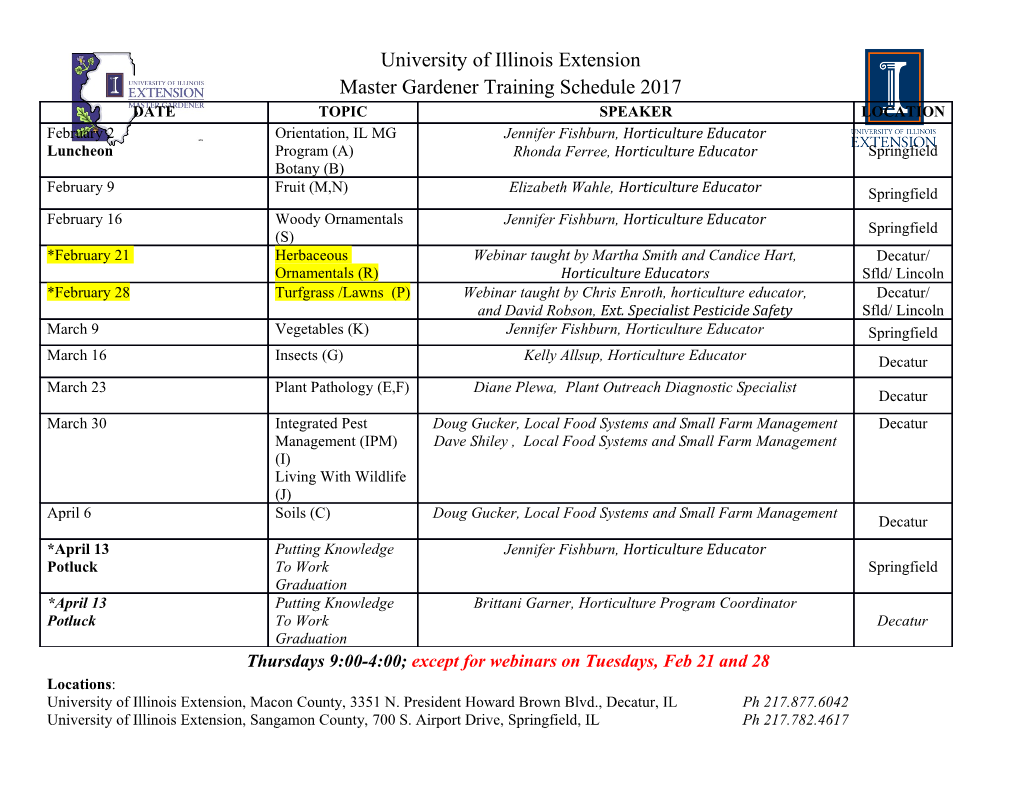
Selected Topics in Object Oriented Programming Lecture 3: More OOP principles Leonid Barenboim Permissions Permissions define the areas within a program from which a feature (field/ method) can be accessed: private – only within the same class. protected - only within the class and its subclasses. public – from everywhere. Permission in java Java use somewhat different rules: private – only within the same class. package – only within the same package. protected – within the same package and within the subclasses outside the package. public – from everywhere. Selecting the right permission The principle of encapsulation suggests the following rules: All non-static fields should be private Service methods should be public Helper methods should be private/protected Permission rules in specific languages Smalltalk – all fields are private all methods are public Scripting languages (e.g., python) - No permissions! - Everything is public! The Visitor Pattern Reminder: Polymorphism Reminder: Polymorphism Animal animal; animal = new Dog; Animal * animal.say(); \\ “Woof” animal = new Cat; + say() * animal.say(); \\ “Miau” Cat Dog + say() + say() System.out.println(“Miau”) System.out.println(“Woof”) What happens if a method accepts an argument? boolean b; Animal * Animal cat = new Cat(); Animal mouse = new + say() * Mouse(); + eats(mouse: Mouse) : bool * b = cat.eats(mouse); + eats(cat: Cat) : bool * //compilation error Cat Mouse + say() + say() +eats(mouse : Mouse) : bool +eats(mouse : Mouse) : bool +eats(cat : Cat) : bool +eats(cat : Cat) : bool No support for polymorphism of arguments • Java (and many other languages) supports only polymorphism of the receiver (the variable on which the method is invoked). • Polymorphism of the receiver is performed using single dispatch. • Polymorphism of arguments is performed using multiple-level dispatch, which is resource consuming. Therefore, it is not supported. • Nevertheless, we can simulate it using the Visitor Pattern . The visitor pattern (for methods with one argument) Define two interfaces: Visitor and Visited. • The Visitor interface contains a method for each possible argument type. The visitor pattern (for methods with one argument) Define two interfaces: Visitor and Visited. • The Visitor interface contains a method for each possible argument type. Visitor +visit (cat : Cat) +visit (mouse : Mouse) The visitor pattern (for methods with one argument) Define two interfaces: Visitor and Visited. • The Visitor interface contains a method for each possible argument type. • The Visited interface contains an accpet() method. Visitor Visited +visit (cat : Cat) +accept(v : Visitor) +visit (mouse : Mouse) The visitor pattern (cont.) (for methods with one argument) • The reciever’s base class implements the visitor interface • The argument’s base class implements the visited interface The visitor pattern (cont.) (for methods with one argument) • The reciever’s base class implements the visitor interface • The argument’s base class implements the visited interface Visitor +visit (cat : Cat) +visit (mouse : Mouse) Animal * +eats(animal: Animal)* +visit (cat : Cat)* +visit (mouse : Mouse)* The visitor pattern (cont.) (for methods with one argument) • The reciever’s base class implements the visitor interface • The argument’s base class implements the visited interface Visitor +visit (cat : Cat) Visited +visit (mouse : Mouse) +accept (v : Visitor) Animal * +eats (animal: Animal)* +accept (v : Visitor)* +visit (cat : Cat)* +visit (mouse : Mouse)* Implementation of Visitor subclasses Implementation of Visited subclasses public class Cat extends Animal{ … public void eats(Animal animal){ public void accept(Animal animal){ animal.accept(this); } animal.visit(this); public void visit(Cat cat){ } s.o.p(“No”); } } public void visit(Mouse mouse){ s.o.p(“Yes”); } … Implementation of Visitor subclasses Implementation of Visited subclasses public class Mouse extends Animal{ … public void eats(Animal animal){ public void accept(Animal animal){ animal.accept(this); } animal.visit(this); public void visit(Cat cat){ } s.o.p(“No”); } } public void visit(Mouse mouse){ s.o.p(“No”); } … Putting it all together Visitor Visited +visit (cat : Cat) +accept (v : Visitor) +visit (mouse : Mouse) Animal * +eats (animal: Animal) animal.accept(this) Cat Mouse +accept(animal: Animal) +accept(animal: Animal) +visit (cat : Cat) +visit (cat : Cat) +visit (mouse : Mouse) +visit (mouse : Mouse) animal.visit(this) animal.visit(this) Different types of Visitor and Visited Employees and Reports example Visited Visitor accept(v: Visitor) visit(r : Regular) visit(m :Manager) Employee Format visit(s :Specialist) accept(v: Visitor) print(e :Employee)+ Regular e.accept(this) accept(v: Visitor) + Manager Block Csv accept(v: Visitor)+ Specialist visit(r: Regular)+ visit(r : Regular)+ accept(v: Visitor)+ visit(m: Manager)+ visit(m :Manager)+ v.visit(this) visit(s: Specialist)+ visit(s :Specialist)+ The Visitor Pattern Advantages • Simulating multiple-level dispatch. • Type-based treatment without instanceof. • Extending the functionality of a class hierarchy by an external class. The Visitor Pattern Shortcomings • Tedious to code. • Adding a Visited class requires modifying existing Visitor classes. • Dispatching multiple arguments becomes inconvenient..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages23 Page
-
File Size-