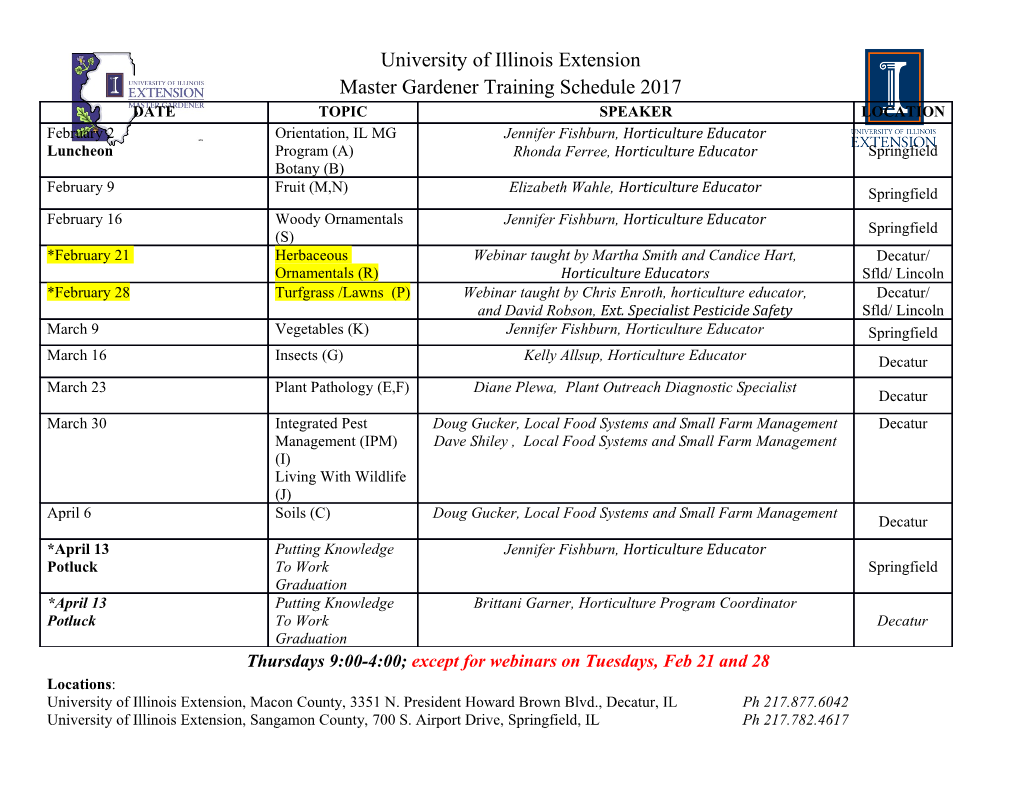
Vu Nguyen Implementation of Linux GPIO Device Driver on Raspberry Pi Platform Helsinki Metropolia University of Applied Sciences Bachelor of Engineering Information Technology Bachelor’s Thesis 10 May 2014 Abstract Author(s) Vu Nguyen Title Implementation of Linux GPIO Device Driver on Raspberry Pi Platform Number of Pages 48 pages + 3 appendices Date 10 May 2014 Degree Bachelor of Engineering Degree Programme Information Technology Specialisation option Embedded Engineering Instructor(s) Markku Nuutinen, Project supervisor The project was aimed at implementing a General Purpose Input/Output (GPIO) device driver for the Raspberry Pi model B rev 2.0 platform. Specific attention was given to im- plement the device driver based on the Linux character device driver. Each of the GPIO pins on Raspberry Pi is exposed to userspace for use by a device file in the /dev directory. While a dynamically assigned major number was used to identify the device driver associated with the GPIO device, a minor number was used by the kernel to differentiate between GPIO pins that the device driver controls. Four entry points were implemented to handle low-level hardware GPIO resources on behalf of system calls re- quested by a userspace application. Besides, interrupt handling was implemented as an experimental feature to see how a device driver supports interrupt in an embedded Linux system. The GPIO device driver could be used on the Raspberry Pi platform by loading it either as a kernel module or as an integral component of the Linux kernel. One or more userspace processes could open a device file for reading the logic level of a GPIO pin, controlling the direction of a GPIO pin, and setting its logic level to high state or low state. Besides, inter- rupt handling feature was tested; the result showed that more than one process could share an interrupt resource on a GPIO pin and could disable interrupt on that GPIO pin. There still remain, however, some limitations to the features offered by the driver. Interrupt handling is still an experimental feature. More work needs to be done to make the feature useful for use. Furthermore, the GPIO character device driver is deprecated with a newer version of the Linux kernel by the gpio-sysfs driver. Keywords Linux GPIO driver, Raspberry Pi, Linux character driver, GPIO kernel module Contents 1 Introduction 1 2 Theoretical Background on the Embedded Linux System of the Project 2 2.1 Raspberry Pi Model B Rev 2.0 Platform 2 2.2 Overview of Raspberry Pi GPIO Pins 3 2.3 Raspbian Linux Distribution 5 2.4 Linux Character Device Driver 8 3 Design of the GPIO Device Driver 11 3.1 Project Setup 11 3.2 Design of Per-device Structure and Initialization and Exit Routines 16 3.3 Design of the Entry Points for the GPIO Device Driver 17 3.4 Design of Interrupt Handling Functions 22 4 Implementation of the GPIO Device Driver 23 4.1 Linux Kernel Compilation and Installation 23 4.2 Implementation of Per-device Structure and Initialization and Exit Routines 26 4.3 Implementation of the Entry Points for the GPIO Device Driver 30 4.4 Implementation of Interrupt Handling Functions 35 5 Testing of the GPIO Device Driver 37 5.1 Testing Preparation 37 5.2 Testing of Output Functionality 37 5.3 Testing of Input Functionality 39 5.4 Testing of Interrupt Functionality 42 6 Results and Discussion 45 7 Conclusion 47 References 48 Appendices Appendix 1. Source code for GPIO Linux device driver Appendix 2. Source code for output testing of GPIO device driver Appendix 3. Source code for input testing of GPIO device driver List of Abbreviations API Application programming interface DHCP Dynamic host configuration protocol DMM Digital multimeter DNS Domain name system FAT File allocation table GCC GNU compiler collection GND Ground GPIO General-purpose input/output GPU Graphics processing unit I2C Inter-integrated circuit IC Integrated circuit IDE Integrated development environment IO Input/ouput IP Internet protocol IPC Inter-process communication LAN Local area network LED Light-emitting diode LTS Long term support MAC Media access control PC Personal computer PLD Programmable logic device PWM Pulse-width modulation SD Secure digital SoC System on chip SPI Serial peripheral interface SSH Secure shell UART Universal asynchronous receiver/transmitter USB Universal serial bus UTP Unshielded twisted pair VFS Virtual filesystem 1 1 Introduction Nowadays Linux is an operating system of choice for many computing systems ranging from personal computers (PC), servers, mainframe computers and supercomputers to electronic devices known as embedded systems. An embedded system is a computing system which is specifically designed to carry out a set of dedicated tasks, and it typi- cally uses a custom microcontroller as its main processing unit. Linux is a highly porta- ble operating system; it can run on a variety of hardware architectures such as x86, PowerPC, MIPS, H8, SPARC, or ARM, to name just a few. This portability also comes with limitations, however. It is no doubt that while the modu- lar architecture of Linux makes it easy when porting to different types of architectures, a great deal of effort is required to build new kernel components in order to support a target platform. The real hard work of these efforts is to develop device drivers for a particular target platform. A device driver (kernel module) is a piece of software that consists of a set of low-level interfaces, and is designed to control a hardware device. The Raspberry Pi platform is an example of a target device that Linux can be ported to run on it. GPIO device driver is one of the kernel components that can be developed to support the Raspberry Pi platform. The goal of this project was to implement a GPIO device driver for Raspberry Pi. Specifically, attention was given to the implementation of the GPIO device driver based on Linux character device drivers. The intended result of this work was to give a deeper understanding on the Raspberry Pi platform, to learn what a Linux device driver does and how it works, and finally to implement a GPIO character device driver for the Raspberry Pi platform from scratch. 2 2 Theoretical Background on the Embedded Linux System of the Project 2.1 Raspberry Pi Model B Rev 2.0 Platform The Raspberry Pi model B rev 2.0 platform was chosen for the project to implement the Linux GPIO device driver. This is due to the fact that Raspberry Pi is a low-cost Linux computer which costs approximately $35 from the Farnell distributor. The platform is widely used by hobbyists and professional embedded developers around the world. Therefore, there is a large support from the community when it comes to getting help or information. For convenience, Raspberry Pi will be used when referring to the Raspber- ry Pi model B rev 2.0 platform throughout this report. Figure 1 shows the Raspberry Pi platform used in the project. Figure 1. Raspberry Pi model B rev 2.0 platform Reprinted from Raspberry Pi Wiki (2014) [1] Raspberry Pi is a fan-less embedded computer which is equipped with a wide range of peripherals such as Universal Serial Bus (USB), Ethernet, Universal Asynchronous Receiver/Transmitter (UART), High-Definition Multimedia Interface (HDMI), Serial Pe- ripheral Interface (SPI), Inter-Integrated Circuit (I2C), audio codec and so on. The heart of the platform is a Broadcom BCM2835 System on Chip (SoC) that has an ARM1176JZF-S 700 MHz core processor, an integrated graphics processor VideoCore IV Graphic Processing Unit (GPU), 512MB of RAM for model B rev 2.0, and an SD 3 card in place of a hard disk drive [2]. The platform is powered from an external power supply via a USB micro connector. It is recommended from the official Raspberry Pi website that the board be powered from a good-quality power supply that gives at least 700mA at 5V [3]. This is because if the board is powered by a USB micro connector, there will not be enough current and, as a result, the board my reboot sometimes when it draws too much power. 2.2 Overview of Raspberry Pi GPIO Pins Raspberry Pi comes with a 26-pin connector called P1 and an 8-pin connector called P5. The pins on these connectors expose to users a set of buses such as I2C, SPI, Pulse Width Modulation (PWM), and signals. There are eight GPIO pins on P1 con- nector and four GPIO pins on P5 connector. In addition, the P1 connector has an I2C interface which consists of two pins, a 5-wire SPI interface, and a serial interface with two pins. These pins can be configured to be GPIO pins, thus making the total number of GPIO pins on Raspberry Pi to be 21 pins. A point worth mentioning here is that four GPIO pins on the P5 connector are available only on the Raspberry Pi model B rev 2.0 platform. Figure 2. Mapping between BCM2835 GPIO pins and Raspberry Pi P5 connector Modified from Henderson (2013) [4] Figure 2 shows the mapping between Broadcom BCM2835 SoC's GPIO pins and the Raspberry Pi P5 connector. The P5 connector which consists of eight pins as seen in 4 figure 2 is viewed from the bottom side of the board. Since Raspberry Pi was originally shipped without any header on P5 connector, a double sided 2.54mm header has to be soldered either on top or at the bottom of the board for use. Figure 3. Mapping between BCM2835 GPIO pins and Raspberry Pi P1 connectors Modified from Henderson (2013) [4] As can be seen from figure 3, the pins on the P1 connector, on the other hand, are viewed looking at the platform from above with the USB micro connector at the top-left to the Raspberry Pi and P1 connector to the top-right of the platform.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages70 Page
-
File Size-