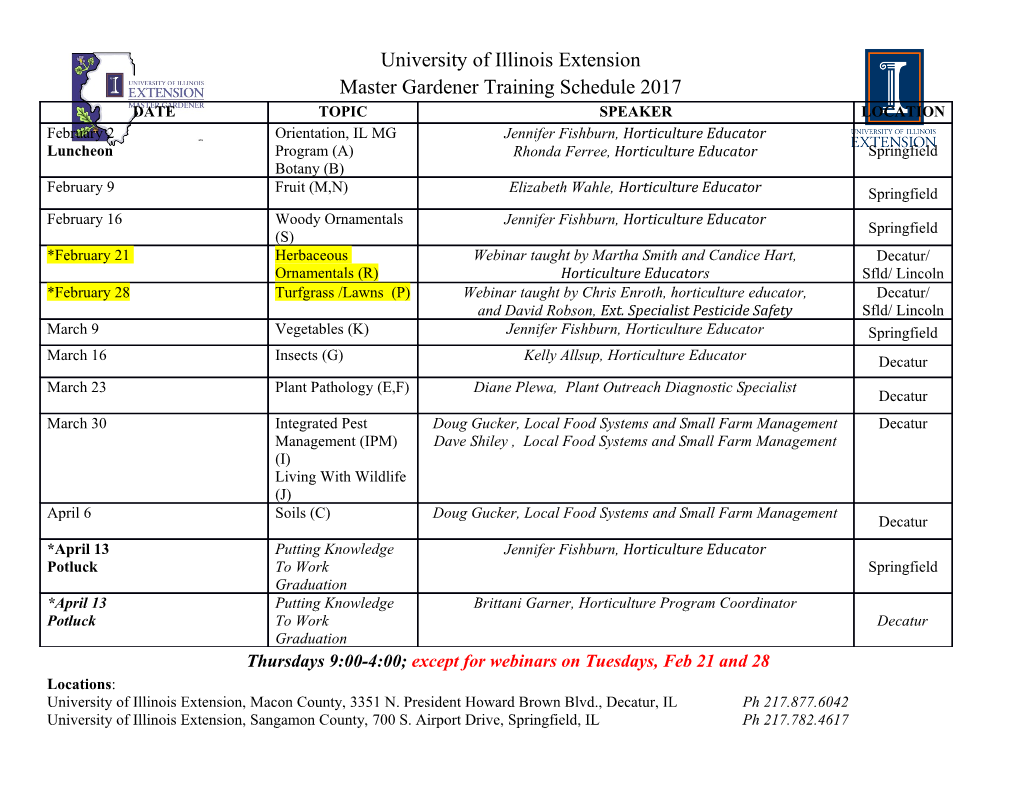
Pyomo Documentation Release 5.6.2.dev0 Pyomo Feb 06, 2019 Contents 1 Installation 3 1.1 Using CONDA..............................................3 1.2 Using PIP.................................................3 2 Citing Pyomo 5 2.1 Pyomo..................................................5 2.2 PySP...................................................5 3 Pyomo Overview 7 3.1 Mathematical Modeling.........................................7 3.2 Overview of Modeling Components and Processes...........................9 3.3 Abstract Versus Concrete Models....................................9 3.4 Simple Models.............................................. 10 4 Pyomo Modeling Components 17 4.1 Sets.................................................... 17 4.2 Parameters................................................ 23 4.3 Variables................................................. 24 4.4 Objectives................................................ 25 4.5 Constraints................................................ 26 4.6 Expressions................................................ 26 4.7 Suffixes.................................................. 31 5 Solving Pyomo Models 41 5.1 Solving ConcreteModels......................................... 41 5.2 Solving AbstractModels......................................... 41 5.3 pyomo solve Command....................................... 41 5.4 Supported Solvers............................................ 42 6 Working with Pyomo Models 43 6.1 Repeated Solves............................................. 43 6.2 Changing the Model or Data and Re-solving.............................. 46 6.3 Fixing Variables and Re-solving..................................... 47 6.4 Extending the Objective Function.................................... 48 6.5 Activating and Deactivating Objectives................................. 49 6.6 Accessing Variable Values........................................ 49 6.7 Accessing Parameter Values....................................... 51 i 6.8 Accessing Duals............................................. 51 6.9 Accessing Slacks............................................. 53 6.10 Accessing Solver Status......................................... 53 6.11 Display of Solver Output......................................... 54 6.12 Sending Options to the Solver...................................... 54 6.13 Specifying the Path to a Solver...................................... 55 6.14 Warm Starts............................................... 55 6.15 Solving Multiple Instances in Parallel.................................. 55 6.16 Changing the temporary directory.................................... 57 7 Working with Abstract Models 59 7.1 Instantiating Models........................................... 59 7.2 Managing Data in AbstractModels.................................... 61 7.3 The pyomo Command.......................................... 91 7.4 BuildAction and BuildCheck ................................... 93 8 Modeling Extensions 97 8.1 Bilevel Programming........................................... 97 8.2 Dynamic Optimization with pyomo.DAE................................ 97 8.3 MPEC.................................................. 114 8.4 Generalized Disjunctive Programming................................. 114 8.5 Stochastic Programming......................................... 116 8.6 Pyomo Network............................................. 140 9 Pyomo Tutorial Examples 153 10 Debugging Pyomo Models 155 10.1 Interrogating Pyomo Models....................................... 155 10.2 FAQ.................................................... 155 10.3 Getting Help............................................... 156 11 Advanced Topics 157 11.1 Persistent Solvers............................................. 157 11.2 rapper: a PySP wrapper......................................... 160 12 Developer Reference 167 12.1 Pyomo Expressions........................................... 167 13 Library Reference 191 13.1 AML Library Reference......................................... 191 13.2 Expression Reference.......................................... 218 13.3 Solver Interfaces............................................. 251 13.4 Model Data Management........................................ 260 13.5 The Kernel Library............................................ 263 14 Contributing to Pyomo 301 14.1 Contribution Requirements........................................ 301 14.2 Review Process.............................................. 302 14.3 Where to put contributed code...................................... 302 14.4 pyomo.contrib ............................................ 302 15 Third-Party Contributions 305 15.1 Pyomo Nonlinear Preprocessing..................................... 305 15.2 GDPopt logic-based solver........................................ 311 15.3 Multistart Solver............................................. 314 ii 15.4 parmest.................................................. 315 15.5 Pyomo Interface to MC++........................................ 327 16 Bibliography 329 17 Indices and Tables 331 18 Pyomo Resources 333 Bibliography 335 Python Module Index 337 iii iv Pyomo Documentation, Release 5.6.2.dev0 Pyomo is a Python-based, open-source optimization modeling language with a diverse set of optimization capabilities. Contents 1 Pyomo Documentation, Release 5.6.2.dev0 2 Contents CHAPTER 1 Installation Pyomo currently supports the following versions of Python: • CPython: 2.7, 3.4, 3.5, 3.6, 3.7 1.1 Using CONDA We recommend installation with conda, which is included with the Anaconda distribution of Python. You can install Pyomo in your system Python installation by executing the following in a shell: conda install-c conda-forge pyomo Pyomo also has conditional dependencies on a variety of third-party Python packages. These can also be installed with conda: conda install-c conda-forge pyomo.extras Optimization solvers are not installed with Pyomo, but some open source optimization solvers can be installed with conda as well: conda install-c conda-forge ipopt coincbc glpk 1.2 Using PIP The standard utility for installing Python packages is pip. You can install Pyomo in your system Python installation by executing the following in a shell: pip install pyomo 3 Pyomo Documentation, Release 5.6.2.dev0 4 Chapter 1. Installation CHAPTER 2 Citing Pyomo 2.1 Pyomo Hart, William E., Jean-Paul Watson, and David L. Woodruff. “Pyomo: modeling and solving mathematical programs in Python.” Mathematical Programming Computation 3, no. 3 (2011): 219-260. Hart, William E., Carl Laird, Jean-Paul Watson, David L. Woodruff, Gabriel A. Hackebeil, Bethany L. Nicholson, and John D. Siirola. Pyomo – Optimization Modeling in Python. Springer, 2017. 2.2 PySP Watson, Jean-Paul, David L. Woodruff, and William E. Hart. “PySP: modeling and solving stochastic programs in Python.” Mathematical Programming Computation 4, no. 2 (2012): 109-149. 5 Pyomo Documentation, Release 5.6.2.dev0 6 Chapter 2. Citing Pyomo CHAPTER 3 Pyomo Overview 3.1 Mathematical Modeling This section provides an introduction to Pyomo: Python Optimization Modeling Objects. A more complete description is contained in the [PyomoBookII] book. Pyomo supports the formulation and analysis of mathematical models for complex optimization applications. This capability is commonly associated with commerically available algebraic modeling languages (AMLs) such as [AMPL], [AIMMS], and [GAMS]. Pyomo’s modeling objects are embedded within Python, a full-featured, high-level programming language that contains a rich set of supporting libraries. Modeling is a fundamental process in many aspects of scientific research, engineering and business. Modeling involves the formulation of a simplified representation of a system or real-world object. Thus, modeling tools like Pyomo can be used in a variety of ways: • Explain phenomena that arise in a system, • Make predictions about future states of a system, • Assess key factors that influence phenomena in a system, • Identify extreme states in a system, that might represent worst-case scenarios or minimal cost plans, and • Analyze trade-offs to support human decision makers. Mathematical models represent system knowledge with a formalized language. The following mathematical concepts are central to modern modeling activities: 3.1.1 Variables Variables represent unknown or changing parts of a model (e.g., whether or not to make a decision, or the characteristic of a system outcome). The values taken by the variables are often referred to as a solution and are usually an output of the optimization process. 7 Pyomo Documentation, Release 5.6.2.dev0 3.1.2 Parameters Parameters represents the data that must be supplied to perform the optimization. In fact, in some settings the word data is used in place of the word parameters. 3.1.3 Relations These are equations, inequalities or other mathematical relationships that define how different parts of a model are connected to each other. 3.1.4 Goals These are functions that reflect goals and objectives for the system being modeled. The widespread availability of computing resources has made the numerical analysis of mathematical models a com- monplace activity. Without a modeling language, the process of setting up input files, executing a solver and extracting the final results from the solver output is tedious and error-prone. This difficulty is compounded in complex, large-scale real-world applications which are difficult to debug when errors occur. Additionally, there are many different formats
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages365 Page
-
File Size-