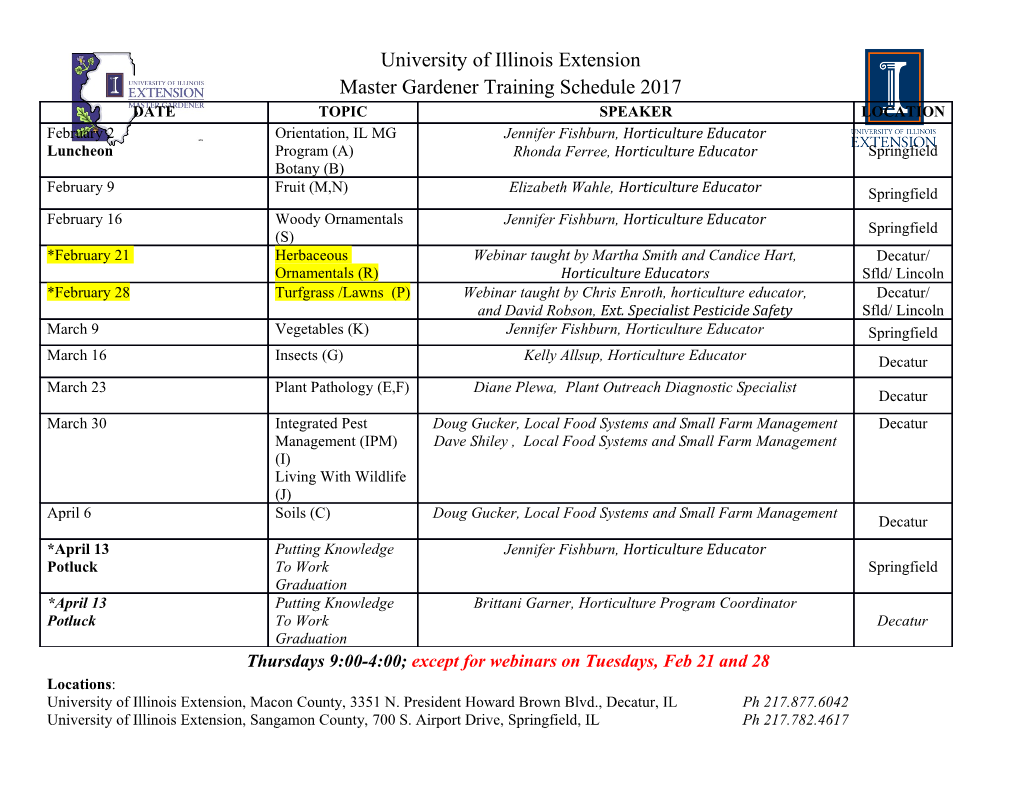
Functional Languages CS345H: Programming Languages I All languages we have studies so far were variants of lambda calculus Lecture 14: Introduction to Imperative Languages I Such languages are known as functional languages Thomas Dillig I We have also seen that these languages allow us to design powerful type systems I And even perform type inference Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 1/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 2/31 Salient Features of Functional Languages No Side Effects I The functional languages we studied have a set of defining features: I No side effects means no assignments and no variables! I Most noticeable feature: No side effects! I Recall: Let-bindings are only names for values I This means that evaluating an expression never changes the value of any other expression I The value they stand for can never change I Example: I Example: let x = 3+4 in let y = x+5 in x+y let x = 3 in let x = 4 in x I Here, evaluating the expression x+5 cannot change the value of any other expression Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 3/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 4/31 Impact of No Side Effects Impact of No Side Effects Cont. I Question: How can we exploit the fact that evaluating expressions never changes the value of any other expression? I Unfortunately, run-time errors negate all the benefits we listed! I Answers: I Question: What can we do about this? I We can evaluate expressions in parallel I Solution: Type systems I We can delay evaluation until a value is actually used I Any sound type system will guarantee no run-time errors I Question: What kind of side effect can evaluating expressions still have? I Conclusion: We can only fully take advantage of functional features if we use a sound type system I Answer: They may still trigger a run-time error Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 5/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 6/31 1 The Alternative to Functional Programming Imperative Programming Languages I However, there is also an alternative (and much more I You have all used imperative programming languages common) way of programming called imperative programming I Imperative Languages: I Features of imperative programming: I FORTRAN I Side effects I ALGOL I Assignments that change the values of variables I C, C++ I Programs are sequences of statements instead of one expression I Java I Imperative programming is the dominant model I Python I This style is much closer to the way hardware executes I ... Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 7/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 8/31 Features of Imperative Languages Example Compare and Contrast I Let’s look at some example imperative programs I At a minimum, a language must have the following features to be considered imperative: I I will use C style since most of you should be familiar with this I Variables and assignments I Adding all numbers from 1 to 10 in L: I Loops and Conditionals and/or goto fun add with n = if n == 0 then 0 else n + (add (n-1)) in (n 10) I Observe that features such as pointers, recursion and arrays are optional I Here is the same program in C: int res = 0, i; I For example, FORTRAN originally only had integers and for(i=0; i < 10; i++) res += i; floats, loops, conditionals and goto statements return res; I Question: Which style do you prefer? Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 9/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 10/31 Very basic imperative programming GOTOs in Programming I All early imperative languages include goto statements I Rational: 1) Hardware supports only compare and jump I Now, let’s get even more basic and only use conditionals and instructions 2) GOTOs allow for more expressive control flow goto statements to write the same program: int res = 0, i; I Example of GOTO use: again: int i = 0; res +=i; int sum; i++; again: if(i<10) goto again; i++; return res; int z = get_input(); if(z < 0) goto error: I Which style do you prefer? n+=z; if(i < 5) goto again: return n; error: return -1; Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 11/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 12/31 2 GOTOs in Programming Cont. GOTOs in Programming Cont. I Not so long ago, it was universally accepted that GOTO statements are necessary for expressive programs I In much early (and also more recent) code, GOTO not only implemented loops but was also used for code reuse I However, as software became larger, GOTO statements started becoming problematic I Real Comment from numerical analyst: “Why bother writing a function if I can just jump to the label?” I Central Problem of GOTO: “Spagetti Code” I In 1968, Dijkstra wrote a very influential essay called “GOTO I This means that thread of execution is very hard to follow in Statement Considered Harmful” in which he argued that program text GOTO statements facilitate unreadable code and should be removed from programming languages I Jumps to a label could come from almost anyplace (in extreme cases even from other functions!) Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 13/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 14/31 The End of GOTO Side Trip: GOTO and COBOL I COBOL stands for COmmon Business Oriented Language I At first, this article was very controversial I In addition to GOTO, COBOL also includes the ALTER I But over time, most programmers started to agree that keyword GOTO constructs should be avoided I After executing ALTER X TO PROCEED TO Y, any future GOTO I Imperative programming without GOTOs is known as X means GOTO Y instead structural programming I Can change control flow structures at runtime! I But not everyone was on board... I This was marketed as allowing polymorphism Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 15/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 16/31 Side Trip: GOTO and COBOL Structured Programming I Today there is a consensus that GOTOs are not a good idea I Instead, imperative languages include many kinds of loops and I Dijkstra’s comment: “The use of branching constructs COBOL cripples the mind; its teaching should, therefore, be I Examples in C++: while, do-while, for, if, switch regarded as a criminal offense.” I One legitimate use of GOTO: Error-handling code I This popularized exceptions in most modern languages Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 17/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 18/31 3 A Simple Imperative Language Semantics of IMP1 I Let’s start by looking at at a very basic imperative language we will call IMP1: I Let’s try to give operational semantics for this language P S1; S2 → | I First, we will again use an environment E to map variables to S if(C ) then S else S fi id = e → 1 2 | their values while(C ) do S od | e id e1 + e2 e1 e2 int → | | − | I Start with the semantics of expressions C e e e = e not C C and C → 1 ≤ 2 | 1 2 | | 1 2 I Question: What do expressions evaluate to? I This language has variables, declarations, conditionals and I Answer: Integers loops I Therefore, the result (value after colon) in operational I But no pointers, functions, ... semantics rules for expression is an integer I What are some example programs in IMP1? Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 19/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 20/31 Semantics of IMP1 Semantics of IMP1 Cont. I Here are operational semantics for expressions in IMP1 (first cut) I Variable: I On to the semantics of Predicates: E v : E(id) ` I Question: What do predicates evaluate to? I Plus E e1 : v1 E ` e : v I Answer: True and False ` 2 2 E e1 + e2 : v1 + v2 ` I Therefore, the result (value after colon) in operation I Minus semantics rules for predicates is a boolean E e1 : v1 E ` e : v ` 2 2 E e e : v v ` 1 − 2 1 − 2 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 21/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 22/31 Semantics of IMP1 Cont. Semantics of Statements I Here are operational semantics for predicates in IMP1 I Now, all we have left are the statements I Less than or equal to: E e : v I However, there is one big problem: Statements do not ` 1 1 E e2 : v2 evaluate to anything! v ` v 1 ≤ 2 E e e : True ` 1 ≤ 2 I Instead, statements update the values of variables E e1 : v1 ` I In other words, they change E! E e2 : v2 v ` v 1 6≤ 2 E e e : False I Therefore, the rules for statements will produce a new ` 1 ≤ 2 I Or (slightly imprecise) shorthand environment E e1 : v1 ` I Specifically, they are of the form E S : E 0 E e2 : v2 ` ` E e1 e2 : v1 v2 ` ≤ ≤ I Changing the environment is the technical way of having side I What about the other predicates? effects in the language Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 23/31 Thomas Dillig, CS345H: Programming Languages Lecture 14: Introduction to Imperative Languages 24/31 4 Semantics of Statements Cont.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-