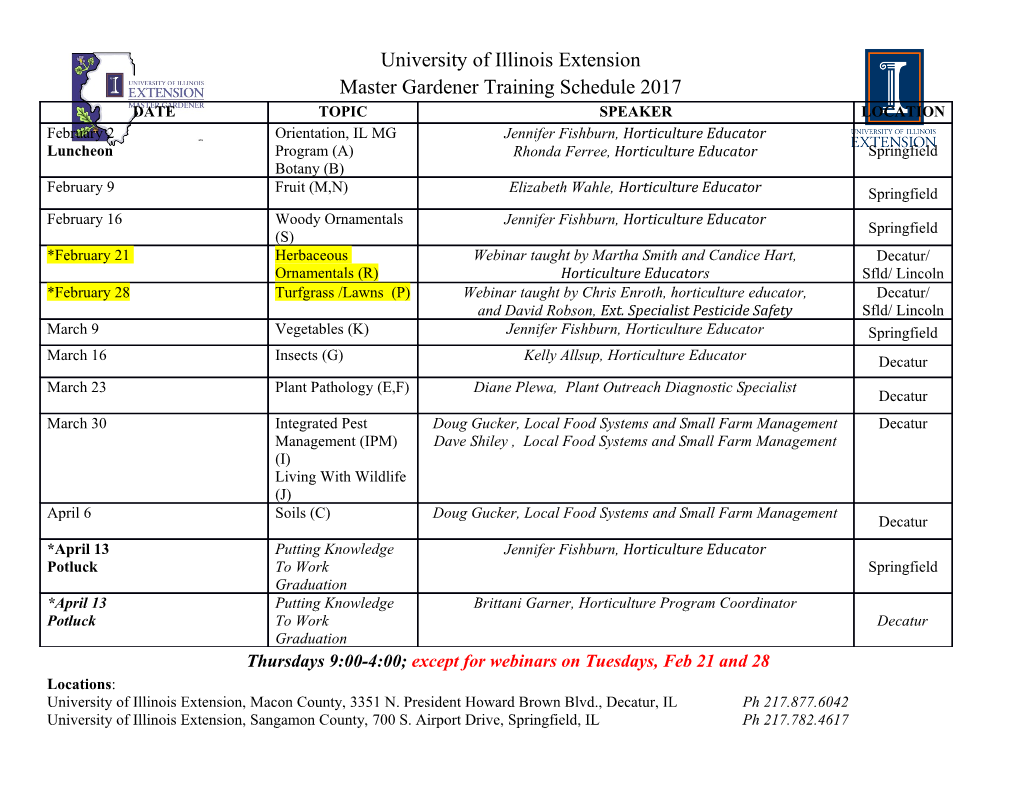
Introducing Java’s Enumerated Type J. Mohr University of Alberta, Augustana Campus, 4901 46 Ave., Camrose, Alberta, Canada T4V 2R3 [email protected] Abstract— The enumerated types that were introduced in associativity or of operators with arity other than binary. Java 5.0 provide a way to treat arithmetic operators as enu- Of the textbooks cited above, only Weiss [10] and Kruse meration constants with an eval method that is customized and Ryba [11] discuss right associativity (the right-to-left for each operator, allowing us to take an object-oriented evaluation of operators like exponentiation), and only the approach to applying an operator to its arguments, using latter textbook deals with both unary and binary operators. dynamic dispatch instead of case logic, when implementing a Kruse and Ryba are also unique in their use calculator or an arithmetic expression evaluator. When used of an enumeration for token types, including enum in conjunction with variable arity methods (or varargs, constants such as operand, unaryop, binaryop, also introduced in Java 5.0) or by passing an operand rightunaryop, leftparen and rightparen.1 stack as the single argument to an operator’s eval method, The introduction of enumerated types in Java 5.0 (release an enumeration for operators can be extended to handle 1.5) provided the opportunity to treat arithmetic operators operators of differing arities using a single abstract eval as enumeration constants with an apply or eval method method in the enumerated type. Such an example gives that is customized for each operator. This allows us to an opportunity to expose our students to Java’s version of take an object-oriented approach to applying an operator enumerated types by using an Operator enumeration in to its arguments, using dynamic dispatch instead of case an assignment on infix expression parsing and evaluation. logic through constant-specific method implementations [12, p. 152]. This also provides a good opportunity to expose our Keywords: Enumerated types, enum, expression evaluation, Java, students to Java’s version of enumerated types in conjunction variable arity parameters with the discussion of arithmetic expression parsing and evaluation. 1. Introduction Arithmetic expression evaluation is a topic of study in 2. Enumerated Types many data structures or algorithms courses and is covered in several data structures and a few algorithms textbooks. In Niklaus Wirth introduced the enumerated type in Pascal some cases, the evaluation of postfix expressions is intro- (1970) as a user-defined ordinal type that “specifies an duced as part of the discussion of stacks [1]–[5]; in others, ordered set of values by enumerating the constant identifiers the evaluation of expression trees is discussed when binary which denote the values” [13, Ch. 5]. It associated the trees are introduced [1]–[3], [6]–[8]. Some textbooks present first constant listed with the value 0; the second, with 1; the subject of infix-to-postfix conversion in conjunction with and so on. It provided a high-level, application-oriented expression evaluation [3], [6], [9], [10]. Kruse and Ryba binding construct [14] that improved program readability and [11] dedicate a chapter (Ch. 13, pp. 594–645) to a case reduced errors by replacing ad hoc constructs such as study on Polish notation, including the evaluation of prefix const and postfix expressions and the translation from infix form Mon = 0; to reverse Polish (postfix) form. Smith [5] shows how to Tue = 1; combine infix-to-postfix translation with postfix expression ... evaluation to evaluate infix expressions using two stacks; Sun = 6; Koffman and Wolfgang [6] present the same algorithm in an end-of-chapter programming project. with Most textbooks that treat the topic of arithmetic expression type Day = (Mon, Tue, Wed, Thu, Fri, evaluation confine their coverage to the evaluation of binary Sat, Sun); operators, which is especially appropriate if the topic is presented as part of the discussion of binary trees. Those that 1Lambert and Osborne [3] give an example of a parser that uses a discuss the conversion of infix expressions to their postfix switch statement with case labels such as PLUS, MINUS, MUL, DIV and L_PAR, but these are implemented as defined constants rather than equivalents usually include the handling of parentheses and enum constants, an ad hoc approach that will be discussed below as the operator priorities, but there is typically no discussion of int enum pattern [12]. The relational operators can be used to compare values the static methods valueOf(String) and values() of Pascal enumerated types, and the predefined functions are implicitly declared; the former returns the enumeration ord(X), pred(X), and succ(X) return the ordinal num- constant corresponding to a name, and the latter returns all ber, predecessor, and successor of X, respectively. A for the constants of the enumerated type [24, §8.9]. statement can iterate over the values of an enumeration (or Java enumerated types can have constructors, instance data a subrange of them), and enumeration constants can be used fields, and methods. The combination of a constructor, an as selectors in a case statement and to index arrays. instance field and an accessor can be used to associate values An important feature of Pascal’s enumerated types is that other than the default ordinals with enumeration constants, they are typesafe: each enumerated type is different from as illustrated in this example from the Java Language and incompatible with all other types, including integers and Specification, 3e [24, §8.9]: other enumerated types. The compiler can enforce strong public enum Coin { typing by ensuring that only the enumerated values of an PENNY(1), NICKEL(5), DIME(10), enumerated type can be assigned to a variable of that type QUARTER(25); or passed as a parameter to a function or procedure that Coin(int value) {this.value = value;} is defined to accept an argument of that enumerated type. private final int value; Pascal’s enumerated types are also secure: given the decla- public int value() {return value;} ration of type Day above, succ(Sun) and pred(Mon) } are compile-time errors. An enum type may not be declared abstract, but Subsequent languages introduced variations of the enu- it may include an abstract method if all the enumeration merated type. Several languages, including C++ and Ada, constants have class bodies that provide concrete imple- allow the ordinal values of enumeration constants to be mentations of the method. This feature can be used to specified, as in implement an enumeration of arithmetic operations [24, enum coins {penny = 1, nickel = 5, §8.9] [12, p. 152], as shown in Figure 1. This simple example dime = 10, quarter = 25}; deals only with binary operators, but Java’s enumerated type facility can easily be used to implement nullary, unary, Ada and C# allow enumerations to be overloaded (i.e., the and ternary operators as well as binary operators, and to same literal can be used in the declaration of more than one distinguish between left- and right-associative operators and enumeration in the same scope); C# requires that overloaded between prefix and postfix operators. names be fully qualified, but Ada allows the type prefix to be omitted if the compiler can infer it from the context [15]. 3. An Enumerated Type for Arithmetic Java did not include support for an enumeration type until release 1.5 (Java 5.0, 2004). Prior to its introduction, many Operators of Various Arities Java programmers used the ad hoc solution that is referred 3.1 A Simple Enumeration for Arities int enum pattern to as the [12]: The first step in supporting operators of various arities is public static final int MON = 0; to add an Arity enumerated type as a nested type within public static final int TUE = 1; an Operator enumeration: ... public enum Arity { public static final int SUN = 6; NULLARY, UNARY, BINARY, TERNARY, Several writers suggested ways of implementing typesafe GROUPING enumerations using Java classes [16]–[20]. In its “Taxonomy } of Problems in Teaching Java” [21], the ACM Java Task Implementing an Operator enumeration is like design- Force identified the lack of enumerated types as a “weakness ing a little language [25]: we can decide what operations in the Java type system.” can be included in arithmetic expressions that use our The enum type introduced in Java 5.0 shifted the concept Operator class to process them. The Arity enumeration of an enumerated type away from a set of integer constants above allows us to support unary operators such as factorial toward the concept of classes that is central to object- (‘!’) and percent (‘%’) and single-argument functions (such oriented programming [22, p. 112]. A Java enum type is as abs, sqrt, sqr, trunc, round, log, lg, ln, sin, a class of which “the possible values of the enumeration cos, and exp or pow); binary operators such as exponenti- are the only possible instances of the class” [23, p. 257]. ation (‘ˆ’) and modulus (mod) in addition to the usual ‘+’, All enum types are implicitly subclasses of the predefined ‘-’, ‘*’, and ‘/’; and ternary operators such as C and Java’s class Enum, from which they inherit toString, equals, conditional operator ‘?:’ and variations on that operator compareTo, hashCode, ordinal (which returns the such as pos, neg, and zero. zero-based position of the constant in its enum declaration), The NULLARY arity is provided for pseudo-operators such and a few other methods. In addition, for each enum type, as constants (e and pi) and no-argument functions such public enum Operation { PLUS { double apply(double x, double y) {return x + y;} }, MINUS { double apply(double x, double y) {return x - y;} }, TIMES { double apply(double x, double y) {return x * y;} }, DIVIDE { double apply(double x, double y) {return x / y;} }; abstract double apply(double x, double y); } Fig.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-