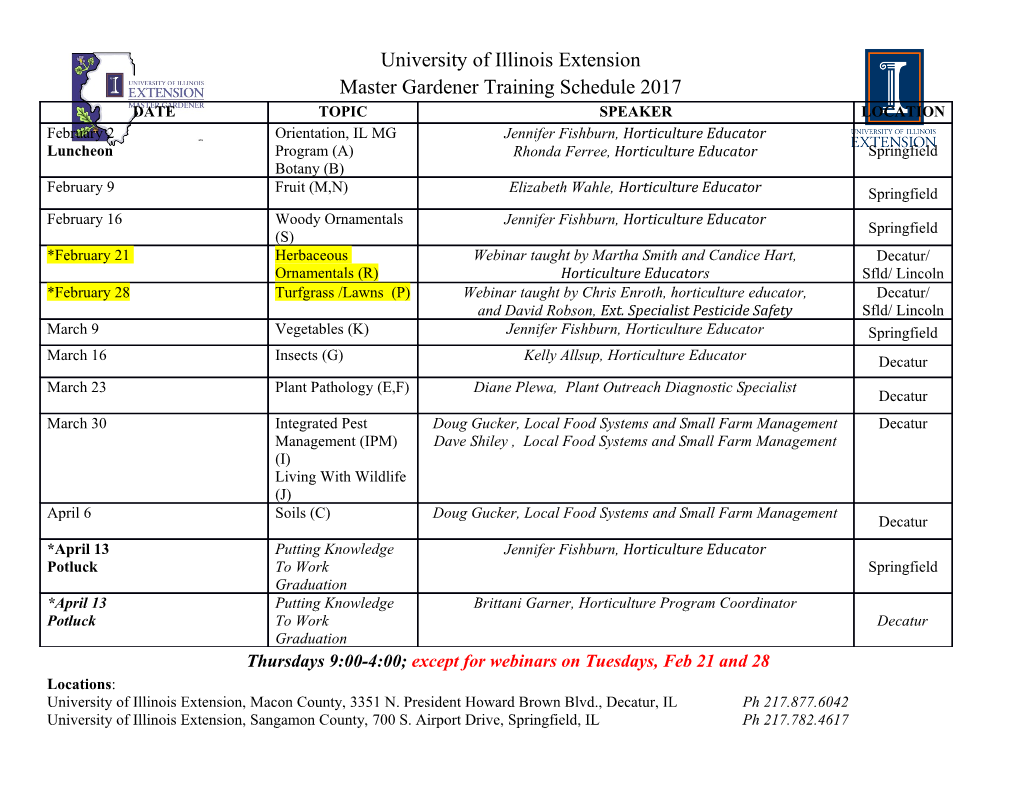
The GGZ Game Development Guide Development of games for the GGZ Gaming Zone 0.99.4+ GGZ Development Team This manual is about game development for the GGZ Gaming Zone environment. Copyright c 2002 - 2008 Josef Spillner Published under the GNU General Public License. i Table of Contents 1 Foreword :::::::::::::::::::::::::::::::::::::::: 1 2 The World of GGZ Games ::::::::::::::::::::: 3 2.1 Games already shipped with GGZ :::::::::::::::::::::::::::::: 3 2.2 Externally developed games which use GGZ :::::::::::::::::::: 3 2.3 Embedded games :::::::::::::::::::::::::::::::::::::::::::::: 3 3 Diving into GGZ:::::::::::::::::::::::::::::::: 5 3.1 Preparing a developer setup :::::::::::::::::::::::::::::::::::: 5 3.2 Distribution:::::::::::::::::::::::::::::::::::::::::::::::::::: 5 4 Game Clients ::::::::::::::::::::::::::::::::::: 7 4.1 Introduction ::::::::::::::::::::::::::::::::::::::::::::::::::: 7 4.2 The client base library: libggzmod:::::::::::::::::::::::::::::: 7 4.2.1 Example in C, using glib :::::::::::::::::::::::::::::::::: 8 4.2.2 Example in C, using GGZPassive :::::::::::::::::::::::::: 9 4.2.3 Example in Python:::::::::::::::::::::::::::::::::::::::: 9 4.2.4 Example in C++ for Qt-based games ::::::::::::::::::::: 10 4.3 Game Registry :::::::::::::::::::::::::::::::::::::::::::::::: 12 4.4 Client Toolkits :::::::::::::::::::::::::::::::::::::::::::::::: 13 4.4.1 KDE or Qt (C++, Python) :::::::::::::::::::::::::::::: 13 4.4.2 Gtk+, GNOME (C) :::::::::::::::::::::::::::::::::::::: 14 4.4.3 SDL (C, C++, Python) :::::::::::::::::::::::::::::::::: 14 4.4.4 Swing and AWT (Java) :::::::::::::::::::::::::::::::::: 14 4.4.5 Others ::::::::::::::::::::::::::::::::::::::::::::::::::: 15 5 Game Servers :::::::::::::::::::::::::::::::::: 17 5.1 Introduction :::::::::::::::::::::::::::::::::::::::::::::::::: 17 5.2 The server base library: libggzdmod ::::::::::::::::::::::::::: 17 5.3 Game statistics: libggzstats ::::::::::::::::::::::::::::::::::: 18 5.4 Configuration Files:::::::::::::::::::::::::::::::::::::::::::: 18 5.5 Programming Language ::::::::::::::::::::::::::::::::::::::: 19 5.5.1 C Development::::::::::::::::::::::::::::::::::::::::::: 19 5.5.2 C++ Development ::::::::::::::::::::::::::::::::::::::: 19 5.5.3 Python Development ::::::::::::::::::::::::::::::::::::: 20 5.5.4 Ruby Development ::::::::::::::::::::::::::::::::::::::: 20 5.5.5 Java development :::::::::::::::::::::::::::::::::::::::: 20 ii The GGZ Gaming Zone 0.99.2+ - Game Development Guide 6 Programming Details ::::::::::::::::::::::::: 21 6.1 Introduction :::::::::::::::::::::::::::::::::::::::::::::::::: 21 6.2 Game features :::::::::::::::::::::::::::::::::::::::::::::::: 21 6.2.1 Statistics::::::::::::::::::::::::::::::::::::::::::::::::: 21 6.2.2 Savegames ::::::::::::::::::::::::::::::::::::::::::::::: 21 6.2.3 Named bots :::::::::::::::::::::::::::::::::::::::::::::: 21 6.3 Development and debugging tools ::::::::::::::::::::::::::::: 22 6.4 General purpose wrappers ::::::::::::::::::::::::::::::::::::: 22 6.4.1 ggzwrap:::::::::::::::::::::::::::::::::::::::::::::::::: 22 6.4.2 ShadowBridge:::::::::::::::::::::::::::::::::::::::::::: 22 6.4.3 Go client ::::::::::::::::::::::::::::::::::::::::::::::::: 22 6.4.4 External AI players :::::::::::::::::::::::::::::::::::::: 22 6.5 Network connections :::::::::::::::::::::::::::::::::::::::::: 23 6.5.1 Easysock (C), now libggz ::::::::::::::::::::::::::::::::: 23 6.5.2 DIO (C), in libggz:::::::::::::::::::::::::::::::::::::::: 23 6.5.3 Qt (C++) ::::::::::::::::::::::::::::::::::::::::::::::: 24 6.5.4 KDE (C++) ::::::::::::::::::::::::::::::::::::::::::::: 24 6.5.5 Net/MNet (C++), now ggzdmod++ ::::::::::::::::::::: 25 6.5.6 Network (Python) :::::::::::::::::::::::::::::::::::::::: 25 6.6 Configuration ::::::::::::::::::::::::::::::::::::::::::::::::: 26 6.6.1 GGZConfIO (ini-Style), in libggz ::::::::::::::::::::::::: 26 6.6.2 Minidom (XML-Style), now in libggzmeta :::::::::::::::: 26 6.7 Game development frameworks :::::::::::::::::::::::::::::::: 27 6.7.1 Developing card game servers with GGZCards :::::::::::: 27 6.7.2 GGZBoard, the board game client container :::::::::::::: 27 6.8 Special libraries ::::::::::::::::::::::::::::::::::::::::::::::: 27 6.8.1 GGZChess, an artificial intelligence for chess:::::::::::::: 27 6.8.2 Data updates with Get Hot New Stuff :::::::::::::::::::: 27 Chapter 1: Foreword 1 1 Foreword The central element of the GGZ Gaming Zone is, obviously, everything related to games, especially the games themselves. From the very beginning, games of any kind have been a part of GGZ. After a long time of premature development, a lot of experience is present to be shared with the humble reader. As games are very different from applications, it is not easy to recommend forthis or against that. Instead, a general collection of hints is presented, divided into the major differences on the client side (frontends) and the server side (programming languages). This guide covers the following platforms for GGZ game development: KDE/Qt, GNOME/Gtk+, Python (with SDL/Pygame), pure SDL in C, Java and pure C/C++ environments without any graphical toolkits. The more games provide GGZ support, the more fun it becomes. After reading this guide, all questions about how to provide GGZ support from a game developer's perspective should be answered. Chapter 2: The World of GGZ Games 3 2 The World of GGZ Games 2.1 Games already shipped with GGZ With more than a dozen games available, which can be played using nearly two dozen game clients, GGZ offers a solid bunch of different games by itself. They're all developed based on a client/server model. So how does that work? The player, using a GGZ core client to chat with others in a room, launches a game which he wants to play with some others. Technically, a table is created to hold that game, and the player is put onto that table, occupying one seat. The other available seats can be left empty, filled up with bots or used by other players. The quantity ofeachtype is determined by the game server, for example, some games might not support bots (AI players) at all, or it might support an unlimited number of players. GGZ games are available for basically all standard development platforms. This makes it convenient to take a look at one of the existing games in order to find out programming details. In particular, the Tic-Tac-Toe game is simple enough that it has been awarded the title of being the example game for all platforms. 2.2 Externally developed games which use GGZ GGZ offers wonderful features to consider for integration into any game. With little work, game developers can foster player communities, integrate flexible protocols and promote their game to a wider audience. The chapters on client and server development will discuss the available options in detail. External game work no different to internal ones. However, a few assumptions canbe made, for example, most external game have been present before they got a GGZ mode, and are thus more complex in design. In the future, some GGZ game servers and clients will most likely also provide a standalone mode, just like some clients already do for local games, namely Muehle, KTicTacTux, Geekgame, Escape/SDL, Xadrez Chines, GGZBoard and Koenig. 2.3 Embedded games Some games already come with a connection dialog, running in fullscreen and displaying chat messages of other players. In these cases it might be advisable to "embed" the ggzcore library into the game client, letting it perform all the work which traditionally would be done by a GGZ core client. Right now, only one external game runs in embedded mode, namely Widelands. In order to do so, ggzcore must be initialized with the OPT EMBEDDED flag switched on. The ggzcore API documentation provides more facts and details about the embedded mode. Chapter 3: Diving into GGZ 5 3 Diving into GGZ 3.1 Preparing a developer setup Prior to looking into adding GGZ support to a game, it might be useful to understand the GGZ build process. Most likely the game will have a different one but sometimes the situation arises in which one wants to debug GGZ itself. If you're sure you do not ever want this, feel free to skip this part of the guide. A lot of external software is used in GGZ which should first be installed, along with the developer tools. The ggzprep utility, as found in playground/maintenance, can do this automatically for some popular Linux distributions. A full GGZ dependency tree weighs in more than 150 MB of binary packages, so the download might take some time. Building GGZ from source is quite easy but depending on the package a number of tools might be needed. Most parts (C/C++) are autotools-based, meaning they use automake, autoconf and libtool to produce makefiles. The Python package uses autoconf only and the Java package uses the ant system. GGZ Community comes with its own hand-rolled system. The KDE package has switched to CMake recently. Aligning with the build system of the respective platform has proven to be the best and easiest for developers of the platform in question, but of course where they all come together (in GGZ) some care is needed. Thankfully, the ggzbuild tool has been invented. It can be found in playground/maintenance as well, together with a ready-to-go configuration file named ggzbuildrc.sample. Copy this file to ~/.ggzbuildrc and modify as needed (changing the installation directory is recommended). Afterwards, calling ggzbuild either in GGZ's top-level trunk/branch directory or in each individual module directory
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages32 Page
-
File Size-