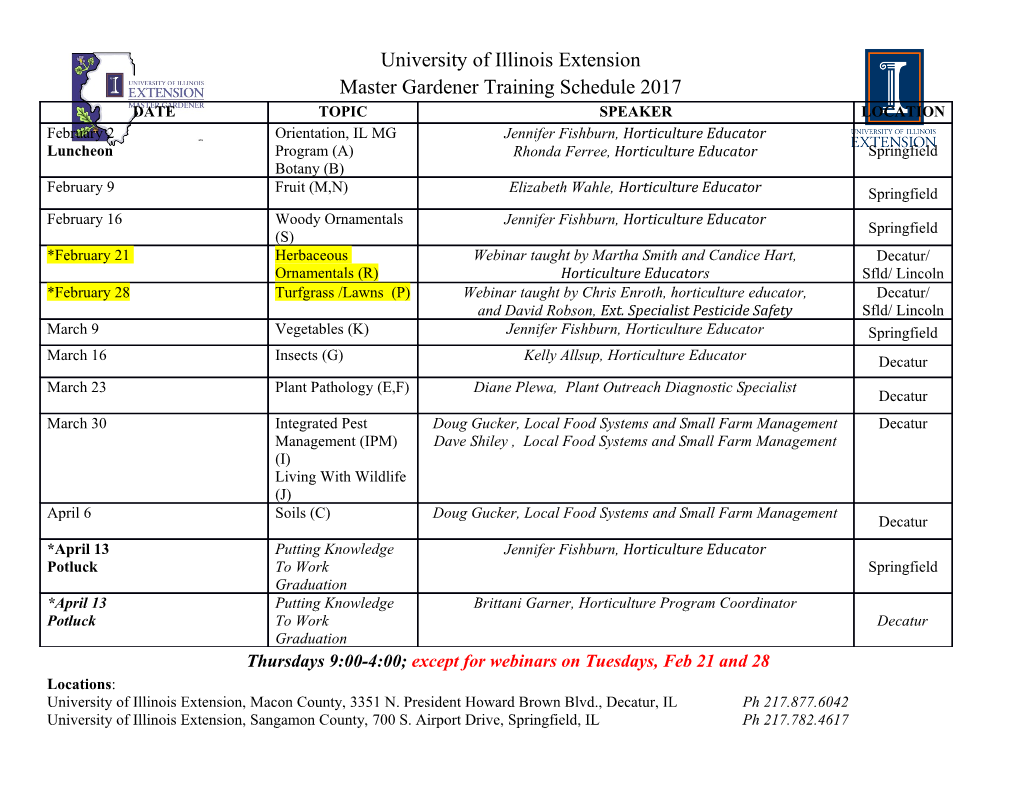
What is MATLAB MathWorks Logo I high-level language (garbage collecting, var-len structures) Introduction to MATLAB I BASIC-like syntax, with elements from C, GUI IDE I basic data type: 2- or 3-dimensional floating-point matrix I most operators and functions work on entire matrices Markus Kuhn ) hardly ever necessary to write out loops I uses internally highly optimized numerics libraries (BLAS, LAPACK, FFTW) I comprehensive toolboxes for easy access to standard algorithms from Computer Laboratory, University of Cambridge many fields: statistics, machine learning, image processing, signal processing, neural networks, wavelets, communications systems https://www.cl.cam.ac.uk/teaching/1920/TeX+MATLAB/ I very simple I/O for many data/multimedia file formats I popular for experimental/rapid-prototype number crunching I widely used as a visualization and teaching tool matlab-slides-4up.pdf 2019-10-05 17:41 d4e9c33 1 2 What MATLAB is not Open-source MATLAB alternatives Similar to MATLAB, subset compatible: GNU Octave, SciLab, FreeMat Other high-level languages for technical computing: I R { focus on statistics and plotting I not a computer algebra system https://www.r-project.org/ I not a strong general purpose programming language I Python { a full-featured programming language. Modules: • • limited support for other data structures numpy { numerical arrays, fast linear algebra • • few software-engineering features; matplotlib { MATLAB-like plotting functions https://matplotlib.org/ typical MATLAB programs are only a few lines long • SciPy { scientific computing, Pandas { data analysis, etc. • not well-suited for teaching OOP I Julia { modern, fast, full-featured, compiled, interactive language • limited GUI features https://julialang.org/ I not a high-performance language (but fast matrix operators) • MATLAB-inspired syntax (especially for arrays) got better since introduction of JIT compiler (JVM) • combines dynamic and static type systems, multiple dispatch I not freely available (but local campus licence) • LLVM backend, can also call C, C++, Python, R, Fortran functions • LISP-like metaprogramming, rich flexible type system I others: LuaJIT (SciLua), Perl Data Language (PDL), OCaml (Owl) Jupyter is a popular browser-based notebook environment for recording and presenting experiments in Julia, Python, R, . 3 4 Local availability Documentation MATLAB is installed and ready to use on I Intel Lab, etc.: MCS Windows I Intel Lab, etc.: MCS Linux (/usr/bin/matlab) I Full documentation built in: start matlab then type • I CL MCS Linux server: ssh -X linux.cl.ds.cam.ac.uk doc { to browse built-in hypertext manual • I MCS Linux server: ssh -X linux.ds.cam.ac.uk doc command { to jump to a specific manual page (e.g. plot) • I Computer Laboratory managed Linux PCs help command { to show plain-text summary of a command cl-matlab -> /usr/groups/matlab/current/bin/matlab I Read first: doc ! MATLAB ! Getting Started Campus license (666637) allows installation on staff/student home PCs I Tutorial videos: (Linux, macOS, Windows): https://uk.mathworks.com/videos.html I https://uk.mathworks.com/academia/tah-portal/ Documentation also available online (HTML and PDF): the-university-of-cambridge-666637.html I • https://uk.mathworks.com/help/matlab/ I Now (since 2018) includes full suite of ≈ 180 toolboxes: Statistics and Machine Learning, Signal Processing, Image Processing, Image • https://uk.mathworks.com/help/ { toolboxes Acquisition, Bioinformatics, Control Systems, Wavelets, Deep Locally installed MATLAB may be half a year behind the latest release. If you spot problems with Learning, Audio System, GPU Coder, Instrument Control, RF, . the MCS MATLAB installation, please do let the lecturer know (! [email protected]). I Installation requires setting up a https://uk.mathworks.com/ account with your @cam.ac.uk email address. The older Computer Laboratory license (136605) was replaced in 2018 by the University one. 5 6 MATLAB matrices (1) MATLAB matrices (2) Colon generates number sequence: Specify step size with second colon: Generate a \magic square" with equal row/column/diagonal sums and assign the resulting 3 × 3 matrix to variable a: >> 11:14 >> 1:3:12 ans = ans = >> a = magic(3) 11 12 13 14 1 4 7 10 a = 8 1 6 >> -1:1 >> 4:-1:1 3 5 7 ans = ans = 4 9 2 -1 0 1 4 3 2 1 Assignments and subroutine calls normally end with a semicolon. >> 3:0 >> 3:-0.5:2 Without, MATLAB will print each result. Useful for debugging! ans = ans = Results from functions not called inside an expression are assigned to the Empty matrix: 1-by-0 3.0000 2.5000 2.0000 default variable ans. Single matrix cell: a(2,3) == 7. Vectors as indices select several rows and Type help magic for the manual page of this library function. columns. When used inside a matrix index, the variable end provides the highest index value: a(end, end-1) == 9. Using just \:" is equivalent to \1:end" and can be used to select an entire row or column. 7 8 • = • 0 •••• 1 B •••• C B C · = B •••• C scalar product, dot product B C @ •••• A •••• 0 •••• 1 B •••• C = B C @ •••• A •••• row first factor column second factor matrix of all pair-wise products MATLAB matrices (3) MATLAB matrices (4) Select rows, columns and Matrices can also be accessed as a Use [] to build new matrices, where , or space as a delimiter joins submatrices of a: 1-dimensional vector: submatrices horizontally and ; joins them vertically. >> a(1:5) >> a(1,:) ans = >> c = [2 7; 3 1] Mask matrix elements: ans = 8 3 4 1 5 c = 8 1 6 2 7 >> find(a > 5) >> a(6:end) 3 1 ans = >> a(:,1) ans = >> d = [a(:,end) a(1,:)'] 1 ans = 9 6 7 2 d = 6 8 6 8 7 3 >> b = a(1:4:9) 7 1 8 4 ans = 2 6 >> a(find(a > 5)) = 0 8 5 2 >> e = [zeros(1,3); a(2,:)] a = >> a(2:3,1:2) e = 0 1 0 ans = >> size(b) 0 0 0 3 5 0 3 5 ans = 3 5 7 4 0 2 4 9 1 3 9 10 Review: matrix multiplication Review: inner and outer product of vectors Special cases of matrix multiplication 0 •••• 1 @ •••• A •••• 0 • 1 B • C · k •••• · B C = @ • A 0 ••• 1 0 •••• 1 • B ••• C B •••• C B C B C B ••• C B •••• C Row vector times column vector: B C B C @ ••• A @ •••• A ••• •••• 0 • 1 B • C B C · •••• @ • A Each element of the matrix product is the scalar product of • the corresponding in the and the corresponding in the Column vector times row vector: 11 12 MATLAB matrices (5) Plotting 20-point raised cosine 1 1 real 0.8 imaginary 0.8 Operators on scalars and matrices: Inner and outer vector product: 0.6 >> [2 3 5] * [1 7 11]' 0.6 0.4 >> [1 1; 1 0] * [2 3]' ans = 0.2 ans = 78 0.4 5 >> [2 3 5]' * [1 7 11] 0 2 ans = 0.2 -0.2 >> [1 2 3] .* [10 10 15] 2 14 22 -0.4 0 0 2 4 6 8 10 ans = 3 21 33 0 5 10 15 20 10 20 45 5 35 55 x = 0:20; t = 0:0.1:10; y = 0.5 - 0.5*cos(2*pi * x/20); x = exp(t * (j - 1/3)); p stem(x, y); plot(t, real(x), t, imag(x)); The imaginary unit vector −1 is available as both i and j, and title('20-point raised cosine'); grid; legend('real', 'imaginary') matrices can be complex. Related functions: real, imag, conj, exp, abs, angle Plotting functions plot, semilogx, semilogy, loglog all expect a pair of vectors for each curve, with x and y coordinates, respectively. Use saveas(gcf, 'plot2.eps') to save current figure as graphics file. 13 14 2D plotting Some common functions and operators -20 *, ^ imread, imwrite, image, matrix multiplication, exponentiation imagesc, colormap 1 -15 /, \, inv bitmap image I/O A=B = AB−1;AnB = A−1B; A−1 0.5 -10 plot, semilogfx,yg, loglog +, -, .*, ./, .^ 2D curve plotting element-wise add/sub/mul/div/exp 0 -5 conv, conv2, xcorr ==, ~=, <, >, <=, >= 1D/2D convolution, -0.5 0 relations result in element-wise 0/1 20 cross/auto-correlation sequence 20 length, size 0 10 5 fft, ifft, fft2 0 size of vectors and matrices -10 discrete Fourier transform -20 -20 zeros, ones, eye, diag 10 all-0, all-1, identity, diag. matrices sum, prod, min, max sum up rows or columns 15 xlim, ylim, zlim xl = -20:0.3:20; set plot axes ranges cumsum, cumprod, diff yl = -20:0.3:20; 20 xlabel, ylabel, zlabel cumulative sum or product, [x,y] = meshgrid(xl, yl); -20 -10 0 10 20 r = sqrt(x.^2 + y.^2); label plot axes differentiate row/column s = sin(r) ./ r; s(find(r==0)) = 1; imagesc(xl, yl, s, [-1 1]); audioread, audiowrite, sound find plot3(x, y, s); colormap(gray); audio I/O list non-zero indices grid on; set(gca, 'DataAspectRatio', [1 1 1]); csvread, csvwrite figure, saveas comma-separated-value I/O open new figure, save figure 15 16 Functions and m-files Example: generating an audio illusion To define a new function, for example decibel(x) = 10x=20, write into a Generate an audio file with 12 sine tones of apparently continuously file decibel.m the lines exponentially increasing frequency, which never leave the frequency range function f = decibel(x) 300{3400 Hz. Do this by letting them wrap around the frequency interval % DECIBEL(X) converts a decibel figure X into a factor and reduce their volume near the interval boundaries based on a f = 10 .^ (x ./ 20); raised-cosine curve applied to the logarithm of the frequency.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-