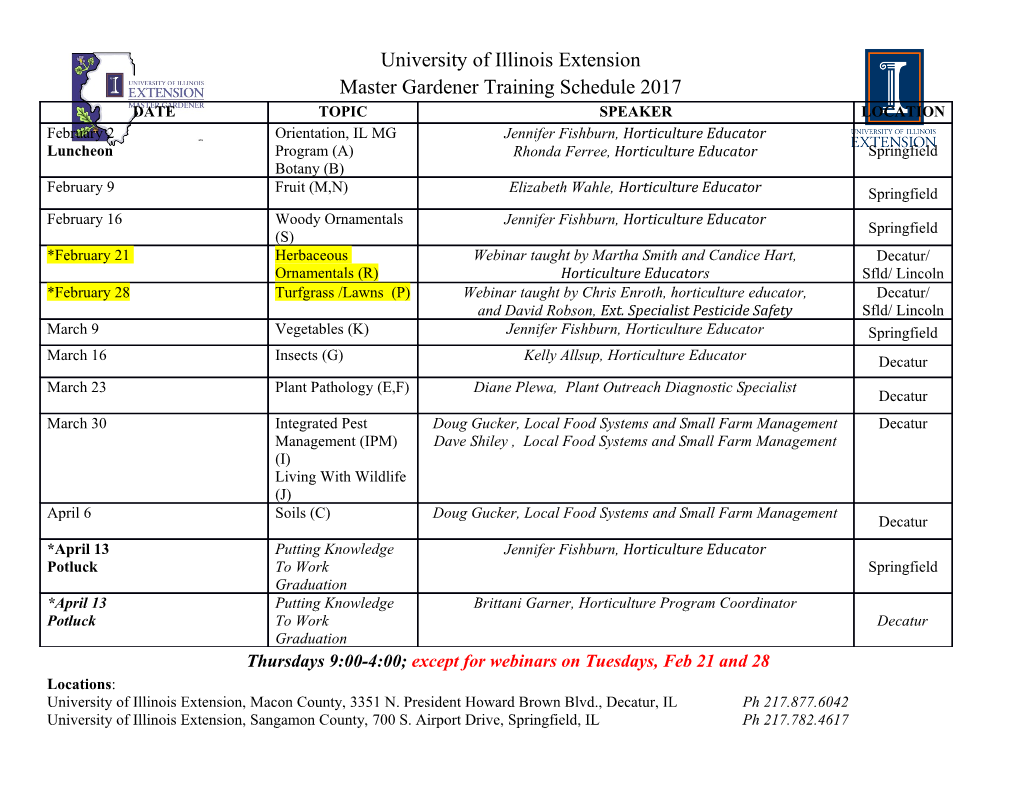
Solid Shape Drawing on the Commodore 64 by Richard Rylander omputer graphics is one of the easy-to-use graphics capabilities to fastest growing areas of com­ the masses. The “ masses,” however, Cputer software and hardware may still find the Macintosh pricey, development. The old saying that especially if they just want to play “ one picture is worth a thousand around a bit with MacPaint. Low- words” couldn’t be truer when you cost computers, such as the Commo­ consider the effectiveness of a pie dore 64, while lacking the slick chart or bar graph versus columns of graphic interaction of the Mac, are numbers. Even the simplest of home selling for such ridiculously low computers is capable of at least this prices ($150 at the time o f writing basic form of computer graphics. But this article) that they are hard to pass the term computer graphics usually up. They have the potential for some brings to mind more exciting images fairly sophisticated graphics, but so than just mundane business far most of the commercially avail­ applications. able software has done little more Special visual effects for motion than add the usual “ point plotting” pictures and some television adver­ and “ line drawing” commands. tisements are now being done with This article describes a software computers to create scenes o f “ en­ package that will allow you to create hanced reality.” W e see spacecraft your own computer-generated making impossible manuevers or scenes, such as “ Coffee and Donuts” shimmering corporate logos “ flying in Figure 1 (page 55) and “ Goblets” Pumping pixels requires tight, efficient code. Here are halftone shading, backlighting and other visual effects in a tight little package. by” in ways that would be difficult to in Figure 2 (page 55). You can con­ simulate with conventional photo­ struct images from combinations of graphic techniques. The enormous elementary shapes (spheres, cylin­ amount of data and processing neces­ ders, and toroids in various orienta­ sary to produce such images has tions) or by the “ polygon mesh” tech­ made the use of “ super computers” nique typically used to render more essential. Even with the largest com­ complex objects. You can produce puters working full time on the task, drawings with realistic shading ef­ only a few feet of film are produced fects and in a variety o f styles to per day. For home computer owners make some surprisingly detailed pic­ who want to create their own com­ tures with a minimum of effort. The puter-generated masterpiece, howev­ package includes demonstration pro­ er, the task is not hopeless. grams to illustrate how you use each The Apple Macintosh represents a graphic function and style option. major step in bringing powerful, In keeping with the “ running light without overbyte” theme of DDJ, the Richard Rylander, 179 TV. McKnight entire graphics package fits in 3K of Rd. Apt. 203, St. Paul, M N 55119. RAM. The program sits in an area of Dr. Dobb’s Journal, May 1985 50 Q C 7 RAM that is inaccessible to BASIC (a arithmetic routines. Because we will in the code. The multiplication rou­ 4K block following the BASIC ROM) draw our shaded shapes on a high- tine also contains a special case to so that you don’t lose any useful pro­ resolution display of 320 x 200 treat a single-precision number as a gram space. Commodore supplies a points, we need only single-precision signed integer, which is then squared. “ DOS Wedge” program that occu­ arguments for most functions and The integer-square-root routine pies the last 1K of this block, and one double-precision results for some in­ deserves some special comment. o f my objectives in the design of the termediate values. Have you ever tried the BASIC graphics package was to maintain The first two routines, multiplying square-root function? If you have, compatibility with this useful utility. two single-precision numbers to yield you know it takes about 52 millisec­ The compactness of the code re­ a double-precision product and divid­ onds. A t this rate, plotting the 64,000 quired me to leave out some “ bells ing a double-precision dividend by a pixels o f a 320 x 200 screen would and whistles,” but I ’ve made no sacri­ double-precision divisor to yield a take about 3,328 seconds, or nearly fices to execution speed or user-inter- double-precision quotient, are fairly an hour, if we need a square root per face ease. The main omission is error standard routines that should need no point! O f course, we don’t need a trapping— the software performs no description other than the comments square root for each point, but it is an checking to ensure that you use only “ legal” point coordinates and so on. This may make program develop­ ment a little more difficult, but once you have written and debugged a pro­ gram properly, error checking be­ comes extraneous and only slows pro­ gram execution. W e must address several general tasks in developing a shape-drawing program. The first is, o f course, how to calculate the apparent brightness for all points on visible surfaces o f ob­ jects. Next, how do we display these different brightness levels on a high- resolution display that is basically on or off? Finally, a “ general” task, but detailed in nature, is creating the spe­ cific software tools that will let us do the required calculations and bit-map manipulations. The program itself is written in machine language, because even with our simplifying restrictions, the plot­ ting of each pixel still involves consid­ erable computation. The program uses integer arithmetic throughout, and we utilize all the symmetry avail­ able to eliminate redundant calcula­ tions. The main program actually consists of five separate subprograms to break the process into manageable pieces and to provide a library o f sep­ arate utilities that you may find use­ ful in other programs. Before getting into the problem of shade calculation, I’ll present the first subprogram, which is a collection of integer arith­ metic utilities that all the later sub­ programs need. Integer Arithmetic Utilities Listing One (page 61) provides the source code for a set o f four integer 51 Dr. Dobb's Journal, May 1985 essential part o f many shade calcula­ Kaner and John R. Vokey in the June and Y screen coordinates (w e take X tions. Obviously, anything we can do 1984 issue o f M IC R O ). A gain , modulo 8 and Y modulo 8) with the to speed up this particular function though, because we are interested Y bits shifted to become the upper will have a great effect on the overall only in generating random bytes, we three bits. This effectively repeats the program speed. can use a much faster routine. threshold pattern over the entire bit­ The BASIC square-root routine is The method we use is to store two map area. For each point within an slow because BASIC is written to save previous random bytes, exclusive OR object to be drawn, we calculate a space, not time. Virtually all small them bit by bit, and rotate the result shade value normalized to the range BASICs find a square root by first to the right by one bit to generate a 0-63 and then compare it to the taking the log o f the argument, divid­ new random byte. Because it needs threshold value at that screen point to ing that result by 2, and then expon­ no multiply or divide operations, pro­ decide whether to plot or unplot. entiating. Because LOG and EXP vides a fairly uniform distribution of If we have, for example, a large functions are already available, why random bytes, and has a long period, area where the shade value is con­ use any more space than necessary to this process is fast. Note that even stant at 31 (a 50 percent gray), then derive SQR? though we are only generating ran­ within each 8 X 8-pixel character One way to speed up this function dom bytes (0-255), the pattern or se­ cell block, half of the threshold values is to use Newton’s method, an itera­ quence o f successive bytes doesn’t re­ will be greater than or equal to the tive procedure that can give full float­ peat until after 35,805 calls to the shade value, turning on half the pix­ ing-point precision in less time. Be­ random-number-generator routine els. The order in which the values 0 to cause we are interested only in (fo r the initial parameters used in the 63 are arranged in the threshold table integer results, we can do much bet­ program). The long period is neces­ determines how these on pixels are ter yet, though. sary to ensure that we won’t produce distributed. A common pattern for The method we actually use is es­ any unwanted secondary patterns in these so called ordered-dither matri­ sentially as fast as doing a single-di­ what should be “ randomly” shaded ces is a recursive arrangement such vide operation. We construct the root, images— the human eye is adept at as that shown in Table 1 (page 53). If bit by bit, in a manner similar to the picking out such correlations. that matrix is subdivided into quar­ way we would take a decimal square Now that some low-level number ters, sixteenths, and so on, each sub­ root by hand using pencil and paper crunching is out of the way, we can matrix has the same general pattern, (does anyone remember how to do concentrate on the graphics aspects with offsets between submatrices.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages22 Page
-
File Size-