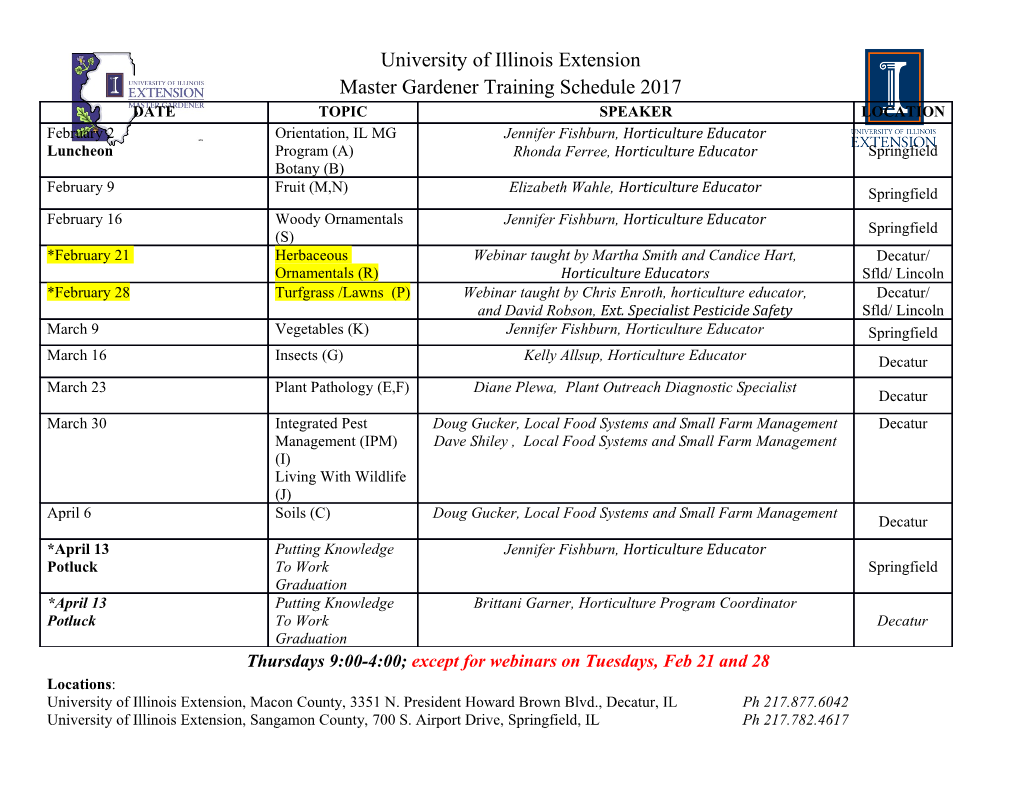
Swift Language #swift Table of Contents About 1 Chapter 1: Getting started with Swift Language 2 Remarks 2 Other Resources 2 Versions 2 Examples 3 Your first Swift program 3 Installing Swift 4 Your first program in Swift on a Mac (using a Playground) 5 Your first program in Swift Playgrounds app on iPad 9 Optional Value and Optional enum 10 Chapter 2: (Unsafe) Buffer Pointers 12 Introduction 12 Remarks 12 Examples 12 UnsafeMutablePointer 12 Practical Use-Case for Buffer Pointers 13 Chapter 3: Access Control 15 Syntax 15 Remarks 15 Examples 15 Basic Example using a Struct 15 Car.make (public) 16 Car.model (internal) 16 Car.otherName (fileprivate) 16 Car.fullName (private) 16 Subclassing Example 17 Getters and Setters Example 17 Chapter 4: Advanced Operators 18 Examples 18 Custom Operators 18 Overloading + for Dictionaries 19 Commutative Operators 20 Bitwise Operators 20 Overflow Operators 21 Precedence of standard Swift operators 22 Chapter 5: AES encryption 24 Examples 24 AES encryption in CBC mode with a random IV (Swift 3.0) 24 AES encryption in CBC mode with a random IV (Swift 2.3) 27 AES encryption in ECB mode with PKCS7 padding 28 Chapter 6: Algorithms with Swift 30 Introduction 30 Examples 30 Insertion Sort 30 Sorting 30 Selection sort 33 Asymptotic analysis 34 Quick Sort - O(n log n) complexity time 35 Graph, Trie, Stack 36 Graph 36 Trie 43 Stack 46 Chapter 7: Arrays 50 Introduction 50 Syntax 50 Remarks 50 Examples 50 Value Semantics 50 Basics of Arrays 50 Empty arrays 51 Array literals 51 Arrays with repeated values 51 Creating arrays from other sequences 51 Multi-dimensional arrays 51 Accessing Array Values 52 Useful Methods 53 Modifying values in an array 53 Sorting an Array 53 Creating a new sorted array 54 Sorting an existing array in place 54 Sorting an array with a custom ordering 54 Transforming the elements of an Array with map(_:) 55 Extracting values of a given type from an Array with flatMap(_:) 56 Filtering an Array 56 Filtering out nil from an Array transformation with flatMap(_:) 56 Subscripting an Array with a Range 57 Grouping Array values 58 Flattening the result of an Array transformation with flatMap(_:) 58 Combining the characters in an array of strings 59 Flattening a multidimensional array 59 Sorting an Array of Strings 59 Lazily flattening a multidimensional Array with flatten() 60 Combining an Array's elements with reduce(_:combine:) 61 Removing element from an array without knowing it's index 61 Swift3 61 Finding the minimum or maximum element of an Array 62 Finding the minimum or maximum element with a custom ordering 62 Accessing indices safely 63 Comparing 2 Arrays with zip 63 Chapter 8: Associated Objects 65 Examples 65 Property, in a protocol extension, achieved using associated object. 65 Chapter 9: Blocks 69 Introduction 69 Examples 69 Non-escaping closure 69 Escaping closure 69 Chapter 10: Booleans 71 Examples 71 What is Bool? 71 Negate a Bool with the prefix ! operator 71 Boolean Logical Operators 71 Booleans and Inline Conditionals 72 Chapter 11: Caching on disk space 74 Introduction 74 Examples 74 Saving 74 Reading 74 Chapter 12: Classes 75 Remarks 75 Examples 75 Defining a Class 75 Reference Semantics 75 Properties and Methods 76 Classes and Multiple Inheritance 77 deinit 77 Chapter 13: Closures 78 Syntax 78 Remarks 78 Examples 78 Closure basics 78 Syntax variations 79 Passing closures into functions 80 Trailing closure syntax 80 @noescape parameters 80 Swift 3 note: 81 throws and rethrows 81 Captures, strong/weak references, and retain cycles 82 Retain cycles 83 Using closures for asynchronous coding 83 Closures and Type Alias 84 Chapter 14: Completion Handler 85 Introduction 85 Examples 85 Completion handler with no input argument 85 Completion handler with input argument 85 Chapter 15: Concurrency 87 Syntax 87 Examples 87 Obtaining a Grand Central Dispatch (GCD) queue 87 Running tasks in a Grand Central Dispatch (GCD) queue 89 Concurrent Loops 90 Running Tasks in an OperationQueue 91 Creating High-Level Operations 93 Chapter 16: Conditionals 95 Introduction 95 Remarks 95 Examples 95 Using Guard 95 Basic conditionals: if-statements 96 The logical AND operator 96 The logical OR operator 96 The logical NOT operator 97 Optional binding and "where" clauses 97 Ternary operator 98 Nil-Coalescing Operator 99 Chapter 17: Cryptographic Hashing 100 Examples 100 MD2, MD4, MD5, SHA1, SHA224, SHA256, SHA384, SHA512 (Swift 3) 100 HMAC with MD5, SHA1, SHA224, SHA256, SHA384, SHA512 (Swift 3) 101 Chapter 18: Dependency Injection 104 Examples 104 Dependency Injection with View Controllers 104 Dependenct Injection Intro 104 Example Without DI 104 Example with Dependancy Injection 105 Dependency Injection Types 108 Example Setup without DI 108 Initializer Dependency Injection 109 Properties Dependency Injection 110 Method Dependency Injection 110 Chapter 19: Design Patterns - Creational 111 Introduction 111 Examples 111 Singleton 111 Factory Method 111 Observer 112 Chain of responsibility 114 Iterator 115 Builder Pattern 116 Example: 116 Take it Further: 119 Chapter 20: Design Patterns - Structural 123 Introduction 123 Examples 123 Adapter 123 Facade 123 Chapter 21: Dictionaries 125 Remarks 125 Examples 125 Declaring Dictionaries 125 Modifying Dictionaries 125 Accessing Values 126 Change Value of Dictionary using Key 127 Get all keys in Dictionary 127 Merge two dictionaries 127 Chapter 22: Documentation markup 128 Examples 128 Class documentation 128 Documentation styles 128 Chapter 23: Enums 133 Remarks 133 Examples 133 Basic enumerations 133 Enums with associated values 134 Indirect payloads 135 Raw and Hash values 135 Initializers 136 Enumerations share many features with classes and structures 137 Nested Enumerations 138 Chapter 24: Error Handling 140 Remarks 140 Examples 140 Error handling basics 140 Catching different error types 141 Catch and Switch Pattern for Explicit Error Handling 142 Disabling Error Propagation 143 Create custom Error with localized description 143 Chapter 25: Extensions 145 Remarks 145 Examples 145 Variables and functions 145 Initializers in Extensions 145 What are Extensions? 146 Protocol extensions 146 Restrictions 147 What are extensions and when to use them 147 Subscripts 148 Chapter 26: Function as first class citizens in Swift 149 Introduction 149 Examples 149 Assigning function to a variable 149 Passing function as an argument to another function, thus creating a Higher-Order Function 150 Function as return type from another function 150 Chapter 27: Functional Programming in Swift 151 Examples 151 Extracting a list of names from a list of Person(s) 151 Traversing 151 Projecting 151 Filtering 152 Using Filter with Structs 153 Chapter 28: Functions 155 Examples 155 Basic Use 155 Functions with Parameters 155 Returning Values 156 Throwing Errors 157 Methods 157 Instance Methods 157 Type Methods 158 Inout Parameters 158 Trailing Closure Syntax 158 Operators are Functions 158 Variadic Parameters 159 Subscripts 160 Subscripts Options: 160 Functions With Closures 161 Passing and returning functions 162 Function types 162 Chapter 29: Generate UIImage of Initials from String 164 Introduction 164 Examples 164 InitialsImageFactory 164 Chapter 30: Generics 166 Remarks 166 Examples 166 Constraining Generic Placeholder Types 166 The Basics of Generics 167 Generic Functions 167 Generic Types 167 Passing Around Generic Types 168 Generic Placeholder Naming 168 Generic Class Examples 168 Generic Class Inheritance 170 Using Generics to Simplify Array Functions 170 Use generics to enhance type-safety 171 Advanced Type Constraints 171 Chapter 31: Getting Started with Protocol Oriented Programming 173 Remarks 173 Examples 173 Leveraging Protocol Oriented Programming for Unit Testing 173 Using protocols as first class types 174 Chapter 32: Initializers 179 Examples 179 Setting default property values 179 Customizing initialization with paramaters 179 Convenience init 180 Designated Initializer 183 Convenience init() 183 Convenience init(otherString: String) 183 Designated Initializer (will call the superclass Designated Initializer) 184 Convenience init() 184 Throwable Initilizer 184 Chapter 33: Logging in Swift 186 Remarks 186 Examples 186 Debug Print 186 Updating a classes debug and print values 187 dump 187 print() vs dump() 188 print vs NSLog 189 Chapter 34: Loops 191 Syntax 191 Examples 191 For-in loop 191 Iterating over a range 191 Iterating over an array or set 191 Iterating over a dictionary 192 Iterating in reverse 192 Iterating over ranges with custom stride 193 Repeat-while loop 194 while loop 194 Sequence Type forEach block 194 For-in loop with filtering 195 Breaking a loop 196 Chapter 35: Memory Management 197 Introduction 197 Remarks 197 When to use the weak-keyword: 197 When to use the unowned-keyword: 197 Pitfalls 197 Examples 198 Reference Cycles and Weak References 198 Weak References 198 Manual Memory Management 199 Chapter 36: Method Swizzling 201 Remarks 201 Links 201 Examples 201 Extending UIViewController and Swizzling viewDidLoad 201 Basics of Swift Swizzling 202 Basics of Swizzling - Objective-C 203 Chapter 37: NSRegularExpression in Swift 205 Remarks 205 Examples 205 Extending String to do simple pattern matching 205 Basic Usage 206 Replacing Substrings 207 Special Characters 207 Validation 207 NSRegularExpression for mail validation 208 Chapter 38: Numbers 209 Examples 209 Number types and literals 209 Literals 209 Integer literal syntax 209 Floating-point literal syntax 210 Convert one numeric type to another 210 Convert numbers to/from strings 211
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages353 Page
-
File Size-