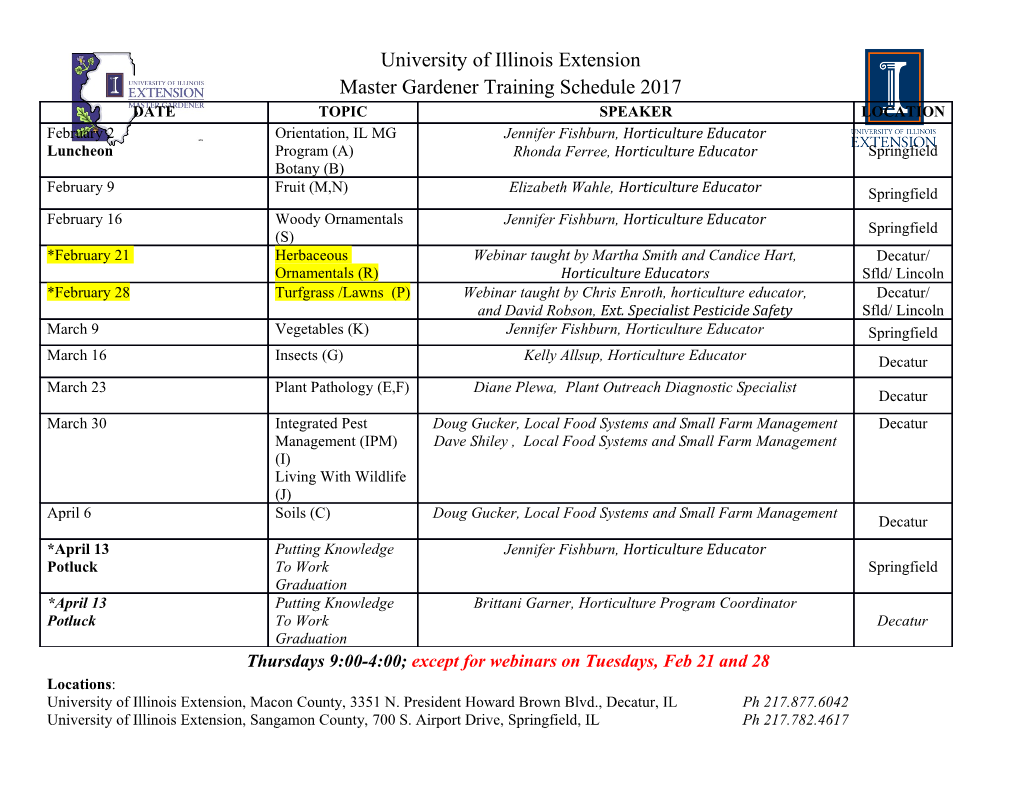
A category theoretic introduction to computer science Thomas Wright June 2016 The wrong answer: Computer science is the study of computers. A better answer: Computer science is the study of computation. What is computer science? A better answer: Computer science is the study of computation. What is computer science? The wrong answer: Computer science is the study of computers. What is computer science? The wrong answer: Computer science is the study of computers. A better answer: Computer science is the study of computation. Part I An abstract theory of computation Example: computing a mathematical function The successor function: f : N0 ! N0 : x 7! x + 1 We can compute this using a computer program written in, for example, C: intf(intn){ returnn+1; } Example: computing a mathematical function We could however have written the same program in a different language: Python: Matlab: deff(n): functionm=f(n) returnn+1 m=n+1 end We can use the following 3 rules to define when two expressions are equivalent: ∼ λx:f =α λy:f [y=x] ∼ (λx:f )g =β f [g=x] ∼ (λx:fx) =η f It turns out the definitions on this slide are enough to give a foundation for both mathematics and computation. Example: Defining and computing with natural numbers The λ-calculus We can express such functions as λ-terms, which can have form: x fg λx:f where x is a variable and f and g are themselves λ-terms. Example: Defining and computing with natural numbers The λ-calculus We can express such functions as λ-terms, which can have form: x fg λx:f where x is a variable and f and g are themselves λ-terms. We can use the following 3 rules to define when two expressions are equivalent: ∼ λx:f =α λy:f [y=x] ∼ (λx:f )g =β f [g=x] ∼ (λx:fx) =η f It turns out the definitions on this slide are enough to give a foundation for both mathematics and computation. The λ-calculus We can express such functions as λ-terms, which can have form: x fg λx:f where x is a variable and f and g are themselves λ-terms. We can use the following 3 rules to define when two expressions are equivalent: ∼ λx:f =α λy:f [y=x] ∼ (λx:f )g =β f [g=x] ∼ (λx:fx) =η f It turns out the definitions on this slide are enough to give a foundation for both mathematics and computation. Example: Defining and computing with natural numbers That is, we treat integers as fundamental and take them to form a primitive type Int. We also take booleans (which can take value either true or false) to be a primitive type Bool. From these primitive types, we can form compound types: A ! BA × B We also add operations to our language for working with each of these types: +, ∗, h·; ·i, fst, snd Adding types To make the λ-calculus more useful, it helps if we avoid unravelling the definition of a natural number just to say 1 + 1 = 2. We then need to move beyond treating everything as a function, and allow values to take different types. From these primitive types, we can form compound types: A ! BA × B We also add operations to our language for working with each of these types: +, ∗, h·; ·i, fst, snd Adding types To make the λ-calculus more useful, it helps if we avoid unravelling the definition of a natural number just to say 1 + 1 = 2. We then need to move beyond treating everything as a function, and allow values to take different types. That is, we treat integers as fundamental and take them to form a primitive type Int. We also take booleans (which can take value either true or false) to be a primitive type Bool. Adding types To make the λ-calculus more useful, it helps if we avoid unravelling the definition of a natural number just to say 1 + 1 = 2. We then need to move beyond treating everything as a function, and allow values to take different types. That is, we treat integers as fundamental and take them to form a primitive type Int. We also take booleans (which can take value either true or false) to be a primitive type Bool. From these primitive types, we can form compound types: A ! BA × B We also add operations to our language for working with each of these types: +, ∗, h·; ·i, fst, snd The λ-calculus as category theory It turns out that the λ-calculus forms a category: The objects are types. The arrows are λ-terms upto equivalence e.g. Hom(A; B) consists of all equivalence classes [f ] of λ-terms f of type A ! B. 1A = [λx:x] (where x : A) [f ] ◦ [g] = [λx:f (gx)] (Verify axioms) Question: What would coproduct types look like? The λ-calculus as category theory C a (a;b) b ~ ! AAo × B / B pA=λz:fst(z) pB =λz:snd(z) where (a; b) = λc:hac; bci The λ-calculus as category theory C a (a;b) b ~ ! AAo × B / B pA=λz:fst(z) pB =λz:snd(z) where (a; b) = λc:hac; bci Question: What would coproduct types look like? More surprisingly, any cartesian closed category arises from the simply typed λ-calculus. The theory of cartesian closed categories is exactly the simply typed λ-calculus. The λ-calculus as category theory We can also extend our type system with a unit type Unit which contains only the value hi (the empty product type); this provides the category of types with a terminal object. Then the category of types of the λ-calculus is cartesian closed: Definition A category C is cartesian closed if it has all finite products and all exponentials. The λ-calculus as category theory We can also extend our type system with a unit type Unit which contains only the value hi (the empty product type); this provides the category of types with a terminal object. Then the category of types of the λ-calculus is cartesian closed: Definition A category C is cartesian closed if it has all finite products and all exponentials. More surprisingly, any cartesian closed category arises from the simply typed λ-calculus. The theory of cartesian closed categories is exactly the simply typed λ-calculus. Part II Computation with the messy bits left in Surprise: programming is difficult Actually, there is more to programming than we have hinted at. Studying programs upto βη-equivalence gives a theory of computable functions. However real computer programs do many things not captured by a theory of functions: • Crash • Loop forever • Act randomly • Input and output data • Change global state We need to extend out theory of computation in order to capture this behaviour; we can do this using monads. Monads A monad over a category C is a triple (T ; η; µ) where T : C!C is 2 a functor, η : 1C ! T and µ : T ! T are natural transformations making the following diagrams commute: µTA µ T µ T 3A / T 2A TA / 2 o A TA^ T A TA@ 1TA 1TA T µA µA µA T 2A / TA µA TA Kleisli triples A Kleisli triple over a category C is a triple (T ; µ, ∗) where T : Obj(C) ! Obj(C), νA : A ! TA for A 2 Obj(C), and f ∗ : TA ! TB for f : A ! TB and the following equations hold: ∗ • µA = 1TA; ∗ • f ◦ µA = f for f : A ! TB; • g ∗ ◦ f ∗ = (g ∗ ◦ f )∗ Notions of computation Using a Kleisli triple to define a monad gives a model of computation: • T is a notion of computation; it takes types of values to types of computations. • The unit µA takes a value of type A to a program which computes this value. • Given a function f on values, we extend it to a function f ∗ on computations by applying the function to the result of the computation. Then the programs in this model come from the Kleisli category CT of the monad. Partial computation • TA = A + f?g where ? denotes the diverging computation (that is, the program breaking). • µA is inclusion. • if f : A ! TB then f ∗(?) = ? f ∗(a) = f (a) Nondeterminism • TA = Pfin(A). • µA(a) = fag. • if f : A ! TB and c 2 TA then [ f ∗(c) = f (a) x2c Side-effects • TA = (A × S)S . • µA(a) = λs : S:ha; si. • if f : A ! TB and c 2 TA then f ∗ evaluates f given stored data s and updates the store. Part III Haskell: a category theory based programming language Introducing Haskell Haskell takes these models of computation and turns them into an actual programming language. Successor: Hello world: f:: Int -> Int main::IO() fn=n+1 main= putStrLn "Hello, World!" Sources on Monads in Haskell: • http://learnyouahaskell.com/input-and-output • https://www.schoolofhaskell.com/school/ starting-with-haskell/basics-of-haskell/ the-tao-of-monad.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages28 Page
-
File Size-