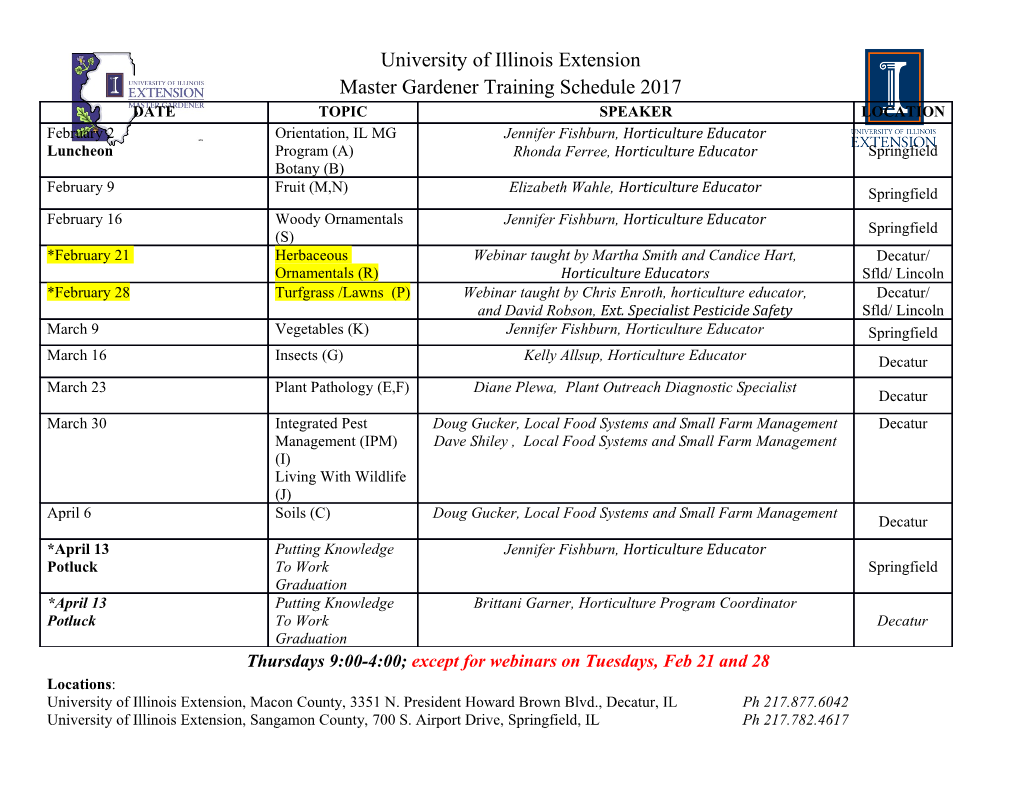
Massachusetts Institute of Technology Dept. of Electrical Engineering and Computer Science Spring Semester, 2007 6.082 Introduction to EECS 2 Lab #2: Time-Frequency Analysis Goal:.................................................................................................................................... 2 Instructions:......................................................................................................................... 2 Prelab: ................................................................................................................................. 3 Understanding Sample Period, Exponential Damping, and the FFT function ............... 3 Lab Exercises (2pm – 5pm, Wed., February 14, 2007):..................................................... 9 Background on Piano Music........................................................................................... 9 Starting Matlab and creating a Lab2 Directory............................................................. 11 Time-Domain Analysis of Piano Notes ........................................................................ 11 Frequency-Domain Analysis of Piano Notes................................................................ 14 Signal Modeling and Synthesis of Piano Notes............................................................ 15 An Even Simpler Model for our Synthesized Piano Notes........................................... 18 A Composition Script for Matlab ................................................................................. 20 Exercises ....................................................................................................................... 21 PostLab (Due on Friday, February 16, 2007) ................................................................... 24 Check-off for Lab 2 .......................................................................................................... 25 6.082 Fall 2006 1 of 25 Lab #2 Goal: Examination of signals in both the time and frequency domains, and observation of the benefits that each domain provides toward understanding the structure of a given signal. We will also briefly touch upon signal modeling concepts which are used to approximate certain signals through knowledge of their underlying structure. The context of our exercises will be examination of a recorded song from a piece of piano music, and an attempt to synthesize that music. Instructions: 1. Complete the Prelab exercises BEFORE Wednesday’s lab. 2. Complete the activities for Wednesday’s lab (see below) and get checked off by one of the TAs before leaving. Be sure to work in pairs with one computer per pair. 3. Complete the Postlab exercises BEFORE Friday, and turn them in at the beginning of lecture on Friday. 6.082 Fall 2006 2 of 25 Lab #2 Prelab: Understanding Sample Period, Exponential Damping, and the FFT function Before we enter Lab 2 and examine signals created by a piano, we need to provide some additional background that will help us in our future analysis. • To understand the concept of sample period, type the following commands in Matlab (note that you need not include the statements following the % symbols since they are simply comments): Fs = 22050; % sample frequency in Hz Ts = 1/Fs; % sample period in seconds t = (1:1000)*Ts; % time vector with 1001 samples y = 0.5*sin(2*pi*330*t); % sine wave with amplitude 0.5 % and frequency 330 Hz plot(t,y); o You should see a Figure 1 plot that appears as below. Note that the amplitude of the sine wave is easily seen to be 0.5, but that its frequency of 330 Hz requires more work to verify. To do so, note that the period of the sine wave should be 1/330 Hz = 0.003 seconds. To verify that the sine wave period is indeed that value, type axis([0 0.003 -1 1]) in Matlab and observe the resulting plot. o In the above example, we see that sample period corresponds to the increment between each time sample in the vector t that we created. To better understand this concept, consider the following two examples: t = 1:1000 creates a vector [1 2 3 ….. 1000] t = (1:1000)*Ts creates a vector [1*Ts 2*Ts 3*Ts …. 1000*Ts] 6.082 Fall 2006 3 of 25 Lab #2 o We also see that sample frequency simply corresponds to the inverse of sample period. Note that the sine wave frequency and period are different than the sample frequency and period. In the case where the sample frequency is much higher than the sine wave frequency (or, equivalently, where the sample period is much lower than the sine wave period), the sampled waveform resembles a continuous-time waveform. We will provide more details of sampling later in the class. • To understand the concept of exponential damping, type the following commands in Matlab: tau = 7e-3; r = exp(-t/tau); plot(t,r); o You should see a plot as shown below, which displays the decaying exponential function as a function of time. The rate of decay is set by the time constant of the exponential, which corresponds to variable tau in the above example. Try different values of tau in the above example to see how it influences the decay time of the exponential. o One important point – the decaying exponential that you are seeing above is not the same as the complex exponentials that we have been discussing in lecture. o While the above plot shows a decaying exponential, the expression exponential damping is typically associated with a non-exponential waveform whose amplitude decays in exponential fashion. A very relevant example is exponential damping of a sine wave, as illustrated by executing the following Matlab commands (with tau = 7e-3): y_damped = sin(2*pi*330*t).*exp(-t/tau); plot(t,y_damped); 6.082 Fall 2006 4 of 25 Lab #2 o After execution of the above command, you should see the plot below. Be sure to note the use of the two characters “.*” in the above example – the “.*” character combination is used when multipling two vectors on an element-by-element basis. Try changing tau to a few different values to see the resulting waveform changes. • To see the frequency domain content of a given signal in Matlab, we typically use the function fft (which stands for Fast Fourier Transform). Based on the Fourier analysis discussed in lecture, the fft operates on non-periodic signals which are sampled in time and have finite length. The output of the fft is very similar to what we would expect from doing the Fourier Series of a periodic signal – we essentially obtain a and b coefficients which can be examined to see the frequency content of a given signal. o In Matlab, type edit fft_example.m o Be sure to answer yes when prompted for creation of a new file. o Within the new edit window, type in the following commands (note that there is no need to type the comments following the % symbols): t = 0:19; f = -10:9; % use index of frequencies for easy viewing in stem plot y = sin(2*pi*1/20*t); % sine wave with frequency 1/20 yf = fft(y); a = real(yf); % a coefficients of transform b = imag(yf); % b coefficients of transform subplot(221); % left, upper corner of 2x2 plot stem(f,fftshift(a)); % make stick figure plot, fftshift used to % properly show positive and negative frequencies title(‘a coeff’); 6.082 Fall 2006 5 of 25 Lab #2 subplot(222); % right, upper corner of 2x2 plot stem(f,fftshift(b)); title(‘b coeff’); subplot(223); % left, lower corner of 2x2 plot stem(f,fftshift(abs(yf))); % magnitude of fft coefficients ( = sqrt(a2 + b2) ) title(‘magnitude’); o You should now see a plot as shown below. Notice that for the sine wave signal, the a coefficients are all essentially zero (notice the scale is 1e-15), whereas the b coefficients have non-zero values only for index values of +1 and -1 (which correspond to frequencies of +1/20 and -1/20). Notice that the magnitude plot is even symmetric about zero frequency, and the phase plot is odd symmetric about zero frequency. • To better see when the a or b coefficients are essentially zero, add the following axis commands (which are in bold) to the above script. Upon re-running the script, you should see the plot shown below. …. title(‘a coeff’); axis([-10 10 -10 10]); …. title(‘b coeff’); axis([-10 10 -10 10]); ….. 6.082 Fall 2006 6 of 25 Lab #2 • Now change y in the above script from a sine to a cosine waveform and then re- run the script to the plot shown below: ….. y = cos(2*pi*1/20*t); % cosine wave with frequency 1/20 ….. o The above plot reveals that the b coefficients are now zero. • Finally, double the frequency of the cosine waveform and re-run the script to get the plot shown below: ….. y = cos(2*pi*1/10*t); % cosine wave with frequency 1/10 ….. 6.082 Fall 2006 7 of 25 Lab #2 o The above plot shows that the frequency content of the signal has shifted to index values of +2 and -2 (which correspond to frequencies +2/20 and -2/20). • As we complete our exercise on the fft function, we mention a few things that will useful to know in the remaining exercises in this lab: o Notice that we did not bother plotting the phase of the fft coefficients in the above examples. For many applications, the magnitude of the fft is of primary importance, and, in such cases, we rarely look at the phase or even the a and b coefficients themselves. o Since the magnitude is always even symmetric, we often just focus on the positive frequency range. 6.082 Fall 2006 8 of 25 Lab #2 Lab Exercises (2pm – 5pm, Wed., February 14, 2007): Background on Piano Music Pressing a piano’s key causes a hammer to strike a set of taut strings; the hammer then falls away from the strings so as not to dampen their vibration. The vibration of the strings produces the sound that we hear and recognize as the strike of a piano’s key. The quality of the sound we hear is determined by the amplitude and frequency of the string’s vibration, which in turn are related to the length, diameter, tension and density of the string. Most pianos have 88 keys with the leftmost key producing the lowest frequency sound. The keys on a piano are arranged in a repeating pattern of 12 keys (7 white and 5 black) as shown below. 6.082 Fall 2006 9 of 25 Lab #2 Increasing Frequency One Octave: 12 Notes Each of the 12 keys in a pattern corresponds to a note in the twelve-note Western Music Scale.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages25 Page
-
File Size-