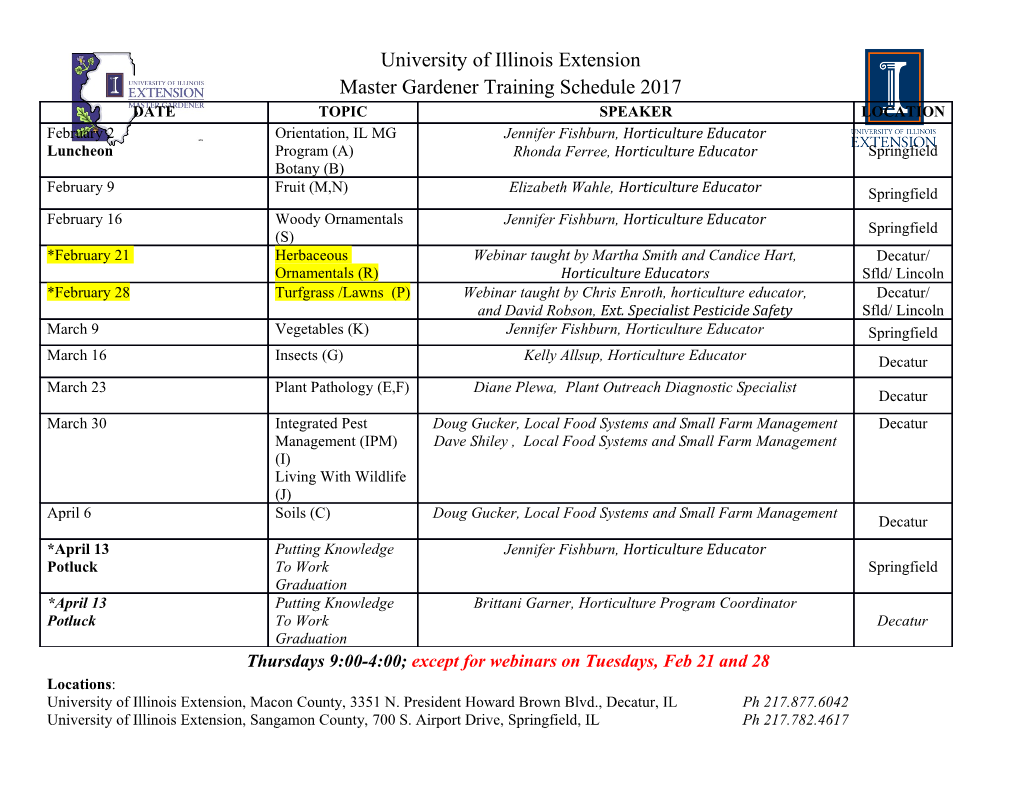
MEAP Edition Manning Early Access Program Copyright 2009 Manning Publications For more information on this and other Manning titles go to www.manning.com Licensed to Alison Tyler <[email protected]> Table of Contents 1. Introduction ................................................................................................................... 1 The JavaScript Language ............................................................................................. 2 Writing Cross-Browser Code ........................................................................................ 3 Best Practices ............................................................................................................ 5 Summary .................................................................................................................. 6 2. Testing and Debugging .................................................................................................... 7 Debugging Code ........................................................................................................ 7 Test Generation .......................................................................................................... 8 Building a Test Suite ................................................................................................. 10 Asynchronous Testing ....................................................................................... 12 Summary ................................................................................................................. 13 3. Functions ..................................................................................................................... 14 Function Definition ................................................................................................... 14 Anonymous Functions and Recursion ........................................................................... 15 Functions as Objects ................................................................................................. 17 Storing Functions .............................................................................................. 18 Self-Memoizing Functions .................................................................................. 18 Context ................................................................................................................... 20 Looping .......................................................................................................... 21 Fake Array Methods .......................................................................................... 22 Variable Arguments .................................................................................................. 22 Min/Max Number in an Array ............................................................................. 23 Function Overloading ........................................................................................ 23 Function Length ............................................................................................... 24 Function Type .......................................................................................................... 26 Summary ................................................................................................................. 27 4. Closures ...................................................................................................................... 28 How closures work ................................................................................................... 28 Private Variables .............................................................................................. 29 Callbacks and Timers ........................................................................................ 29 Enforcing Function Context ........................................................................................ 30 Partially Applying Functions ....................................................................................... 32 Overriding Function Behavior ..................................................................................... 34 Memoization .................................................................................................... 34 Function Wrapping ............................................................................................ 36 (function(){})() ......................................................................................................... 37 Temporary Scope and Private Variables ................................................................ 37 Loops ............................................................................................................. 39 Library Wrapping ............................................................................................. 39 Summary ................................................................................................................. 40 5. Function Prototypes ....................................................................................................... 42 Instantiation and Prototypes ........................................................................................ 42 Instantiation ..................................................................................................... 42 Object Type ..................................................................................................... 44 Inheritance and the Prototype Chain ..................................................................... 44 HTML Prototypes ............................................................................................. 46 Gotchas ................................................................................................................... 47 Extending Object .............................................................................................. 47 Extending Number ............................................................................................ 48 Sub-classing Native Objects ................................................................................ 49 Instantiation ..................................................................................................... 50 iii Licensed to Alison Tyler <[email protected]> Secrets of the JavaScript Ninja Class-like Code ........................................................................................................ 51 Summary ................................................................................................................. 55 6. Timers ......................................................................................................................... 56 How Timers Work .................................................................................................... 56 Minimum Timer Delay and Reliability ......................................................................... 58 Computationally-Expensive Processing ......................................................................... 60 Central Timer Control ............................................................................................... 63 Asynchronous Testing ............................................................................................... 65 Summary ................................................................................................................. 66 7. Regular Expressions ...................................................................................................... 67 Compiling ............................................................................................................... 67 Capturing ................................................................................................................ 69 References to Captures ...................................................................................... 71 Non-capturing Groups ....................................................................................... 71 Replacing with Functions ........................................................................................... 72 Common Problems .................................................................................................... 73 Trimming a String ............................................................................................ 73 Matching Endlines ............................................................................................ 74 Unicode .......................................................................................................... 75 Escaped Characters ........................................................................................... 75 Summary ................................................................................................................. 75 8. With Statements ............................................................................................................ 77 Convenience ............................................................................................................ 79 Importing Namespaced Code ...................................................................................... 81 Clarified Object-Oriented Code ................................................................................... 81 Testing .................................................................................................................... 82 Templating .............................................................................................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages148 Page
-
File Size-