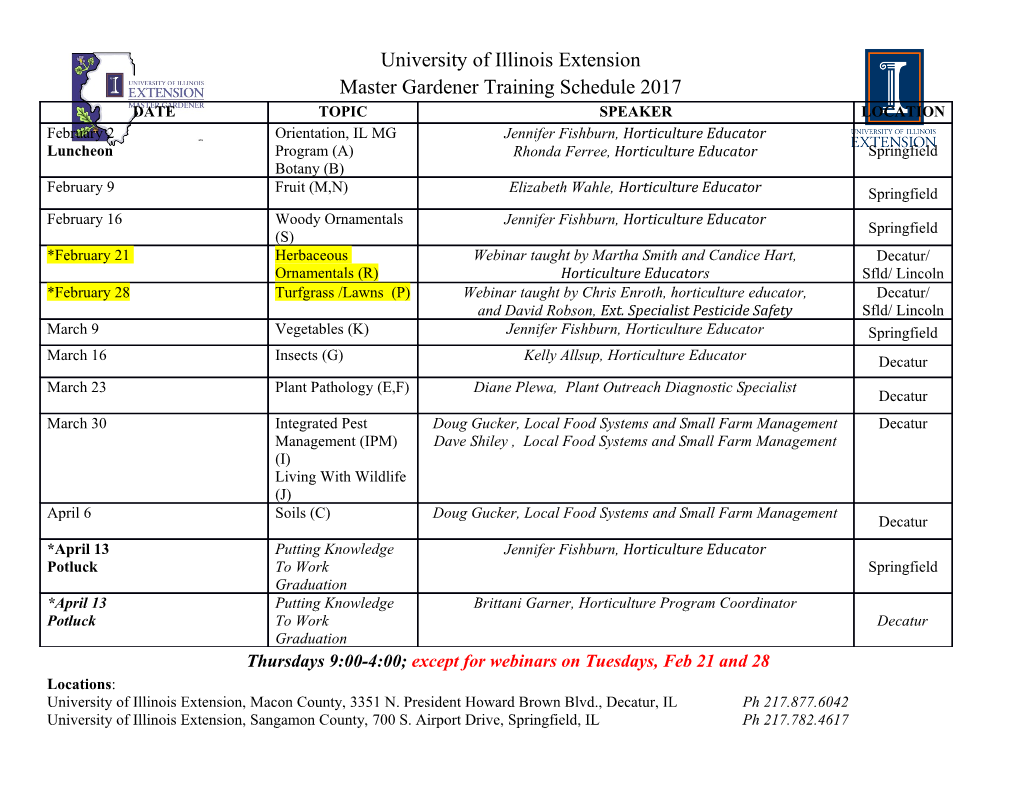
Coding Guidelines How to not shoot yourself in the foot Richard Peschke | Coding Guidelines | | Page 1 0. Use the right Tools >Use version control! >Use an IDE! >Use a debugger! Richard Peschke | Coding Guidelines | | Page 2 Richard Peschke | Coding Guidelines | | Page 3 Backup Richard Peschke | Coding Guidelines | | Page 4 General Things >C++ is most likely the worst language to start programming >We have not chosen it because it is such a nice language >If you are new to >programming start with: . Python, JavaScript or Matlab . a scripting language! . If you are teaching C++ >“STOP TEACHING C” Richard Peschke | Coding Guidelines | | Page 5 Performance >Why C++? Because Performance! >Cell phones: How to save energy? Performance! >Computer farm: How to save money? Performance! >1% of performance boost saves Facebook 100k€ in electricity Source: Ima Dethi-Sup Richard Peschke | Coding Guidelines | | Page 6 Performance >Don’t use new >Don’t use Objects features . Objects have the overhead . Fortran is the peak of constructing and deleting of computer science >Don’t use garbage evolution collection >Functions are evil . Modern computer have . Copy all code in enough memory one function to >Exceptions are avoid jumps dangerous >Comments . Don’t write errors . Every three lines Richard Peschke | Coding Guidelines | | Page 7 Stop doing Cargo Cult Programming Richard Peschke | Coding Guidelines | | Page 8 Why do I care so little about Performance? >For the next two years All your programs are just “Hello World” programs They do not need to run fast! Understanding how they work is the most important thing You are programming for the trash bin! (90% of the time) Richard Peschke | Coding Guidelines | | Page 9 How do you get Performance? >By not using your code! >If the performance has Use libraries to come from your code Copy examples from the internet >If you have a problem, somebody else had it before you! Richard Peschke | Coding Guidelines | | Page 10 Refactoring Requirements >Readability >Use an environment where you can easily jump through your code . Two button presses to get to the definition of something is to much . Creating function definitions from declaration should not be more than two buttons . The environment needs to work with symbols and not with Text . Do not use regex to search in your code >Automated Build system . Getting to the line where an error occurred must not be more expensive than one click Richard Peschke | Coding Guidelines | | Page 11 Readability Understanding your Code Refactoring Modular Design Documentation Testability Reuse Sharing Correctness Richard Peschke | Coding Guidelines | | Page 12 Performance How to get your code nice and clean >We are no professionals we are just physicists! >Nobody writes bad code on purpose! >Nobody writes good code alone! Richard Peschke | Coding Guidelines | | Page 13 How to measure code Quality? Industry standard: >someValue wtf/min >HEP: >100*someValue wtf/min >HEP solution: don’t have code reviews at all Richard Peschke | Coding Guidelines | | Page 14 How to start Before you start the computer >Write down what your code needs to do (in English or your native language) >Find specific tasks >Find the dependencies . (tasks, data, handles, hardware …) . What belongs together >Speak with somebody >Somebody else! Richard Peschke | Coding Guidelines | | Page 15 What is Programming? Debugging Documenting Planning Testing Coding Code Review Richard Peschke | Coding Guidelines | | Page 16 Organizational Stuff Organizational: >Use a version control system Git SVN >Use automatic build system . Cmake . make . MSBuild Richard Peschke | Coding Guidelines | | Page 17 Git Richard Peschke | Coding Guidelines | | Page 18 CMake Richard Peschke | Coding Guidelines | | Page 19 Design Styles Keep it Simple! >Code must be human readable! . Code that only you can read is wrong! Even if it gives reasonable results! >Correct is better than fast! >Use self-explaining names >One entity has one purpose . Every function should do exactly one thing Stay on one Level of abstraction . Do not reuse variables . Give classes specific tasks Richard Peschke | Coding Guidelines | | Page 20 Easy things to avoid >Copy and paste >Ambiguous names . If you need it twice make it a . Variable names do not need to be short function . Auto completion was invented in 1988 > >Magic numbers Raw Pointer . Make it a variable, give it a Use Shared_ptr . meaningful name! Do you really need a pointer? > >Global variables Don’t use c style cast . Use dynamic_cast or static_cast . You never need them >C style arrays . Use vector! >Avoid to write loops . Use algorithms Richard Peschke | Coding Guidelines | | Page 21 Comments Comments can be gossip Richard Peschke | Coding Guidelines | | Page 22 Comments Bad >Don’t rewrite your code in pseudo code in comments . Expect that who ever reads your code knows C++ better than you (maybe even better than English) Richard Peschke | Coding Guidelines | | Page 23 Comments Void f1(){ … 1000 lines of code Bad /* Check to see if the employee is eligible for full benefits*/ >You want to say something if((employee.flags & HOURLY_FLAG) && in English what you can’t (employee.age > 65)) say in C++ } Learn programming >Your code is a mess and Do it like this: if(employee.isEligibleForFullBenefits( )) you want to give some hints what it does Learn programming Richard Peschke | Coding Guidelines | | Page 24 Comments Void MyMonsterFunctionWithMoreThan1000lines(){ // declaring some parameter // which i need later for sure Bad ... 100 lines // Initilizing Variables >You want to say something ... 100 lines // using Variable in English what you can’t ... 100 lines say in C++ //clean up } Learn programming >Your code is a mess and Do it like this: you want to give some hints Void MyFunctionWithAUsefulName(){ what it does Object myObjectWithAMeaningfullName; Learn programming myObjectWithAMeaningfullName.Init(); useMyObjectForASpecificTask( myObjectWithAMeaningfullName) maybeDoSomethingElseWithIt( myObjectWithAMeaningfullName) } Richard Peschke | Coding Guidelines | | Page 25 Comments Good /*True if collection is the default collection for the given type. This implies that the collection is >At the library, program, or complete and unambigous. Convenient method function level, describe that checks bit BITDefault of the flag word Implements EVENT::LCCollection.*/ what bool isDefault ( ); >Inside the library, program, /* We're going to use newton's method to find the root of a number because there is no analytical or function, describe how way to solve these equations */ >At the statement level, /* We need to divide items by 2 here because describe why they are bought in pairs*/ cost = items / 2 * storePrice; Richard Peschke | Coding Guidelines | | Page 26 Magic Numbers What are magic numbers? Why they are evil? >Plain numbers that appear >You can’t search for them! somewhere in your source >You can’t make changes code >They contain unknown > HANDLE hSerial; information > hSerial = reateFile("COM1", > GENERIC_READ | GENERIC_WRITE, > 0, > 0, > OPEN_EXISTING, > FILE_ATTRIBUTE_NORMAL, 0); >What do all the zeroes mean? Richard Peschke | Coding Guidelines | | Page 27 How to avoid magic numbers On library level >If your function only accepts certain parameters Provide them alongside with the function >Think about using enums Richard Peschke | Coding Guidelines | | Page 28 How to avoid magic numbers inside a library or a program >Why is it different from global variables? Because they are const! >Use const values over preprocessor definitions . They are type safe >Group them in namespaces >Comment them where you define them! Richard Peschke | Coding Guidelines | | Page 29 How to avoid magic numbers Inside a function … >Your are using the wrong class or type These are >Use specific types for magic Numbers specific tasks … Richard Peschke | Coding Guidelines | | Page 30 Identifying Magic Numbers M agi f1(105);c Nu RAW Strings are Magic numbers too! mbe r >They are not checked by the f1({ 105,32,97, compiler r >Hard to search for 109,32,100,be um >Hard to maintain N ic117,109,98,0}); >Almost impossible to change ag M afterwards f1((char*)new int[] { 105,32,97, 109,32,100, 117,109,98,0});r be um N gic f1("i amM astupid"); mber Magic Nu Richard Peschke | Coding Guidelines | | Page 31 Magic Numbers (Real Live Experience) m yTTree "m yAxisW ithRidiculousLongN am e : m yO therAxisW ithRidiculousLongN am e > > m yFancyO utputHistogram " "M ySuperAw esom eCutVariable> 5 & & M ySuperAw esom eCutVariable< 7“ -> , "CO LZ“ ) D raw ( , Magic numbers everywhere Richard Peschke | Coding Guidelines | | Page 32 33 Page | | Where is the problem? TTrees are generic container Not possible in C++ > The types and names are not provided by the library Getting objects by there names ( as string) is a common approach for > any generic container (zB. file I/O) Richard Peschke | Coding Guidelines Dynamic typed languages overcome the problem by creating during > runtime a handler object Magic Numbers (Real Live Experience) Create your own custom class class M yCustom Tree { public: No Magic (numbers) TAxis_t G etAx1(); Anymore TAxis_t G etAx2(); private: TTree m _tree; }; TAxis_2D _t operator& (TAxis_t, TAxis_t); TAxis_2D _w ith_h2_t operator>> (TAxis_2D _t, const TH 2& ); Long_t D raw (TD raw Axis); int m ain(){ M yCustum Tree m yTree; TH 2* h2 = new TH 2D (… ); D raw ((m yTree.G etAx1()& m yTree.G etAx2())> > *h2); return 0; } Magic is heresy Richard Peschke | Coding Guidelines | | Page 34 The Three Base Types in C++ >Float (double…)
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages101 Page
-
File Size-