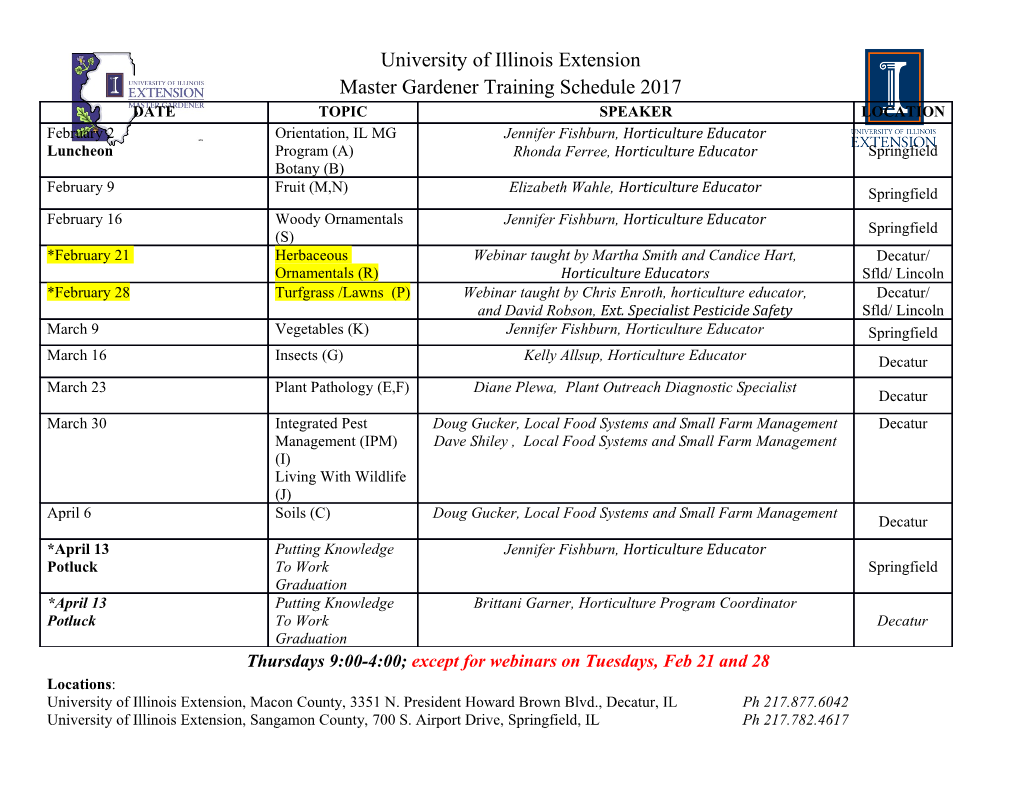
Programming Languages and Techniques Lecture Notes for CIS 120 Steve Zdancewic Stephanie Weirich University of Pennsylvania March 22, 2016 2 CIS 120 Lecture Notes Draft of March 22, 2016 Contents 1 Overview and Program Design 9 1.1 Introduction and Prerequisites . .9 1.2 Course Philosophy . .9 1.3 How the different parts of CIS 120 fit together . 13 1.4 Course History . 15 1.5 Program Design . 15 2 Introductory OCaml 21 2.1 OCaml in CIS 120 . 21 2.2 Primitive Types and Expressions . 21 2.3 Value-oriented programming . 23 2.4 let declarations . 25 2.5 Local let declarations . 27 2.6 Function Declarations . 29 2.7 Types . 31 2.8 Failwith . 33 2.9 Commands . 34 2.10 A complete example . 37 2.11 Notes . 39 3 Lists and Recursion 41 3.1 Lists . 41 3.2 Recursion . 45 4 Tuples and Nested Patterns 55 4.1 Tuples . 55 4.2 Nested patterns . 57 4.3 Exhaustiveness . 58 4.4 Wildcard (underscore) patterns . 58 4.5 Examples . 59 CIS 120 Lecture Notes Draft of March 22, 2016 4 CONTENTS 5 User-defined Datatypes 61 5.1 Atomic datatypes: enumerations . 61 5.2 Datatypes that carry more information . 63 5.3 Type abbreviations . 65 5.4 Recursive types: lists . 66 6 Binary Trees 69 7 Binary Search Trees 73 7.1 Creating Binary Search Trees . 75 8 Generic Functions and Datatypes 79 8.1 User-defined generic datatypes . 81 8.2 Why use generics? . 82 9 First-class Functions 83 9.1 Partial Application and Anonymous Functions . 84 9.2 List transformation . 86 9.3 List fold . 88 10 Modularity and Abstraction 91 10.1 A motivating example: finite sets . 91 10.2 Abstract types and modularity . 92 10.3 Another example: Finite Maps . 99 11 Partial Functions: option types 103 12 Unit and Sequencing Commands 107 12.1 The use of ‘;’................................. 108 13 Records of Named Fields 111 13.1 Immutable Records . 111 14 Mutable State and Aliasing 113 14.1 Mutable Records . 114 14.2 Aliasing: The Blessing and Curse of Mutable State . 117 15 The Abstract Stack Machine 121 15.1 Parts of the ASM . 122 15.2 Values and References to the Heap . 123 15.3 Simplification in the ASM . 126 15.4 Reference Equality . 134 CIS 120 Lecture Notes Draft of March 22, 2016 5 CONTENTS 16 Linked Structures: Queues 137 16.1 Representing Queues . 138 16.2 The Queue Invariants . 140 16.3 Implementing the basic Queue operations . 142 16.4 Iteration and Tail Calls . 144 16.5 Loop-the-loop: Examples of Iteration . 147 16.6 Infinite Loops . 149 17 Local State 151 17.1 Closures . 152 17.2 Objects . 153 17.3 The generic ’a ref type . 155 17.4 Reference (==) vs. Structural Equality (=)................. 156 18 Wrapping up OCaml: Designing a GUI Library 159 18.1 Taking Stock . 159 18.2 The Paint Application . 160 18.3 OCaml’s Graphics Library . 160 18.4 Design of the GUI Library . 162 18.5 Localizing Coordinates . 163 18.6 Simple Widgets & Layout . 167 18.7 The widget hierarchy and the run function . 170 18.8 The Event Loop . 171 18.9 GUI Events . 173 18.10Event Handlers . 173 18.11Stateful Widgets . 176 18.12Listeners and Notifiers . 177 18.13Buttons (at last!) . 180 18.14Building a GUI App: Lightswitch . 181 19 Transition to Java 185 19.1 Farewell to OCaml . 185 19.2 Three programming paradigms . 186 19.3 Functional Programming in OCaml . 187 19.4 Object-oriented programming in Java . 189 19.5 Imperative programming . 192 19.6 Types and Interfaces . 195 20 Connecting OCaml to Java 199 20.1 Core Java . 199 20.2 Static vs. Dynamic Methods . 201 CIS 120 Lecture Notes Draft of March 22, 2016 6 CONTENTS 21 Arrays 205 21.1 Arrays . 205 22 The Java ASM 211 22.1 Differences between OCaml and Java Abstract Stack Machines . 211 23 Subtyping, Extension and Inheritance 217 23.1 Interface Recap . 217 23.2 Subtyping . 219 23.3 Multiple Interfaces . 219 23.4 Interface Extension . 222 23.5 Inheritance . 223 23.6 Object .................................... 227 23.7 Static Types vs. Dynamic Classes . 227 24 The Java ASM and dynamic methods 231 24.1 Refinements to the Abstract Stack Machine . 232 24.2 The revised ASM in action . 233 25 Generics, Collections, and Iteration 247 25.1 Polymorphism and Generics . 248 25.2 Subtyping and Generics . 249 25.3 The Java Collections Framework . 250 25.4 Iterating over Collections . 253 26 Overriding and Equality 257 26.1 Method Overriding and the Java ASM . 257 26.2 Overriding and Equality . 262 26.2.1 When to override equals . 263 26.2.2 How to override equals . 264 26.3 Equals and subtyping . 268 26.3.1 Restoring symmetry . 269 27 Exceptions 271 27.1 Ways to handle failure . 272 27.2 Exceptions in Java . 273 27.3 Exceptions and the abstract stack machine . 274 27.4 Catching multiple exceptions . 275 27.5 Finally . 276 27.6 The Exception Class Hierarchy . 277 27.7 Checked exceptions . 278 27.8 Undeclared exceptions . 279 CIS 120 Lecture Notes Draft of March 22, 2016 7 CONTENTS 27.9 Good style for exceptions . 280 28 IO 283 28.1 Working with (Binary) Files . 284 28.2 PrintStream . 286 28.3 Reading text . 287 28.4 Writing text . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages359 Page
-
File Size-