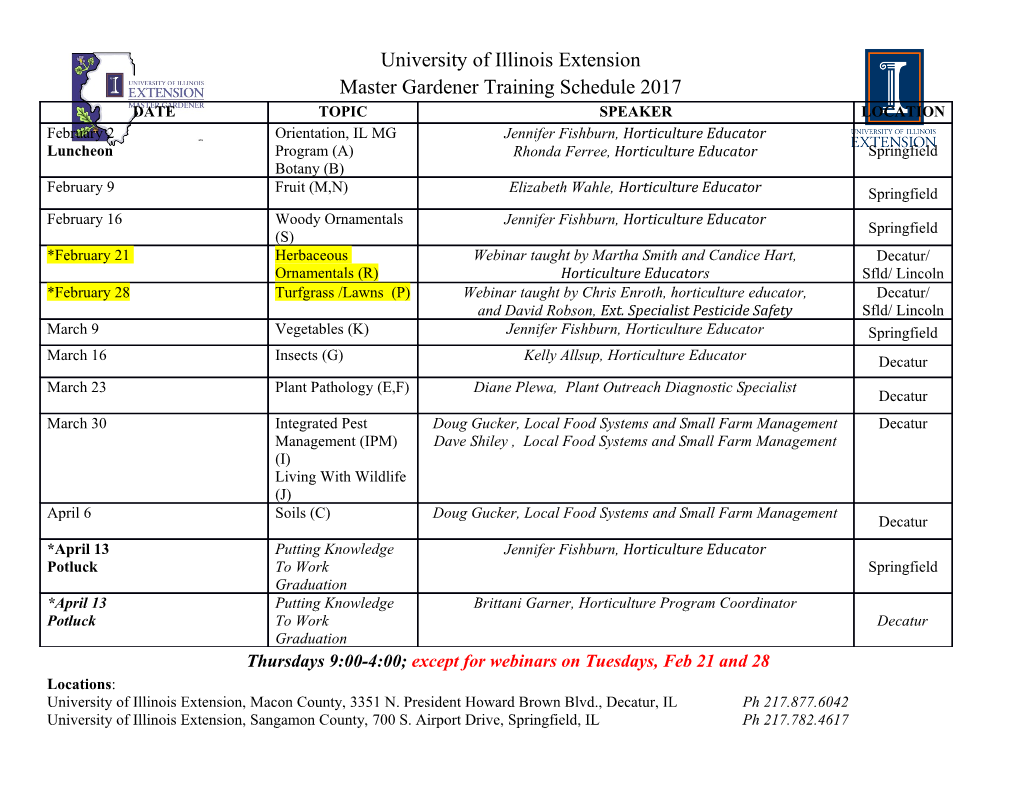
Python Programming for Economics and Finance Thomas J. Sargent & John Stachurski Sep 17, 2021 CONTENTS I Introduction to Python 3 1 About Python 5 1.1 Overview ................................................. 5 1.2 What’s Python? .............................................. 5 1.3 Scientific Programming .......................................... 7 1.4 Learn More ................................................ 15 2 Setting up Your Python Environment 17 2.1 Overview ................................................. 17 2.2 Anaconda ................................................. 17 2.3 Jupyter Notebooks ............................................ 18 2.4 Installing Libraries ............................................ 30 2.5 Working with Python Files ........................................ 31 2.6 Exercises ................................................. 32 3 An Introductory Example 35 3.1 Overview ................................................. 35 3.2 The Task: Plotting a White Noise Process ................................ 35 3.3 Version 1 ................................................. 36 3.4 Alternative Implementations ....................................... 40 3.5 Another Application ........................................... 44 3.6 Exercises ................................................. 45 3.7 Solutions ................................................. 47 4 Functions 53 4.1 Overview ................................................. 53 4.2 Function Basics .............................................. 54 4.3 Defining Functions ............................................ 55 4.4 Applications ............................................... 56 4.5 Exercises ................................................. 61 4.6 Solutions ................................................. 61 5 Python Essentials 65 5.1 Overview ................................................. 65 5.2 Data Types ................................................ 65 5.3 Input and Output ............................................. 69 5.4 Iterating .................................................. 70 5.5 Comparisons and Logical Operators ................................... 73 5.6 More Functions .............................................. 75 5.7 Coding Style and PEP8 .......................................... 78 i 5.8 Exercises ................................................. 78 5.9 Solutions ................................................. 80 6 OOP I: Introduction to Object Oriented Programming 85 6.1 Overview ................................................. 85 6.2 Objects .................................................. 86 6.3 Summary ................................................. 89 7 OOP II: Building Classes 91 7.1 Overview ................................................. 91 7.2 OOP Review ............................................... 92 7.3 Defining Your Own Classes ........................................ 93 7.4 Special Methods ............................................. 104 7.5 Exercises ................................................. 105 7.6 Solutions ................................................. 106 II The Scientific Libraries 109 8 Python for Scientific Computing 111 8.1 Overview ................................................. 111 8.2 Scientific Libraries ............................................ 112 8.3 The Need for Speed ........................................... 113 8.4 Vectorization ............................................... 115 8.5 Beyond Vectorization ........................................... 119 9 NumPy 121 9.1 Overview ................................................. 121 9.2 NumPy Arrays .............................................. 122 9.3 Operations on Arrays ........................................... 128 9.4 Additional Functionality ......................................... 131 9.5 Exercises ................................................. 134 9.6 Solutions ................................................. 135 10 Matplotlib 139 10.1 Overview ................................................. 139 10.2 The APIs ................................................. 140 10.3 More Features .............................................. 144 10.4 Further Reading ............................................. 149 10.5 Exercises ................................................. 149 10.6 Solutions ................................................. 149 11 SciPy 151 11.1 Overview ................................................. 151 11.2 SciPy versus NumPy ........................................... 152 11.3 Statistics ................................................. 152 11.4 Roots and Fixed Points .......................................... 155 11.5 Optimization ............................................... 158 11.6 Integration ................................................ 159 11.7 Linear Algebra .............................................. 159 11.8 Exercises ................................................. 160 11.9 Solutions ................................................. 160 12 Numba 161 12.1 Overview ................................................. 161 ii 12.2 Compiling Functions ........................................... 162 12.3 Decorators and “nopython” Mode .................................... 164 12.4 Compiling Classes ............................................ 166 12.5 Alternatives to Numba .......................................... 168 12.6 Summary and Comments ......................................... 169 12.7 Exercises ................................................. 170 12.8 Solutions ................................................. 171 13 Parallelization 173 13.1 Overview ................................................. 173 13.2 Types of Parallelization .......................................... 174 13.3 Implicit Multithreading in NumPy .................................... 175 13.4 Multithreaded Loops in Numba ..................................... 177 13.5 Exercises ................................................. 180 13.6 Solutions ................................................. 181 14 Pandas 183 14.1 Overview ................................................. 183 14.2 Series ................................................... 184 14.3 DataFrames ................................................ 186 14.4 On-Line Data Sources .......................................... 191 14.5 Exercises ................................................. 196 14.6 Solutions ................................................. 198 III Advanced Python Programming 205 15 Writing Good Code 207 15.1 Overview ................................................. 207 15.2 An Example of Poor Code ........................................ 207 15.3 Good Coding Practice .......................................... 211 15.4 Revisiting the Example .......................................... 213 15.5 Exercises ................................................. 215 15.6 Solutions ................................................. 218 16 More Language Features 221 16.1 Overview ................................................. 221 16.2 Iterables and Iterators ........................................... 222 16.3 Names and Name Resolution ....................................... 226 16.4 Handling Errors .............................................. 236 16.5 Decorators and Descriptors ........................................ 240 16.6 Generators ................................................ 246 16.7 Recursive Function Calls ......................................... 250 16.8 Exercises ................................................. 251 16.9 Solutions ................................................. 252 17 Debugging 255 17.1 Overview ................................................. 255 17.2 Debugging ................................................ 256 17.3 Other Useful Magics ........................................... 260 IV Other 261 18 Troubleshooting 263 iii 18.1 Fixing Your Local Environment ..................................... 263 18.2 Reporting an Issue ............................................ 264 19 Execution Statistics 265 Index 267 iv Python Programming for Economics and Finance This website presents a set of lectures on Python programming for economics and finance, designed and written by Thomas J. Sargent and John Stachurski. This is the first text in the series, which focuses on programming in Python. For an overview of the series, see this page • Introduction to Python – About Python – Setting up Your Python Environment – An Introductory Example – Functions – Python Essentials – OOP I: Introduction to Object Oriented Programming – OOP II: Building Classes • The Scientific Libraries – Python for Scientific Computing – NumPy – Matplotlib – SciPy – Numba – Parallelization – Pandas • Advanced Python Programming – Writing Good Code – More Language Features – Debugging • Other – Troubleshooting – Execution Statistics CONTENTS 1 Python Programming for Economics and Finance 2 CONTENTS Part I Introduction to Python 3 CHAPTER ONE ABOUT PYTHON Contents • About Python – Overview – What’s Python? – Scientific Programming – Learn More “Python has gotten sufficiently weapons grade that we don’t descend into R anymore. Sorry, R people. Iused to be one of you but we no longer descend into R.” – Chris Wiggins 1.1 Overview In this lecture we will • outline what Python is • showcase some of its abilities • compare it to some other languages. At this stage, it’s not our intention that you try to replicate all you see.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages274 Page
-
File Size-