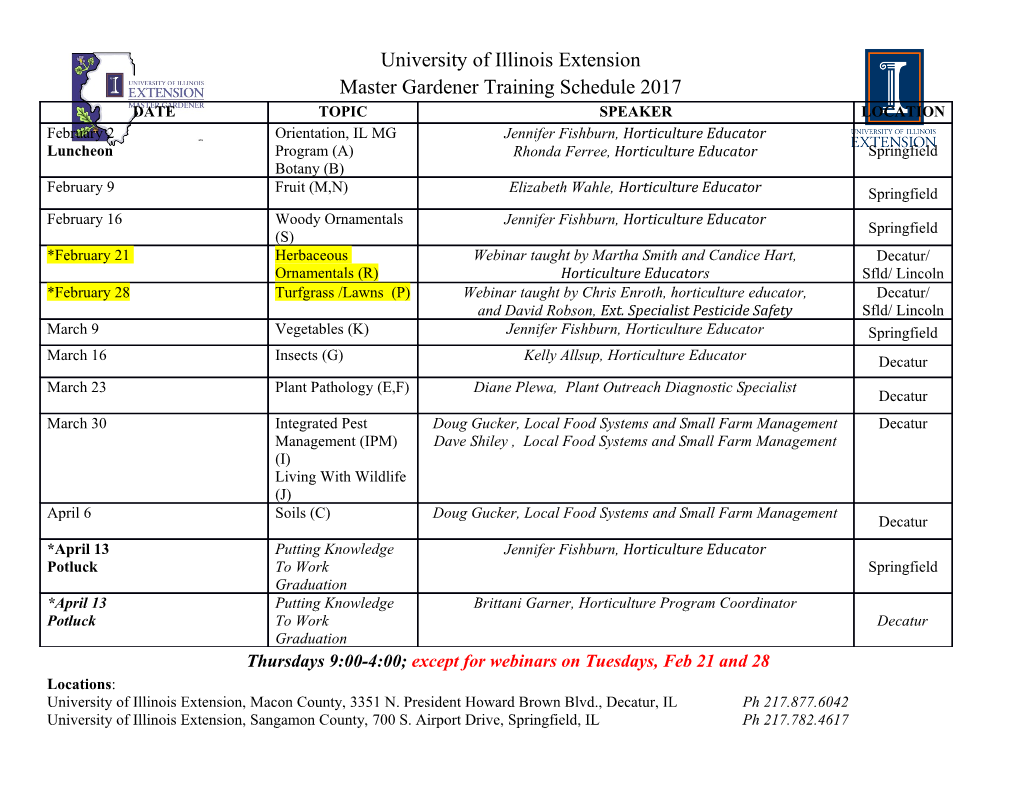
Python { AN IntroduCtion . default parameters . self documenting code Example for extensions: Gnuplot Hans Fangohr, [email protected], CED Seminar 05/02/2004 ² The wc program in Python ² Summary Overview ² Outlook ² Why Python ² Literature: How to get started ² Interactive Python (IPython) ² M. Lutz & D. Ascher: Learning Python Installing extra modules ² ² ISBN: 1565924649 (1999) (new edition 2004, ISBN: Lists ² 0596002815). We point to this book (1999) where For-loops appropriate: Chapter 1 in LP ² ! if-then ² modules and name spaces Alex Martelli: Python in a Nutshell ² ² while ISBN: 0596001886 ² string handling ² ¯le-input, output Deitel & Deitel et al: Python { How to Program ² ² functions ISBN: 0130923613 ² Numerical computation ² some other features Other resources: ² . long numbers www.python.org provides extensive . exceptions ² documentation, tools and download. dictionaries Python { an introduction 1 Python { an introduction 2 Why Python? How to get started: The interpreter and how to run code Chapter 1, p3 in LP Chapter 1, p12 in LP ! Two options: ! All sorts of reasons ;-) interactive session ² ² . Object-oriented scripting language . start Python interpreter (python.exe, python, . power of high-level language double click on icon, . ) . portable, powerful, free . prompt appears (>>>) . mixable (glue together with C/C++, Fortran, . can enter commands (as on MATLAB prompt) . ) . easy to use (save time developing code) execute program ² . easy to learn . Either start interpreter and pass program name . (in-built complex numbers) as argument: python.exe myfirstprogram.py Today: . ² Or make python-program executable . easy to learn (Unix/Linux): . some interesting features of the language ./myfirstprogram.py . use as tool for small sysadmin/data . Note: python-programs tend to end with .py, processing/collecting tasks but this is not necessary. On Unix/Linux: best if ¯rst line in ¯le reads #!/usr/bin/env python Note: Python programs are interpreted (but use ² platform independent byte-code, as Java) Python { an introduction 3 Python { an introduction 4 Interactive Python (IPython) Note: installing extra Python programs/modules small tool (written in Python) ² The standard Python distribution contains many provides \more interactive" interpreter ² modules and tools. command and variable name completion ² The standard procedure for installation of additional browsing of objects ² Python modules or stand-alone programs written in easy access to help Python is: ² command line history stays over di®erent sessions ² unpack tar or zip ¯le other (more advanced tools), such as very simple ² ² pro¯ling etc cd into new subdirectory ² run python setup.py install ² IPython can be downloaded from ipython.scipy.org. (This is the equivalent to the normal ./configure; make; make install in UNIX, and a double-click on an icon in MS Windows.) Python { an introduction 5 Python { an introduction 6 Lists . Fetch the ¯rst element from end of list: >>>a[-1] #same as a[len(a)-1] Chapter 2, p44 in LP ! -400 . Change an element in list important data structure, similar to vector in >>>a[0]=20 ² MATLAB Access parts of the list (using \slicing") ² Creating and extending lists Chapter 2, p44 in LP ² ! . Creation of empty list . Leave out ¯rst element >>>a=[] >>>a[1:] . Adding elements [20, 45, 50, -400] >>>a.append( 10 ) . show ¯rst three elements only . Or initialise with several values: >>>a[:3] >>>a=[10, 20, 45, 50, -400] [10, 20, 45] . Leave out ¯rst and last element length of a list is given by len (but don't need >>>a[1:-1] ² this often) [10, 20, 45] >>>len(a) Elements (objects) in a list can be of any type, 5 ² including lists . animals = ['cat','dog','mouse'] Accessing (and changing) individual elements ² . wired mix = ['ape', 42, [0,1,2,3,4], 'spam'] . Access individual elements (indexing as in C, starting from 0): >>>a[0] 10 Python { an introduction 7 Python { an introduction 8 The range(a,b,d) command returns a list ranging For-loop ² from a up-to but not including b in increments of d. (a defaults to 0, d defaults to 1): Chapter 3, p87 in LP ! >>>range(4,8) #similar to MATLAB's #colon-operater 4:7 For-loops are actually for-each-loops [4, 5, 6, 7] ² For-loops iterate (generally) over lists >>>range(8) [0, 1, 2, 3, 4, 5, 6, 7] Typical example, increasing counter ² More methods for lists including sorting, for i in range(5): ² reversing, searching, inserting Chapter 2, p46 in LP print i ! Example: >>> a.sort() This is equivalent to: >>> a=range(-10,10,2) >>> a for i in [0, 1, 2, 3, 4]: [-10, -8, -6, -4, -2, 0, 2, 4, 6, 8] print i >>> a.reverse() >>> a [8, 6, 4, 2, 0, -2, -4, -6, -8, -10] >>> a.sort() Compare with MATLAB equivalent: >>> a [-10, -8, -6, -4, -2, 0, 2, 4, 6, 8] for i=0:4 %this is MATLAB code >>> disp( i ) %this is MATLAB code end %this is MATLAB code Python { an introduction 9 Python { an introduction 10 Grouping of statements is done by indentation. if-then ² . sounds strange (at ¯rst) . very easy to get used to Chapter 3, p77 in LP ! . enforces code that is easy to read if-then Example: print list of strings ² ² . Conventional way (for-loop) a=10 animals=['dog','cat','mouse'] b=20 for i in range( len(animals) ): print animals[i] if a == b: print "a is the same as b" Output: dog cat if-then-else mouse ² . Use for-each-loop a=10 animals=['dog','cat','mouse'] b=20 for animal in animals: print animal if a == b: print "a is the same as b" else: print "a and be are not equal" Python { an introduction 11 Python { an introduction 12 Modules and namespaces While Chapter 5, p126 in LP ! Chapter 3, p84 in LP ! Lots of libraries for python (called \modules") example 1: ² ² . string-handling . maths a=1 . more maths (LA-pack, ®tw, ...) while a<100: a = a*2 . data bases . XML print "final a =",a . Output: final a = 128 access by importing them ² example 2: determine the square root of a using Example: for simple maths ² 1 a pa = lim xn and xn+1 = xn + ² n 2 ³ xn ´ . Either !1 import math #only once at start of prog. a = 2.0 # want to find sqrt(a) a = math.sqrt( 2 ) x = a # take x a as a first guess for pa ¼ . or import into current namespace while abs( x**2 - a ) > 1e-5: from math import sqrt x = 0.5*( x + a / x ) a = sqrt( 2 ) print x . Can also be lazy: from math import * Output: 1.41421568627 Python { an introduction 13 Python { an introduction 14 example 3: determine the square root of a, but Strings ² store xn in list: Chapter 2, p35 in LP ! a = 2 x = [a] # use x for sqrt_a use singe (') or double (") quotes, or triple ² # create list with a as 1st entry double (""") quotes as limiters: while abs( x[-1]**2 - a ) > 1e-5: . a = "string 1" x.append( 0.5*( x[-1] + a / x[-1]) ) . a = 'string 1' . a = """string 1""" print x Handy to include quotes in string ² Output: [2, 1.5, 1.4166666666666665, 1.4142156862745097] matlab help = "in Matlab the single quote ' is used a lot" matlab help = """... the single quote "'" is used a lot""" \Slicing" and indexing as for lists: ² >>> text = "this is a test." >>> text[-1] '.' >>> text[:] 'this is a test.' >>> text[:10] 'this is a ' >>> text[4:-4] ' is a t' Python { an introduction 15 Python { an introduction 16 Simple conversion functions available print is the general purpose command for ² ² printing >>> str(10) #convert 10 into a string . '10' separate objects to print by comma (automatic >>> int(str(10)) #and back to an int conversion to string): >>> dogs = 10 10 >>> cats = 2 >>> float(str(10)) #and back to a float >>> print cats,"cats and",dogs,"dogs" 10.0 2 cats and 10 dogs >>> complex('10') #and convert to complex number (10+0j) . add comma at the end of print-statement to avoid new line Can concatenate strings using + . Explicit formatting similar to C's printf-syntax: ² >>> a = "this is"; b="a test" >>> print "%d cats and %d dogs" % ( cats, dogs ) >>> c = a + " " + b >>> c 'this is a test' Variety of string processing commands ² (replace, ¯nd, count, index, split, upper, lower, swapcase, . ) To ¯nd out available methods for a string, try in ipython: >>> a="test" >>> a. [and then press tab] Python { an introduction 17 Python { an introduction 18 File input/output File conversion example Convert comma-separated data given in radian to Chapter 9, p249 in LP space separated data in degree Example: print ¯le line by line ! ² Input Output phi,sin(phi) f = open('printfile.py','r') #open file 0,0,rad 0 0.0 deg 0.2,0.198669,rad 0.2 11.3828952201 deg lines = f.readlines() #read lines, 0.4,0.389418,rad 0.4 22.3120078664 deg #lines is list 0.6,0.564642,rad 0.6 32.3516035358 deg 0.8,0.717356,rad 0.8 41.1014712084 deg f.close() #close file 1,0.841471,rad 1 48.2127368827 deg 1.2,0.932039,rad 1.2 53.4019010416 deg for line in lines: Python programme print line, # comma means: don't do linefeed import string, math filename = "data.csv" #input file Example: print ¯le word by word in one line f = open( filename, 'r' ) #open ² lines = f.readlines() #read f = open('printfile.py','r') f.close() #close lines = f.readlines() g = open( filename[0:-4]+".txt", "w") #open output file f.close() for line in lines[1:]: #skip first line (header) import string bits = string.split( line, ',' ) #separate columns into list #use comma as separator for line in lines: # now convert numerical value from rad to deg #split splits line into words.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-