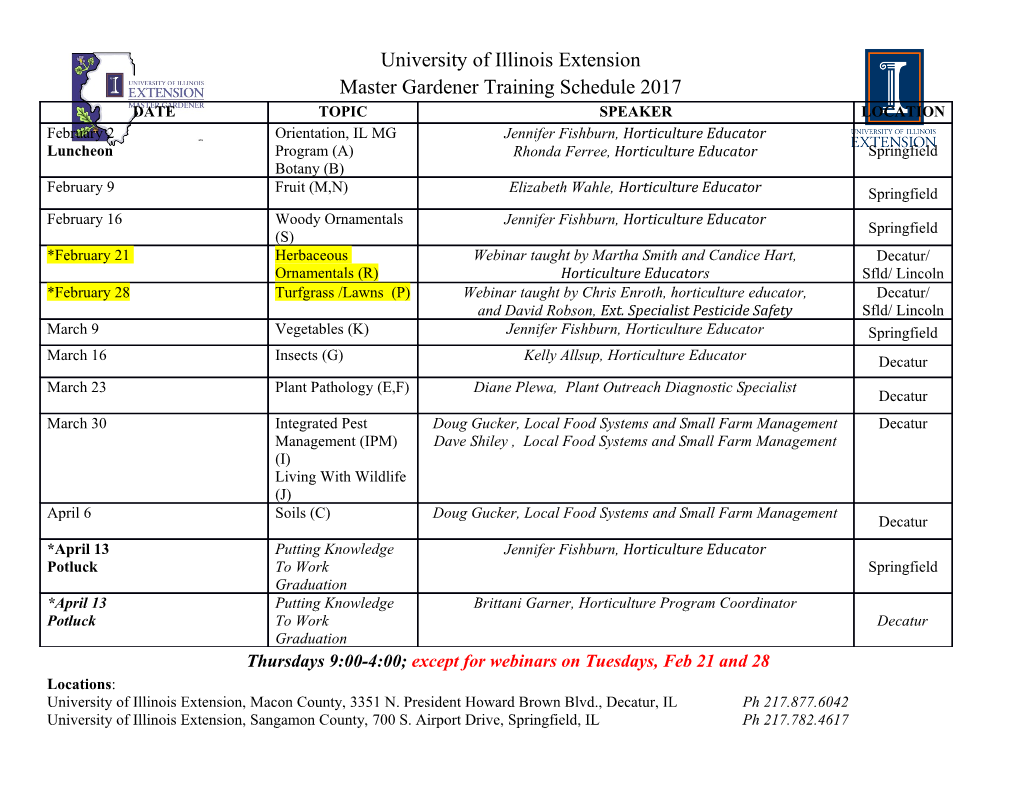
13/02/2017 Basics of HTML Canvas Material taken from http://www.w3schools.com CSCU9B2 CSCU9B2 1 We are going to cover • What HTML canvas is, and what it can do • Most commonly used canvas methods • Example of a simple animated application CSCU9B2 2 1 13/02/2017 What is Canvas? Canvas is a medium for oil painting, typically stretched across a wooden frame. What is HTML Canvas? • HTML canvas is about drawing graphics • There is a set of JavaScript methods (APIs) for drawing graphics (lines, boxes, circles, shapes). • HTML canvas is a rectangular area on a web page, specified with the <canvas> element. • The HTML <canvas> element (introduced in HTML5) is a container for HTML graphics. CSCU9B2 4 2 13/02/2017 What can HTML canvas do? • Draw colorful text • Draw graphical shapes • Can be animated. Everything is possible: from simple bouncing balls to complex animations • Can be interactive and respond to events • Offer lots of possibilities for HTML gaming applications CSCU9B2 5 Examples http://www.effectgames.com/demos/canvascycle/ http://hakim.se/experiments/html5/blob/03/ http://bomomo.com/ http://hakim.se/experiments/html5/magnetic/02/ http://worldsbiggestpacman.com/ CSCU9B2 6 3 13/02/2017 Canvas element Looks like this: <canvas id="myCanvas" width="200" height="100"></canvas> • Must have an id attribute so it can be referred to by JavaScript; • The width and height attribute is necessary to define the size of the canvas. CSCU9B2 7 Drawing on the Canvas All drawing on the HTML canvas must be done with JavaScript in three steps: 1. Find the canvas element 2. Create a drawing object 3. Draw on the canvas E.g. <canvas id="myCanvas" width="200" height="100"></canvas> var canvas = document.getElementById("myCanvas"); var ctx = canvas.getContext("2d"); ctx.fillStyle = "#FF0000"; ctx.fillRect(0,0,150,75); CSCU9B2 8 4 13/02/2017 HTML Canvas Coordinates • The HTML canvas is two-dimensional. • The upper-left corner of the canvas has the coordinates (0,0) • In the previous example, you saw the method: fillRect(0,0,150,75) • This means: Start at the upper-left corner (0,0) and draw a 150 x 75 pixels rectangle. CSCU9B2 9 Canvas – fillStyle and strokeStyle • The fillStyle property is used to define a fill-color (or gradient) for the drawing. • The strokeStyle defines the color of the line around the drawing. • E.g. ctx.fillRect(20,20,150,50); ctx.fillStyle="#FF0000"; ctx.strokeRect(20,20,200,100); ctx.strokeStyle = “blue”; CSCU9B2 10 5 13/02/2017 Canvas - Gradients • Gradients can be used to fill rectangles, circles, lines, text, etc. • Two different types of gradient: • createLinearGradient(x,y,x1,y1) • createRadialGradient(x,y,r,x1,y1,r1) • http://www.w3schools.com/css/css3_gradients.asp • Need to specify two or more color "stops“ • Eg start and finish colours • To use the gradient, set the fillStyle or strokeStyle property to the gradient CSCU9B2 11 Example of linear gradient Create a linear gradient. Fill rectangle with the gradient: var c=document.getElementById("myCanvas"); var ctx=c.getContext("2d"); // Create gradient var grd=ctx.createLinearGradient(0,0,200,0); grd.addColorStop(0,"red"); grd.addColorStop(1,"white"); // Fill with gradient ctx.fillStyle=grd; ctx.fillRect(25,25,150,75); CSCU9B2 12 6 13/02/2017 Draw a Line To draw a straight line on a canvas, you use these methods: – moveTo(x,y) defines the starting point of the line – lineTo(x,y) defines the ending point of the line – stroke() method draws the line E.g. var canvas = document.getElementById("myCanvas"); var ctx = canvas.getContext("2d"); ctx.moveTo(0,0); ctx.lineTo(200,100); ctx.stroke(); CSCU9B2 13 Draw a Circle To draw a circle on a canvas, you use the following methods: – beginPath(); – arc(x,y,r,start,stop) Eg. var canvas = document.getElementById("myCanvas"); var ctx = canvas.getContext("2d"); ctx.beginPath(); ctx.arc(95,50,40,0,2*Math.PI); ctx.stroke(); CSCU9B2 14 7 13/02/2017 Drawing Text on the Canvas To draw text on a canvas, the most important property and methods are: font - defines the font properties for the text textAlign – Aligns the text. Possible values: start, end, left, right, center strokeText(text,x,y) - Draws text on the canvas fillText(text,x,y) - Draws "filled" text on the canvas E.g., Write the text in orange with a blue border around the text ctx.font = "italic bold 56px Arial, sans-serif"; ctx.fillStyle="orange"; ctx.textAlign = "start"; ctx.fillText("Some text", 10, 50); ctx.lineWidth = 3; ctx.strokeStyle="blue"; ctx.strokeText("Some text", 10, 50); CSCU9B2 15 Canvas Images To draw an image on a canvas, use the following method: – drawImage(image,x,y,w,h) E.g. var myimage = new Image(); myimage.src = "image2.jpeg"; ctx.drawImage(myimage, 20, 20, 100, 100); CSCU9B2 16 8 13/02/2017 Animation – Changing things over time Animation on a canvas is achieved by: 1. Defining drawing operations in a function a) Clear the canvas b) Draw objects slightly differently from previous time, e.g. location, size, rotation 2. Call function repeatedly at a defined time interval setInterval(animate, 30); CSCU9B2 17 A simple animation - example <canvas id="my_canvas" width="800" height="700"> <script> var ctx = document.getElementById("my_canvas").getContext("2d"); var cW = ctx.canvas.width; var cH = ctx.canvas.height; var y = 0, x=0; function animate() { ctx.save(); ctx.clearRect(0, 0, cW, cH); ctx.rotate(-0.3); ctx.fillStyle="green"; ctx.fillRect(0, y, 50, 50); y++; ctx.rotate(0.8); ctx.fillStyle=”red”; ctx.fillRect(x, 0, 50, 50); x++; ctx.restore(); } var animateInterval = setInterval(animate, 30); ctx.canvas.addEventListener('click', function(event){ clearInterval(animateInterval);}); </script> CSCU9B2 18 9 13/02/2017 Animation using CSS • Animations can be created with CSS • Let an element gradually change styles • Color, position or any other property • Transitions • Key frames • Style values at a particular time point • Timing CSCU9B2 19 CSS Transitions - example <html> <head> <style> div { width: 100px; height: 100px; background: red; transition: width 2s, height 2s, transform 2s; } div:hover { width: 300px; height: 300px; transform: rotate(180deg); } </style> </head> <body> <div><p>Some text.</p></div> </body> </html> CSCU9B2 20 10 13/02/2017 CSS Keyframes - example <html> <head> <style> div { width: 100px; height: 100px; background-color: red; animation-name: example; animation-duration: 4s; } @keyframes example { from {background-color: red; width: 100px;} to {background-color: yellow; width: 200px;} } </style> </head> <body> <div></div> </body> </html> CSCU9B2 21 Web Technologies The End… CSCU9B2 22 11 .
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages11 Page
-
File Size-