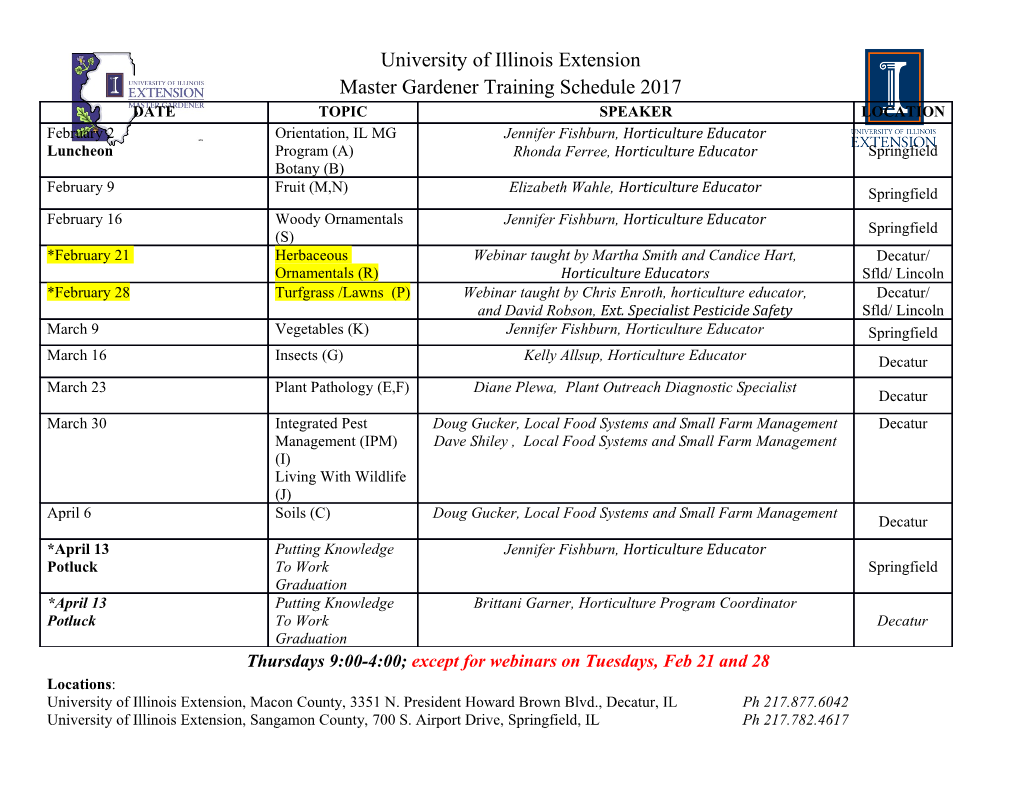
LEARNING TO PROGRAM WITH PYTHON Richard L. Halterman Copyright © 2011 Richard L. Halterman. All rights reserved. i Contents 1 The Context of Software Development 1 1.1 Software . 2 1.2 Development Tools . 2 1.3 Learning Programming with Python . 4 1.4 Writing a Python Program . 5 1.5 A Longer Python program . 8 1.6 Summary . 9 1.7 Exercises . 9 2 Values and Variables 11 2.1 Integer Values . 11 2.2 Variables and Assignment . 16 2.3 Identifiers . 19 2.4 Floating-point Types . 23 2.5 Control Codes within Strings . 24 2.6 User Input . 26 2.7 The eval Function . 27 2.8 Controlling the print Function . 29 2.9 Summary . 31 2.10 Exercises . 32 3 Expressions and Arithmetic 35 3.1 Expressions . 35 3.2 Operator Precedence and Associativity . 40 3.3 Comments . 41 3.4 Errors . 42 ©2011 Richard L. Halterman Draft date: November 13, 2011 CONTENTS ii 3.4.1 Syntax Errors . 42 3.4.2 Run-time Errors . 43 3.4.3 Logic Errors . 45 3.5 Arithmetic Examples . 46 3.6 More Arithmetic Operators . 49 3.7 Algorithms . 50 3.8 Summary . 51 3.9 Exercises . 53 4 Conditional Execution 57 4.1 Boolean Expressions . 57 4.2 Boolean Expressions . 58 4.3 The Simple if Statement . 59 4.4 The if/else Statement . 63 4.5 Compound Boolean Expressions . 65 4.6 Nested Conditionals . 68 4.7 Multi-way Decision Statements . 71 4.8 Conditional Expressions . 74 4.9 Errors in Conditional Statements . 76 4.10 Summary . 76 4.11 Exercises . 77 5 Iteration 81 5.1 The while Statement . 81 5.2 Definite Loops vs. Indefinite Loops . 86 5.3 The for Statement . 87 5.4 Nested Loops . 89 5.5 Abnormal Loop Termination . 92 5.5.1 The break statement . 92 5.5.2 The continue Statement . 95 5.6 Infinite Loops . 97 5.7 Iteration Examples . 100 5.7.1 Computing Square Root . 101 5.7.2 Drawing a Tree . 102 5.7.3 Printing Prime Numbers . 104 ©2011 Richard L. Halterman Draft date: November 13, 2011 CONTENTS iii 5.7.4 Insisting on the Proper Input . 107 5.8 Summary . 108 5.9 Exercises . 109 6 Using Functions 115 6.1 Introduction to Using Functions . 115 6.2 Standard Mathematical Functions . 120 6.3 time Functions . 123 6.4 Random Numbers . 125 6.5 Importing Issues . 128 6.6 Summary . 129 6.7 Exercises . 131 7 Writing Functions 133 7.1 Function Basics . 134 7.2 Using Functions . 139 7.3 Main Function . 141 7.4 Parameter Passing . 142 7.5 Function Examples . 144 7.5.1 Better Organized Prime Generator . 144 7.5.2 Command Interpreter . 145 7.5.3 Restricted Input . 146 7.5.4 Better Die Rolling Simulator . 148 7.5.5 Tree Drawing Function . 150 7.5.6 Floating-point Equality . 151 7.6 Custom Functions vs. Standard Functions . 153 7.7 Summary . 155 7.8 Exercises . 156 8 More on Functions 161 8.1 Global Variables . 161 8.2 Default Parameters . 166 8.3 Recursion . 167 8.4 Making Functions Reusable . 170 8.5 Documenting Functions and Modules . 172 ©2011 Richard L. Halterman Draft date: November 13, 2011 CONTENTS iv 8.6 Functions as Data . 174 8.7 Summary . 176 8.8 Exercises . 176 9 Lists 181 9.1 Using Lists . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages283 Page
-
File Size-