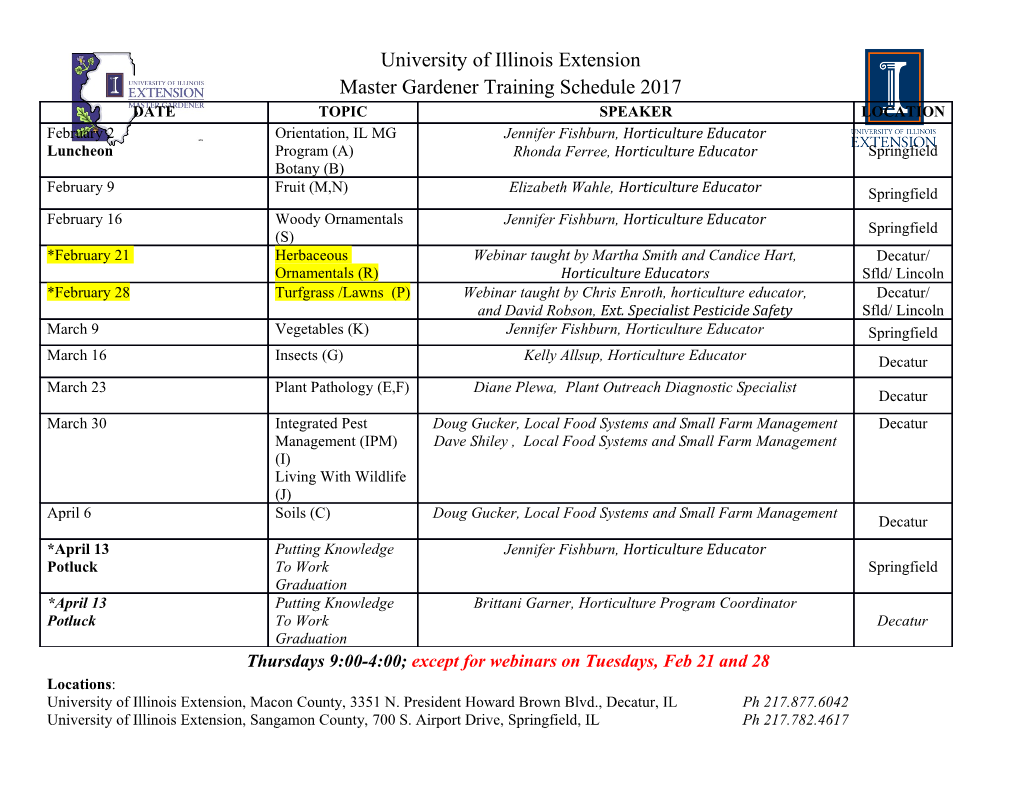
TUTORIAL PYPARTED PYPARTED: PYTHON DOES TUTORIAL DISK PARTITIONING VALENTINE Build a custom, command line disk partitioning tool by joining the user-friendliness of Python and the power of C. SINITSYN artitioning is a traditional way to split disk install to build and install it. It’s a good idea to install WHY DO THIS? drives into manageable chunks. Linux comes PyParted you’ve built yourself inside the virtualenv • Take complete control of Pwith variety of tools to do the job: there are (see http://docs.python-guide.org/en/latest/dev/ your system’s hard disk. fdisk, cfdisk or GNU Parted, to name a few. GNU virtualenvs for details), to keep your system • Write a custom installer Parted is powered by a library by the name of directories clean. There is also a Makefile, if you wish. to make things easier libparted, which also lends functionality to many This article’s examples use PyParted 3.10, but the for your users. graphical tools such as famous GParted. Although it’s concepts will stay the same regardless of the version • Amaze your friends and family with your mastery powerful, libparted is written in pure C and thus not you actually use. of the libparted C library. very easy for the non-expert to tap into. If you’re Before we start, a standard caution: partitioning writing your own custom partitioner, to use libparted may harm the data on your hard drive. Back you’re going to have to manage memory manually everything up before you do anything else! and do all the other elbow grease you do in C. This is where PyParted comes in – a set of Python bindings Basic concepts to libparted and a class library built on top of them, The PyParted API has two layers. At the bottom one is initially developed by Red Hat for use in the Anaconda the _ped module. Implemented entirely in C, it tries to installer. keep as close as possible to the native libparted C API. So why would you consider writing disk partitioning On top of that, the ‘parted’ package with high-level software? There could be several reasons: Python classes, functions and exceptions is built. You You are developing a system-level component like can use _ped functions directly if you wish; however, an installer for your own custom Linux distribution the parted package provides a more Pythonic You are automating a system administration task approach, and is the recommended way to use such as batch creation of virtual machine (VM) PyParted in your programs unless you have some images. Tools like ubuntu-vm-builder are great, but special requirements. We won’t go into any details of they do have their limitations using the _ped module in this article. You’re just having fun. Before you do anything useful with PyParted, you’ll PyParted hasn’t need a Device instance. A Device represents a piece of made its way into the physical hardware in your system, and provides the “A word of caution: partitioning Python Package Index means to obtain its basic properties like model, may harm the data on your (PyPI) yet, but you may geometry (cylinders/heads/sectors – it is mostly fake be lucky enough to find for modern disks, but still used sometimes), logical hard drive. Back everything up!” it in your distribution’s and physical sector sizes and so on. The Device class repositories. Fedora also has methods to read data from the hardware and (naturally), Ubuntu and Debian provide PyParted write it back. To obtain a Device instance, you call one packages, and you can always build PyParted yourself of the global functions exported by PyParted (in the from the sources. You will need the libparted headers examples below, >>> denotes the interactive Python (usually found in libparted-dev or similar package), prompt, and … is an omission for readability reasons Python development files and GCC. PyParted uses the or line continuation if placed at the beginning): distutils package, so simply enter python setup.py >>> import parted >>> # requires root privileges to communicate ... with the kernel >>> [dev.path for dev in parted.getAllDevices()] [u’/dev/sda’, u’/dev/mapper/ubuntu--vg-swap_1’, u’/dev/mapper/ubuntu--vg-root’, u’/dev/mapper/sda5_crypt’] >>> # get Device instance by path >>> sda = parted.getDevice(‘/dev/sda’) PyParted was developed to >>> sda.model facilate Red Hat’s installer, u’ATA WDC WD1002FAEX-0’ Anaconda. >>> sda.hardwareGeometry, sda.biosGeometry 94 www.linuxvoice.com PYPARTED TUTORIAL ((121601, 255, 63), (121601, 255, 63)) # cylinders, heads, sectors Caution: partitioning may void your warranty >>> sda.sectorSize, sda.physicalSectorSize Playing with partitioning is fun but also quite is writable for the user that you are running (512L, 512L) dangerous: wiping the partition table on your scripts as). The last command removes the >>> # Destroy partition table; NEVER do this on machine’s hard drive will almost certainly device when it is no longer needed. ... your computer’s disk! result in data loss. It is much safer to do If you feel adventurous, you can also fake >>> sda.clobber() your experiments in a virtual machine (like your hard drive with a qcow2 (as used by VirtualBox) with two hard drives attached. If Qemu), VDI, VMDK or other image directly True this is not an option, you can ‘fake’ the hard supported by virt-manager, Oracle VirtualBox Next comes the Disk, which is the lowest-level drive with an image file ($ is a normal user or VMware Workstation/Player. These operating system-specific abstraction in the PyParted and # is a superuser shell prompt): images can be created with qemu-img and class hierarchy. To get a Disk instance, you’ll need a $ dd if=/dev/zero of=<image_file_name> \ mounted with qemu-nbd: Device first: bs=512 count=<disk_size_in_sectors> $ qemu-img create -f vdi disk.vdi 10G This will almost work; however, Partition. # modprobe nbd >>> disk = parted.newDisk(sda) getDeviceNodeName() will return non- # qemu-nbd -c /dev/nbd0 disk.img Traceback (most recent call last): existent nodes for partitions on that device. You can then mount the partitions on ... For more accurate emulation, use losetup disk.img as /dev/nbd0pX (where X is _ped.DiskException: /dev/sda: unrecognised disk label and kpartx: partition number), provided the label you use This reads the disk label (ie the partitioning scheme) # losetup -f <image_file_name> is supported by your OS kernel (unless you from /dev/sda and returns the Disk instance that # kpartx -a /dev/loopX are creating something very exotic, this will ... be the case). When you are done, run: represents it. If /dev/sda has no partitions (consider # losetup -d /dev/loopX # qemu-nbd -d /dev/nbd0 the sda.clobber() call before), parted.DiskException is where X is the losetup device assigned to to disconnect image from the device. This raised. In this case, you can create a new disk label of your image file (get it with losetup -a). After way, you can create smaller images that are your choice: that, you may refer to the partitions on your directly usable in virtual machines. image file via /dev/loopXpY (or /dev/mapper/ Sometimes, it may look like changes you >>> disk = parted.freshDisk(sda, ‘msdos’) # or ‘gpt’ loopXpY, depending on your distribution). make to such virtual drives via external tools You can do it even if the disk already has partition This will require root privileges, so be careful. (like mkfs) are silently ignored. If this is your table on it, but again, beware of data-loss. PyParted You can still run your partitioning scripts case, flush the disk buffers: supports many disk labels. However, traditional on an image file as an ordinary user, given # blockdev --flushbufs <device_node_name> ‘msdos’ (MBR) and newer ‘gpt’ (GUID Partition Table) that the file has sufficient permissions (ie are probably most popular in PC world. Disk’s primary purpose is to hold partitions: # Will be empty after clobber() or freshDisk() >>> geometry = parted.Geometry(start=0, >>> disk.partitions ... length=parted.sizeToSectors(128, ‘MiB’, [<parted.partition.Partition object at 0x1043050>, ...] ... sda.sectorSize), device=sda) Each partition is represented by Partition object >>> new_partition = parted.Partition(disk=disk, which provides ‘type’ and ‘number’ properties: ... type=parted.PARTITION_NORMAL, >>> existing_partition = disk.partitions[0] ... geometry=geometry) >>> existing_partition.type, existing_partition.number >>> new_partition.geometry (0L, 1) # 0 is normal partition <parted.geometry.Geometry object at 0xdc9050> >>> parted.PARTITION_NORMAL Partitions (or geometries, to be more precise) may 0 also have an associated FileSystem object. PyParted Besides parted.PARTITION_NORMAL, there are can’t create new filesystems itself (parted can, but it is other partition types (most importantly, parted. still recommended that you use special-purpose PARTITION_EXTENDED and parted.PARTITION_ utilities like mke2fs). However, it can probe for existing LOGICAL). The ‘msdos’ (MBR) disk label supports all filesystems: of them, however ‘gpt’ can hold only normal partitions. >>> parted.probeFileSystem(new_partition.geometry) PRO TIP Partitions can also have flags like parted. Traceback (most recent call last): Python virtual PARTITION_BOOT or parted.PARTITION_LVM. Flags ... environments are set by the Partition.setFlag() method, and _ped.FileSystemException: Failed to find any filesystem (virtualenvs) are a great retrieved by Partition.getFlag(). We’ll see some in given geometry to play with modules that you don’t need on your examples later. >>> parted.probeFileSystem(existing_partition.geometry) system permanently. The partition’s position and size on the disk are u’ext2’ defined by the Geometry object. Disk-related values >>> new_partition.fileSystem (offsets, sizes, etc) in PyParted are expressed in <parted.filesystem.FileSystem object at 0x27a1310> sectors; this holds true for Geometry and other The names (and corresponding FileSystem objects) classes we’ll see later. You can use the convenient for filesystems recognised by PyParted are stored in function parted.sizeToSectors(value, ‘B’, device.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-