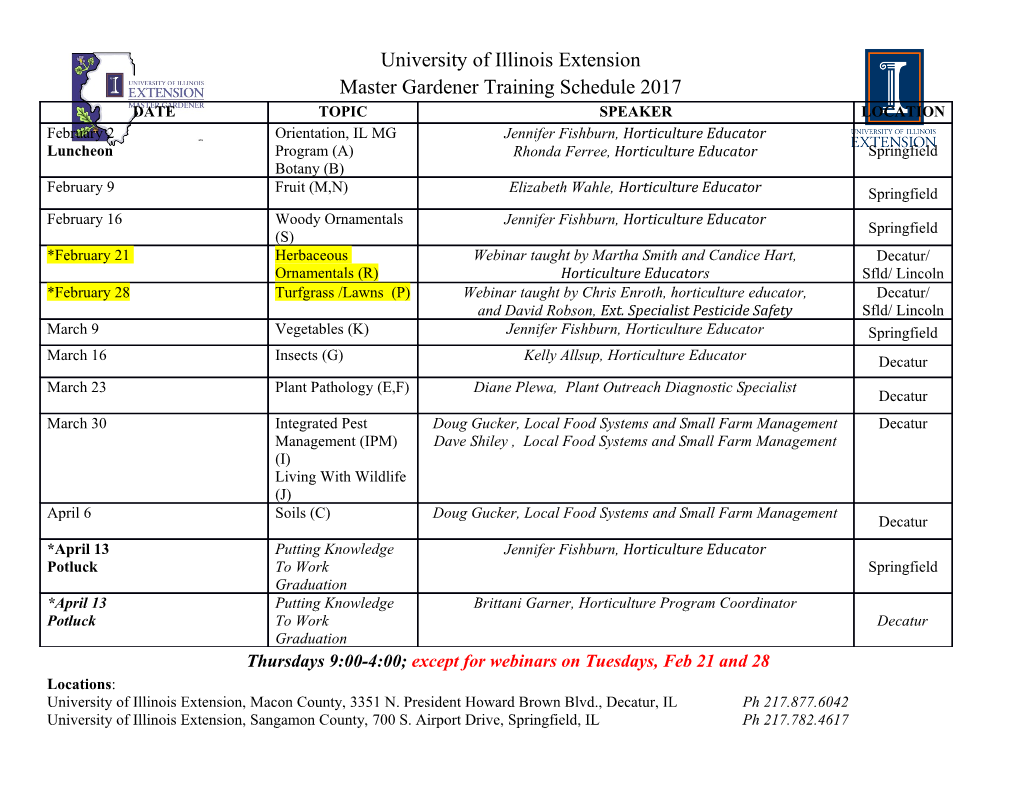
Introduction to Python for Computational Science and Engineering (A beginner's guide) Hans Fangohr Faculty of Engineering and the Environment University of Southampton September 7, 2015 2 Contents 1 Introduction 9 1.1 Computational Modelling . .9 1.1.1 Introduction . .9 1.1.2 Computational Modelling . .9 1.1.3 Programming to support computational modelling . 10 1.2 Why Python for scientific computing? . 11 1.2.1 Optimisation strategies . 12 1.2.2 Get it right first, then make it fast . 13 1.2.3 Prototyping in Python . 13 1.3 Literature . 13 1.3.1 Recorded video lectures on Python for beginners . 13 1.3.2 Python tutor mailing list . 14 1.4 Python version . 14 1.5 This document . 14 1.6 Your feedback . 14 2 A powerful calculator 17 2.1 Python prompt and Read-Eval-Print Loop (REPL) . 17 2.2 Calculator . 17 2.3 Integer division . 18 2.3.1 How to avoid integer division . 18 2.3.2 Why should I care about this division problem? . 19 2.4 Mathematical functions . 20 2.5 Variables . 21 2.5.1 Terminology . 22 2.6 Impossible equations . 22 2.6.1 The += notation . 23 3 Data Types and Data Structures 25 3.1 What type is it? . 25 3.2 Numbers . 25 3.2.1 Integers . 25 3.2.2 Long integers . 26 3.2.3 Floating Point numbers . 26 3.2.4 Complex numbers . 27 3.2.5 Functions applicable to all types of numbers . 27 3.3 Sequences . 27 3.3.1 Sequence type 1: String . 28 3.3.2 Sequence type 2: List . 29 3.3.3 Sequence type 3: Tuples . 31 3 4 CONTENTS 3.3.4 Indexing sequences . 32 3.3.5 Slicing sequences . 33 3.3.6 Dictionaries . 35 3.4 Passing arguments to functions . 37 3.4.1 Call by value . 37 3.4.2 Call by reference . 38 3.4.3 Argument passing in Python . 39 3.4.4 Performance considerations . 40 3.4.5 Inadvertent modification of data . 41 3.4.6 Copying objects . 42 3.5 Equality and Identity/Sameness . 42 3.5.1 Equality . 42 3.5.2 Identity / Sameness . 43 3.5.3 Example: Equality and identity . 43 4 Introspection 45 4.1 dir() . 45 4.1.1 Magic names . 46 4.2 type .............................................. 46 4.3 isinstance . 47 4.4 help . 47 4.5 Docstrings . 49 5 Input and Output 51 5.1 Printing to standard output (normally the screen) . 51 5.1.1 Simple print (not compatible with Python 3.x) . 51 5.1.2 Formatted printing . 52 5.1.3 \str" and \ str ".................................. 53 5.1.4 \repr" and \ repr "................................. 53 5.1.5 Changes from Python 2 to Python 3: print .................... 54 5.1.6 Changes from Python 2 to Python 3: formatting of strings . 54 5.2 Reading and writing files . 55 5.2.1 File reading examples . 56 6 Control Flow 59 6.1 Basics . 59 6.1.1 Conditionals . 59 6.2 If-then-else . 61 6.3 For loop . 61 6.4 While loop . 62 6.5 Relational operators (comparisons) in if and while statements . 62 6.6 Exceptions . 63 6.6.1 Raising Exceptions . 64 6.6.2 Creating our own exceptions . 65 6.6.3 LBYL vs EAFP . 65 7 Functions and modules 67 7.1 Introduction . 67 7.2 Using functions . 67 7.3 Defining functions . 68 7.4 Default values and optional parameters . 70 CONTENTS 5 7.5 Modules . 71 7.5.1 Importing modules . 71 7.5.2 Creating modules . 72 7.5.3 Use of name .................................... 73 7.5.4 Example 1 . 73 7.5.5 Example 2 . 74 8 Functional tools 77 8.1 Anonymous functions . 77 8.2 Map.............................................. 78 8.3 Filter . 78 8.4 List comprehension . 79 8.5 Reduce . 80 8.6 Why not just use for-loops? . 82 8.7 Speed . 83 9 Common tasks 85 9.1 Many ways to compute a series . 85 9.2 Sorting . 88 10 From Matlab to Python 91 10.1 Important commands . 91 10.1.1 The for-loop . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages167 Page
-
File Size-