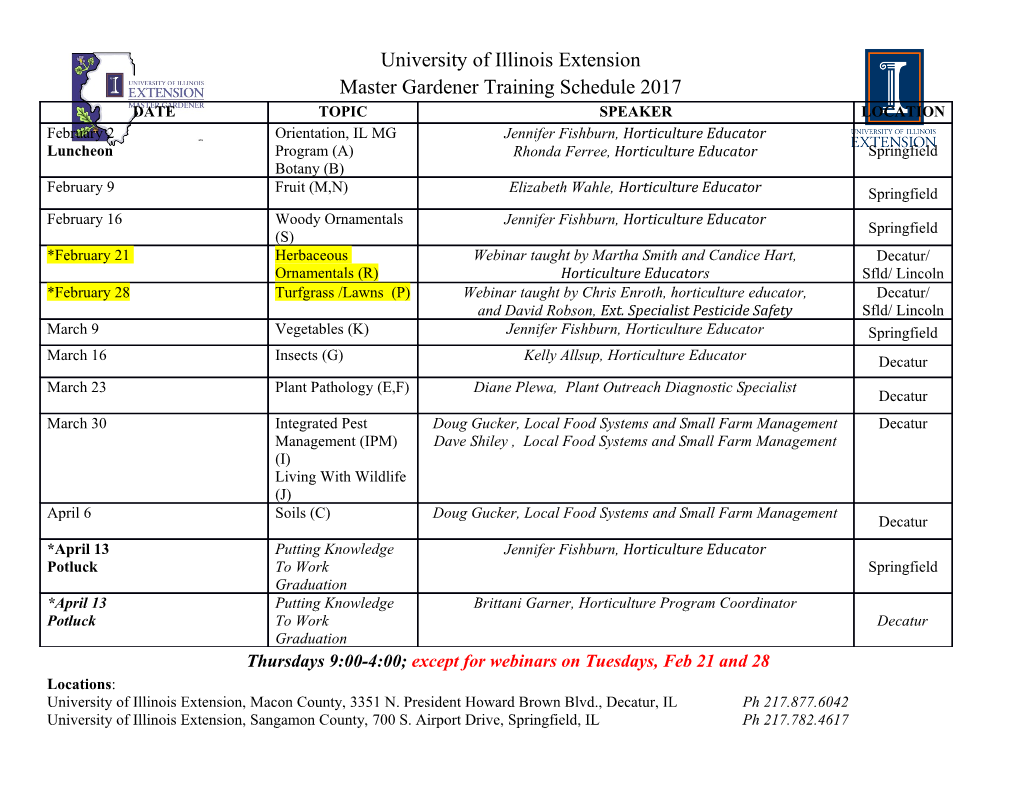
ECE232: Hardware Organization and Design Lecture 2: Hardware/Software Interface Adapted from Computer Organization and Design, Patterson & Hennessy, UCB Overview . Basic computer components • How does a microprocessor view memory? . Basic microprocessor components • ALU, register file, registers . Microprocessor instructions • Logic operations (AND, OR, etc) • Memory operations . MIPs instruction format • Control implemented as a series of instructions ECE232: MIPS Instructions-I 2 Computer Organization . 5 classic components of any computer Computer Keyboard, Processor Mouse (CPU) Memory Devices (active) (passive) Disk Control (where Input (where (“brain”) programs, programs, & data & data live when live when Datapath running) not running) Output Display, Printer . Fits in closely with datapath ECE232: MIPS Instructions-I 3 Program View of Memory 0 8 bits of data Computer 1 8 bits of data Processor Devices (CPU) 2 8 bits of data Input 8 bits of data Control Memory 3 4 8 bits of data Datapath Output 5 8 bits of data 6 8 bits of data ... . Memory viewed as a large, single -dimension array, with an address ? 8 bits of data . A memory address is an index into array . The index points to a byte of memory - "Byte addressing" . A 32-bit machine addresses memory by a 32-bit address . Access bytes (8 bits), words (32 bits) or half-words ECE232: MIPS Instructions-I 4 Memory – word addressing Memory Word 0 (bytes 0 to 3) Word 1 (bytes 4 to 7) CPU Address 0x00000000 Bus 0x00000004 0x00000008 0x0000000C 0x00000010 . Every word in memory has 0x00000014 an address 0x00000018 0x0000001C . Today machines address memory as bytes, hence word addresses differ by 4 0xfffffff4 • Memory[0], Memory[4], 0xfffffffc Memory[8], … 0xfffffffc Memory 4GB Max Called the “address” of a word (Typically 512MB-2GB) ECE232: MIPS Instructions-I 5 Addressing words: Big or Small Endian . Big Endian: address of most significant byte = word address (xx00 = Big End of word) • IBM 360/370, Motorola 68k, MIPS, Sparc, HP PA . Little Endian: address of least significant byte = word address (xx00 = Little End of word) • Intel 80x86, DEC Vax, DEC Alpha 3 2 1 0 little endian byte 0 msb lsb 0 1 2 3 big endian byte 0 ECE232: MIPS Instructions-I 6 Registers Computer Processor Devices (CPU) Input Control Memory Datapath Registers Output . Once a memory is fetched, the data must be placed somewhere in CPU . Advantages of registers • registers are faster than memory • registers can hold variables and intermediate results • memory traffic is reduced, so program runs faster • code density improves (later) ECE232: MIPS Instructions-I 7 Registers . code for A = B + C (This is not MIPS code, It is in English) load R1,B # R1 = B load R2,C # R2 = C add R3,R1,R2 # R3 = R1+R2 store R3,A # A = R3 . Many current processors support 32 registers (MIPS) . The more registers available, the fewer memory accesses will be necessary • Registers can hold lots of intermediate values . Instructions must include bits to specify which registers to operate on • register address ECE232: MIPS Instructions-I 8 Instruction Set Architecture (ISA) Application (FireFox) Operating System Compiler (Unix; Windows) Software Assembler Instruction Set Architecture Hardware Processor Memory I/O system Datapath & Control Digital Design Circuit Design transistors, IC layout . Key Idea: abstraction • hide unnecessary implementation details • helps us cope with enormous complexity of real systems ECE232: MIPS Instructions-I 9 The MIPS Instruction Set . Used as the example throughout the book . Stanford MIPS commercialized by MIPS Technologies (www.mips.com) . Large share of embedded core market • Applications in consumer electronics, network/storage equipment, cameras, printers, … . Typical of many modern ISAs • See MIPS Reference Data tear-out card, and Appendices B and E ECE232: MIPS Instructions-I 10 Arithmetic Operations . Add and subtract, three operands • Two sources and one destination add a, b, c # a gets b + c . All arithmetic operations have this form . Design Principle 1: Simplicity favors regularity • Regularity makes implementation simpler • Simplicity enables higher performance at lower cost ECE232: MIPS Instructions-I 11 Arithmetic Example . C or Java code: f = (g + h) - (i + j); . Compiled MIPS code: add t0, g, h # temp t0 = g + h add t1, i, j # temp t1 = i + j sub f, t0, t1 # f = t0 - t1 ECE232: MIPS Instructions-I 12 Register Operands . Arithmetic instructions use register operands . MIPS has a 32 × 32-bit register file • Use for frequently accessed data • Numbered 0 to 31 • 32-bit data called a “word” . Assembler names • $t0, $t1, …, $t9 for temporary values • $s0, $s1, …, $s7 for saved variables . Design Principle 2: Smaller is faster • c.f. main memory: millions of locations ECE232: MIPS Instructions-I 13 Register Operand Example . C or Java code: f = (g + h) - (i + j); • f, …, j in $s0, …, $s4 . Compiled MIPS code: add $t0, $s1, $s2 add $t1, $s3, $s4 sub $s0, $t0, $t1 ECE232: MIPS Instructions-I 14 Simplified Datapath . Two register fetches in one cycle . Load from memory . Store to memory A Registers L Data U Memory From Register for SW To register for LW ECE232: MIPS Instructions-I 15 Memory Operands . Main memory used for composite data • Arrays, structures, dynamic data . To apply arithmetic operations • Load values from memory into registers • Store result from register to memory . Memory is byte addressed • Each address identifies an 8-bit byte . Words are aligned in memory • Address must be a multiple of 4 . MIPS is Big Endian • Most-significant byte at least address of a word • c.f. Little Endian: least-significant byte at least address ECE232: MIPS Instructions-I 16 Memory Operand Example 1 . C code: g = h + A[8]; • g in $s1, h in $s2, base address of A in $s3 . Compiled MIPS code: • Index 8 requires offset of 32 • 4 bytes per word lw $t0, 32($s3) # load word add $s1, $s2, $t0 offset base register ECE232: MIPS Instructions-I 17 Memory Operand Example 2 . C code: A[12] = h + A[8]; • h in $s2, base address of A in $s3 . Compiled MIPS code: • Index 8 requires offset of 32 lw $t0, 32($s3) # load word add $t0, $s2, $t0 sw $t0, 48($s3) # store word ECE232: MIPS Instructions-I 18 Registers vs. Memory . Registers in a register file are faster to access than memory . Operating on memory data requires loads and stores • More instructions to be executed . Compiler must use registers for variables as much as possible • Only spill to memory for less frequently used variables • Register optimization is important! . Registers are a fixed resources ECE232: MIPS Instructions-I 19 Immediate Operands . Constant data specified in an instruction addi $s3, $s3, 4 . No subtract immediate instruction • Just use a negative constant addi $s2, $s1, -1 . Design Principle 3: Make the common case fast • Small constants are common • Immediate operand avoids a load instruction ECE232: MIPS Instructions-I 20 The Constant Zero . MIPS register 0 ($zero) is the constant 0 • Cannot be overwritten . Zero is used a lot for arithmetic operations . Useful for common operations • E.g., move between registers add $t2, $s1, $zero ECE232: MIPS Instructions-I 21 MIPS Registers . Fast access to program data . Register R0/$0/$zero: hardwired to constant zero . Register names: • $0-$31 or R0-R31 • Specialized names based on usage convention • $zero ($0) - always zero • $s0-$s7 ($16-$23) - “saved” registers • $t0-$t7 ($8-$15) - “temporary” registers • $sp - stack pointer • Other special-purpose registers ECE232: MIPS Instructions-I 22 MIPS Registers and Usage Name Register number Usage $zero 0 the constant value 0 $at 1 reserved for assembler $v0-$v1 2-3 values for results and expression evaluation $a0-$a3 4-7 arguments $t0-$t7 8-15 temporary registers $s0-$s7 16-23 saved registers $t8-$t9 24-25 more temporary registers $k0-$k1 26-27 reserved for Operating System kernel $gp 28 global pointer $sp 29 stack pointer $fp 30 frame pointer $ra 31 return address ECE232: MIPS Instructions-I 23 Summary . Basic microprocessor includes a register file, an ALU, and control circuitry . Microprocessors interact with memory • Memory access is slow and (when possible) should be avoided • Load and store instructions used to access memory . Microprocessors operate using primitive instructions • Logic operations • Arithmetic operations • Load and stores • More to come . Next time: More instructions, instruction implementation ECE232: MIPS Instructions-I 24.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages24 Page
-
File Size-