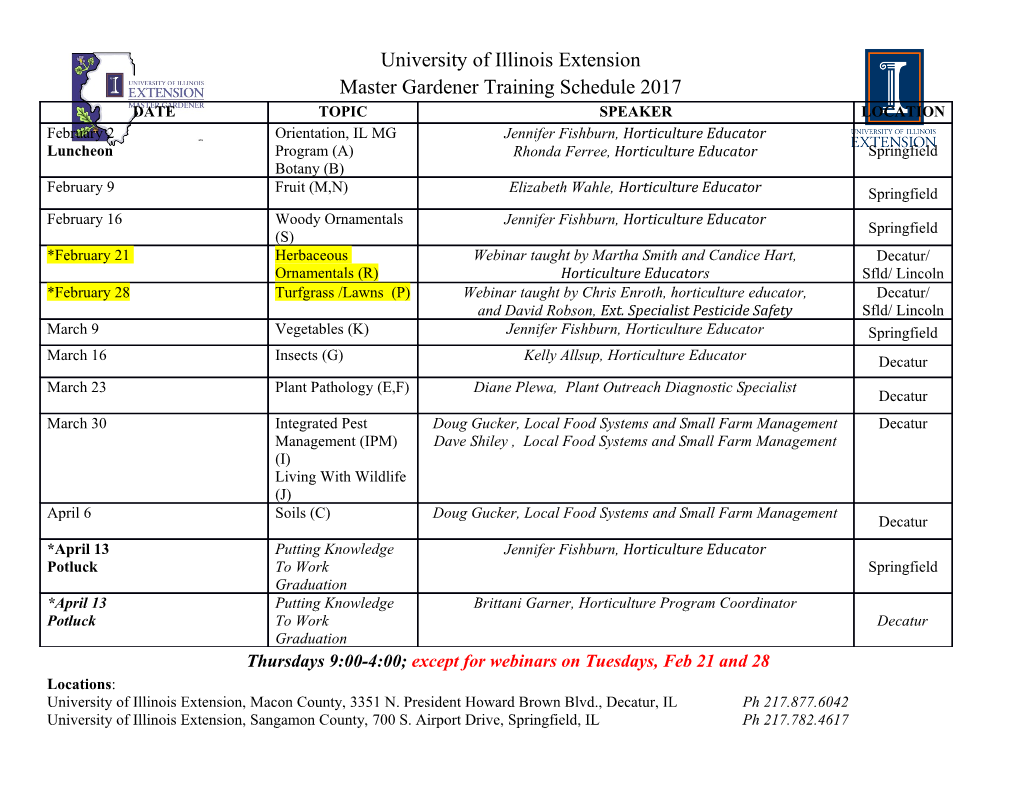
xford hysics x V1 x V2 V1 V3 V2 handbook of the V3 PHYSICS PRACTICAL COURSE 2010–2011 PHYSICS C PROGRAMMING COURSE UNIVERSITY OF OXFORD PHYSICS TEACHING FACULTY 2 Contents 1 Introduction 7 1.1 The Computing Course and Laboratory . 7 1.2 The C course . 7 1.3 The marking system and use of your logbook . 7 1.4 Hilary term . 8 1.5 The C language . 8 1.6 Typographical conventions . 9 2 Learning to use the system 11 2.1 Logging in and getting started . 11 2.2 Applications you will be using . 11 2.3 The C development environment . 12 2.3.1 Creating a new project . 12 2.3.2 Compiling, running and debugging your program . 13 2.3.3 Opening and closing existing programs . 15 2.4 Terminal and the Unix command line . 15 2.4.1 Creating and manipulating directories . 15 2.4.2 Manipulating files . 16 2.4.3 Editing, compiling and running programs from the Unix shell . 16 2.4.4 Command line compilation on the Mac using xcodebuild . 16 2.4.5 Interaction between the command line and graphical environments . 17 2.5 Searching with Spotlight . 17 2.6 Logging out . 17 3 The elements of C 19 3.1 Program Structure . 19 3.1.1 Hello world . 19 3.1.2 Code layout and style . 20 3.2 Variables . 21 3.2.1 Types . 21 3.2.2 Declaration and Names . 22 3.2.3 Assignment . 22 3.3 Output using printf() . 24 3.4 Input using scanf() . 26 3.4.1 Reading a single character . 28 3.5 Arithmetic Expressions and Operators . 28 3.5.1 Type conversions and casts . 29 3 3.5.2 Some other operators . 30 3.6 Expressions, statements, and compound statements . 31 3.7 if statements . 32 3.7.1 Relational operators . 32 3.7.2 Logical operators and else-ifs . 33 3.8 Loops and Iteration . 34 3.8.1 for loops . 34 3.8.2 while loops . 35 3.8.3 do...while loops . 36 3.9 Mathematical functions . 37 3.10 Arrays . 38 3.10.1 Declaration . 39 3.10.2 Array elements . 39 3.10.3 Initialisation . 39 3.11 Functions . 40 3.12 File I/O . 42 3.12.1 Writing to Files . 44 3.12.2 Reading from Files . 44 4 Further C 47 4.1 Random Numbers . 47 4.2 Complex numbers . 48 4.3 Multi-dimensional Arrays . 49 4.4 String manipulation functions . 50 4.5 switch statements . 52 4.6 The ternary operator . 54 4.7 More about variables . 54 4.7.1 Scope . 54 4.7.2 Global Variables . 55 4.7.3 Storage classes . 56 4.8 The C Preprocessor . 56 4.9 Data structures . 57 4.9.1 union . 58 4.10 Enumerated types . 59 4.11 Pointers . 59 4.11.1 What is a pointer? . 59 4.11.2 Pointers and arrays . 60 4.11.3 Pointers and functions . 60 4.11.4 Command line input . 61 4.12 Custom variable types with typedef . 61 4.13 Variable–argument fuctions . 62 4.13.1 Introduction to variable–argument functions . 62 4.13.2 Declaring a variable–argument function . 62 4.13.3 Accessing the varargs list . 62 A Reserved Words and Program Filenames 65 4 Contents B Creating Larger Projects 67 B.1 Header Files . 67 B.2 Makefiles . 68 C Debugging Code 69 C.1 Understand Your Code . 69 C.2 Debuggers . 69 C.2.1 Getting GDB . 69 C.2.2 Using GDB . 69 C.2.3 Using GDB within Xcode . 71 C.3 Profiling and code optimisation . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages75 Page
-
File Size-