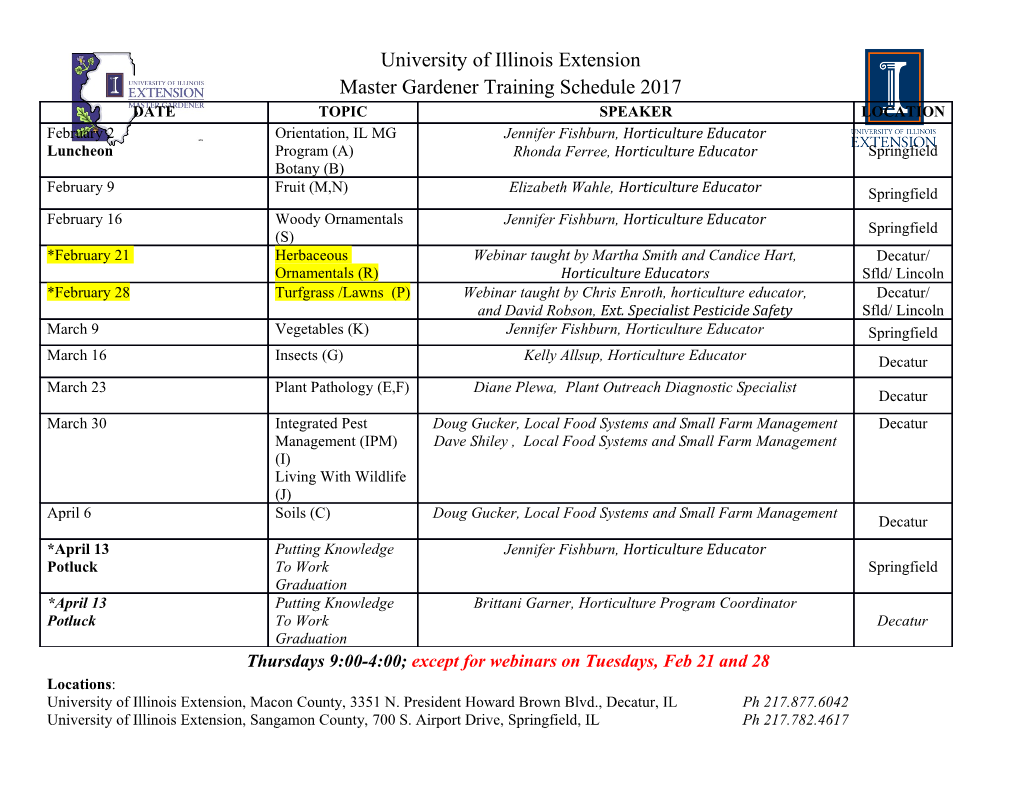
1 Client-Side Scripting with VBScript 2 Introduction • Visual Basic Script (VBScript) – Subset of Microsoft Visual Basic – IE contains VBScript scripting engine (interpreter) – Similar to JavaScript • JavaScript used more for client-side scripting – VBScript de facto language for ASP (Active Server Pages) 3 VBScript: Client Side Scripting VBScript was created to allow web page developers the ability to create dynamic web pages for their viewers who used Internet Explorer. With HTML, not a lot can be done to make a web page interactive, but VBScript unlocked many tools like: the ability to print the current date and time, access to the web servers file system, and allow advanced web programmers to develop web applications. 4 VBScript: Why Learn and Use It? VBScript is a prerequisite for ASP developers and should be learned thoroughly before attempting any sophisticated ASP programming. Programmers who have used Visual Basic in the past will feel more at home when designing their dynamic web pages with VBScript, but it should be known that only visitor's using Internet Explorer will be able to access your code, while other browsers will not be able to process the VBScript code. 5 Do's & Don'ts DO Learn VBScript – If you have a solid understanding of HTML – If you want to program successfully in ASP – If you already know Visual Basic and would like to experiment with VBScript – If you are a hobbyist that enjoys knowing many programming DON'T Learn VBScript – If you are developing a web page for the general public – If your site has visitors who use a browser other that Internet Explorer – If prefer to avoid dying languages. – If you want something more supported, you should learn Javascript. 6 VBScript Installation • VBScript is a client side scripting language that can be developed in any text editor. • An example of a text editor would be Notepad. • VBScript is a Microsoft proprietary language that does not work outside of Microsoft programs. VBScript Supported Browsers • After you have written your VBScript code you need the Internet Explorer to process your code. • Firefox, Opera, Netscape, etc will not be able to run VBScript. 7 VBScript First Script <html> <body> <script type="text/vbscript"> document.write("Hello World") </script> </body> </html> 8 VBScript <script> Tag • The HTML script tag lets the browser know we are going to use a script and the attribute type specifies which scripting language we are using. VBScript's type is "text/vbscript". • All the VBScript code that you write must be contained within script tags, otherwise they will not function properly. • No Semicolons! • VBScript Multiple Line Syntax Microsoft has included a special character for the multiple lines of code: the underscore "_". 9 VBScript Variables Variable is a named memory location used to hold a value that can be changed during the script execution. VBScript has only ONE fundamental data type, Variant. Rules for Declaring Variables: • Variable Name must begin with an alphabet. • Variable names cannot exceed 255 characters. • Variables Should NOT contain a period(.) • Variable Names should be unique in the declared context. 10 Declaring Variables Variables are declared using “dim” keyword. Since there is only ONE fundamental data type, all the declared variables are variant by default. Hence, a user NEED NOT mention the type of data during declaration. Example 1: In this Example, Var can be used as a String, Integer or even arrays. Dim Var Example 2: Two or more declarations are separated by comma(,) Dim Variable1,Variable2 11 Declaring Variables in VBScript <script type="text/vbscript"> Dim myVariable1 Dim myVariable2 Dim myVariable3, myVariable4 </script> <script type="text/vbscript"> Dim myVariable1, myVariable2 myVariable1 = 22 myVariable2 = "Howdy" document.write("My number is " & myVariable1) document.write("<br />My string is " & myVariable2) </script> 12 Assigning Values to the Variables Values are assigned similar to an algebraic expression. The variable name on the left hand side followed by an equal to (=) symbol and then its value on the right hand side. Rules : • The numeric values should be declared without double quotes. • The String values should be enclosed within doublequotes(") • Date and Time variables should be enclosed within hash symbol(#) 13 Examples ' Below Example, The value 25 is assigned to the variable. Value1 = 25 ' A String Value ‘VBScript’ is assigned to the variable StrValue. StrValue = “VBScript” ' The date 01/01/2020 is assigned to the variable DToday. Date1 = #01/01/2020# ' A Specific Time Stamp is assigned to a variable in the below example. Time1 = #12:30:44 PM# 14 Scope of the Variables Variables can be declared using the following statements that determines the scope of the variable. The scope of the variable plays a crucial role when used within a procedure or classes. • Dim • Public • Private 15 Scope of the Variables - Dim Variables declared using “Dim” keyword at a Procedure level are available only within the same procedure. Variables declared using “Dim” Keyword at script level are available to all the procedures within the same script. Example : In the below example, the value of Var1 and Var2 are declared at script level while Var3 is declared at procedure level. <!DOCTYPE html> 16 <html> <body> <script language="vbscript" type="text/vbscript"> Dim Var1 Dim Var2 Call add() Function add() Var1 = 10 Var2 = 15 Dim Var3 Var3 = Var1+Var2 Msgbox Var3 'Displays 25, the sum of two values. End Function Msgbox Var1 ' Displays 10 as Var1 is declared at Script level Msgbox Var2 ' Displays 15 as Var2 is declared at Script level Msgbox Var3 ' Var3 has No Scope outside the procedure. Prints Empty </script> </body> </html> 17 Scope of the Variables - PUBLIC Variables declared using "Public" Keyword are available to all the procedures across all the associated scripts. When declaring a variable of type "public", Dim keyword is replaced by "Public". Example : In the below example, Var1 and Var2 are available at script level while Var3 is available across the associated scripts and procedures as it is declared as Public. <!DOCTYPE html> 18 <html> <body> <script language="vbscript" type="text/vbscript"> Dim Var1 Dim Var2 Public Var3 Call add() Function add() Var1 = 10 Var2 = 15 Var3 = Var1+Var2 Msgbox Var3 'Displays 25, the sum of two values. End Function Msgbox Var1 ' Displays 10 as Var1 is declared at Script level Msgbox Var2 ' Displays 15 as Var2 is declared at Script level Msgbox Var3 ' Displays 25 as Var3 is declared as Public </script> </body> </html> 19 Scope of the Variables - PRIVATE Variables that are declared as "Private" have scope only within that script in which they are declared. When declaring a variable of type "Private", Dim keyword is replaced by "Private". Example : In the below example, Var1 and Var2 are available at Script Level. Var3 is declared as Private and it is available only for this particular script. Use of "Private" Variables is more pronounced within the Class. 20 <!DOCTYPE html> <html> <body> <script language="vbscript" type="text/vbscript"> Dim Var1 Dim Var2 Private Var3 Call add() Function add() Var1 = 10 Var2 = 15 Var3 = Var1+Var2 Msgbox Var3 'Displays the sum of two values. End Function Msgbox Var1 ' Displays 10 as Var1 is declared at Script level Msgbox Var2 ' Displays 15 as Var2 is declared at Script level Msgbox Var3 ' Displays 25 but Var3 is available only for this script. </script> </body> </html> 21 VBScript Constants Constant is a named memory location used to hold a value that CANNOT be changed during the script execution. If a user tries to change a Constant Value, the Script execution ends up with an error. Constants are declared the same way the variables are declared. 22 Declaring Constants Syntax: [Public | Private] Const Constant_Name = Value • The Constant can be of type Public or Private. • The Use of Public or Private is Optional. • The Public constants are available for all the scripts and procedures while the Private Constants are available within the procedure or Class. • One can assign any value such as number, String or Date to the declared Constant. 23 <!DOCTYPE html> <html> <body> <script language="vbscript" type="text/vbscript"> Dim intRadius intRadius = 20 const pi=3.14 Area = pi*intRadius*intRadius Msgbox Area </script> </body> </html> 24 Operators • VBScript – Not case-sensitive – Provides arithmetic operators, logical operators, concatenation operators, comparison operators and relational operators – Arithmetic operators • Similar to JavaScript arithmetic operators • Division operator – \ – Returns integer result • Exponentiation operator – ^ – Raises a value to a power 25 Operators • Arithmetic operators VBScript operation Arithmetic Algebraic VBScript operator expression expression Addition + x + y x + y Subtraction - z – 8 z – 8 Multiplication * yb y * b Division (floating-point) / v u or <Anchor0> v / u Division (integer) \ none u \ u p Exponentiation ^ q Q ^ p Negation - -e -e Modulus q mod r Mod q Mod r 26 Operators • Comparison operators Standard algebraic V BSc r i p t Example of Mea ning of VBScrip t equality operator or comparison V BSc r i p t c ond ition relational operator operator c ond ition = = d = g d is equal to g ≠ <> s <> r s is not equal to r > > y > x y is greater than x < < p < m p is less than m ≥ >= c >= z c is greater than or equal to z ≤ is less than or equal to <= m <= s m s 27 Operators – Comparison operators • Only symbols for equality operator (=) and inequality operator (<>) differ from JavaScript • Can also
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages72 Page
-
File Size-