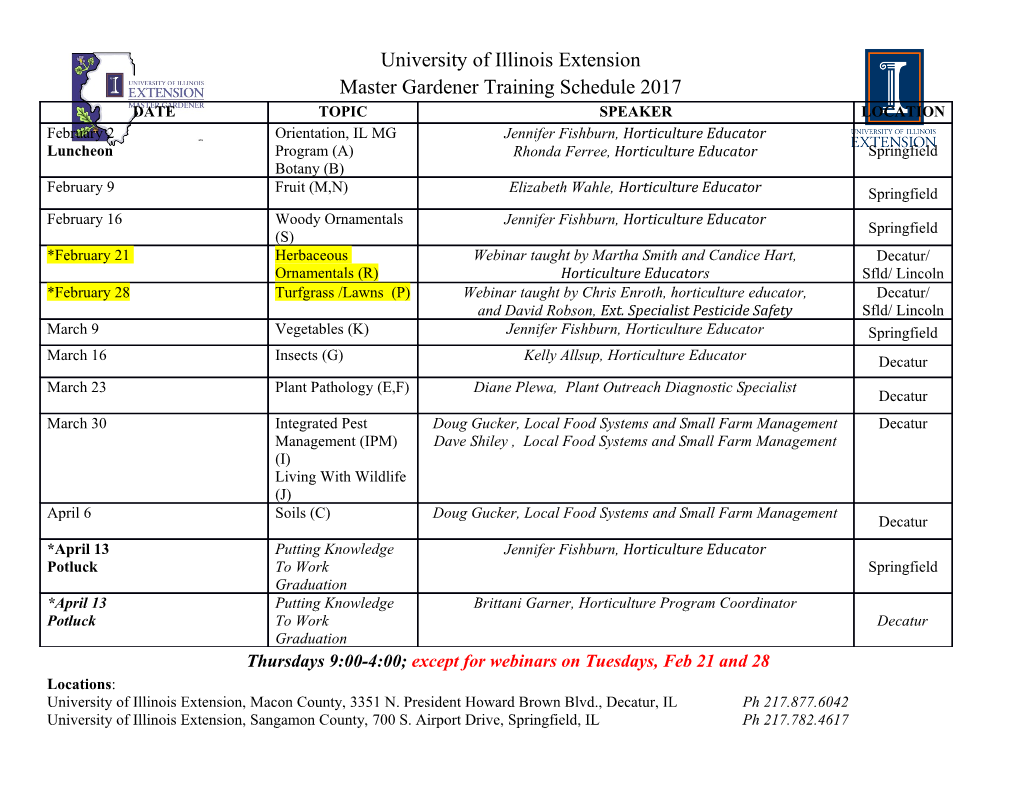
Programming with Categories (DRAFT) Brendan Fong Bartosz Milewski David I. Spivak (Last updated: October 6, 2020) Contents Preface vii Learning programming................................ ix Installing Haskell................................ x Haskell points.................................. xi Acknowledgments ..................................xiv 1 Categories, Types, and Functions1 1.1 Programming: the art of composition .................... 1 1.2 Two fundamental ideas: sets and functions................. 3 1.2.1 What is a set?.............................. 3 1.2.2 Functions ................................ 4 1.2.3 Some intuitions about functions ................... 6 1.3 Categories.................................... 9 1.3.1 Motivation: the category of sets.................... 9 1.3.2 The definition of a category...................... 12 1.3.3 Examples of categories......................... 14 1.3.4 Thinking in a category: the Yoneda perspective .......... 20 1.4 Categories and Haskell............................. 23 1.4.1 The lambda calculus.......................... 23 1.4.2 Types................................... 26 1.4.3 Haskell functions............................ 28 1.4.4 Composing functions.......................... 30 1.4.5 Thinking categorically about Haskell ................ 33 2 Universal constructions and the algebra of types 35 2.1 Constructing datatypes............................. 35 2.2 Universal constructions............................. 37 2.2.1 Terminal objects............................. 37 2.2.2 Initial objects .............................. 39 2.2.3 Products................................. 40 ii CONTENTS iii 2.2.4 Coproducts ............................... 43 2.3 Type constructors................................ 44 2.3.1 Type constructors............................ 45 2.3.2 Unit and void.............................. 46 2.3.3 Tuple types ............................... 48 2.3.4 Sum types................................ 53 2.4 Exponentials and function types ....................... 56 2.4.1 Interlude: Distributivity........................ 56 2.4.2 Exponential objects........................... 57 2.4.3 Function types, and currying in Haskell............... 60 3 Functors, natural transformations, and type polymorphism 65 3.1 Relationships, relationships, relationships.................. 65 3.2 Functors ..................................... 66 3.2.1 Definition ................................ 66 3.2.2 Examples of functors.......................... 67 3.2.3 Functors and shapes.......................... 71 3.2.4 The category of categories....................... 74 3.3 Type classes ................................... 74 3.3.1 Polymorphism in Haskell....................... 74 3.3.2 Defining type classes and instances ................. 75 3.4 Functors in Haskell............................... 79 3.4.1 The Functor type class......................... 79 3.4.2 First examples of functors in Haskell................. 81 3.4.3 Bifunctors................................ 83 3.4.4 A first glance at profunctors...................... 86 3.5 Natural transformations ............................ 88 3.5.1 Definition ................................ 88 3.5.2 Natural transformations in Haskell.................. 89 3.6 Bonus: Representable functors and the Yoneda embedding . 92 4 Algebras and recursive data structures 97 4.1 The string before the knot ........................... 98 4.2 What you can do with recursive data types . 100 4.3 Algebras .....................................103 4.4 Initial algebras..................................105 4.4.1 Lambek’s lemma............................107 4.5 Recursive data structures............................108 4.5.1 Returning to expression trees.....................110 4.5.2 The essence of recursion........................112 4.5.3 Algebras, catamorphsims, and folds . 113 4.6 Coalgebras, anamorphsims, and unfolds...................114 iv CONTENTS 4.6.1 The type of streams, as a terminal coalgebra . 116 4.6.2 The stream of prime numbers.....................117 4.7 Fixed points in Haskell.............................120 4.7.1 Implementing initial algebras by universal property . 121 4.7.2 Implementing terminal coalgebras by universal property . 123 5 Monads 125 5.1 A teaser......................................125 5.2 Different ways of working with monads...................127 5.2.1 Monads in terms of the “fish”.....................127 5.2.2 Monads in terms of join ........................127 5.2.3 Monads in terms of bind........................130 5.2.4 Monads in terms of the do notation . 131 5.2.5 Monads and effects...........................134 5.3 Examples of monads ..............................134 5.3.1 The exceptions monads ........................134 5.3.2 The list monad and nondeterminism . 135 5.3.3 The writer monads...........................136 5.3.4 The reader monads...........................137 5.3.5 The state monads............................139 5.3.6 The continuation monads.......................140 5.3.7 The IO monad..............................141 6 Monoidal Categories 143 6.1 Lax Monoidal Functors.............................143 6.1.1 Monad as Applicative . 143 6.2 Strength and enrichment............................146 7 Profunctors 149 7.1 Profunctors revisited..............................149 7.2 Ends and Coends................................153 7.3 Profunctors in Haskell .............................156 7.4 Category of profunctors ............................158 7.4.1 Category of profunctors in Haskell..................160 7.5 Day convolution.................................161 7.5.1 Day convolution in Haskell ......................163 7.6 Optics.......................................163 7.6.1 Motivation................................163 7.6.2 Tensorial optics.............................164 7.6.3 Lens ...................................164 7.6.4 Lens in Haskell.............................165 7.6.5 Prism...................................165 CONTENTS v 7.6.6 Prism in Haskell ............................166 7.7 Profunctor optics ................................166 7.7.1 Tambara modules............................166 7.7.2 Profunctor optics in Haskell......................167 Index 169 Preface This book is about how to think using category theory. What does this mean? Category theory is a favorite tool of ours for structuring our thoughts, whether they be about pure math, science, programming, engineering, or society. It’s a formal, rigorous toolkit designed to emphasise notions of relationship, composition, and connection. It’s a mathematical tool, and so is about abstraction, stripping away details and noticing patterns; one power it gains from this is that it is very concise. It’s great for compressing thoughts, and communicating them in short phrases that can be reliably decoded. The flip side of this is that it can feel very unfamiliar at first – a strange, new mode of thought – and also that it often needs to be complemented by more concrete tools to fully realise its practicality. One also needs to practice a certain art, or taste, in knowing when an abstraction is useful for structuring thought, and when it should be broken, or approximated. Programmers know this well, of course: a programming language never fully meets its specification; there are always implementation details that the best must be familiar with. In this book, we’ve chosen programming, in particular functional programming, as the vehicle through which we’ll learn how to think using category theory. This choice is not arbitrary: category theory is older than digital computing itself, and has heavily influenced the design of modern functional programming languages such as Haskell. As we’ll see, it’s also influenced best practice for how to structure code using these languages. Thinking is not just an abstract matter; the best thought has practical consequences. In order to teach you how to think using category theory, we believe that it’s important to give a mechanism for implementation and feedback on how category theory is affecting your thought. So in this book, we’ll complement the abstraction of category theory – lessons on precise definitions of important mathematical structures – with an introduction to programming in Haskell, and lessons on how category theoretic abstractions are approximated to perform practical programming tasks. That said, this book is not intended to be a book about Haskell, and there are many other programming languages that you could use as a world in which to try out these categorical ideas. In fact, we hope you do! Thinking categorically is about using a set vii viii PREFACE of design patterns that emphasise composition, universal constructions, and algebraic structure. Used judiciously, we believe this style of thought can improve the clarity and correctness of code in any language, including those currently popular, and those that will be developed in the years to come. Category theory is a vast toolbox, that is still under active construction. A vibrant community of mathematicians and computer scientists is working hard to find new perspectives, structures, and definitions that lead to compact, insightful ways to reason about the world. We won’t teach all of it. In fact, we’ll teach a very small core, some central ideas all over fifty years old. The first we’ll teach is the namesake: categories. Programming is about composi- tion: it’s about taking programs, some perhaps just single functions, and making them work in sync to construct a larger, usually more
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages183 Page
-
File Size-