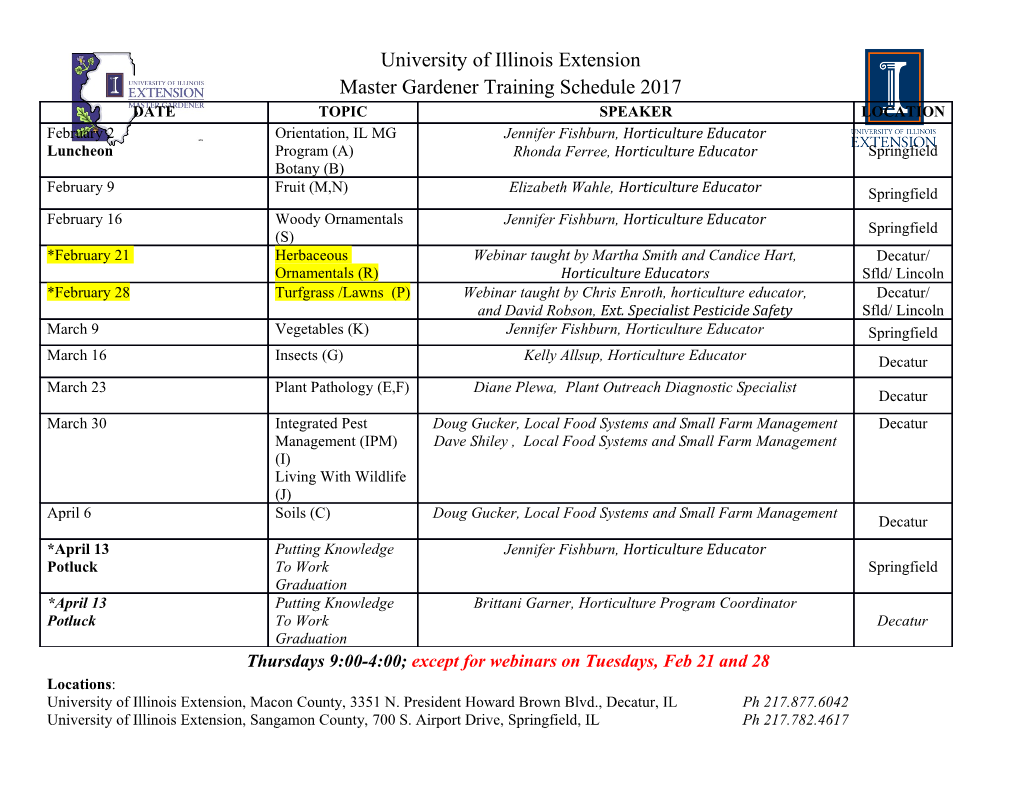
A comprehensive step-by-step guide Programming in Scala Martin Odersky Lex Spoon artima Bill Venners Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Programming in Scala PrePrint™ Edition Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index iii Thank you for purchasing the PrePrint™ Edition of Programming in Scala. A PrePrint™ is a work-in-progress, a book that has not yet been fully written, reviewed, edited, or formatted. We are publishing this book as a PrePrint™ for two main reasons. First, even though this book is not quite finished, the information contained in its pages can already provide value to many readers. Second, we hope to get reports of errata and suggestions for improvement from those readers while we still have time incorporate them into the first printing. As a PrePrint™ customer, you’ll be able to download new PrePrint™ versions from Artima as the book evolves, as well as the final PDF of the book once finished. You’ll have access to the book’s content prior to its print publication, and can participate in its creation by submitting feedback. Please submit by clicking on the Suggest link at the bottom of each page. Thanks for your participation. We hope you find the book useful and enjoyable. Bill Venners President, Artima, Inc. Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Programming in Scala PrePrint™ Edition Martin Odersky, Lex Spoon, Bill Venners artima ARTIMA PRESS MOUNTAIN VIEW,CALIFORNIA Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index v Programming in Scala PrePrint™ Edition Version 2 Martin Odersky is the creator of the Scala language and a professor at EPFL in Lausanne, Switzerland. Lex Spoon worked on Scala for two years as a post-doc with Martin Odersky. Bill Venners is president of Artima, Inc. Artima Press is an imprint of Artima, Inc. P.O. Box 390122, Mountain View, California 94039 Copyright © 2007, 2008 Martin Odersky, Lex Spoon, and Bill Venners. All rights reserved. PrePrint™ Edition first published 2007 Version 2 published February 18, 2008 Produced in the United States of America 12 11 10 09 08 2 3 4 5 6 No part of this publication may be reproduced, modified, distributed, stored in a retrieval system, republished, displayed, or performed, for commercial or noncommercial purposes or for compensation of any kind without prior written permission from Artima, Inc. All information and materials in this book are provided "as is" and without warranty of any kind. The term “Artima” and the Artima logo are trademarks or registered trademarks of Artima, Inc. All other company and/or product names may be trademarks or registered trademarks of their owners. Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index to Nastaran - M.O. to Fay - L.S. to Siew - B.V. Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Overview Contents viii Preface xvi Acknowledgments xvii Introduction xx 1. A Scalable Language 27 2. First Steps in Scala 47 3. Next Steps in Scala 63 4. Classes and Objects 90 5. Basic Types and Operations 114 6. Functional Objects 136 7. Built-in Control Structures 151 8. Functions and Closures 169 9. Control Abstraction 190 10. Composition and Inheritance 205 11. Traits and Mixins 233 12. Case Classes and Pattern Matching 249 13. Packages and Imports 279 14. Working with Lists 292 15. Collections 322 16. Stateful Objects 342 17. Type Parameterization 362 18. Abstract Members and Properties 379 19. Implicit Conversions and Parameters 396 20. Implementing Lists 413 21. Object Equality 424 22. Working with XML 438 23. Actors and Concurrency 450 24. Extractors 461 25. Objects As Modules 473 26. Annotations 484 27. Combining Scala and Java 490 28. Combinator Parsing 502 Glossary 530 Bibliography 544 About the Authors 546 Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Contents Contents viii Preface xvi Acknowledgments xvii Introduction xx 1 A Scalable Language 27 1.1 A language that grows on you ................ 28 1.2 What makes Scala scalable? ................. 33 1.3 Why Scala?.......................... 36 1.4 Scala’s roots.......................... 44 1.5 Conclusion .......................... 45 2 First Steps in Scala 47 Step 1. Learn to use the Scala interpreter.............. 47 Step 2. Define some variables.................... 49 Step 3. Define some functions ................... 51 Step 4. Write some Scala scripts.................. 55 Step 5. Loop with while, decide with if . 57 Step 6. Iterate with foreach and for . 59 Conclusion ............................. 62 3 Next Steps in Scala 63 Step 7. Understand the importance of vals............. 63 Step 8. Parameterize Arrays with types .............. 65 Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Contents ix Step 9. Use Lists and Tuples ................... 69 Step 10. Use Sets and Maps..................... 74 Step 11. Understand classes and singleton objects......... 79 Step 12. Understand traits and mixins ............... 86 Conclusion ............................. 89 4 Classes and Objects 90 4.1 Objects and variables..................... 91 4.2 Mapping to Java........................ 93 4.3 Classes and types....................... 96 4.4 Fields and methods...................... 97 4.5 Class documentation .....................102 4.6 Variable scope.........................104 4.7 Semicolon inference .....................108 4.8 Singleton objects .......................110 4.9 A Scala application......................111 4.10 Conclusion ..........................113 5 Basic Types and Operations 114 5.1 Some basic types .......................115 5.2 Literals ............................116 5.3 Operators are methods ....................121 5.4 Arithmetic operations.....................125 5.5 Relational and logical operations . 126 5.6 Object equality ........................128 5.7 Bitwise operations ......................130 5.8 Operator precedence and associativity . 131 5.9 Rich wrappers.........................133 5.10 Conclusion ..........................134 6 Functional Objects 136 6.1 A class for rational numbers . 136 6.2 Choosing between val and var . 138 6.3 Class parameters and constructors . 139 6.4 Multiple constructors.....................140 6.5 Reimplementing the toString method . 141 6.6 Private methods and fields ..................142 Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Contentsx 6.7 Self references ........................143 6.8 Defining operators ......................143 6.9 Identifiers in Scala ......................145 6.10 Method overloading......................147 6.11 Going further .........................148 6.12 A word of caution.......................149 6.13 Conclusion ..........................150 7 Built-in Control Structures 151 7.1 If expressions.........................152 7.2 While loops..........................154 7.3 For expressions........................156 7.4 Try expressions........................161 7.5 Match expressions ......................164 7.6 Living without break and continue . 166 7.7 Conclusion ..........................168 8 Functions and Closures 169 8.1 Methods............................169 8.2 Nested functions .......................171 8.3 First-class functions......................172 8.4 Short forms of function literals . 175 8.5 Placeholder syntax ......................175 8.6 Partially applied functions ..................177 8.7 Closures............................181 8.8 Repeated parameters .....................184 8.9 Tail recursion.........................185 8.10 Conclusion ..........................189 9 Control Abstraction 190 9.1 Reducing code duplication ..................190 9.2 Simplifying client code....................194 9.3 Currying............................196 9.4 Writing new control structures . 198 9.5 By-name parameters .....................201 9.6 Conclusion ..........................204 Prepared for jacques weiss Cover· Overview· Contents· Discuss· Suggest· Glossary· Index Contents xi 10 Composition and Inheritance 205 10.1 Introduction..........................205 10.2 Abstract classes........................206 10.3 The Uniform Access Principle . 207 10.4 Assertions and assumptions..................209 10.5 Subclasses...........................210 10.6 Two name spaces, not four ..................211 10.7 Class parameter fields.....................213 10.8 More method implementations . 214 10.9 Private helper methods ....................215 10.10Imperative or functional?...................216 10.11Adding other subclasses ...................218 10.12Override modifiers and the fragile base class problem . 219 10.13Factories............................221 10.14Putting it all together .....................222 10.15Scala’s class hierarchy ....................225 10.16Implementing primitives ...................229 10.17Bottom types .........................231 10.18Conclusion ..........................232 11 Traits and Mixins 233 11.1 Syntax.............................233 11.2 Thin versus thick interfaces..................235 11.3 The standard Ordered trait..................236 11.4 Traits for modifying interfaces . 238 11.5 Stacking modifications ....................241 11.6 Locking and logging queues . 242 11.7 Traits versus multiple inheritance . 245 11.8 To trait, or not to trait? ....................247
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages547 Page
-
File Size-