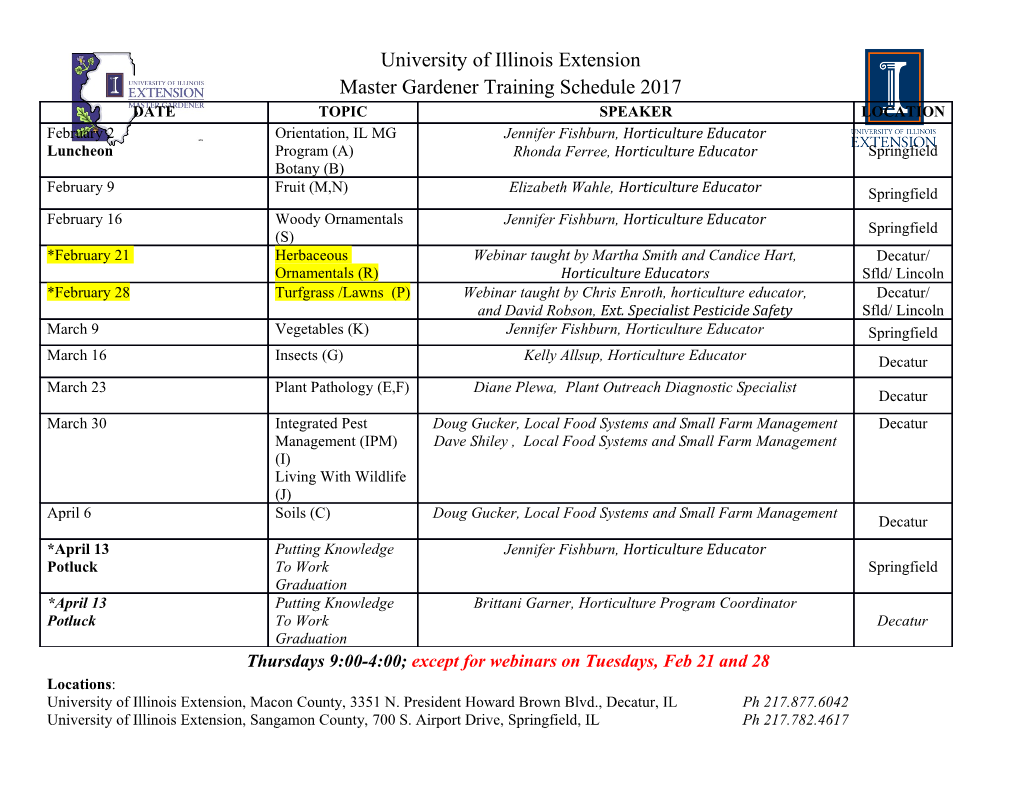
Bug Catching: Automated Program Verification 15414/15614 Spring 2020 Lecture 1: Introduction Matt Fredrikson January 14, 2020 Matt Fredrikson Model Checking 1 / 22 Course Staff Matt Fredrikson Di Wang Ryan Chen Instructor TA TA Matt Fredrikson Model Checking 2 / 22 I “The Heartbleed bug allows anyone on the Internet to read the memory of the systems protected by the vulnerable versions of the OpenSSL software.” I “...this allows attackers to eavesdrop on communications, steal data directly from the services and users and to impersonate services and users.” Bad code I April, 2014 OpenSSL announced critical vulnerability in their implementation of the Heartbeat Extension. Matt Fredrikson Model Checking 3 / 22 I “...this allows attackers to eavesdrop on communications, steal data directly from the services and users and to impersonate services and users.” Bad code I April, 2014 OpenSSL announced critical vulnerability in their implementation of the Heartbeat Extension. I “The Heartbleed bug allows anyone on the Internet to read the memory of the systems protected by the vulnerable versions of the OpenSSL software.” Matt Fredrikson Model Checking 3 / 22 Bad code I April, 2014 OpenSSL announced critical vulnerability in their implementation of the Heartbeat Extension. I “The Heartbleed bug allows anyone on the Internet to read the memory of the systems protected by the vulnerable versions of the OpenSSL software.” I “...this allows attackers to eavesdrop on communications, steal data directly from the services and users and to impersonate services and users.” Matt Fredrikson Model Checking 3 / 22 Heartbleed, explained Image source: Randall Munroe, xkcd.com Matt Fredrikson Model Checking 4 / 22 Heartbleed, explained Image source: Randall Munroe, xkcd.com Matt Fredrikson Model Checking 4 / 22 Heartbleed, explained Image source: Randall Munroe, xkcd.com Matt Fredrikson Model Checking 4 / 22 Heartbleed, explained Image source: Randall Munroe, xkcd.com Matt Fredrikson Model Checking 4 / 22 Heartbleed, explained Image source: Randall Munroe, xkcd.com Matt Fredrikson Model Checking 4 / 22 Heartbleed, explained Image source: Randall Munroe, xkcd.com Matt Fredrikson Model Checking 4 / 22 Algorithms vs. code 1 int binarySearch(int key, int[] a, int n) { 2 int low = 0; 3 int high = n; 4 5 while (low < high) { 6 int mid = (low + high) / 2; 7 8 if(a[mid] == key) return mid; // key found 9 else if(a[mid] < key) { 10 low = mid + 1; 11 } else { 12 high = mid; 13 } 14 } 15 return -1; // key not found. 16 } Matt Fredrikson Model Checking 5 / 22 But what if low + high > 231 − 1? Then mid = (low + high)/ 2 becomes negative I Best case: ArrayIndexOutOfBoundsException I Worst case: undefined behavior Algorithm may be correct. But we run code, not algorithms. Code Matters This is a correct binary search algorithm. Matt Fredrikson Model Checking 6 / 22 Then mid = (low + high)/ 2 becomes negative I Best case: ArrayIndexOutOfBoundsException I Worst case: undefined behavior Algorithm may be correct. But we run code, not algorithms. Code Matters This is a correct binary search algorithm. But what if low + high > 231 − 1? Matt Fredrikson Model Checking 6 / 22 I Best case: ArrayIndexOutOfBoundsException I Worst case: undefined behavior Algorithm may be correct. But we run code, not algorithms. Code Matters This is a correct binary search algorithm. But what if low + high > 231 − 1? Then mid = (low + high)/ 2 becomes negative Matt Fredrikson Model Checking 6 / 22 I Worst case: undefined behavior Algorithm may be correct. But we run code, not algorithms. Code Matters This is a correct binary search algorithm. But what if low + high > 231 − 1? Then mid = (low + high)/ 2 becomes negative I Best case: ArrayIndexOutOfBoundsException Matt Fredrikson Model Checking 6 / 22 Algorithm may be correct. But we run code, not algorithms. Code Matters This is a correct binary search algorithm. But what if low + high > 231 − 1? Then mid = (low + high)/ 2 becomes negative I Best case: ArrayIndexOutOfBoundsException I Worst case: undefined behavior Matt Fredrikson Model Checking 6 / 22 Code Matters This is a correct binary search algorithm. But what if low + high > 231 − 1? Then mid = (low + high)/ 2 becomes negative I Best case: ArrayIndexOutOfBoundsException I Worst case: undefined behavior Algorithm may be correct. But we run code, not algorithms. Matt Fredrikson Model Checking 6 / 22 Need to make sure we don’t overflow at any point Solution: mid = low + (high - low)/2 How do we fix it? The culprit: mid = (low + high) / 2 Matt Fredrikson Model Checking 7 / 22 Solution: mid = low + (high - low)/2 How do we fix it? The culprit: mid = (low + high) / 2 Need to make sure we don’t overflow at any point Matt Fredrikson Model Checking 7 / 22 How do we fix it? The culprit: mid = (low + high) / 2 Need to make sure we don’t overflow at any point Solution: mid = low + (high - low)/2 Matt Fredrikson Model Checking 7 / 22 The fix 1 int binarySearch(int key, int[] a, int n) { 2 int low = 0; 3 int high = n; 4 5 while (low < high) { 6 int mid = low + (high - low) / 2; 7 8 if(a[mid] == key) return mid; // key found 9 else if(a[mid] < key) { 10 low = mid + 1; 11 } else { 12 high = mid; 13 } 14 } 15 return -1; // key not found. 16 } Matt Fredrikson Model Checking 8 / 22 The fix 1 int binarySearch(int key, int[] a, int n) 2 //@requires 0 <= n && n <= \length(A); 3 { 4 int low = 0; 5 int high = n; 6 7 while (low < high) { 8 int mid = low + (high - low) / 2; 9 10 if(a[mid] == key) return mid; // key found 11 else if(a[mid] < key) { 12 low = mid + 1; 13 } else { 14 high = mid; 15 } 16 } 17 return -1; // key not found. 18 } Matt Fredrikson Model Checking 8 / 22 The fix 1 int binarySearch(int key, int[] a, int n) 2 //@requires 0 <= n && n <= \length(a); 3 /*@ensures (\result == -1 && !is_in(key, A, 0, n)) 4 @ || (0 <= \result , \result < n 5 @ && A[\result] == key); @*/ 6 { 7 int low = 0; 8 int high = n; 9 10 while (low < high) { 11 int mid = low + (high - low) / 2; 12 13 if(a[mid] == key) return mid; // key found 14 else if(a[mid] < key) { 15 low = mid + 1; 16 } else { 17 high = mid; 18 } 19 } 20 return -1; // key not found. 21 } Matt Fredrikson Model Checking 8 / 22 The fix 1 int binarySearch(int key, int[] a, int n) 2 //@requires 0 <= n && n <= \length(a); 3 //@requires is_sorted(a, 0, n); 4 /*@ensures (\result == -1 && !is_in(key, A, 0, n)) 5 @ || (0 <= \result , \result < n 6 @ && A[\result] == key); @*/ 7 { 8 int low = 0; 9 int high = n; 10 11 while (low < high) { 12 int mid = low + (high - low) / 2; 13 14 if(a[mid] == key) return mid; // key found 15 else if(a[mid] < key) { 16 low = mid + 1; 17 } else { 18 high = mid; 19 } 20 } 21 return -1; // key not found. 22 } Matt Fredrikson Model Checking 8 / 22 One solution: testing I Probably incomplete −! uncertain answer I Exhaustive testing not feasible Another: code review I Correctness definitely important, but not the only thing I Humans are fallable, bugs are subtle I What’s the specification? Better: prove correctness Specification () Implementation I Specification must be precise I Meaning of code must be well-defined I Reasoning must be sound How do we know if it’s correct? Matt Fredrikson Model Checking 9 / 22 I Probably incomplete −! uncertain answer I Exhaustive testing not feasible Another: code review I Correctness definitely important, but not the only thing I Humans are fallable, bugs are subtle I What’s the specification? Better: prove correctness Specification () Implementation I Specification must be precise I Meaning of code must be well-defined I Reasoning must be sound How do we know if it’s correct? One solution: testing Matt Fredrikson Model Checking 9 / 22 Another: code review I Correctness definitely important, but not the only thing I Humans are fallable, bugs are subtle I What’s the specification? Better: prove correctness Specification () Implementation I Specification must be precise I Meaning of code must be well-defined I Reasoning must be sound How do we know if it’s correct? One solution: testing I Probably incomplete −! uncertain answer I Exhaustive testing not feasible Matt Fredrikson Model Checking 9 / 22 I Correctness definitely important, but not the only thing I Humans are fallable, bugs are subtle I What’s the specification? Better: prove correctness Specification () Implementation I Specification must be precise I Meaning of code must be well-defined I Reasoning must be sound How do we know if it’s correct? One solution: testing I Probably incomplete −! uncertain answer I Exhaustive testing not feasible Another: code review Matt Fredrikson Model Checking 9 / 22 Better: prove correctness Specification () Implementation I Specification must be precise I Meaning of code must be well-defined I Reasoning must be sound How do we know if it’s correct? One solution: testing I Probably incomplete −! uncertain answer I Exhaustive testing not feasible Another: code review I Correctness definitely important, but not the only thing I Humans are fallable, bugs are subtle I What’s the specification? Matt Fredrikson Model Checking 9 / 22 I Specification must be precise I Meaning of code must be well-defined I Reasoning must be sound How do we know if it’s correct? One solution: testing I Probably incomplete −! uncertain answer I Exhaustive testing not feasible Another: code review I Correctness definitely important, but not the only thing I Humans are fallable, bugs are subtle I What’s the specification? Better: prove correctness Specification () Implementation Matt Fredrikson Model Checking 9 / 22 How do we know if it’s correct? One solution: testing I Probably incomplete
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages61 Page
-
File Size-