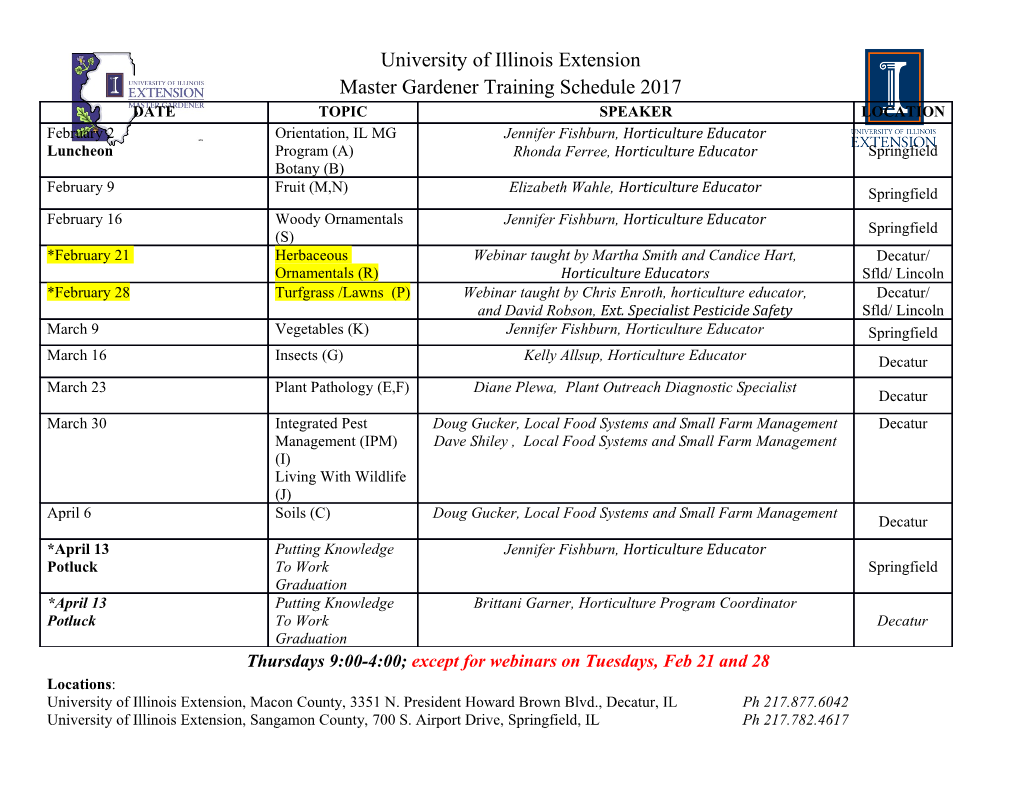
Linux GPU Driver Developer’s Guide Release 4.13.0-rc4+ The kernel development community Sep 05, 2017 CONTENTS 1 Introduction 1 1.1 Style Guidelines ............................................... 1 1.2 Getting Started ............................................... 1 1.3 Contribution Process ............................................ 2 2 DRM Internals 3 2.1 Driver Initialization ............................................. 3 2.2 Open/Close, File Operations and IOCTLs ................................ 14 2.3 Misc Utilities ................................................. 21 2.4 Legacy Support Code ........................................... 22 3 DRM Memory Management 23 3.1 The Translation Table Manager (TTM) .................................. 23 3.2 The Graphics Execution Manager (GEM) ................................ 24 3.3 VMA Offset Manager ............................................ 38 3.4 PRIME Buffer Sharing ............................................ 44 3.5 DRM MM Range Allocator ......................................... 47 3.6 DRM Cache Handling ............................................ 56 3.7 DRM Sync Objects ............................................. 56 4 Kernel Mode Setting (KMS) 59 4.1 Overview ................................................... 59 4.2 KMS Core Structures and Functions ................................... 62 4.3 Modeset Base Object Abstraction .................................... 70 4.4 Atomic Mode Setting ............................................ 73 4.5 CRTC Abstraction .............................................. 91 4.6 Frame Buffer Abstraction ......................................... 102 4.7 DRM Format Handling ........................................... 107 4.8 Dumb Buffer Objects ............................................ 109 4.9 Plane Abstraction .............................................. 110 4.10 Display Modes Function Reference ................................... 118 4.11 Connector Abstraction ........................................... 130 4.12 Encoder Abstraction ............................................ 146 4.13 KMS Initialization and Cleanup ...................................... 149 4.14 KMS Locking ................................................. 151 4.15 KMS Properties ............................................... 154 4.16 Vertical Blanking .............................................. 174 5 Mode Setting Helper Functions 183 5.1 Modeset Helper Reference for Common Vtables ........................... 183 5.2 Atomic Modeset Helper Functions Reference ............................. 196 5.3 Legacy CRTC/Modeset Helper Functions Reference .......................... 214 5.4 Simple KMS Helper Reference ...................................... 217 5.5 fbdev Helper Functions Reference .................................... 219 5.6 Framebuffer CMA Helper Functions Reference ............................. 229 i 5.7 Bridges .................................................... 232 5.8 Panel Helper Reference .......................................... 238 5.9 Display Port Helper Functions Reference ................................ 242 5.10 Display Port Dual Mode Adaptor Helper Functions Reference .................... 247 5.11 Display Port MST Helper Functions Reference ............................. 250 5.12 MIPI DSI Helper Functions Reference .................................. 258 5.13 Output Probing Helper Functions Reference .............................. 267 5.14 EDID Helper Functions Reference .................................... 270 5.15 SCDC Helper Functions Reference .................................... 277 5.16 Rectangle Utilities Reference ....................................... 279 5.17 HDMI Infoframes Helper Reference ................................... 284 5.18 Flip-work Helper Reference ........................................ 287 5.19 Plane Helper Reference .......................................... 289 5.20 Auxiliary Modeset Helpers ......................................... 292 6 Userland interfaces 295 6.1 libdrm Device Lookup ........................................... 295 6.2 Primary Nodes, DRM Master and Authentication ........................... 296 6.3 Open-Source Userspace Requirements ................................. 297 6.4 Render nodes ................................................ 298 6.5 IOCTL Support on Device Nodes ..................................... 299 6.6 Testing and validation ........................................... 302 6.7 Sysfs Support ................................................ 305 6.8 VBlank event handling ........................................... 305 7 drm/i915 Intel GFX Driver 307 7.1 Core Driver Infrastructure ......................................... 307 7.2 Display Hardware Handling ........................................ 316 7.3 Memory Management and Command Submission .......................... 343 7.4 GuC ...................................................... 355 7.5 Tracing .................................................... 358 7.6 Perf ...................................................... 359 8 drm/meson AmLogic Meson Video Processing Unit 379 8.1 Video Processing Unit ........................................... 379 8.2 Video Input Unit ............................................... 379 8.3 Video Post Processing ........................................... 380 8.4 Video Encoder ................................................ 380 8.5 Video Canvas Management ........................................ 381 8.6 Video Clocks ................................................. 381 8.7 HDMI Video Output ............................................. 381 9 drm/pl111 ARM PrimeCell PL111 CLCD Driver 383 10drm/tegra NVIDIA Tegra GPU and display driver 385 10.1 Driver Infrastructure ............................................ 385 10.2 KMS driver .................................................. 389 10.3 Userspace Interface ............................................ 391 11drm/tinydrm Driver library 393 11.1 Core functionality .............................................. 393 11.2 Additional helpers ............................................. 396 11.3 MIPI DBI Compatible Controllers ..................................... 399 12drm/vc4 Broadcom VC4 Graphics Driver 405 12.1 Display Hardware Handling ........................................ 405 12.2 Memory Management and 3D Command Submission ........................ 406 13VGA Switcheroo 409 ii 13.1 Modes of Use ................................................ 409 13.2 API ....................................................... 410 13.3 Handlers ................................................... 417 14VGA Arbiter 421 14.1 vgaarb kernel/userspace ABI ....................................... 421 14.2 In-kernel interface ............................................. 422 14.3 libpciaccess ................................................. 424 14.4 xf86VGAArbiter (X server implementation) .............................. 425 14.5 References .................................................. 425 15drm/bridge/dw-hdmi Synopsys DesignWare HDMI Controller 427 15.1 Synopsys DesignWare HDMI Controller ................................. 427 16TODO list 429 16.1 Subsystem-wide refactorings ....................................... 429 16.2 Core refactorings .............................................. 431 16.3 Better Testing ................................................ 433 16.4 Driver Specific ................................................ 434 16.5 Outside DRM ................................................. 434 Index 435 iii iv CHAPTER ONE INTRODUCTION The Linux DRM layer contains code intended to support the needs of complex graphics devices, usually containing programmable pipelines well suited to 3D graphics acceleration. Graphics drivers in the kernel may make use of DRM functions to make tasks like memory management, interrupt handling and DMA easier, and provide a uniform interface to applications. A note on versions: this guide covers features found in the DRM tree, including the TTM memory manager, output configuration and mode setting, and the new vblank internals, in addition to all the regular features found in current kernels. [Insert diagram of typical DRM stack here] 1.1 Style Guidelines For consistency this documentation uses American English. Abbreviations are written as all-uppercase, for example: DRM, KMS, IOCTL, CRTC, and so on. To aid in reading, documentations make full use of the markup characters kerneldoc provides: @parameter for function parameters, @member for structure members (within the same structure), &struct structure to reference structures and function() for func- tions. These all get automatically hyperlinked if kerneldoc for the referenced objects exists. When ref- erencing entries in function vtables (and structure members in general) please use &vtable_name.vfunc. Unfortunately this does not yet yield a direct link to the member, only the structure. Except in special situations (to separate locked from unlocked variants) locking requirements for func- tions aren’t documented in the kerneldoc. Instead locking should be check at runtime using e.g. WARN_ON(!mutex_is_locked(...));. Since it’s much easier to ignore documentation than runtime noise this provides more value. And on top of that runtime checks do need to be updated when the locking rules change, increasing the chances that they’re correct. Within the documentation the locking rules should be explained in the relevant structures: Either in the comment for the lock explaining what it protects,
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages452 Page
-
File Size-