definition . 17 2.19. Meta-Variable . 17 2.19.1. is variable ........................................ 18 2.19.2. Definition . 18 2.19.3. get pointer ........................................ 18 2.20. Meta-DataMember . 18 2.20.1. Definition . 19 3. Reflection operator 19 3.1. Redeclarations . 19 3.2. Required header . 20 3.3. Future extensions . 20 4. Experimental implementation 20 2 P0194R0- Static reflection (revision 4) 5. Impact on the standard 21 5.1. Alternative spelling of the operator . 21 6. Known issues 21 7. References 21 Appendix 23 Appendix A. Diagrams 23 Appendix B. Examples of usage 27 B.1. Scope reflection . 27 B.2. Namespace reflection . 28 B.3. Type reflection . 29 B.4. Typedef reflection . 30 B.5. Class alias reflection . 31 B.6. Class data members (1) . 32 B.7. Class data members (2) . 34 B.8. Class data members (3) . 36 B.9. Simple serialization to JSON . 37 1. Introduction In this paper we propose to add native support for compile-time reflection to C++ by the means of compiler generated types providing basic metadata describing various program declarations. This paper introduces a new reflection operator and the initial subset of metaobject concepts which we assume to be essential and which will provide a good starting point for future extensions. When finalized, these metaobjects, together with some additions to the standard library can later be used to implement other third-party libraries providing both compile-time and run-time, high-level reflection utilities. Please refer to the previous papers [1, 2, 3, 4] for the motivation for this proposal, including some examples, design considerations and rationale. In [5] a complex use-case for a static reflection is described. 1.1. Revision history • Revision 4 { Further refines the concepts from N4111; prefixes the names of the metaobject oper- ations with get , adds new operations, replaces the metaobject category tags with new metaobject traits. Introduces a nested namespace std::meta which contains most of the reflection-related addi- tions to the standard library. Rephrases definition of meta objects using Concepts Lite. Specifies the reflection operator name { reflexpr. Introduces an experimental implementation of the reflection operator in clang. Drops the context-dependent reflection from N4111 (will be re-introduced later). • Revision 3 (N4451 [3]) { Incorporates the feedback from the discussion about N4111 at the Urbana meeting, most notably reduces the set of metaobject concepts and refines their definitions, removes some of the additions to the standard library added in the previous revisions. Adds context-dependent reflection. 3 P0194R0- Static reflection (revision 4) • Revision 2 (N4111 [2]) { Refines the metaobject concepts and introduces a concrete implementation of their interface by the means of templates similar to the standard type traits. Describes some additions to the standard library (mostly meta-programming utilities), which simpilfy the use of the metaobjects. Answers some questions from the discussion about N3996 and expands the design rationale. • Revision 1 (N3996 [1]) { Describes the method of static reflection by the means of compiler-generated anonymous types. Introduces the first version of the metaobject concepts and some possiblities of their implementation. Also includes discussion about the motivation and the design rationale for the proposal. 2. Concept specification We propose that the basic metadata describing a program written in C++ should be made available through a set of anonymous types { metaobjects, defined by the compiler and through related template classes implementing the interface of the metaobjects. At the moment these types should describe only the following program declarations: namespaces1, types, typedefs, classes and their data members and enum types. In the future, the set of metaobjects should be extended to reflect class inheritance, free functions, class member functions, templates, template parameters, enumerated values, possibly the C++ specifiers, etc. The compiler should generate metadata for the program declarations in the currently processed translation unit, when requested by the invocation of the reflection operator. Members of ordered sets (sequences) of metaobjects, like scope members, parameters of a function, base classes, and so on, should be listed in the order of appearance in the processed translation unit. Since we want the metadata to be available at compile-time, different base-level declarations should be reflected by statically different metaobjects and thus by different types. For example a metaobject reflecting the global scope namespace should be a different type than a metaobject reflecting the std namespace2, a metaobject reflecting the int type should have a different type then a metaobject reflecting the double type, etc. This section describes a set of metaobject concepts and their requirements, traits for metaobject classifi- cation and operations providing the individual bits of meta-data. Unless stated otherwise, all proposed named templates described below should go into the std::meta nested namespace in order to contain reflection-related definitions and to help avoiding potential name conflicts. 2.1. StringConstant StringConstant represents a compile-time character string type. struct StringConstant { typedef const char value_type[N+1]; static constexpr const char value[N+1]; typedef StringConstant type; }; 1in a limited form 2this means that they should be distinguishable for example by the std::is same type trait 4 P0194R0- Static reflection (revision 4) This concept could be replaced by the basic string constant from N4236. 2.2. Meta-Object A Meta-Object is a stateless anonymous type generated by the compiler (at the request of the programmer via the invocation of the reflexpr operator), providing metadata reflecting a specific program declaration. Instances of metaobjects cannot be constructed, i.e. metaobject types do not have any constructors. If the need for instantiation of metaobject types arises in practical use, then this requirement can be added in the future. 2.2.1. is metaobject In order to distinguish between regular types and metaobjects generated by the compiler, the is metaobject trait should be added directly to the std namespace as one of the type traits. template<typenameT> struct is_metaobject: integral_constant<bool, ...>{}; template<typenameT> constexpr bool is_metaobject_v= is_metaobject<T>::value;
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages38 Page
-
File Size-