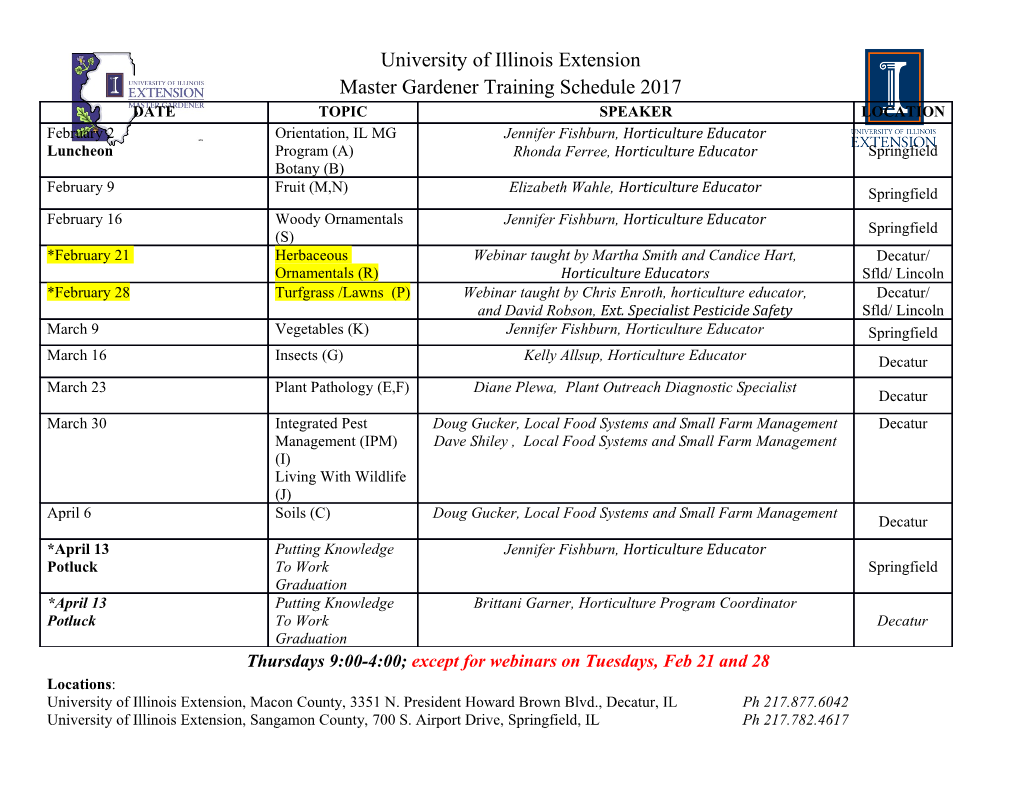
JAVA™ FOR COBOL PROGRAMMERS, THIRD EDITION JOHN C. BYRNE Charles River Media A part of Course Technology, Cengage Learning Australia, Brazil, Japan, Korea, Mexico, Singapore, Spain, United Kingdom, United States Java for COBOL Programmers, © 2009 Course Technology, a part of Cengage Learning. Third Edition ALL RIGHTS RESERVED. No part of this work covered by the copyright herein may be reproduced, transmitted, stored, or used in any form or by John C. Byrne any means graphic, electronic, or mechanical, including but not limited to photocopying, recording, scanning, digitizing, taping, Web distribution, information networks, or information storage and retrieval systems, except Publisher and General Manager, as permitted under Section 107 or 108 of the 1976 United States Copyright Course Technology PTR: Act, without the prior written permission of the publisher. Stacy L. Hiquet For product information and technology assistance, contact us at Associate Director of Marketing: Cengage Learning Customer & Sales Support, 1-800-354-9706 Sarah Panella For permission to use material from this text or product, submit all requests online at cengage.com/permissions Content Project Manager: Further permissions questions can be emailed to Jessica McNavich [email protected] Marketing Manager: Mark Hughes Sun, Sun Microsystems, the Sun logo, Java, JavaBeans, and all trademarks and logos that contain Sun, Solaris, or Java, are trademarks or registered trademarks Acquisitions Editor: Mitzi Koontz of Sun Microsystems, Inc. or its subsidiaries in the United States and other countries. Microsoft, Windows, and Internet Explorer are either registered trademarks or trademarks of Microsoft Corporation in the United States and/or Development Editor and other countries. Apache Tomcat and Tomcat are trademarks of the Apache Technical Reviewer: Arron Ferguson Software Foundation. Eclipse, Built on Eclipse, and Eclipse Ready are trademarks of Eclipse Foundation, Inc. LegacyJ is the property of LegacyJ Corp. SoftwareMining is a trademark of SoftwareMining, UK. Project Editor and Copy Editor: Kim Benbow All other trademarks are the property of their respective owners. CRM Editorial Services Coordinator: Library of Congress Control Number: 2008931028 Jen Blaney ISBN-13: 978-1-59863-565-5 Interior Layout: Jill Flores ISBN-10: 1-58450-565-6 eISBN-10: 1-58450-618-0 Cover Designer: Mike Tanamachi Course Technology 25 Thomson Place CD-ROM Producer: Brandon Penticuff Boston, MA 02210 USA Indexer: Jerilyn Sproston Cengage Learning is a leading provider of customized learning solutions with office locations around the globe, including Singapore, the United Kingdom, Proofreader: Ruth Saavedra Australia, Mexico, Brazil, and Japan. Locate your local office at: international. cengage.com/region Cengage Learning products are represented in Canada by Nelson Education, Ltd. For your lifelong learning solutions, visit courseptr.com Visit our corporate website at cengage.com Printed in the United States of America 1 2 3 4 5 6 7 11 10 09 To the memory of Charles Byrne, whose passion for learning still inspires those who knew him. About the Author John C. Byrne is the Vice President of Technology and primary system architect for a high-end enterprise software vendor and has over 20 years experience in the software industry. Contents Introduction xvi Part I Introducing Java 1 1 Objects and Classes 3 The COBOL Subroutine 4 Calling a Subroutine 4 MYSUB COBOL 5 CALLER COBOL 6 CALLER COBOL: CONTROL 7 Terms to Review: Subroutines 9 Objects and Java 10 ErrorMsg Class 11 Caller Class 12 Terms to Review: Objects 13 2 Introducing the Java Development Environment 17 Runtime Interpretation and Java Byte Codes 17 Getting Started with Java’s SDK 21 Applets with SDK 24 Classes and Filenames 26 CLASSPATH 27 v vi Contents CODEBASE 28 Packages 28 Inside a Package 29 Name Collisions 31 Packages and Filenames 32 Compressed Packages 33 Applications vs. Applets 34 Reviewing the Samples 34 3 Messages and Methods 37 MYSUB COBOL 38 CALLER COBOL 39 Messages in Java 40 ErrorMsg Class 40 Caller Class 41 Multiple Messages 41 Class ErrorMsg 41 Method Overloading 43 Caller Class 43 Method Overloading in COBOL 44 MYSUB COBOL 44 CALLER COBOL 46 Terms to Review 48 Exercises: Classes, Objects, and Methods 48 Reviewing the Samples 57 HelloWorld: The Application 61 HelloWorld: The Applet 62 ErrorMsg: The Class 63 Contents vii 4 Class Members 65 MYSUB COBOL 66 MYSUB COBOL: ACTION-SWITCH 68 Java Variables 68 ErrorMsg Class 69 Classes, Objects, and Members Review 71 Objects and COBOL 72 Using Objects in Java 76 Java Data Members 77 ErrorMsg Class 78 Caller Class 78 Local Variables 79 Primitive Data Types 80 Arrays 83 Arrays as Parameters 87 Method Members 87 ErrorMsg Class: Static Variable 88 ErrorMsg Class: Static Initializer 88 Constructors 89 Exercises: Class Members 91 Reviewing the Samples 99 5 Inheritance, Interfaces, and Polymorphism 101 Inheritance and Object-Oriented Design 101 Inheritance and Objects 103 Inheriting Methods 103 Caller Class 104 Redefining a Method 105 ErrorMsg Class 105 Caller Class 106 viii Contents Extending a Method 106 ErrorMsg Class 106 Caller Class 108 Why Inheritance? 108 Inheritance, Objects, and COBOL 109 NEWSUB COBOL 109 NEWSUB COBOL: MYSUB 110 More COBOL Object-Oriented Design Patterns 112 CALLER COBOL 114 MYSUB COBOL 116 Inheritance and Java 119 ErrorMsg Class 120 PopupErrorMsg Class 120 PrintfileErrorMsg Class 120 TextMessage Class 121 ErrorMsg Class 121 PopupErrorMsg Class 122 PrintfileErrorMsg Class 122 Consumer Class 122 TextMessage Class 123 ErrorMsg Class 123 ErrorMsg Class: Override 124 PopupErrorMsg Class 124 PrintfileErrorMsg Class 124 Consumer Class 124 PrintfileErrorMsg Class 125 Sharing Variables and Methods 126 Hiding Variables and Methods 126 The this Variable 127 Java Interfaces 128 Writeline Interface 129 Contents ix PrintfileErrorMsg Class 129 Caller Class 130 Using Interfaces 130 Hiding Methods and Members 131 Polymorphism 132 Exercises 133 Reviewing the Samples 144 Part II Java’s Syntax 147 6 Java Syntax 149 COBOL vs. Java Syntax 149 Java Statements 150 Java Comments 152 Java Operators 153 Binary Arithmetic Operations 154 Understanding Reference Variables with COBOL 159 Exercises: Java’s Syntax 160 Reviewing the Exercises 165 7 Flow Control 167 Code Block 168 The if Statement 168 The while Statement 172 The do...while Statement 174 The for Statement 175 The switch Statement 176 The break, continue Statements 177 Exercises: Flow Control 181 Reviewing the Exercises 186 x Contents 8 Strings, StringBuffers, StringBuilders, Numbers, and BigNumbers 189 Strings 189 Comparing Strings 191 Working with Strings 193 Numeric Wrapper Classes 196 StringBuffers 200 BigNumbers 202 Exercises: Strings, StringBuffers, Numbers, and BigNumbers 208 Reviewing the Exercises 216 9 Exceptions, Threads, and Garbage Collectors 219 Exception Class Hierarchy 221 Creating Exceptions 221 Using Exceptions 223 Exception-Processing Suggestions 228 Exception-Processing Summary 229 Threads 229 Inheriting from Thread 230 Implementing Runnable 232 Synchronization 233 Benefits and Cautions 235 Garbage Collection 236 Exercises: Java’s Exceptions and Threads 237 Reviewing the Exercises 240 10 I/O in Java 243 Streams vs. Record-Based I/O 244 The File Class 246 InputStream and OutputStream 249 Serialization 252 Contents xi Readers and Writers 254 RandomAccessFile 256 Exercises 258 Reviewing the Exercises 262 11 Java Collections 263 Collections Background 264 Ordered Collections: Vectors and ArrayLists 266 Keyed Collections: Hashtable and HashMap 270 Other Collections 271 Iterators 272 Ordering and Comparison Functions 273 Exercises: Java Collections 275 Reviewing the Exercises 278 12 Other Java Topics 279 Graphical User Interface Development 279 Properties Files 281 Java Utilities 282 Jar Utility 282 Javadoc Utility 284 Exercises 287 Reviewing the Exercises 289 Part III Introducing Enterprise Java 291 13 Java Database Connectivity 293 How JDBC Works 294 Connecting to the Database 296 Querying a Table 298 xii Contents Inserting, Updating, and Deleting 300 Configuring the JDBC-ODBC Bridge 301 Exercises 302 Reviewing the Exercises 304 14 Servlets and Java Server Pages 305 Browsers and Web Servers 306 The Servlet as Transaction Processor 308 Servlet Protocol 310 Java Server Pages 313 Getting Started with Servlets and JSPs 316 Exercises 317 Reviewing the Exercises 320 15 Introduction to Enterprise JavaBeans 321 Distributed Computing 322 The Different Kinds of EJBs 323 Container Services 324 The Interfaces and the Implementation Class 325 Accessing the Bean from the Client 327 Exercises 327 Reviewing the Exercises 328 16 Introduction to XML 329 The Basics 330 XML vs. HTML 330 Document Type Definitions (DTDs) 332 DTD Components 333 Elements 334 Attributes 335 Entities 336 Contents xiii XML Declaration 337 A Complete XML Document 338 XML Schemas 339 Authoring XML Documents 339 XML and Java 339 XML and HTML 340 Where to Use XML 341 Electronic Data Interchange (EDI) 342 Online XML or Web Services 343 XML and OAG 344 Other Opportunities 351 Exercises 352 Reviewing the Exercises 354 17 Introducing Eclipse 355 Installing Eclipse 356 Start Using Eclipse 356 Make a New Eclipse Project 358 Run with Eclipse 362 Debug with Eclipse 362 Refactoring with Eclipse 363 Part IV Appendixes 369 Appendix A: About the CD-ROM 371 Exercises 371 Java SDK 371 LegacyJ 372 Eclipse 372 Tomcat 372 xiv Contents SoftwareMining 373 Case Studies 373 Appendix B: Java Information Available Elsewhere 375 Java Resources 375 Java
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages432 Page
-
File Size-