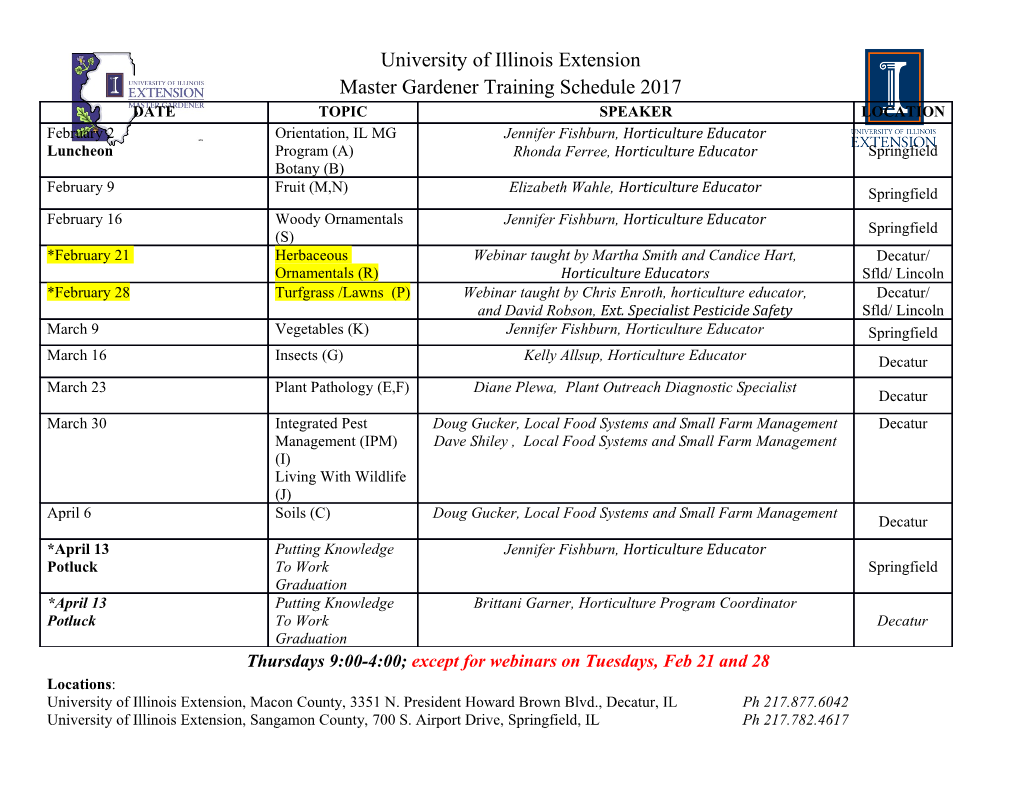
MATJUICE: A MATLAB TO JAVASCRIPT STATIC COMPILER by Vincent Foley-Bourgon School of Computer Science McGill University, Montréal A THESIS SUBMITTED TO THE FACULTY OF GRADUATE STUDIES AND RESEARCH IN PARTIAL FULFILLMENT OF THE REQUIREMENTS FOR THE DEGREE OF MASTER OF SCIENCE ©2016 Vincent Foley-Bourgon Abstract A large number of scientists, engineers, and researchers in fields as varied as physics, musicology, biology, and statistics use MATLAB daily as part of their work. These users appreciate the conciseness and expressiveness of the MATLAB language, the impressive number of powerful matrix operations and visualization functions, the easy-to-use IDE, and its interactive environment. At the same time, the web platform keeps growing and innovating. At the center of this evolution is the JavaScript language. Though it was initially used only for simple tasks in web pages such as form validation, JavaScript is today the driving technology behind extremely powerful and complex applications such as Google Maps, the diagram tool draw.io, and the presentation tool Prezi. One very desirable property of web applications is their universality; whether it’s the smart phone in our pocket, the laptop on our desk, or the powerful workstation in our lab, all these devices have a modern web browser that can execute an application on the web. The advantage for end-users is that they can use their favorite tools from the device of their choice and wherever they are without fear of compatibility issues. The developers of these applications also benefit by being able to deploy and update applications multiple times per day at a low cost. MatJuice is a tool to connect MATLAB users to the web: it automatically translates MATLAB code into JavaScript. Scientists need not spend time manually converting their applications to JavaScript, nor become experts in web technologies to publish the fruit of their labor on the web. This thesis will present MatJuice, discuss the challenges of converting from one i dynamic language to another, how to handle the differences in semantics, and how to make the output code fast. ii Résumé Un grand nombre de scientifiques, ingénieurs et chercheurs dans des domaines variés tels que la physique, la musicologie, la biologie et les statistiques utilisent MATLAB dans le cadre de leur travail quotidien. Ces utilisateurs apprécient la concision et l’expressivité du langage MATLAB, le nombre impressionnant d’opérations matricielles et de fonctions de visualisation, la facilité d’utilisation et l’interactivité de l’environnement de travail. En même temps, le web continue de grandir et d’innover. Au centre de cette fulgurante évolution, on trouve le langage JavaScript. Bien qu’il était initialement utilisé pour des tâches simples telles que la validation d’un formulaire, JavaScript est aujourd’hui la technologie qui fait marcher des applications puissantes et complexes telles que Google Maps, le dessinateur de diagrammes draw.io et l’outil de présentation, Prezi. Une qualité fort désirable des applications web est leur universalité ; qu’il s’agisse du téléphone intelligent dans notre poche, du portable sur notre bureau ou encore de la puissante station de travail dans notre laboratoire, toutes ces machines ont accès à un fureteur moderne duquel on peut exécuter des applications sur le web. L’avantage pour les utilisateurs est qu’ils peuvent utiliser leurs outils favoris à partir de l’appareil de leur choix et où il le veulent sans crainte de problèmes de compatibilité. Les développeurs de ces applications bénéficient aussi en étant capable de déployer et de mettre à jour leurs applications plusieurs fois par jour et à moindre coût. MatJuice est un outil qui connecte les utilisateurs de MATLAB au web : il permet la traduction automatique de code MATLAB en JavaScript. Les scientifiques n’ont ainsi pas à passer du temps précieux à faire la conversion manuelle de leurs programmes vers JavaScript, ni à iii devenir des experts en technologies web pour publier le fruit de leur labeur sur le web. Cette thèse présente MatJuice, discute des défis de la conversion d’un langage dynamique vers un autre, comment gérer les différentes sémantiques des deux langages et comment rendre le code généré rapide. iv Acknowledgements Thank you Prof. Laurie Hendren for helping me make this project as good as it could be. Thank you for your patience, your constant encouragement and for fostering a friendly and relaxed atmosphere in your lab. Thank you Prof. Clark Verbrugge for taking time out of your sabatical to review my thesis. Thank you members of the Sable lab for the work we did together, and more importantly for the laughs we shared: Erick Lavoie, Hanfeng Chen, Prabhjot Sandhu, Rahul Garg, Faiz Khan, Sameer Jagdale, Lei Lopez, Valerie Saunders Duncan, Sujay Kathrotia, Vineet Kumar, Matthieu Dubet, Alex Krolik, Ismail Badawi, Xu Li. Thank you students of COMP-520 and COMP-302 that I had the pleasure to T.A. for your hard work and for making me experience the most rewarding job I ever held. Merci Maman et Papa pour votre support inconditionnel et votre amour. v vi Table of Contents Abstracti Résumé iii Acknowledgementsv Table of Contents vii List of Figures xi 1 Introduction1 1.1 Contributions.................................3 1.2 Organization..................................4 2 Background5 2.1 McLab.....................................5 2.2 Tamer.....................................6 2.2.1 TameIR................................7 2.2.2 Analysis framework.........................8 2.2.3 Analyses...............................8 2.3 JastAdd....................................9 2.4 JavaScript...................................9 2.4.1 Typed arrays............................. 10 2.4.2 sweet.js................................ 10 vii 3 MatJuice compiler structure 11 3.1 Overview................................... 11 3.2 Data representation.............................. 13 3.3 TameIR to JavaScript............................. 14 3.3.1 Functions............................... 14 3.3.2 Assignment statements........................ 15 3.3.3 Flow control statements....................... 18 3.3.4 Operators............................... 22 3.4 Standard library................................ 23 3.4.1 sweet.js................................ 26 4 Points-to analysis 29 4.1 Motivation................................... 29 4.2 Motivating example.............................. 32 4.2.1 Input function parameters...................... 32 4.2.2 Aliasing statements.......................... 33 4.2.3 Output function parameters...................... 34 4.2.4 Summary............................... 35 4.3 Points-to analysis............................... 36 4.3.1 Transformation............................ 39 4.3.2 Analysis-transformation loop..................... 40 4.3.3 Output Parameters Copy....................... 41 5 Rich points-to abstraction 43 5.1 Motivating example: part two......................... 44 5.2 Analysis components............................. 46 5.3 Implementation in MatJuice......................... 51 5.3.1 Representation of points-to sets................... 51 5.3.2 Memory site cache.......................... 51 5.3.3 New TameIR statement........................ 52 6 Copy insertion 55 viii 6.1 Copy insertion................................. 55 6.2 Points-to-based Copy Insertion........................ 57 6.2.1 General process............................ 57 6.2.2 Local variables............................ 58 6.2.3 Output parameters.......................... 59 6.3 Copying input parameters........................... 60 6.3.1 Analysis............................... 60 6.3.2 Transformation............................ 60 6.3.3 Possible improvements........................ 61 6.4 MatJuice implementation........................... 62 6.4.1 No implicit returns.......................... 63 6.4.2 Local variables and output parameters................ 63 6.4.3 Input parameters........................... 64 7 Copy insertion evaluation 65 7.1 Instrumentation and methodology...................... 66 7.1.1 Instrumentation of MatJuice..................... 66 7.1.2 Instrumentation of GNU Octave................... 67 7.1.3 Benchmark suite........................... 67 7.1.4 Methodology............................. 67 7.2 Naive copy vs. copy insertion......................... 68 7.3 Comparison with Octave........................... 71 7.4 Conclusion.................................. 72 8 Performance evaluation 73 8.1 Benchmarks.................................. 73 8.1.1 Executing the benchmarks...................... 73 8.2 Experimental setup.............................. 74 8.3 Results..................................... 75 8.3.1 High-performance numeric routines................. 76 8.3.2 Costly array accesses......................... 77 ix 9 Related Work 81 9.1 Other MATLAB compilers.......................... 81 9.1.1 MATLAB Coder............................ 81 9.1.2 FALCON............................... 82 9.1.3 Mc2For................................ 82 9.1.4 MiX10................................ 82 9.1.5 Differences with MatJuice...................... 83 9.2 Compilers targetting JavaScript........................ 84 9.3 Numerical libraries for JavaScript...................... 84 9.3.1 McNumJS.............................. 85 9.3.2 Ndarray................................ 85 9.3.3 numeric.js............................... 85 10 Conclusion and Future Work 87 10.1 Conclusion.................................. 87 10.2 Future work.................................. 88 Bibliography
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages108 Page
-
File Size-