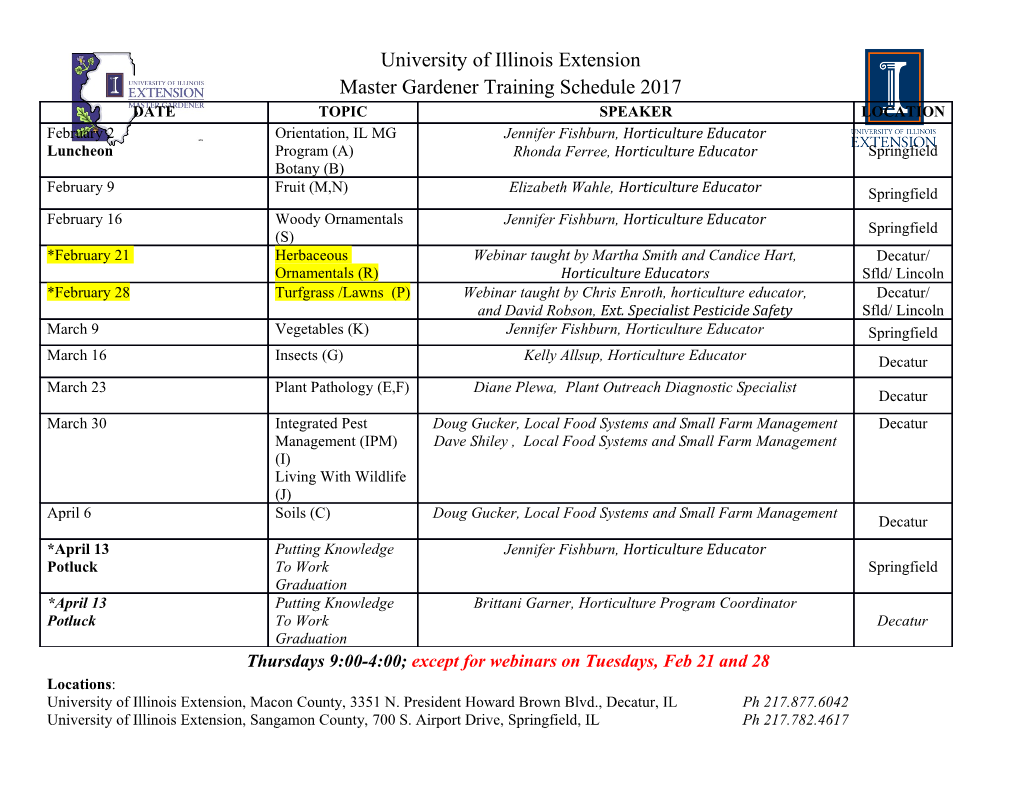
C# Game Programming: For Serious Game Creation Daniel Schuller Course Technology PTR A part of Cengage Learning Australia • Brazil • Japan • Korea • Mexico • Singapore • Spain • United Kingdom • United States C# Game Programming: † 2011 Course Technology, a part of Cengage Learning. For Serious Game Creation ALL RIGHTS RESERVED. No part of this work covered by the copyright herein may Daniel Schuller be reproduced, transmitted, stored, or used in any form or by any means graphic, electronic, or mechanical, including but not limited to photocopying, recording, Publisher and General Manager, scanning, digitizing, taping, Web distribution, information networks, or Course Technology PTR: information storage and retrieval systems, except as permitted under Section 107 Stacy L. Hiquet or 108 of the 1976 United States Copyright Act, without the prior written Associate Director of Marketing: permission of the publisher. Sarah Panella Manager of Editorial Services: For product information and technology assistance, contact us at Heather Talbot Cengage Learning Customer & Sales Support, 1-800-354-9706 Marketing Manager: Jordan For permission to use material from this text or product, Castellani submit all requests online at cengage.com/permissions Further permissions questions can be emailed to Senior Acquisitions Editor: Emi [email protected] Smith Project Editor: Jenny Davidson Visual Studio 2010 Express Web installer is a registered trademark of Technical Reviewer: James E. Perry Microsoft Corporation in the United States and other countries. Interior Layout Tech: MPS Limited, TortoiseSVN is a trademark of CollabNet, Inc. sfxr was created by A Macmillan Company DrPetter. NUnit is a copyright of NUnit.org. Bitmap Font Creator is a Cover Designer: Mike Tanamachi copyright of andreas jo¨ nsson. CD-ROM Producer Brandon Penticuff All other trademarks are the property of their respective owners. Indexer: Broccoli Indexing Services All images † Cengage Learning unless otherwise noted. Proofreader: Sara Gullion Library of Congress Control Number: 2010922097 ISBN-13: 978-1-4354-5556-6 ISBN-10: 1-4354-5556-8 eISBN-10:1-4354-5623-8 Course Technology, a part of Cengage Learning 20 Channel Center Street Boston, MA 02210 USA Cengage Learning is a leading provider of customized learning solutions with office locations around the globe, including Singapore, the United Kingdom, Australia, Mexico, Brazil, and Japan. Locate your local office at: international.cengage.com/region Cengage Learning products are represented in Canada by Nelson Education, Ltd. For your lifelong learning solutions, visit courseptr.com Visit our corporate website at cengage.com Printed in the United States of America 1234567121110 This book is dedicated to Garfield Schuller and Stanley Hyde. Acknowledgments Many thanks to everyone who has helped with this book and to everyone who developed the wonderful libraries and tools that it uses. Thanks to Jenny Davidson, the book’s project editor, for all her help making my writing clearer and ensuring that everything was finished in time. Thanks to my technical editor, Jim Perry, who caught my many coding mistakes and provided valuable suggestions and advice. I would also like to thank my family and coworkers for their support and helpful input. iv About the Author Daniel Schuller is a British-born computer game developer who has worked and lived in the United States, Singapore, Japan, and is currently working in the United Kingdom. He has released games on the PC as well as the Xbox 360 and PlayStation 3. Schuller has developed games for Sony, Ubisoft, Naughty Dog, RedBull, and Wizards of the Coast, and maintains a game development website at http://www.godpatterns.com. In addition to developing computer games, Schuller also studies Japanese and is interested in Artificial Intelligence, cognition, and the use of games in education. v Contents Introduction . ................................................xii PART I BACKGROUND ........................................1 Chapter 1 The History of C# . ....................................3 C# Basics ...................................................... 3 Versions of C# ...............................................5 C#2.0....................................................5 Generics ............................................... 5 Anonymous Delegates ................................... 9 C#3.0...................................................10 LINQ ................................................. 10 Lambda Functions...................................... 12 Object Initializers ...................................... 12 Collection Initializers ................................... 12 Local Variable Type Inference and Anonymous Types. ....... 13 C#4.0...................................................14 Dynamic Objects ....................................... 14 Optional Parameters and Named Arguments............... 15 Summary .................................................... 17 Chapter 2 Introducing OpenGL ..................................19 Architecture of OpenGL........................................ 21 Vertices—The Building Blocks of 3D Graphics ...................21 vi Contents vii The Pipeline ................................................22 OpenGL Is Changing........................................... 24 OpenGL ES .................................................25 WebGL . ...................................................25 OpenGL and the Graphics Card.................................. 25 Shaders—Programs on the Graphics Card .......................27 The Tao Framework ........................................... 28 Summary .................................................... 30 Chapter 3 Modern Methods .....................................31 Pragmatic Programming ....................................... 31 Game Programming Traps....................................32 KISS .......................................................32 DRY.......................................................32 C++.....................................................36 Source Control..............................................37 Using Source Control ......................................39 Unit Testing ................................................40 Test Driven Development ..................................44 Unit Testing in C# .........................................44 Summary .................................................... 44 PART II IMPLEMENTATION . .................................47 Chapter 4 Setup ...............................................49 Introducing Visual Studio Express—A Free IDE for C# . ............ 49 A Quick Hello World. ........................................50 Visual Studio Express Tips ....................................53 Automatic Refactoring and Code Generation .................53 Renaming............................................. 54 Creating Functions ..................................... 56 Separating Chunks of Code . ............................ 57 Shortcuts . .............................................60 Subversion, an Easy Source Control Solution ...................... 60 Where to Get It .............................................60 Installation.................................................60 Creating the Repository ......................................60 Adding to the Repository ....................................64 History . ...................................................67 viii Contents Extending Hello World.......................................68 Tao......................................................... 69 NUnit ....................................................... 71 Using NUnit with a Project ...................................72 Running Tests ..............................................75 An Example Project. .........................................76 Summary .................................................... 80 Chapter 5 The Game Loop and Graphics...........................81 How Do Games Work? ......................................... 81 A Closer Look at the Game Loop ..............................82 Implementing a Fast Game Loop in C# . ......................... 82 Adding High-Precision Timing . ..............................89 Graphics ..................................................... 91 Full Screen Mode. .........................................94 Rendering .................................................96 Clearing the Background...................................96 Vertices. ...............................................98 Triangles. ...............................................99 Coloring and Spinning the Triangle . .......................101 Summary ................................................... 103 Chapter 6 Game Structure . ..................................105 The Basic Pattern of a Game Object ............................. 105 Handling Game State ......................................... 106 Game State Demo ............................................ 112 Setting the Scene with Projections .............................. 114 Form Size and OpenGL Viewport Size .........................114 Aspect Ratio...............................................116 The Projection Matrix.......................................117 2D Graphics ...............................................117 Sprites...................................................... 120 Positioning the Sprite.......................................124 Managing Textures with DevIl . .............................125 Textured Sprites
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages443 Page
-
File Size-