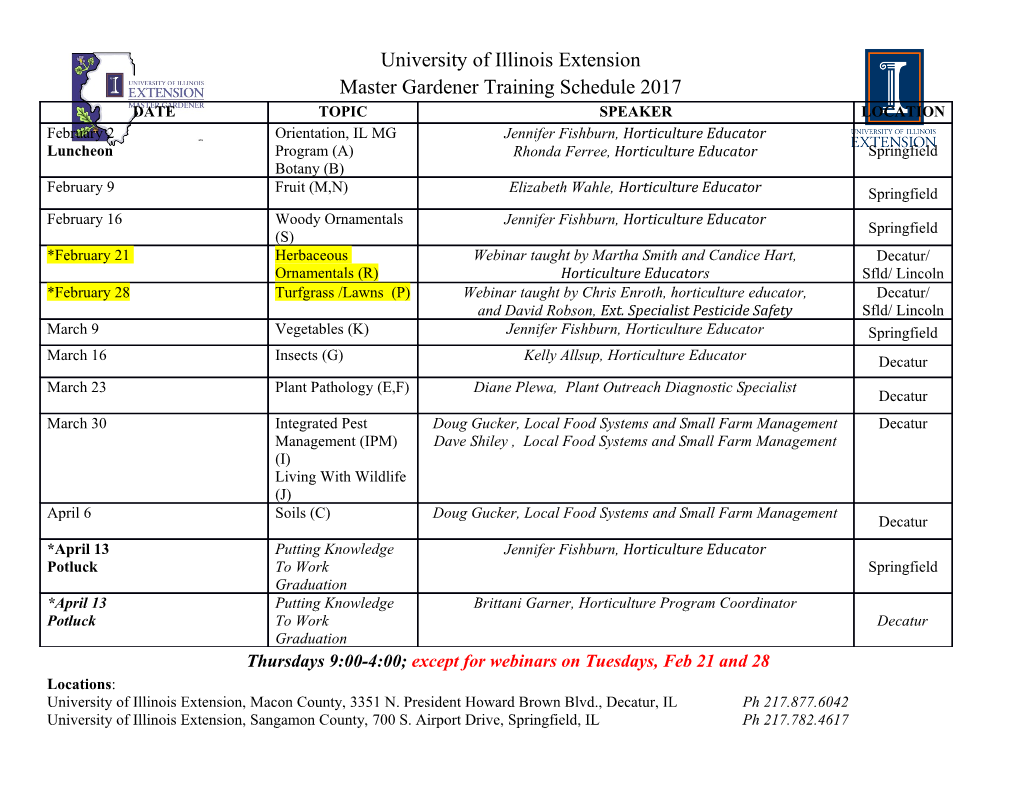
Debugging a Program With dbx Sun™ Studio 11 Sun Microsystems, Inc. www.sun.com Part No 819-3683-10 November 2005, Revision A Submit comments about this document at: http://www.sun.com/hwdocs/feedback Copyright © 2005 Sun Microsystems, Inc., 4150 Network Circle, Santa Clara, California 95054, U.S.A. All rights reserved. U.S. Government Rights - Commercial software. Government users are subject to the Sun Microsystems, Inc. standard license agreement and applicable provisions of the FAR and its supplements. Use is subject to license terms. This distribution may include materials developed by third parties. Parts of the product may be derived from Berkeley BSD systems, licensed from the University of California. UNIX is a registered trademark in the U.S. and in other countries, exclusively licensed through X/Open Company, Ltd. Sun, Sun Microsystems, the Sun logo, Java, and JavaHelp are trademarks or registered trademarks of Sun Microsystems, Inc. in the U.S. and other countries.All SPARC trademarks are used under license and are trademarks or registered trademarks of SPARC International, Inc. in the U.S. and other countries. Products bearing SPARC trademarks are based upon architecture developed by Sun Microsystems, Inc. This product is covered and controlled by U.S. Export Control laws and may be subject to the export or import laws in other countries. Nuclear, missile, chemical biological weapons or nuclear maritime end uses or end users, whether direct or indirect, are strictly prohibited. Export or reexport to countries subject to U.S. embargo or to entities identified on U.S. export exclusion lists, including, but not limited to, the denied persons and specially designated nationals lists is strictly prohibited. DOCUMENTATION IS PROVIDED "AS IS" AND ALL EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE DISCLAIMED, EXCEPT TO THE EXTENT THAT SUCH DISCLAIMERS ARE HELD TO BE LEGALLY INVALID. Copyright © 2005 Sun Microsystems, Inc., 4150 Network Circle, Santa Clara, California 95054, Etats-Unis. Tous droits réservés. L’utilisation est soumise aux termes de la Licence. Cette distribution peut comprendre des composants développés par des tierces parties. Des parties de ce produit pourront être dérivées des systèmes Berkeley BSD licenciés par l’Université de Californie. UNIX est une marque déposée aux Etats-Unis et dans d’autres pays et licenciée exclusivement par X/Open Company, Ltd. Sun, Sun Microsystems, le logo Sun, Java, et JavaHelp sont des marques de fabrique ou des marques déposées de Sun Microsystems, Inc. aux Etats-Unis et dans d’autres pays.Toutes les marques SPARC sont utilisées sous licence et sont des marques de fabrique ou des marques déposées de SPARC International, Inc. aux Etats-Unis et dans d’autres pays. Les produits portant les marques SPARC sont basés sur une architecture développée par Sun Microsystems, Inc. Ce produit est soumis à la législation américaine en matière de contrôle des exportations et peut être soumis à la règlementation en vigueur dans d’autres pays dans le domaine des exportations et importations. Les utilisations, ou utilisateurs finaux, pour des armes nucléaires,des missiles, des armes biologiques et chimiques ou du nucléaire maritime, directement ou indirectement, sont strictement interdites. Les exportations ou réexportations vers les pays sous embargo américain, ou vers des entités figurant sur les listes d’exclusion d’exportation américaines, y compris, mais de manière non exhaustive, la liste de personnes qui font objet d’un ordre de ne pas participer, d’une façon directe ou indirecte, aux exportations des produits ou des services qui sont régis par la législation américaine en matière de contrôle des exportations et la liste de ressortissants spécifiquement désignés, sont rigoureusement interdites. LA DOCUMENTATION EST FOURNIE "EN L’ÉTAT" ET TOUTES AUTRES CONDITIONS, DECLARATIONS ET GARANTIES EXPRESSES OU TACITES SONT FORMELLEMENT EXCLUES, DANS LA MESURE AUTORISEE PAR LA LOI APPLICABLE, Y COMPRIS NOTAMMENT TOUTE GARANTIE IMPLICITE RELATIVE A LA QUALITE MARCHANDE, A L’APTITUDE A UNE UTILISATION PARTICULIERE OU A L’ABSENCE DE CONTREFAÇON. Contents Before You Begin 25 How This Book Is Organized 25 Typographic Conventions 27 Shell Prompts 28 Supported Platforms 28 Accessing Sun Studio Software and Man Pages 28 Accessing Sun Studio Documentation 31 Accessing Related Solaris Documentation 35 Resources for Developers 35 Contacting Sun Technical Support 36 Sending Your Comments 36 1. Getting Started With dbx 37 Compiling Your Code for Debugging 37 Starting dbx and Loading Your Program 38 Running Your Program in dbx 40 Debugging Your Program With dbx 41 Examining a Core File 41 Setting Breakpoints 43 Stepping Through Your Program 44 3 Looking at the Call Stack 45 Examining Variables 46 Finding Memory Access Problems and Memory Leaks 47 Quitting dbx 48 Accessing dbx Online Help 48 2. Starting dbx 49 Starting a Debugging Session 49 Debugging a Core File 50 Debugging a Core File in the Same Operating Environment 51 If Your Core File Is Truncated 51 Debugging a Mismatched Core File 52 Using the Process ID 54 The dbx Startup Sequence 55 Setting Startup Properties 56 Mapping the Compile-time Directory to the Debug-time Directory 56 Setting dbx Environment Variables 57 Creating Your Own dbx Commands 57 Compiling a Program for Debugging 57 Debugging Optimized Code 58 Code Compiled Without the -g Option 58 Shared Libraries Require the -g Option for Full dbx Support 59 Completely Stripped Programs 59 Quitting Debugging 59 Stopping a Process Execution 60 Detaching a Process From dbx 60 Killing a Program Without Terminating the Session 60 Saving and Restoring a Debugging Run 61 Using the save Command 61 4 Debugging a Program With dbx • November 2005 Saving a Series of Debugging Runs as Checkpoints 63 Restoring a Saved Run 63 Saving and Restoring Using replay 64 3. Customizing dbx 65 Using the dbx Initialization File 65 Creating a .dbxrc File 66 Initialization File Sample 66 Setting dbx Environment Variables 66 The dbx Environment Variables and the Korn Shell 72 4. Viewing and Navigating To Code 73 Navigating To Code 73 Navigating To a File 74 Navigating To Functions 74 Printing a Source Listing 75 Walking the Call Stack to Navigate To Code 76 Types of Program Locations 76 Program Scope 76 Variables That Reflect the Current Scope 77 Visiting Scope 77 Qualifying Symbols With Scope Resolution Operators 79 Backquote Operator 79 C++ Double Colon Scope Resolution Operator 79 Block Local Operator 80 Linker Names 82 Locating Symbols 82 Printing a List of Occurrences of a Symbol 82 Determining Which Symbol dbx Uses 83 Contents 5 Scope Resolution Search Path 84 Relaxing the Scope Lookup Rules 84 Viewing Variables, Members, Types, and Classes 85 Looking Up Definitions of Variables, Members, and Functions 85 Looking Up Definitions of Types and Classes 87 Debugging Information in Object files and Executables 89 Object File Loading 89 Listing Debugging Information for Modules 90 Listing Modules 91 Finding Source and Object Files 91 5. Controlling Program Execution 93 Running a Program 93 Attaching dbx to a Running Process 94 Detaching dbx From a Process 95 Stepping Through a Program 96 Single Stepping 97 Continuing Execution of a Program 97 Calling a Function 98 Using Ctrl+C to Stop a Process 100 6. Setting Breakpoints and Traces 101 Setting Breakpoints 101 Setting a stop Breakpoint at a Line of Source Code 102 Setting a stop Breakpoint in a Function 103 Setting Multiple Breaks in C++ Programs 104 Setting Data Change Breakpoints 106 Setting Filters on Breakpoints 109 Tracing Execution 112 6 Debugging a Program With dbx • November 2005 Setting a Trace 112 Controlling the Speed of a Trace 112 Directing Trace Output to a File 113 Setting a when Breakpoint at a Line 113 Setting a Breakpoint in a Shared Library 113 Listing and Clearing Breakpoints 114 Listing Breakpoints and Traces 114 Deleting Specific Breakpoints Using Handler ID Numbers 114 Enabling and Disabling Breakpoints 115 Efficiency Considerations 115 7. Using the Call Stack 117 Finding Your Place on the Stack 117 Walking the Stack and Returning Home 118 Moving Up and Down the Stack 118 Moving Up the Stack 118 Moving Down the Stack 119 Moving to a Specific Frame 119 Popping the Call Stack 119 Hiding Stack Frames 120 Displaying and Reading a Stack Trace 121 8. Evaluating and Displaying Data 123 Evaluating Variables and Expressions 123 Verifying Which Variable dbx Uses 123 Variables Outside the Scope of the Current Function 124 Printing the Value of a Variable, Expression, or Identifier 124 Printing C++ 124 Dereferencing Pointers 126 Contents 7 Monitoring Expressions 126 Turning Off Display (Undisplaying) 127 Assigning a Value to a Variable 127 Evaluating Arrays 127 Array Slicing 128 Slices 131 Strides 132 9. Using Runtime Checking 135 Capabilities of Runtime Checking 136 When to Use Runtime Checking 136 Runtime Checking Requirements 136 Limitations 137 Using Runtime Checking 137 Turning On Memory Use and Memory Leak Checking 137 Turning On Memory Access Checking 138 Turning On All Runtime Checking 138 Turning Off Runtime Checking 138 Running Your Program 139 Using Access Checking 142 Understanding the Memory Access Error Report 143 Memory Access Errors 143 Using Memory Leak Checking 144 Detecting Memory Leak Errors 145 Possible Leaks 145 Checking for Leaks 146 Understanding the Memory Leak Report 147 Fixing Memory Leaks 150 Using Memory Use Checking 150 8 Debugging a Program With dbx • November 2005 Suppressing Errors 152 Types of Suppression 152 Suppressing Error Examples 153 Default Suppressions 154 Using Suppression to Manage Errors 154 Using Runtime Checking on a Child Process 155 Using Runtime Checking on an Attached Process 159 Using Fix and Continue With Runtime Checking 160 Runtime Checking Application Programming Interface 162 Using Runtime Checking in Batch Mode 162 bcheck Syntax 163 bcheck Examples 163 Enabling Batch Mode Directly From dbx 164 Troubleshooting Tips 164 Runtime Checking’s 8 Megabyte Limit 164 Runtime Checking Errors 166 Access Errors 166 Memory Leak Errors 169 10.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages422 Page
-
File Size-