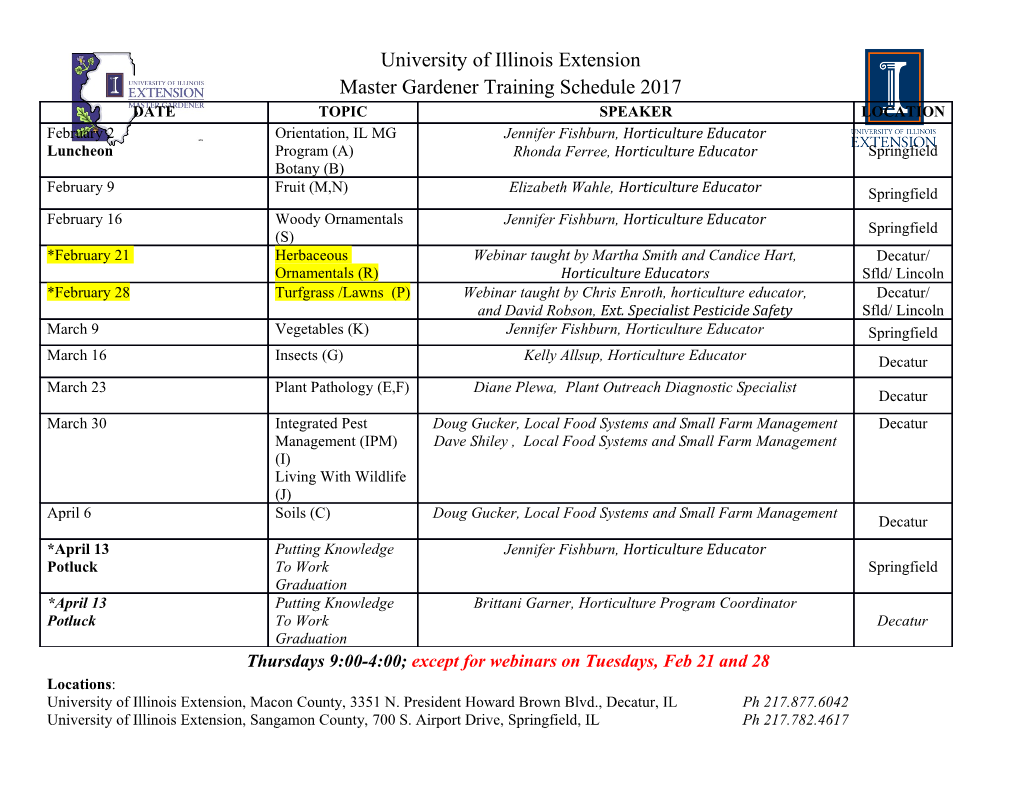
Effective Interprocedural Resource Leak Detection Emina Torlak Satish Chandra IBM T.J. Watson Research Center, USA {etorlak,satishchandra}@us.ibm.com ABSTRACT 1 public void test(File file, String enc) throws IOException f Garbage collection relieves programmers from the burden of 2 PrintWriter out= null; explicit memory management. However, explicit manage- 3 try f 4 try f ment is still required for finite system resources, such as I/O 5 out= new PrintWriter( streams, fonts, and database connections. Failure to release 6 new OutputStreamWriter( unneeded system resources results in resource leaks, which 7 new FileOutputStream(file), enc)); can lead to performance degradation and system crashes. 8 g catch (UnsupportedEncodingException ue) f In this paper, we present a new tool, Tracker, that per- 9 out= new PrintWriter(new FileWriter(file)); forms static analysis to find resource leaks in Java programs. 10 g 11 out.append('c'); Tracker is an industrial-strength tool that is usable in an 12 g catch (IOExceptione) f interactive setting: it works on millions of lines of code in 13 g finally f a matter of minutes and it has a low false positive rate. 14 if (out != null) f We describe the design, implementation and evaluation of 15 out.close(); Tracker, focusing on the features that make the tool scal- 16 g able and its output actionable by the user. 17 g 18 g Categories and Subject Descriptors Figure 1: Code example adapted from ant. D.2.4 [Software Engineering]: Software/Program Verifi- cation; D.2.5 [Software Engineering]: Testing and De- leading to performance degradation and system crashes. En- bugging suring that resources are always released, however, is tricky and error-prone. General Terms As an example, consider the Java program in Fig. 1. The allocation of a FileOutputStream on line 7 acquires a stream, Algorithms, Reliability, Verification which is a system resource that needs to be released by call- ing close() on the stream handle. The acquired stream ob- Keywords ject then passes into the constructor of OutputStreamWriter, resource leaks, alias analysis, inter-procedural analysis which remembers it in a private field. The OutputStream- Writer object, in turn, passes into the constructor of Print- 1. INTRODUCTION Writer. In the finally block, the programmer calls close() on the PrintWriter object. This close() method calls close() While garbage collection frees the programmer from the on the \nested" OutputStreamWriter object, which in turn responsibility of memory management, it does not help with calls close() on the nested FileOutputStream object. By using the management of finite system resources, such as sockets finally, it would appear that the program closes the stream, or database connections. When a program written in a Java- even in the event of an exception. like language acquires an instance of a finite system resource, However, a potential resource leak lurks in this code. The it must release that instance by explicitly calling a dispose constructor of OutputStreamWriter might throw an excep- or close method. Letting the last handle to an unreleased tion: notice that the programmer anticipates the possibil- resource go out of scope leaks the resource: the runtime sys- ity that an UnsupportedEncodingException may occur. If it tem gradually depletes the finite supply of system resources, does, the assignment to the variable out on line 5 will not execute, and consequently the stream allocated on line 7 is never closed. Permission to make digital or hard copies of all or part of this work for Resource management bugs are common in Java code for a personal or classroom use is granted without fee provided that copies are number of reasons. First, a programmer might omit a call to not made or distributed for profit or commercial advantage and that copies bear this notice and the full citation on the first page. To copy otherwise, to close() due to confusion over the role of the garbage collector. republish, to post on servers or to redistribute to lists, requires prior specific Second, even a careful programmer can easily fail to release permission and/or a fee. all resources along all possible exceptional paths, as illus- ICSE ’10, May 2-8 2010, Cape Town, South Africa trated in Fig. 1. Finally, a programmer needs to understand Copyright 2010 ACM 978-1-60558-719-6/10/05 ...$10.00. all relevant API contracts. In the example above, the pro- mistakes in using try-catch-finally blocks. A resource leakage grammer correctly reasoned that closing the PrintWriter in- tool therefore needs to pay special attention to bugs lurk- stance closes the nested resources through cascading close() ing there. Reporting leaks due to all possible runtime errors calls. But cascading close is not universal across all APIs, would overwhelm the user with uninteresting results, but we and a programmer could easily make incorrect assumptions. must deal with those exceptions that the programmer ex- pects to occur. For instance, a programmer would typically Contributions. In this paper we describe the design, im- ignore a leak report based on an OutOfMemoryError, because plementation and evaluation of a static analysis tool, called most programs are not expected to deal with such abnormal Tracker, for resource leak detection in Java programs. Our conditions. Instead, we present a belief-based [14] heuristic contribution is in overcoming the engineering challenges to that suppresses reports due to exceptional flow unless the building a useful, scalable leak detection tool. By useful, analysis finds plausible evidence to justify the report. we mean that the reports produced by the tool must be ac- tionable by a user, as opposed to merely comprehensive. By Summary of Results. We evaluated tracker on a suite of scalable, we mean that the tool must be able to handle real- 5 large open-source applications, ranging in size from 6,900 world Java applications consisting of tens of thousands of to 69,000 classes. All benchmarks were analyzed in a mat- classes. ter of minutes, with our tool identifying resource leaks at Some of the challenges that we address are common to all an actionable (true positive) rate of 84 to 100 percent. The static analysis tools and include the following: engineering techniques that we describe here are crucial for Scalable inter-procedural analysis. Inter-procedural actionability of the output produced by tracker: our ex- reasoning about aliases is crucial to building a useful re- ception filtering mechanism, for example, reduces the false source leak detection tool. Given the program in Fig. 1, positive rate by roughly 2:5×. In comparison to findbugs, for example, the tool needs to reason that the instance of tracker found 5 times more true positives. Our tool has FileOutputStream released in OutputStreamWriter's close() been used internally by IBM developers, who applied it on method is in fact the same instance that was attached to the real code and fixed most of the leaks it reported. A version OutputStreamWriter object when the latter was constructed. of tracker ships in Rational Software Analyzer version 7.1 The need for such reasoning can make it difficult to han- as a part of its Java static analysis suite. dle applications with tens of thousands of classes, as pro- grams of this size are not amenable to precise whole-program Outline. The remainder of this paper is organized as fol- alias analysis. Tracker side-steps the general alias analysis lows. Section 2 presents the core resource tracking algorithm problem by tracking pertinent aliasing information using ac- of tracker. Section 3 presents enhancements germane to cess paths [19] in the context of an efficient inter-procedural producing useful reports for such a tool. Section 4 presents data-flow analysis [21]. We observe, empirically, that sur- selected implementation details, especially how we construct prisingly sparse tracked aliasing information can accord high the call graph. Section 5 presents a detailed empirical eval- precision. uation. Section 6 reviews related work, and Section 7 con- False positives. False positives are inevitable in any tool cludes the paper. that guarantees soundness. However, if a bug-finding tool produces too many false positives, the average user will sim- ply ignore its results. Building a bug-finding tool as opposed 2. CORE ALGORITHM FOR RESOURCE to a verification tool (whose goal is to determine whether or TRACKING not the code is free from a certain class of errors) opens In this section, we first give a quick overview of how the up the possibility|and creates an expectation|of prioritiz- basic analysis works as applied to Fig. 1. Subsequently, we ing reports that are likelier than others to be true positives. give formal details of the core intra-procedural and inter- Tracker offers such prioritization by keeping a record of procedural resource tracking algorithms. whether a bug was witnessed along some path, or whether it was assumed due to the limitations of the analysis. We 2.1 Overview show, empirically, that bugs arising from analysis limitations The analysis takes as input a control-flow graph (CFG) are much more likely to be false positives. and a specification of procedures that acquire and release We also tackle issues that are specific to the problem of system resources. Figure 2 shows the relevant parts of the resource management: CFG for Fig. 1, along with the CFGs of some of the called Nested resources. It is not enough to track just sys- methods. We introduced temporary variables t1 and t2 tem resources, because the corrective action that a user when constructing the CFG (a). The example's specification needs to take may be to call a close method on an object declares that the constructor for FileOutputStream allocates that nests a resource instance.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages10 Page
-
File Size-