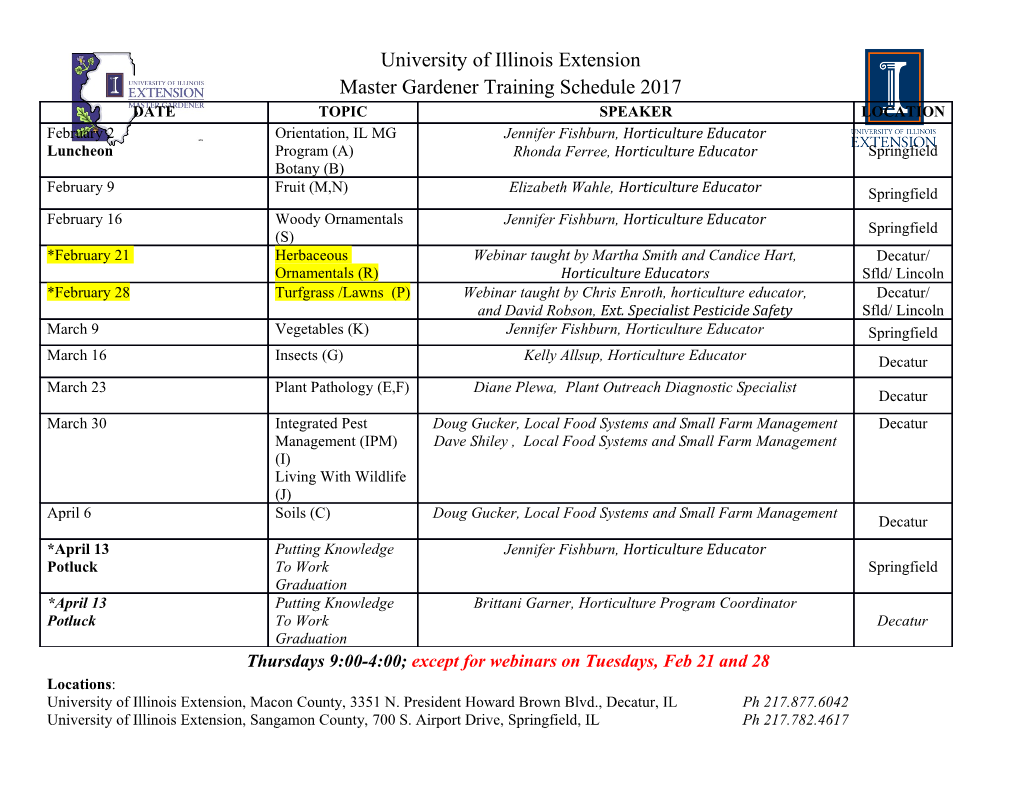
Mathematical recipes (early draft) Dennis Yurichev <[email protected]> June 29, 2021 Contents 1 Unsorted parts 1 1.1 Fencepost error / off-by-one error .......................................... 1 1.2 GCD and LCM ..................................................... 2 1.2.1 GCD ...................................................... 2 1.2.2 Oculus VR Flicks and GCD .......................................... 3 1.2.3 LCM ...................................................... 4 1.3 Signed numbers: two’s complement ........................................ 5 1.3.1 Couple of additions about two’s complement form ............................ 7 1.3.2 -1 ....................................................... 8 1.4 Mandelbrot set .................................................... 8 1.4.1 A word about complex numbers ...................................... 8 1.4.2 How to draw the Mandelbrot set ...................................... 8 2 Boolean algebra 14 2.1 XOR (exclusive OR) .................................................. 14 2.1.1 Logical difference .............................................. 14 2.1.2 Everyday speech ............................................... 14 2.1.3 Encryption .................................................. 14 2.1.4 RAID14 .................................................... 14 2.1.5 XOR swap algorithm ............................................. 15 2.1.6 XOR linked list ................................................ 15 2.1.7 Switching value trick ............................................. 16 2.1.8 Zobrist hashing / tabulation hashing .................................... 16 2.1.9 By the way .................................................. 17 2.2 AND operation .................................................... 17 2.2.1 Checking if a value is on 2n boundary .................................... 17 2.2.2 KOI-8R Cyrillic encoding ........................................... 17 2.3 AND and OR as subtraction and addition ...................................... 18 2.3.1 ZX Spectrum ROM text strings ........................................ 18 2.4 Hamming weight / population count ........................................ 21 3 Set theory 22 3.1 Set theory explanation via boolean operations ................................... 22 3.1.1 Collecting TV series ............................................. 22 3.1.2 Rock band .................................................. 22 3.1.3 Languages .................................................. 23 3.1.4 De Morgan’s laws ............................................... 24 3.1.5 Powerset ................................................... 24 3.1.6 Inclusion-exclusion principle ........................................ 24 4 IEEE 754 27 4.1 IEEE 754 round-off errors ............................................... 27 4.1.1 The example from Wikipedia ........................................ 27 4.1.2 Conversion from double-precision to single-precision and back ..................... 31 4.1.3 The moral of the story ............................................ 35 4.1.4 All the files .................................................. 36 1Redundant Array of Independent Disks 1 2 5 Calculus 37 5.1 What is derivative? .................................................. 37 5.1.1 Square .................................................... 37 5.1.2 Cube ..................................................... 37 5.1.3 Line ...................................................... 38 5.1.4 Circle ..................................................... 38 5.1.5 Cylinder .................................................... 39 5.1.6 Sphere .................................................... 39 5.1.7 Conclusion .................................................. 40 6 Prime numbers 41 6.1 Integer factorization ................................................. 41 6.1.1 Using composite number as a container .................................. 42 6.1.2 Using composite number as a container (another example) ........................ 43 6.2 Coprime numbers .................................................. 44 6.3 Semiprime numbers ................................................. 45 6.4 How RSA2 works ................................................... 45 6.4.1 Fermat little theorem ............................................ 45 6.4.2 Euler’s totient function ........................................... 46 6.4.3 Euler’s theorem ............................................... 46 6.4.4 RSA example ................................................. 46 6.4.5 So how it works? ............................................... 47 6.4.6 Breaking RSA ................................................. 48 6.4.7 The difference between my simplified example and a real RSA algorithm ................ 48 6.4.8 The RSA signature .............................................. 49 6.4.9 Hybrid cryptosystem ............................................. 49 7 Modulo arithmetics 50 7.1 Quick introduction into modular arithmetic .................................... 50 7.1.1 Modular arithmetic on CPUs ......................................... 51 7.1.2 Getting random numbers .......................................... 52 7.1.3 Reverse Engineering ............................................. 53 7.2 Remainder of division ................................................ 53 7.2.1 Remainder of division by modulo 2n .................................... 53 7.3 Modulo inverse, part I ................................................ 54 7.3.1 No remainder? ................................................ 55 7.4 Modulo inverse, part II ................................................ 55 7.5 Reversible linear congruential generator ...................................... 57 7.6 Getting magic number using extended Euclidean algorithm ............................ 58 8 Probability 60 8.1 Text strings right in the middle of compressed data ................................ 60 8.2 Autocomplete using Markov chains ......................................... 62 8.2.1 Dissociated press .............................................. 62 8.2.2 Autocomplete ................................................ 66 8.2.3 Further work ................................................. 73 8.2.4 The files .................................................... 73 8.2.5 Read more .................................................. 73 8.2.6 Another thought-provoking example .................................... 73 8.3 random.choices() in Python 3 ............................................ 76 9 Combinatorics 78 9.1 Soldering a headphones cable ............................................ 78 9.2 Vehicle license plate ................................................. 79 9.3 Forgotten password ................................................. 79 9.4 Executable file watermarking/steganography using Lehmer code and factorial number system ......... 85 9.5 De Bruijn sequences; leading/trailing zero bits counting .............................. 89 9.5.1 Introduction ................................................. 89 9.5.2 Trailing zero bits counting .......................................... 90 9.5.3 Leading zero bits counting .......................................... 93 2Rivest–Shamir–Adleman cryptosystem If you noticed a typo, error or have any suggestions, do not hesitate to drop me a note: <[email protected]>. Thanks! 3 9.5.4 Performance ................................................. 94 9.5.5 Applications ................................................. 94 9.5.6 Generation of De Bruijn sequences ..................................... 94 9.5.7 Other articles ................................................. 94 10 Galois Fields, GF(2) and yet another explanation of CRC3 95 10.1 What is wrong with checksum? ........................................... 95 10.2 Division by prime ................................................... 95 10.3 (Binary) long divison ................................................. 95 10.4 (Binary) long division, version 2 ........................................... 97 10.5 Shortest possible introduction into GF(2) ...................................... 98 10.6 CRC32 ......................................................... 99 10.7 Rationale ....................................................... 100 10.8 Further reading .................................................... 101 11 Logarithms 102 11.1 Introduction ..................................................... 102 11.1.1 Children’s approach ............................................. 102 11.1.2 Scientists’ and engineers’ approach .................................... 102 11.2 Logarithmic scale ................................................... 103 11.2.1 In human perception ............................................. 103 11.2.2 In electronics engineering .......................................... 103 11.2.3 In IT ...................................................... 105 11.2.4 Web 2.0 .................................................... 106 11.2.5 Errors of TeX ................................................. 106 11.2.6 Moore’s Law ................................................. 107 11.2.7 Money .................................................... 107 11.2.8 Sizes, values, weights... ........................................... 107 11.3 Multiplication and division using addition and subtraction ............................ 108 11.3.1 Logarithmic slide rule ............................................ 108 11.3.2 Logarithmic tables .............................................. 109 11.3.3
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages147 Page
-
File Size-